WordPress is an outdated CMS, but in addition the most used one. Because of its historical past of supporting out of date PHP variations and legacy code, it nonetheless lacks in enforcing trendy coding practices — WordPress abstraction is one instance.
For example, it’ll be such a lot higher to separate the WordPress core codebase into programs controlled by means of Composer. Or most likely, to autoload WordPress categories from document paths.
This text will educate you summary WordPress code manually and use summary WordPress plugin features.
Problems With Integrating WordPress and PHP Gear
Because of its historical structure, we on occasion stumble upon issues when integrating WordPress with tooling for PHP codebases, such because the static analyzer PHPStan, the unit check library PHPUnit, and the namespace-scoping library PHP-Scoper. For example, imagine the next circumstances:
- Prematurely of WordPress 5.6 with make stronger for PHP 8.0, a file by means of Yoast described how working PHPStan on WordPress core would produce thousands of issues.
- Because of its nonetheless supporting PHP 5.6, WordPress check suites lately only support PHPUnit up to version 7.5, which has reached the tip of lifestyles.
- Scoping WordPress plugins by means of PHP-Scoper is very challenging.
The WordPress code inside our initiatives will best be a fragment of the entire; the assignment will even include industry code agnostic of the underlying CMS. But, simply by having some WordPress code, the assignment won’t combine with tooling correctly.
As a result of this, it would make sense to separate the assignment into programs, a few of them containing WordPress code and others having best industry code the usage of “vanilla” PHP and no WordPress code. This fashion, those latter programs received’t be suffering from the problems described above however will also be completely built-in with tooling.
What Is Code Abstraction?
Code abstraction gets rid of fastened dependencies from the code, generating programs that have interaction with each and every different by means of contracts. Those programs can then be added to other programs with other stacks, maximizing their usability. The results of code abstraction is a cleanly decoupled codebase in accordance with the next pillars:
- Code towards interfaces, now not implementations.
- Create programs and distribute them by means of Composer.
- Glue all portions in combination by means of dependency injection.
frame a.novashare-ctt{show:block;background:#00abf0;margin:30px auto;padding:20px 20px 20px 15px;colour:#fff;text-decoration:none!vital;box-shadow:none!vital;-webkit-box-shadow:none!vital;-moz-box-shadow:none!vital;border:none;border-left:5px cast #00abf0}frame a.novashare-ctt:hover{colour:#fff;border-left:5px cast #008cc4}frame a.novashare-ctt:visited{colour:#fff}frame a.novashare-ctt *{pointer-events:none}frame a.novashare-ctt .novashare-ctt-tweet{show:block;font-size:18px;line-height:27px;margin-bottom:10px}frame a.novashare-ctt .novashare-ctt-cta-container{show:block;overflow:hidden}frame a.novashare-ctt .novashare-ctt-cta{waft:proper}frame a.novashare-ctt.novashare-ctt-cta-left .novashare-ctt-cta{waft:left}frame a.novashare-ctt .novashare-ctt-cta-text{font-size:16px;line-height:16px;vertical-align:center}frame a.novashare-ctt .novashare-ctt-cta-icon{margin-left:10px;show:inline-block;vertical-align:center}frame a.novashare-ctt .novashare-ctt-cta-icon svg{vertical-align:center;peak:18px}frame a.novashare-ctt.novashare-ctt-simple{background:0 0;padding:10px 0 10px 20px;colour:preliminary}frame a.novashare-ctt.novashare-ctt-simple-alt{background:#f9f9f9;padding:20px;colour:preliminary}frame a.novashare-ctt.novashare-ctt-simple-alt:hover,frame a.novashare-ctt.novashare-ctt-simple:hover{border-left:5px cast #008cc4}frame a.novashare-ctt.novashare-ctt-simple .novashare-ctt-cta,frame a.novashare-ctt.novashare-ctt-simple-alt .novashare-ctt-cta{colour:#00abf0}frame a.novashare-ctt.novashare-ctt-simple-alt:hover .novashare-ctt-cta,frame a.novashare-ctt.novashare-ctt-simple:hover .novashare-ctt-cta{colour:#008cc4}
Coding Towards Interfaces, Now not Implementations
Coding towards interfaces is the follow of the usage of contracts to have items of code have interaction with each and every different. A freelance is just a PHP interface (or any other language) that defines what purposes are to be had and their signatures, i.e., what inputs they obtain and their output.
An interface publicizes the intent of the capability with out explaining how the capability shall be applied. Through gaining access to capability by means of interfaces, our application can depend on self sustaining items of code that accomplish a particular objective with out realizing, or worrying about, how they do it. This fashion, the appliance doesn’t want to be tailored to modify to every other piece of code that accomplishes the similar objective — for example, from a distinct supplier.
Instance of Contracts
The next code makes use of Symfony’s contract CacheInterface
and the PHP Usual Advice (PSR) contract CacheItemInterface
to put in force caching capability:
use PsrCacheCacheItemInterface;
use SymfonyContractsCacheCacheInterface;
$worth = $cache->get('my_cache_key', serve as (CacheItemInterface $merchandise) {
$item->expiresAfter(3600);
go back 'foobar';
});
$cache
implements CacheInterface
, which defines the process get
to retrieve an object from the cache. Through gaining access to this capability by means of the contract, the appliance will also be oblivious to the place the cache is. Whether or not it’s in reminiscence, disk, database, community, or anyplace else. Nonetheless, it has to accomplish the serve as. CacheItemInterface
defines means expiresAfter
to claim how lengthy the object should be saved within the cache. The applying can invoke this technique with out worrying what the cached object is; it best cares how lengthy it should be cached.
Coding Towards Interfaces in WordPress
As a result of we’re abstracting WordPress code, the outcome shall be that the appliance received’t reference WordPress code without delay, however all the time by means of an interface. For example, the WordPress serve as get_posts
has this signature:
/**
* @param array $args
* @go back WP_Post[]|int[] Array of put up items or put up IDs.
*/
serve as get_posts( $args = null )
As an alternative of invoking this technique without delay, we will be able to get right of entry to it by means of the contract OwnerMyAppContractsPostsAPIInterface
:
namespace OwnerMyAppContracts;
interface PostAPIInterface
int[];
Be aware that the WordPress serve as get_posts
can go back items of the category WP_Post
, which is restricted to WordPress. When abstracting the code, we want to take away this sort of fastened dependency. The process get_posts
within the contract returns items of the kind PostInterface
, permitting you to reference the category WP_Post
with out being specific about it. The category PostInterface
will want to supply get right of entry to to all strategies and attributes from WP_Post
:
namespace OwnerMyAppContracts;
interface PostInterface
{
public serve as get_ID(): int;
public serve as get_post_author(): string;
public serve as get_post_date(): string;
// ...
}
Executing this technique can alternate our working out of the place WordPress suits in our stack. As an alternative of considering of WordPress as the appliance itself (over which we set up issues and plugins), we will be able to bring to mind it merely as every other dependency inside the utility, replaceable as some other element. (Despite the fact that we received’t update WordPress in follow, it is replaceable from a conceptual perspective.)
Growing and Distributing Programs
Composer is a bundle supervisor for PHP. It permits PHP programs to retrieve programs (i.e. items of code) from a repository and set up them as dependencies. To decouple the appliance from WordPress, we should distribute its code into programs of 2 differing kinds: the ones containing WordPress code and the others containing industry common sense (i.e. no WordPress code).
After all, we upload all programs as dependencies within the utility, and we set up them by means of Composer. Since tooling shall be implemented to the industry code programs, those should include many of the code of the appliance; the upper the proportion, the easier. Having them arrange round 90% of the total code is a superb objective.
Extracting WordPress Code Into Programs
Following the instance from previous on, contracts PostAPIInterface
and PostInterface
shall be added to the bundle containing industry code, and every other bundle will come with the WordPress implementation of those contracts. To fulfill PostInterface
, we create a PostWrapper
magnificence that can retrieve all attributes from a WP_Post
object:
namespace OwnerMyAppForWPContractImplementations;
use OwnerMyAppContractsPostInterface;
use WP_Post;
magnificence PostWrapper implements PostInterface
{
non-public WP_Post $put up;
public serve as __construct(WP_Post $put up)
{
$this->put up = $put up;
}
public serve as get_ID(): int
{
go back $this->post->ID;
}
public serve as get_post_author(): string
{
go back $this->post->post_author;
}
public serve as get_post_date(): string
{
go back $this->post->post_date;
}
// ...
}
When enforcing PostAPI
, since means get_posts
returns PostInterface[]
, we should convert items from WP_Post
to PostWrapper
:
namespace OwnerMyAppForWPContractImplementations;
use OwnerMyAppContractsPostAPIInterface;
use WP_Post;
magnificence PostAPI implements PostAPIInterface
{
public serve as get_posts(array $args = null): PostInterface[]|int[]
{
// This var will include WP_Post[] or int[]
$wpPosts = get_posts($args);
// Convert WP_Post[] to PostWrapper[]
go back array_map(
serve as (WP_Post|int $put up) {
if ($put up instanceof WP_Post) {
go back new PostWrapper($put up);
}
go back $put up
},
$wpPosts
);
}
}
The use of Dependency Injection
Dependency injection is a design trend that permits you to glue all utility portions in combination in a loosely coupled approach. With dependency injection, the appliance accesses products and services by means of their contracts, and the contract implementations are “injected” into the appliance by means of configuration.
Just by converting the configuration, we will be able to simply transfer from one contract supplier to every other one. There are a number of dependency injection libraries we will be able to make a choice from. We suggest deciding on one that clings to the PHP Standard Recommendations (regularly known as “PSR”), so we will be able to simply update the library with every other one if the will arises. Regarding dependency injection, the library should fulfill PSR-11, which supplies the specification for a “container interface.” Amongst others, the next libraries conform to PSR-11:
- Symfony’s DependencyInjection
- PHP-DI
- Aura.Di
- Container (Dependency Injection)
- Yii Dependency Injection
Gaining access to Services and products by means of the Provider Container
The dependency injection library will make to be had a “carrier container,” which resolves a freelance into its corresponding enforcing magnificence. The applying should depend at the carrier container to get right of entry to all capability. For example, whilst we’d most often invoke WordPress purposes without delay:
$posts = get_posts();
…with the carrier container, we should first download the carrier that satisfies PostAPIInterface
and execute the capability via it:
use OwnerMyAppContractsPostAPIInterface;
// Download the carrier container, as laid out in the library we use
$serviceContainer = ContainerBuilderFactory::getInstance();
// The got carrier shall be of sophistication OwnerMyAppForWPContractImplementationsPostAPI
$postAPI = $serviceContainer->get(PostAPIInterface::magnificence);
// Now we will be able to invoke the WordPress capability
$posts = $postAPI->get_posts();
The use of Symfony’s DependencyInjection
Symfony’s DependencyInjection component is lately the most well liked dependency injection library. It means that you can configure the carrier container by means of PHP, YAML, or XML code. For example, to outline that contract PostAPIInterface
is glad by means of magnificence PostAPI
is configured in YAML like this:
products and services:
OwnerMyAppContractsPostAPIInterface:
magnificence: OwnerMyAppForWPContractImplementationsPostAPI
Symfony’s DependencyInjection additionally permits for cases from one carrier to be robotically injected (or “autowired”) into some other carrier that will depend on it. As well as, it makes it simple to outline {that a} magnificence is an implementation of its personal carrier. For example, imagine the following YAML configuration:
products and services:
_defaults:
public: true
autowire: true
GraphQLAPIGraphQLAPIRegistriesUserAuthorizationSchemeRegistryInterface:
magnificence: 'GraphQLAPIGraphQLAPIRegistriesUserAuthorizationSchemeRegistry'
GraphQLAPIGraphQLAPISecurityUserAuthorizationInterface:
magnificence: 'GraphQLAPIGraphQLAPISecurityUserAuthorization'
GraphQLAPIGraphQLAPISecurityUserAuthorizationSchemes:
useful resource: '../src/Safety/UserAuthorizationSchemes/*'
This configuration defines the next:
- Contract
UserAuthorizationSchemeRegistryInterface
is glad by means of magnificenceUserAuthorizationSchemeRegistry
- Contract
UserAuthorizationInterface
is glad by means of magnificenceUserAuthorization
- All categories underneath the folder
UserAuthorizationSchemes/
are an implementation of themselves - Services and products should be robotically injected into one every other (
autowire: true
)
Let’s see how autowiring works. The category UserAuthorization
will depend on carrier with contract UserAuthorizationSchemeRegistryInterface
:
magnificence UserAuthorization implements UserAuthorizationInterface
{
public serve as __construct(
safe UserAuthorizationSchemeRegistryInterface $userAuthorizationSchemeRegistry
) {
}
// ...
}
Because of autowire: true
, the DependencyInjection element will robotically have the carrier UserAuthorization
obtain its required dependency, which is an example of UserAuthorizationSchemeRegistry
.
When To Summary
Abstracting code may just eat substantial effort and time, so we will have to best adopt it when its advantages outweigh its prices. The next are ideas of when abstracting the code could also be price it. You’ll be able to do that by means of the usage of code snippets on this article or the urged summary WordPress plugins underneath.
Gaining Get entry to to Tooling
As discussed previous, running PHP-Scoper on WordPress is difficult. Through decoupling the WordPress code into unique programs, it turns into possible to scope a WordPress plugin without delay.
Lowering Tooling Time and Value
Operating a PHPUnit check suite takes longer when it must initialize and run WordPress than when it doesn’t. Much less time too can translate into much less cash spent working the exams — as an example, GitHub Movements fees for GitHub-hosted runners in accordance with time spent the usage of them.
Heavy Refactoring Now not Wanted
An current assignment would possibly require heavy refactoring to introduce the specified structure (dependency injection, splitting code into programs, and so on.), making it tough to drag out. Abstracting code when making a assignment from scratch makes it a lot more manageable.
Generating Code for A couple of Platforms
Through extracting 90% of the code right into a CMS-agnostic bundle, we will be able to produce a library model that works for a distinct CMS or framework by means of best changing 10% of the total codebase.
Signal Up For the Publication
Migrating to a Other Platform
If we want to migrate a assignment from Drupal to WordPress, WordPress to Laravel, or some other aggregate, then best 10% of the code should be rewritten — a vital saving.
Absolute best Practices
Whilst designing the contracts to summary our code, there are a number of enhancements we will be able to follow to the codebase.
Adhere to PSR-12
When defining the interface to get right of entry to the WordPress strategies, we will have to adhere to PSR-12. This fresh specification goals to scale back cognitive friction when scanning code from other authors. Adhering to PSR-12 implies renaming the WordPress purposes.
WordPress names purposes the usage of snake_case, whilst PSR-12 makes use of camelCase. Therefore, serve as get_posts
will turn into getPosts
:
interface PostAPIInterface
int[];
…and:
magnificence PostAPI implements PostAPIInterface
{
public serve as getPosts(array $args = null): PostInterface[]|int[]
{
// This var will include WP_Post[] or int[]
$wpPosts = get_posts($args);
// Remainder of the code
// ...
}
}
Break up Strategies
Strategies within the interface don’t want to be a duplicate of those from WordPress. We will change into them every time it is smart. For example, the WordPress serve as get_user_by($box, $worth)
is aware of retrieve the person from the database by means of parameter $box
, which accepts values "identity"
, "ID"
, "slug"
, "electronic mail"
or "login"
. This design has a couple of problems:
- It’ll now not fail at compilation time if we cross a unsuitable string
- Parameter
$worth
wishes to just accept all differing kinds for all choices, although when passing"ID"
it expects anint
, when passing"electronic mail"
it could actually best obtain astring
We will make stronger this example by means of splitting the serve as into a number of ones:
namespace OwnerMyAppContracts;
interface UserAPIInterface
{
public serve as getUserById(int $identity): ?UserInterface;
public serve as getUserByEmail(string $electronic mail): ?UserInterface;
public serve as getUserBySlug(string $slug): ?UserInterface;
public serve as getUserByLogin(string $login): ?UserInterface;
}
The contract is resolved for WordPress like this (assuming we’ve got created UserWrapper
and UserInterface
, as defined previous on):
namespace OwnerMyAppForWPContractImplementations;
use OwnerMyAppContractsUserAPIInterface;
magnificence UserAPI implements UserAPIInterface
{
public serve as getUserById(int $identity): ?UserInterface
{
go back $this->getUserByProp('identity', $identity);
}
public serve as getUserByEmail(string $electronic mail): ?UserInterface
{
go back $this->getUserByProp('electronic mail', $electronic mail);
}
public serve as getUserBySlug(string $slug): ?UserInterface
{
go back $this->getUserByProp('slug', $slug);
}
public serve as getUserByLogin(string $login): ?UserInterface
{
go back $this->getUserByProp('login', $login);
}
non-public serve as getUserByProp(string $prop, int|string $worth): ?UserInterface
{
if ($person = get_user_by($prop, $worth)) {
go back new UserWrapper($person);
}
go back null;
}
}
Take away Implementation Main points from Serve as Signature
Purposes in WordPress would possibly supply data on how they’re applied in their very own signature. This knowledge will also be got rid of when appraising the serve as from an summary standpoint. For instance, acquiring the person’s remaining identify in WordPress is finished by means of calling get_the_author_meta
, making it specific {that a} person’s remaining identify is saved as a “meta” worth (on desk wp_usermeta
):
$userLastname = get_the_author_meta("user_lastname", $user_id);
You don’t need to put across this knowledge to the contract. Interfaces best care in regards to the what, now not the how. Therefore, the contract can as a substitute have one way getUserLastname
, which doesn’t supply any data on the way it’s applied:
All Kinsta internet hosting plans come with 24/7 make stronger from our veteran WordPress builders and engineers. Chat with the similar group that backs our Fortune 500 shoppers. Check out our plans!
interface UserAPIInterface
{
public serve as getUserLastname(UserWrapper $userWrapper): string;
...
}
Upload Stricter Varieties
Some WordPress purposes can obtain parameters in several techniques, resulting in ambiguity. For example, serve as add_query_arg
can both obtain a unmarried key and worth:
$url = add_query_arg('identity', 5, $url);
… or an array of key => worth
:
$url = add_query_arg(['id' => 5], $url);
Our interface can outline a extra understandable intent by means of splitting such purposes into a number of separate ones, each and every of them accepting a singular aggregate of inputs:
public serve as addQueryArg(string $key, string $worth, string $url);
public serve as addQueryArgs(array $keyValues, string $url);
Wipe Out Technical Debt
The WordPress serve as get_posts
returns now not best “posts” but in addition “pages” or any entity of sort “customized posts,” and those entities aren’t interchangeable. Each posts and pages are customized posts, however a web page isn’t a put up and now not a web page. Due to this fact, executing get_posts
can go back pages. This habits is a conceptual discrepancy.
To make it right kind, get_posts
will have to as a substitute be referred to as get_customposts
, but it surely was once by no means renamed in WordPress core. It’s a not unusual factor with maximum long-lasting instrument and is named “technical debt” — code that has issues, however isn’t fastened as it introduces breaking adjustments.
When growing our contracts, despite the fact that, we’ve got the chance to keep away from this sort of technical debt. On this case, we will be able to create a brand new interface ModelAPIInterface
which will take care of entities of various varieties, and we make a number of strategies, each and every to take care of a distinct sort:
interface ModelAPIInterface
{
public serve as getPosts(array $args): array;
public serve as getPages(array $args): array;
public serve as getCustomPosts(array $args): array;
}
This fashion, the discrepancy received’t happen anymore, and also you’ll see those effects:
getPosts
returns best postsgetPages
returns best pagesgetCustomPosts
returns each posts and pages
Advantages of Abstracting Code
The primary benefits of abstracting an utility’s code are:
- Tooling working on programs containing best industry code is more straightforward to arrange and can take much less time (and no more cash) to run.
- We will use tooling that doesn’t paintings with WordPress, comparable to scoping a plugin with PHP-Scoper.
- The programs we produce will also be self sustaining to make use of in different programs simply.
- Migrating an utility to different platforms turns into more straightforward.
- We will shift our mindset from WordPress considering to suppose relating to our industry common sense.
- The contracts describe the intent of the appliance, making it extra comprehensible.
- The applying will get arranged via programs, making a lean utility containing the naked minimal and gradually bettering it as wanted.
- We will transparent up technical debt.
Problems With Abstracting Code
The disadvantages of abstracting an utility’s code are:
- It comes to a large amount of paintings first of all.
- Code turns into extra verbose; upload further layers of code to succeed in the similar consequence.
- Chances are you’ll finally end up generating dozens of packages which should then be controlled and maintained.
- Chances are you’ll require a monorepo to regulate all programs in combination.
- Dependency injection may well be overkill for easy programs (diminishing returns).
- Abstracting the code won’t ever be totally completed since there’s most often a basic choice implicit within the CMS’s structure.
Summary WordPress Plugin Choices
Even supposing it’s most often wisest to extract your code to a local environment earlier than operating on it, some WordPress plugins allow you to towards your abstraction objectives. Those are our most sensible selections.
1. WPide
Produced by means of WebFactory Ltd, the preferred WPide plugin dramatically extends WordPress’s default code editor’s capability. It serves as an summary WordPress plugin by means of permitting you to view your code in situ to visualise higher what wishes consideration.
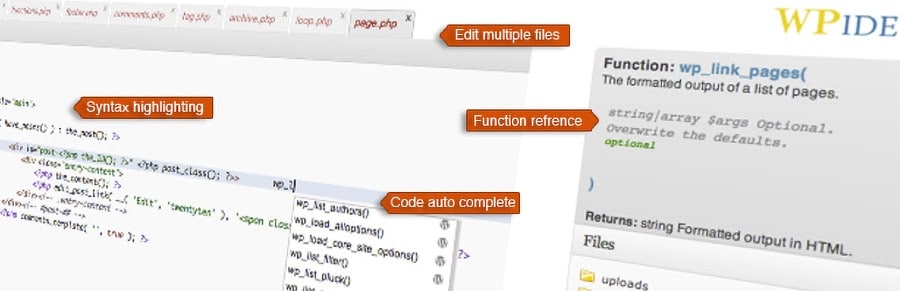
The WPide plugin.
WPide additionally has a search-and-replace serve as for briefly finding out of date or expired code and changing it with a refactored rendition.
On most sensible of this, WPide supplies a variety of further options, together with:
- Syntax and block highlighting
- Automatic backups
- Record and folder introduction
- Complete document tree browser
- Get entry to to the WordPress filesystem API
2. Final DB Supervisor
The Ultimate WP DB Manager plugin from WPHobby will give you a handy guide a rough approach to obtain your databases in complete for extraction and refactoring.
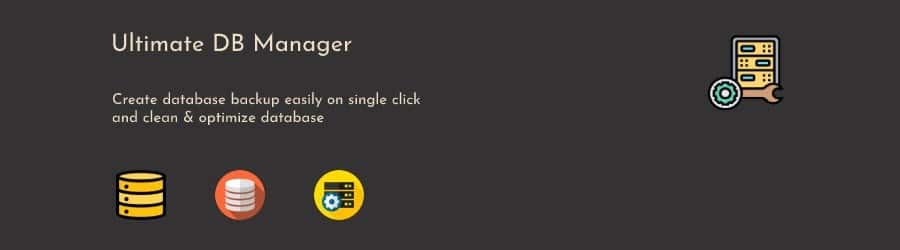
The Final DB Supervisor plugin.
After all, plugins of this sort aren’t essential for Kinsta customers, as Kinsta provides direct database access to all consumers. Alternatively, in the event you don’t have enough database get right of entry to via your internet hosting supplier, Final DB Supervisor may just come in useful as an summary WordPress plugin.
3. Your Personal Customized Summary WordPress Plugin
After all, your only option for abstraction will all the time be to create your plugin. It is going to appear to be a large enterprise, however if in case you have restricted talent to regulate your WordPress core recordsdata without delay, this provides an abstraction-friendly workaround.
Doing so has transparent advantages:
- Abstracts your purposes out of your theme recordsdata
- Preserves your code via theme adjustments and database updates
You’ll be able to discover ways to create your summary WordPress plugin via WordPress’ Plugin Developer Handbook.
Abstract
Must we summary the code in our programs? As with the whole lot, there’s no predefined “proper solution” because it will depend on a project-by-project foundation. The ones initiatives requiring an amazing period of time to research with PHPUnit or PHPStan can receive advantages probably the most, however the effort had to pull it off won’t all the time be price it.
You’ve discovered the whole lot you want to grasp to get began abstracting WordPress code.
Do you intend to put in force this technique on your assignment? If this is the case, will you employ an summary WordPress plugin? Tell us within the feedback phase!
The put up WordPress Abstraction: Best Practices and WordPress Abstraction Plugins gave the impression first on Kinsta.
WP Hosting