In 2018, WordPress offered the Gutenberg editor with model 5.0, bringing a brand new method to construct pages and posts the usage of “blocks.” In the beginning, those blocks had been beautiful elementary, however over time, they’ve developed to supply extra flexibility and a greater modifying revel in.
Nonetheless, there are occasions when a block doesn’t fairly do what you want. Perhaps you wish to have to take away positive options, upload new ones, practice a particular genre by way of default, or make some settings more straightforward to get admission to. In circumstances like this, making a tradition block from scratch would possibly appear to be an possibility, however — let’s be truthful — it’s overkill for small tweaks. Wouldn’t or not it’s more straightforward if it’s essential to simply alter blocks that exist already?
That’s the place the Blocks API is available in. This text explains the right way to lengthen core WordPress blocks the usage of the Blocks API, offering sensible examples that can be utilized in real-world initiatives.
Figuring out the WordPress Blocks API
The WordPress Blocks API is the basis of the block editor, permitting builders to create, alter, and lengthen blocks. The API supplies quite a lot of techniques to have interaction with blocks. You’ll be able to:
- Regulate block settings — Alternate block attributes, default values, and behaviors.
- Upload or take away block helps — Permit or disable options like typography, colours, and spacing.
- Inject tradition controls — Upload new choices within the block settings panel.
- Create block versions — Make pre-configured variations of current blocks to hurry up content material advent.
Each and every block in WordPress, whether or not it’s a Paragraph, Symbol, or Button block, is outlined by way of a suite of attributes and settings saved in a block.json
report. This report comprises metadata in regards to the block, together with its call, class, default attributes, and the options it helps.
WordPress lets you alter those values the usage of both PHP or JavaScript, however this newsletter explains the right way to use clear out hooks within the Blocks API. This guarantees your adjustments are registered at the server with no need to enqueue further JavaScript recordsdata.
For instance, if you wish to allow or disable positive options of a block, the easiest way to do it’s by way of the usage of the register_block_type_args
clear out in PHP. This system lets you tweak block settings dynamically with out editing the block.json
report immediately.
Editing block helps
WordPress blocks include predefined helps, which keep an eye on the editor’s choices. Some blocks, just like the Symbol block (core/picture
), have duotone filters enabled by way of default, permitting customers to use shade overlays.
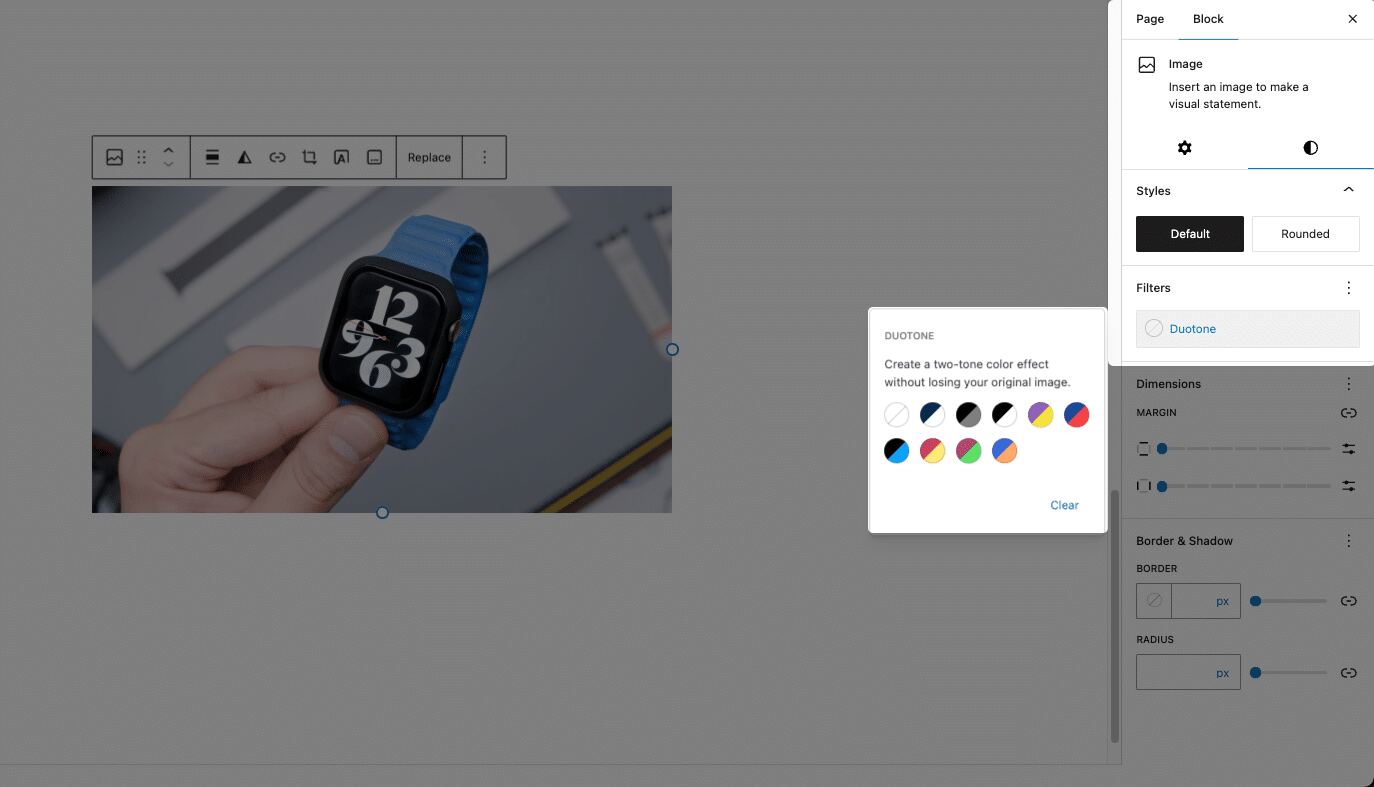
On the other hand, the Media & Textual content block (core/media-text
) does now not strengthen duotone out of the field, even if it lets in customers to insert a picture. Because of this whilst you’ll be able to practice a duotone clear out to a standalone Symbol block, you’ll be able to’t do the similar when a picture is positioned inside of a Media & Textual content block.
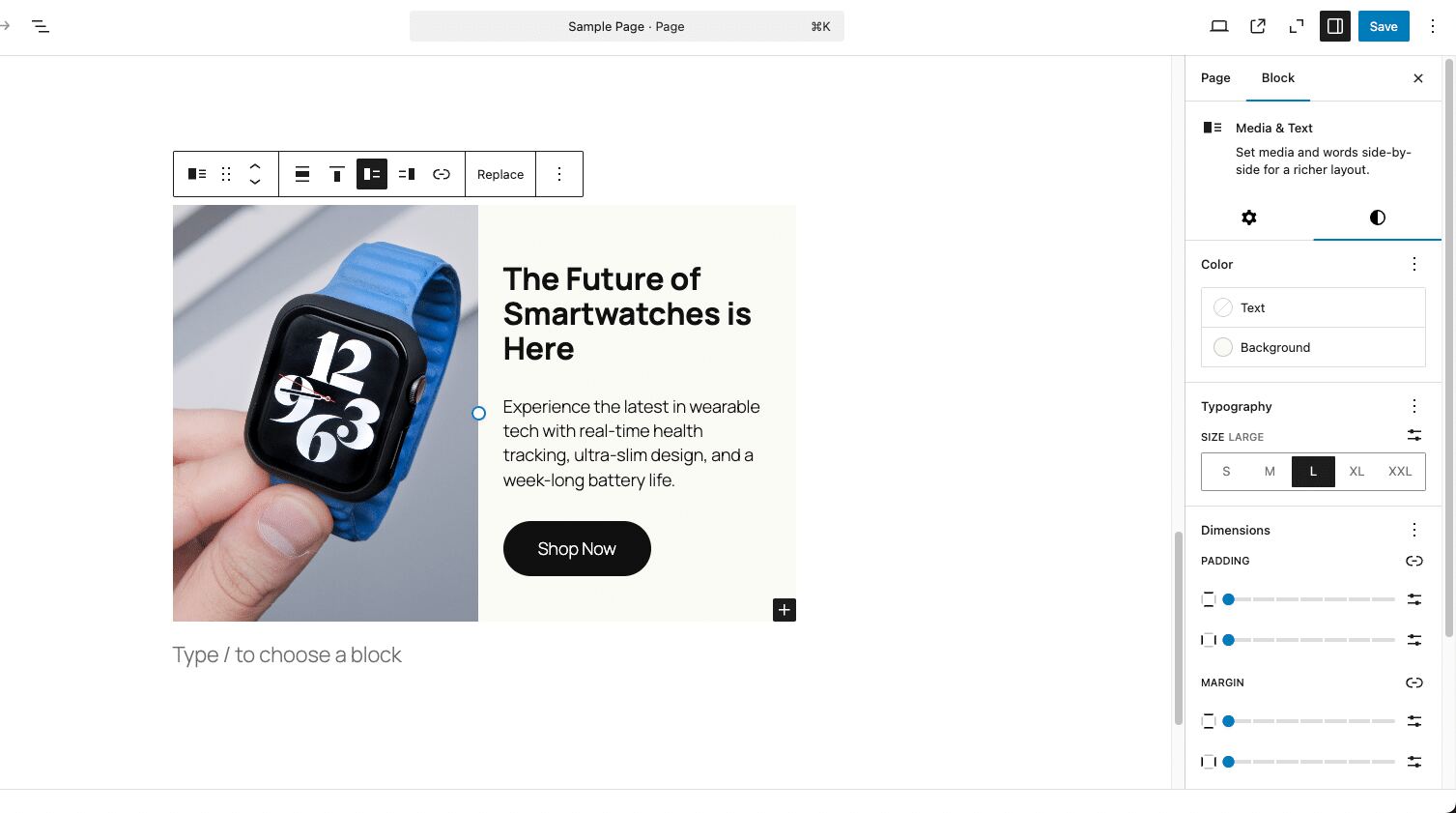
For the reason that Media & Textual content block can comprise a picture, enabling duotone filters is sensible. We will be able to do that by way of editing its helps
array and specifying the right kind CSS selector so the clear out applies as it should be. We will be able to allow it the usage of the register_block_type_args
clear out in PHP.
Upload the next code to the purposes.php
report of your theme:
serve as enable_duotone_for_media_text_block($args, $block_type) {
// Best alter the Media & Textual content block
if ( 'core/media-text' === $block_type ) {
$args['supports'] ??= [];
$args['supports']['filter'] ??= [];
$args['supports']['filter']['duotone'] = true;
$args['selectors'] ??= [];
$args['selectors']['filter'] ??= [];
$args['selectors']['filter']['duotone'] = '.wp-block-media-text .wp-block-media-text__media';
}
go back $args;
}
add_filter('register_block_type_args', 'enable_duotone_for_media_text_block', 10, 2);
The code above allows the duotone clear out within the helps
array and defines the right kind CSS selector to use the duotone impact to pictures within the Media & Textual content block. The add_filter()
serve as makes use of 10
as the concern (when the clear out runs) and 2
to specify the selection of arguments handed ($args
, $block_type
).
While you save the report and reload, you must see the Duotone controls to be had within the Filters segment.
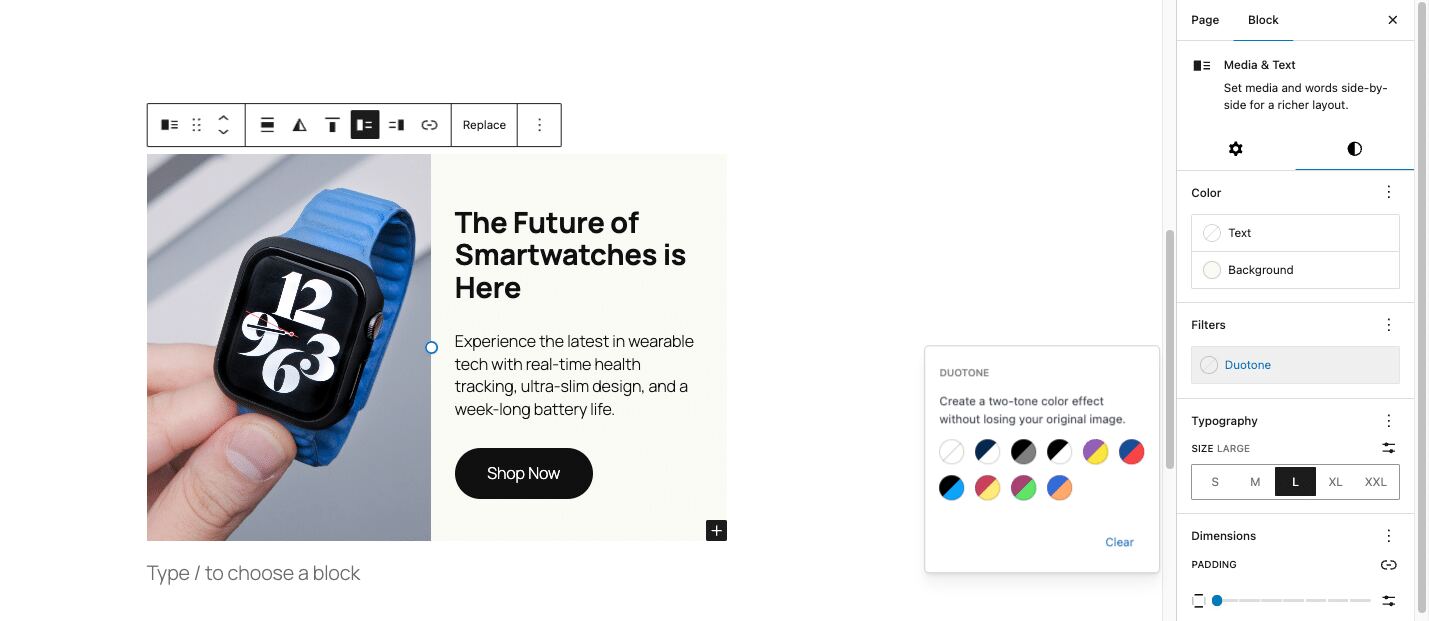
Enabling duotone for the Media & Textual content block the usage of register_block_type_args
is a great way to change block conduct dynamically. On the other hand, WordPress supplies every other manner for editing block settings: overriding block metadata the usage of block_type_metadata
.
Each approaches can help you customise blocks, however they paintings at other phases of the block registration procedure.
For instance, let’s say we wish to modify the Paragraph block (core/paragraph
) in order that it most effective helps margin changes and disables padding. A technique to do that is by way of the usage of register_block_type_args
:
serve as modify_paragraph_spacing_args($args, $block_type) {
if ($block_type === 'core/paragraph') {
$args['supports']['spacing'] = [
'margin' => true,
'padding' => false
];
}
go back $args;
}
add_filter('register_block_type_args', 'modify_paragraph_spacing_args', 10, 2);
This system works effectively usually, however because it modifies the block after it has already been registered, it may once in a while be overridden by way of different plugins or issues that vary the similar block later within the procedure.
A extra structured manner on this case might be to override the block’s metadata immediately the usage of block_type_metadata
:
serve as mytheme_modify_paragraph_spacing($metadata) {
if ($metadata['name'] === 'core/paragraph') {
$metadata['supports']['spacing'] = [
'margin' => true,
'padding' => false
];
}
go back $metadata;
}
add_filter('block_type_metadata', 'mytheme_modify_paragraph_spacing');
Neither manner is inherently higher than the opposite — it simply depends upon when you wish to have to change the block and the way power you wish to have the alternate to be.
Registering block types
Many WordPress blocks include predefined types that customers can make a selection within the editor. A just right instance is the Symbol block (core/picture
), which incorporates a Rounded genre by way of default. On the other hand, the default rounded corners are continuously too excessive, making the picture glance extra like an oval than a smartly styled component.
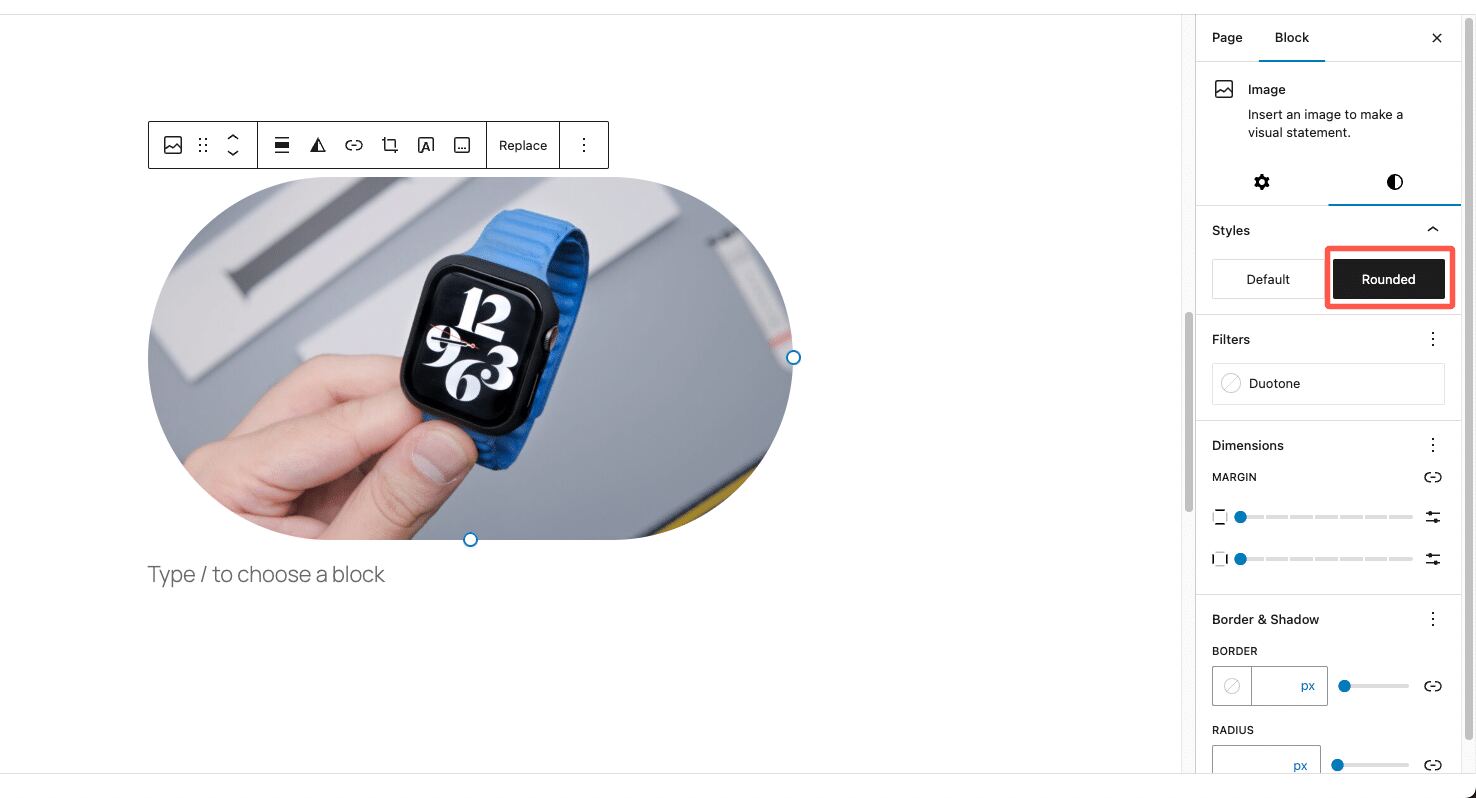
As a substitute of manually adjusting the border radius for each and every picture, a greater manner is to customise the rounded genre so it applies a extra subtle nook radius — possibly including a delicate shadow for a contemporary glance. This fashion, customers can merely click on a button to use a well-designed genre with no need to manually tweak settings each and every time.
Let’s take the Rounded genre within the Symbol block and customise it in order that the corners are somewhat rounded as an alternative of excessively curved, and a delicate field shadow is added for a extra polished glance.
For the reason that block editor lets in registering and unregistering block types, we will be able to take away the default Rounded genre and substitute it with a tradition model.
Right here’s the right way to do it:
serve as modify_image_block_rounded_style() {
// Take away the default "Rounded" genre
unregister_block_style( 'core/picture', 'rounded' );
// Check in a brand new "Rounded" genre with tradition CSS
register_block_style(
'core/picture',
array(
'call' => 'rounded',
'label' => __( 'Rounded', 'your-text-domain' ),
'inline_style' => '
.wp-block-image.is-style-rounded img {
border-radius: 20px; /* Alter this worth */
box-shadow: 0px 4px 6px rgba(0, 0, 0, 0.1); /* Non-compulsory shadow */
}
',
)
);
}
add_action( 'init', 'modify_image_block_rounded_style' );
The code replaces the default overly-rounded genre with a sophisticated model that applies border-radius: 20px;
for softer corners and box-shadow
for a delicate carry.
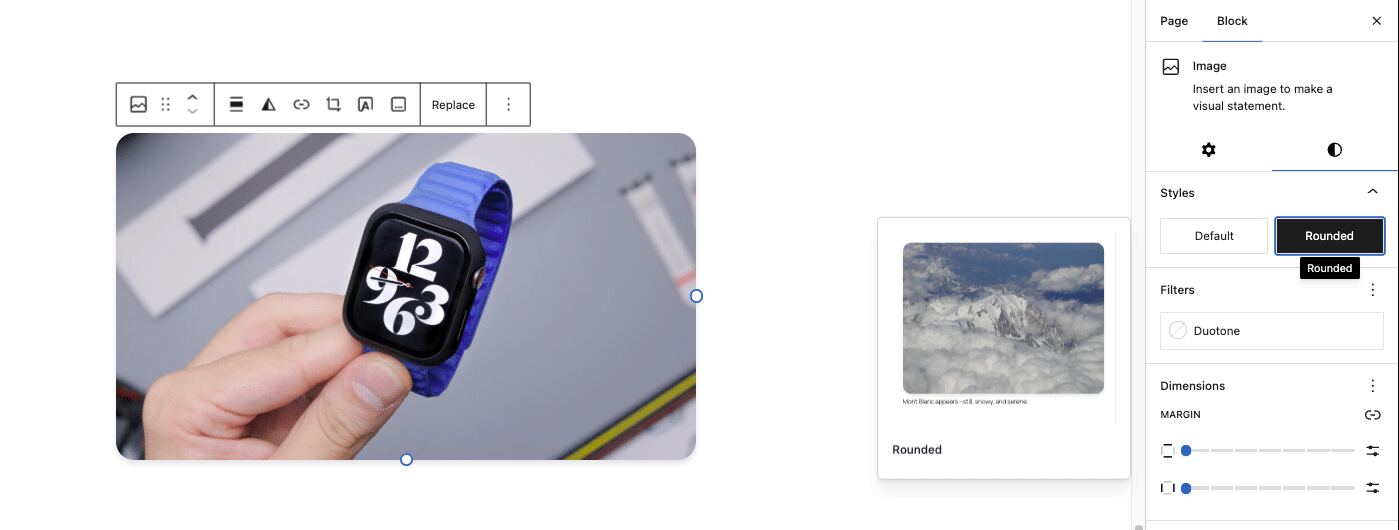
The use of an exterior CSS report as an alternative of inline types
Whilst inline genre works effectively for easy types, it’s now not excellent for maintainability. A greater manner is to outline types in an exterior CSS report and enqueue it as an alternative.
To do that, create a brand new CSS report, e.g., custom-block-styles.css
, and upload this to it:
/* Customized Rounded Symbol Block Taste */
.wp-block-image.is-style-rounded img {
border-radius: 20px; /* Adjusted rounded corners */
box-shadow: 0px 4px 6px rgba(0, 0, 0, 0.1); /* Refined shadow */
}
Subsequent, enqueue the CSS report in purposes.php
:
serve as enqueue_custom_block_styles() {
wp_enqueue_style(
'custom-block-styles',
get_template_directory_uri() . '/css/custom-block-styles.css',
array(),
'1.0'
);
}
add_action('wp_enqueue_scripts', 'enqueue_custom_block_styles');
add_action('enqueue_block_editor_assets', 'enqueue_custom_block_styles');
Now, as an alternative of the usage of inline genre, you’ll be able to sign up the manner with out embedding CSS immediately in PHP:
register_block_style(
'core/picture',
array(
'call' => 'rounded',
'label' => __( 'Rounded', 'your-text-domain' ),
'style_handle' => 'custom-block-styles'
)
);
This fashion, you’ll be able to alter types with out touching PHP.
Registering block versions
Block versions help you create predefined variations of a block with tradition settings, making it more straightforward for customers to use constant designs with a unmarried click on. As a substitute of editing a block’s settings manually each and every time, a variation lets you insert a block that already has the appropriate attributes, types, or configurations implemented.
Some core WordPress blocks in truth paintings this manner. The Embed block isn’t a unmarried block — it’s a suite of versions for various platforms like YouTube, Twitter, and Spotify. The Row block, and Stack block also are simply versions of the Crew block, each and every with other structure settings.
This manner helps to keep issues modular, permitting WordPress to supply adapted reports whilst the usage of a shared underlying construction.
Making a Testimonial variation of the Quote block
Whilst WordPress doesn’t have a devoted Testimonial block, the Quote block (core/quote
) will also be tailored to serve that objective. As a substitute of creating customers manually upload a picture, align the textual content, and layout the whole lot, we will be able to outline a Testimonial variation of the Quote block.
This modification robotically comprises an Symbol block, a Quote block, and two Paragraph blocks for the individual’s call and corporate. This guarantees each and every testimonial follows the similar structured layout with out requiring further changes.
To sign up a block variation in WordPress, we use registerBlockVariation()
in JavaScript. Since block versions are treated at the consumer facet, we want to enqueue a JavaScript report that registers our tradition Testimonial variation.
To enforce this, create a JavaScript report (like custom-block-variations.js
) that defines the Testimonial variation of the Quote block. You’ll be able to create this report for your theme’s property/js/
listing and upload the next code:
wp.domReady(() => {
wp.blocks.registerBlockVariation(
'core/quote',
{
call: 'testimonial',
name: 'Testimonial',
description: 'A variation of the Quote block for testimonials.',
class: 'textual content',
attributes: {
className: 'is-style-testimonial',
},
innerBlocks: [
['core/image', { align: 'center', width: 100, height: 100 }],
['core/quote'],
['core/paragraph', { placeholder: 'Name', align: 'center', fontSize: 'medium', className: 'testimonial-name' }],
['core/paragraph', { placeholder: 'Company / Role', align: 'center', fontSize: 'small', className: 'testimonial-company' }]
],
scope: ['inserter'],
}
);
// Inject inline types for the editor preview
const genre = file.createElement('genre');
genre.innerHTML = `
.wp-block-quote.is-style-testimonial {
background-color: #f9f9f9;
padding: 24px;
border: none !necessary;
border-radius: 8px;
text-align: heart;
font-size: 1.2em;
font-style: commonplace;
shade: #333;
box-shadow: 0px 4px 10px rgba(0, 0, 0, 0.1);
show: flex;
flex-direction: column;
align-items: heart;
}
.wp-block-quote.is-style-testimonial p {
margin-bottom: 12px;
font-size: 1.1em;
}
.wp-block-quote.is-style-testimonial cite {
font-weight: daring;
show: block;
margin-top: 10px;
shade: #0073aa;
}
.wp-block-quote.is-style-testimonial .wp-block-image {
show: flex;
justify-content: heart;
margin: 0 auto 12px;
}
.wp-block-quote.is-style-testimonial .wp-block-image img {
width: 100px;
peak: 100px;
object-fit: duvet;
border-radius: 12px;
}
.wp-block-quote.is-style-testimonial .testimonial-name {
font-weight: daring;
font-size: 1.2em;
margin-top: 12px;
shade: #222;
}
.wp-block-quote.is-style-testimonial .testimonial-company {
font-size: 0.9em;
shade: #555;
}
`;
file.head.appendChild(genre);
});
Within the code above, registerBlockVariation()
defines the Testimonial variation of the Quote block, preloading an Symbol block, a Quote block, and two Paragraph blocks for the individual’s call and corporate. The Symbol block is focused at 100×100 pixels for uniform profile footage, whilst the Quote block stays unchanged because the testimonial textual content.
A tradition elegance (is-style-testimonial
) is implemented for styling, giving the block a gentle background, delicate shadow, and focused textual content. The default left border is got rid of, and the picture helps to keep its side ratio with somewhat rounded corners for a sophisticated glance.
Subsequent, for the reason that JavaScript report must load within the block editor, we will have to enqueue it in purposes.php
.
serve as enqueue_custom_block_variations() {
wp_enqueue_script(
'custom-block-variations',
get_template_directory_uri() . '/property/js/custom-block-variations.js',
array( 'wp-blocks', 'wp-dom-ready', 'wp-edit-post' ),
filemtime( get_template_directory() . '/property/js/custom-block-variations.js' ),
true
);
}
add_action( 'enqueue_block_editor_assets', 'enqueue_custom_block_variations' );
This guarantees the Testimonial block variation seems within the block editor.
Whilst the JavaScript code guarantees the block seems right kind within the editor, we additionally want to practice the types at the frontend so testimonials show as it should be when revealed. Upload the next code to purposes.php
:
serve as register_testimonial_block_style() {
register_block_style(
'core/quote',
array(
'call' => 'testimonial',
'label' => __( 'Testimonial', 'your-text-domain' ),
'inline_style' => '
.wp-block-quote.is-style-testimonial {
background-color: #f9f9f9;
padding: 24px;
border: none !necessary;
border-radius: 8px;
text-align: heart;
font-size: 1.2em;
font-style: commonplace;
shade: #333;
box-shadow: 0px 4px 10px rgba(0, 0, 0, 0.1);
show: flex;
flex-direction: column;
align-items: heart;
}
.wp-block-quote.is-style-testimonial .wp-block-image img {
width: 100px;
peak: 100px;
object-fit: duvet;
border-radius: 12px;
}
.wp-block-quote.is-style-testimonial .testimonial-name {
font-weight: daring;
font-size: 1.2em;
margin-top: 12px;
shade: #222;
}
.wp-block-quote.is-style-testimonial .testimonial-company {
font-size: 0.9em;
shade: #555;
}
',
)
);
}
add_action( 'init', 'register_testimonial_block_style' );
Now, each time a person inserts a Testimonial block, it already comprises the picture, quote, call, and corporation fields, all preformatted and styled as it should be. This gets rid of the desire for guide changes, making sure each and every testimonial follows the similar blank {and professional} construction.
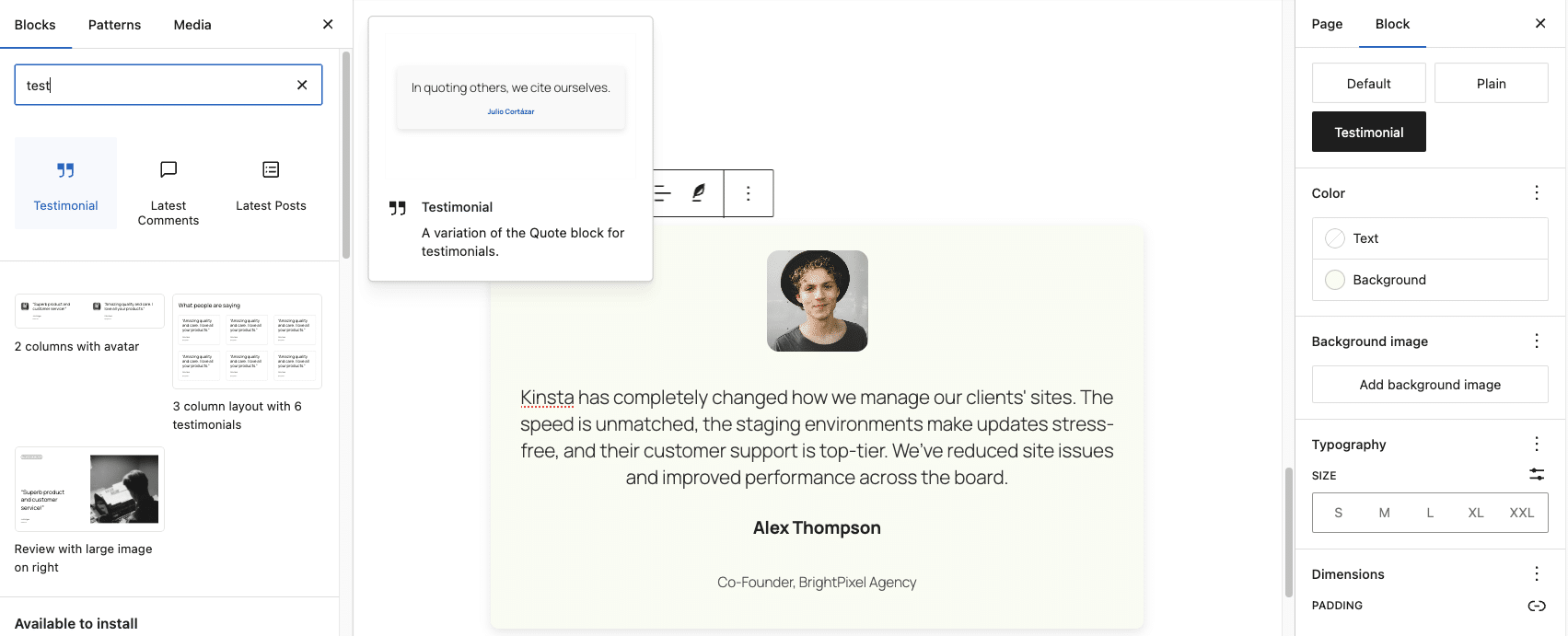
As a substitute of forcing customers to construct testimonials from scratch, this block variation supplies a streamlined workflow that complements content material advent whilst keeping up web site design consistency.
Complex use case: Combining the Helps and Types APIs to create branded buttons
Now that what each and every API can do and the way it works, why don’t we push issues a bit additional? As a substitute of the usage of the Helps API or Types API one at a time, we will be able to use them in combination to unravel a unmarried drawback: keeping up design consistency whilst giving customers a structured method to practice the appropriate types.
Let’s believe a real-world situation. An organization needs all buttons on its web site to apply strict logo pointers. They don’t need customers selecting random colours, converting padding, or making use of funky typography. On the other hand, they do need flexibility — so customers must be ready to choose from two pre-approved button types:
- Number one Button — The primary call-to-action button, with a forged background and ambitious styling.
- Secondary Button — A extra delicate, defined button normally used for secondary movements.
To reach this, we want to:
- Use the Types API to outline the 2 button types.
- Use the Helps API to take away pointless settings, making sure customers don’t manually override branding by way of converting colours, spacing, or typography.
Through combining each APIs, we permit structured alternatives whilst combating customers from breaking the design device.
Step 1: Outline tradition button types
Get started by way of including the next code to the purposes.php
report:
serve as register_custom_button_styles() {
register_block_style(
'core/button',
array(
'call' => 'primary-button',
'label' => __( 'Number one Button', 'your-text-domain' ),
'inline_style' => '
.wp-block-button.is-style-primary-button .wp-block-button__link {
background-color: #4D4D4D;
shade: #ffffff;
padding: 12px 24px;
border-radius: 4px;
font-size: 1em;
font-weight: 500;
text-transform: none;
box-shadow: 2px 2px 6px rgba(0, 0, 0, 0.1);
}
',
)
);
register_block_style(
'core/button',
array(
'call' => 'secondary-button',
'label' => __( 'Secondary Button', 'your-text-domain' ),
'inline_style' => '
.wp-block-button.is-style-secondary-button .wp-block-button__link {
background-color: clear;
shade: #4D4D4D;
padding: 12px 24px;
border: 1px forged #4D4D4D;
border-radius: 4px;
font-size: 1em;
font-weight: 500;
text-transform: none;
box-shadow: none;
}
',
)
);
}
add_action( 'init', 'register_custom_button_styles' );
Now, when customers insert a Button block, they see Number one Button and Secondary Button as genre alternatives.
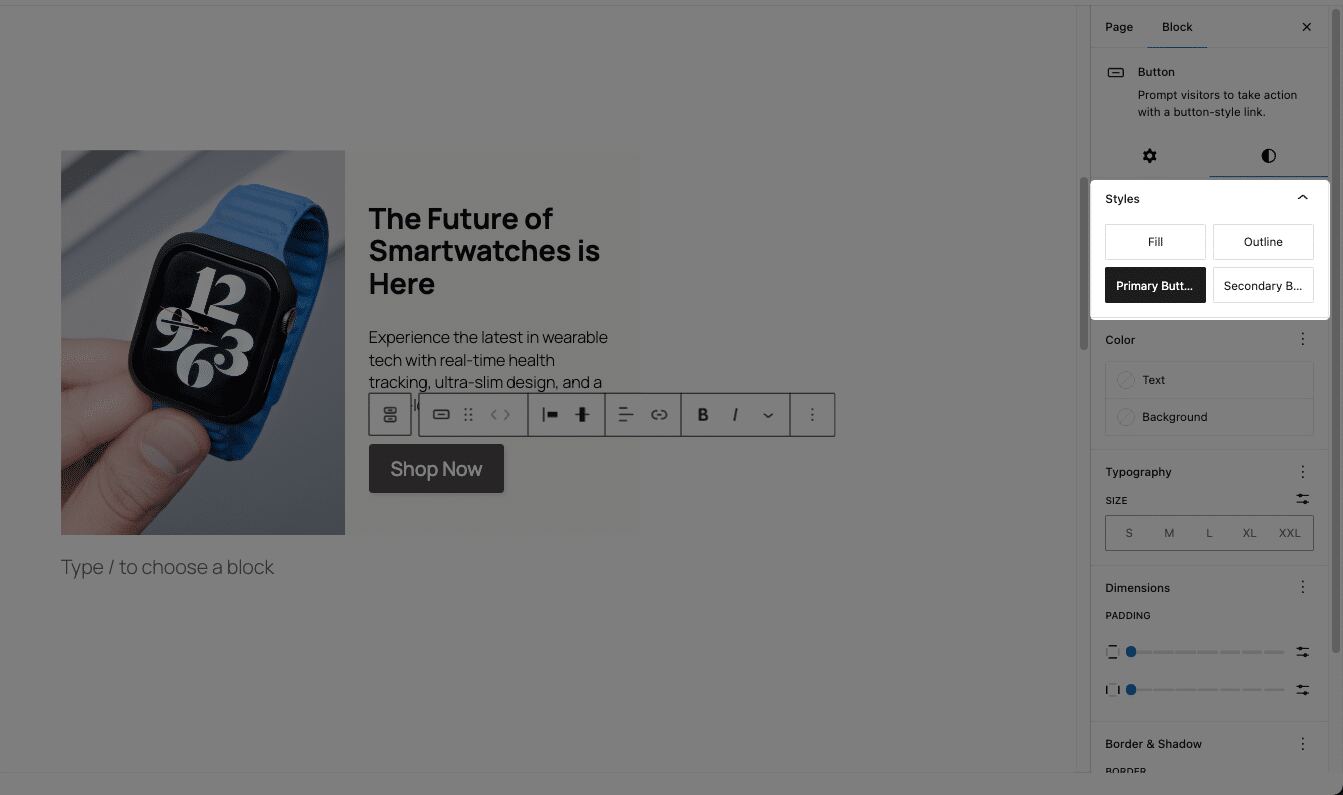
The principle button has a forged darkish grey background, whilst the secondary button has a clear background with a border. Each buttons have constant padding, border radius, and font styling, making sure a certified look around the web site.
Step 2: Limiting Button Customization
Whilst the button types at the moment are predefined, customers may just nonetheless manually override them the usage of WordPress’s block editor settings. If they alter the colours, padding, or typography, the buttons might not fit the emblem pointers.
We will be able to use the Helps API to disable those customization choices, combating customers from making accidental adjustments. Upload this to purposes.php
:
serve as restrict_button_customization($args, $block_type) {
if ($block_type === 'core/button') {
// Disable explicit shade settings (textual content, background, hyperlink)
$args['supports']['color'] = [
'text' => false,
'background' => false,
'link' => false,
];
// Disable typography settings (font length, font weight, line peak)
$args['supports']['typography'] = false;
// Disable spacing settings (padding, margin)
$args['supports']['spacing'] = false;
}
go back $args;
}
add_filter('register_block_type_args', 'restrict_button_customization', 10, 2);
With this in position:
- Customers can’t alternate button colours, so all buttons will have to adhere to the emblem’s shade scheme.
- Typography controls are got rid of, conserving textual content formatting constant.
- Spacing changes are disabled, combating customers from editing padding and margins.
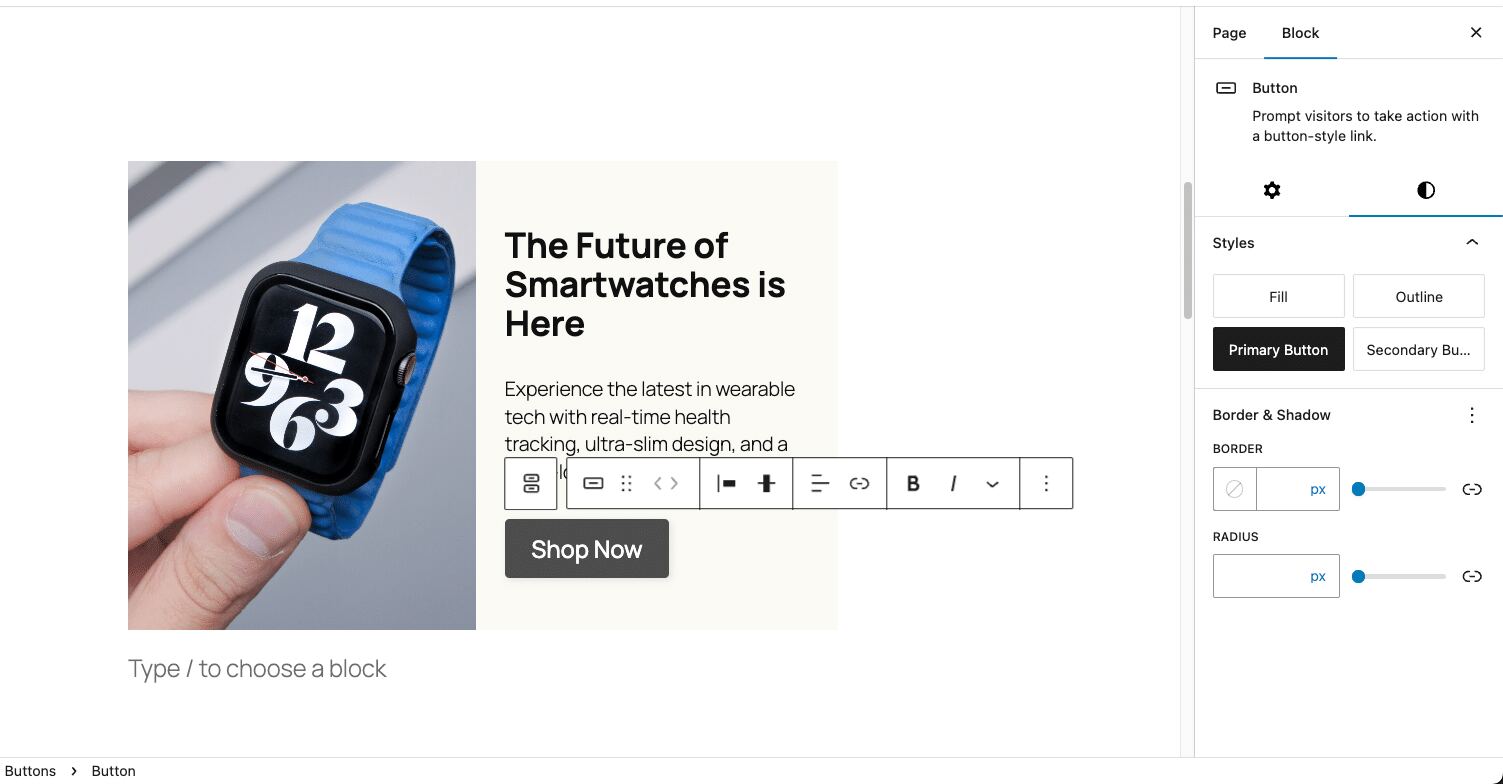
Now, as an alternative of letting customers create a random mixture of button types, they’ll merely make a choice from foremost and secondary types, conserving the design skilled and structured.
Abstract
We’ve most effective scratched the skin of what’s imaginable with WordPress block customization. WordPress supplies an infinite choice of APIs that make it simple to increase and customise blocks, permitting builders to tailor the editor revel in whilst conserving issues structured and user-friendly.
For those who’re occupied with mastering block customization, there’s so a lot more to discover. Take a look at the legitimate WordPress developer documentation, attend WordCamps and meetups, and continue learning from nice sources just like the Kinsta weblog, the place we frequently destroy down complex WordPress ideas.
In fact, having a forged webhosting supplier makes experimenting with those adjustments easy. Whether or not you’re checking out out new block adjustments or managing a heavy-traffic web site, dependable webhosting guarantees easy efficiency and simple scalability. At Kinsta, we’ve constructed our platform to deal with precisely that. In reality, we had been not too long ago ranked #1 in WordPress Internet hosting by way of G2, because of over 930 buyer critiques.
This speaks for itself: You’ll be able to accept as true with us to energy your WordPress revel in.
The put up The way to lengthen core WordPress blocks with Blocks API seemed first on Kinsta®.
WP Hosting