In relation to the backend, builders ultimately come across routes. Routes can also be thought to be the spine of the backend since each request the server receives is redirected to a controller via a routing listing that maps requests to controllers or movements.
Laravel hides many implementation main points for us and is derived with a large number of syntactic sugar to lend a hand each new and skilled builders broaden their internet programs.
Let’s take a detailed take a look at how you can arrange routes in Laravel.
Backend Routing and Pass-Website online Scripting in Laravel
On a server, there exist each private and non-private routes. Public routes is usually a reason of shock because of the likelihood for cross-site scripting (XSS), a kind of injection assault that may depart you and your customers at risk of malicious actors.
The issue is {that a} consumer can also be redirected from a course that doesn’t require a consultation token to 1 that does — and so they’ll nonetheless have get admission to with out the token.
The most simple technique to clear up this factor is to put in force a brand new HTTP header, including “referrer” to the path to mitigate this state of affairs:
'major' => [
'path' => '/main',
'referrer' => 'required,refresh-empty',
'target' => ControllerDashboardController::class . '::mainAction'
]
Laravel Elementary Routing
In Laravel, routes permit customers to course the fitting request to the required controller. Essentially the most elementary Laravel Direction accepts a Uniform Asset Identifier (your course trail) and a closure which can also be each a serve as or a category.
In Laravel, routes are created throughout the internet.php and api.php recordsdata. Laravel comes with two routes through default: one for the WEB and one for the API.
Those routes live within the routes/ folder, however they’re loaded within the Suppliers/RouteServiceProvider.php.
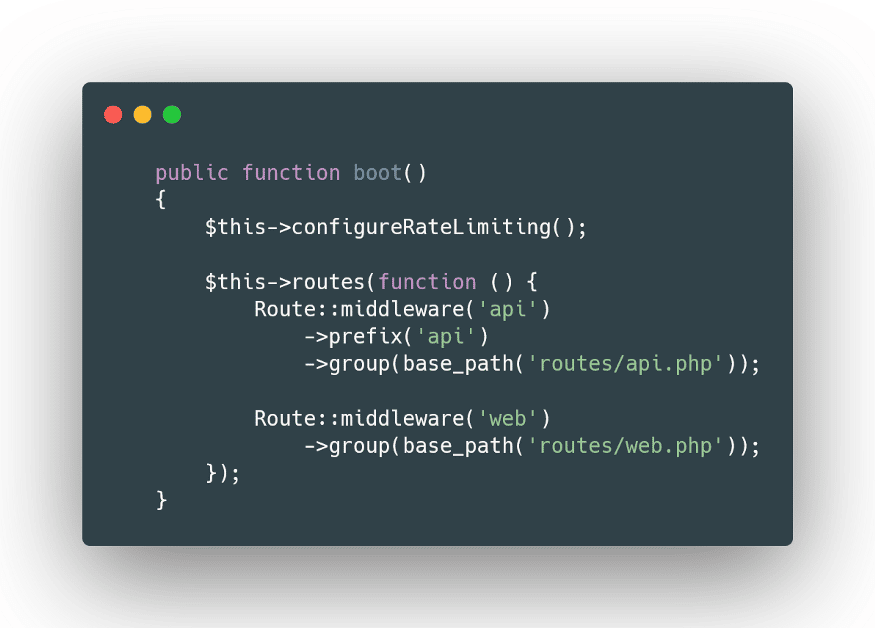
As an alternative of doing this, we will load the routes immediately within RouteServiceProvider.php, skipping the routes/ folder altogether.
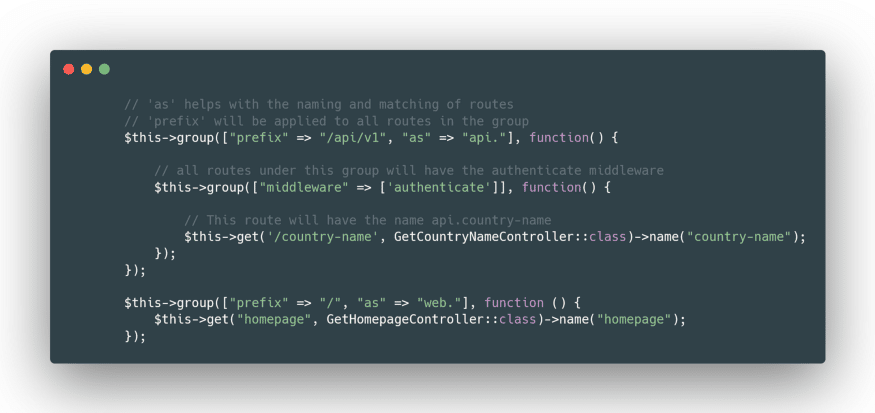
Redirects
Once we outline a course, we’ll generally wish to redirect the consumer that accesses it, and the explanations for this range so much. It can be as it’s a deprecated course and we’ve got modified the backend or the server, or it can be as a result of we wish to set up two-factor authentication (2FA), and so forth.
Laravel has a very simple means of doing this. Due to the framework’s simplicity, we will use the redirect approach at the Direction facade, which accepts the access course and the path to be redirected to.
Optionally, we will give the standing code for the redirect because the 1/3 parameter. The permanentRedirect
approach will do the similar because the redirect
approach, besides it is going to all the time go back a 301 standing code:
// Easy redirect
Direction::redirect("/magnificence", "/myClass");
// Redirect with customized standing
Direction::redirect("/house", "/administrative center", 305);
// Direction redirect with 301 standing code
Direction::permanentRedirect("/house", "administrative center");
Within the redirect routes we’re forbidden to make use of the “vacation spot” and “standing” key phrases as parameters as they’re reserved through Laravel.
// Unlawful to make use of
Direction::redirect("/house", "/administrative center/{standing}");
Perspectives
Perspectives are the .blade.php recordsdata that we use to render the frontend of our Laravel utility. It makes use of the blade templating engine, and it’s the default technique to construct a full-stack utility the use of solely Laravel.
If we would like our course to go back a view, we will merely use the view approach at the Direction facade. It accepts a course parameter, a view title, and an non-compulsory array of values to be handed to the view.
// When the consumer accesses my-domain.com/homepage
// the homepage.blade.php record might be rendered
Direction::view("/homepage", "homepage");
Let’s suppose our view desires to mention “Hi, {title}
” through passing an non-compulsory array with that parameter. We will just do that with the next code (if the lacking parameter is needed within the view, the request will fail and throw an error):
Direction::view('/homepage', 'homepage', ['name' => "Kinsta"]);
Direction Record
As your utility grows in dimension, so will the selection of requests that wish to be routed. And with a perfect quantity of knowledge can come nice confusion.
That is the place the artisan course:listing command
can lend a hand us. It supplies an outline of all of the routes which are outlined within the utility, their middlewares, and controllers.
php artisan course:listing
It’s going to show an inventory of all routes with out the middlewares. For this, we need to use the -v
flag:
php artisan course:listing -v
In a scenario the place you’ll be the use of domain-driven design the place your routes have particular names of their paths, you’ll be able to employ the filtering features of this command like so:
php artisan course:listing –trail=api/account
This will likely display solely the routes that get started with api/account.
Then again, we will instruct Laravel to exclude or come with third-party outlined routes through the use of the –except-vendor
or –only-vendor
choices.
Direction Parameters
Now and again you could wish to seize segments of the URI with the course, like a consumer ID or token. We will do that through defining a course parameter, which is all the time encased inside of curly braces ({}
) and must solely include alphabetic characters.
If our routes have dependencies within their callbacks, the Laravel provider container will routinely inject them:
use IlluminateHttpRequest;
use Controllers/DashboardController;
Direction::put up('/dashboard/{identity}, serve as (Request $request, string $identity) {
go back 'Consumer:' . $identity;
}
Direction::get('/dashboard/{identity}, DashboardController.php);
Required Parameters
Laravel’s required parameters are parameters in routes that we aren’t allowed to skip after we make a choice. Another way, an error might be thrown:
Direction::put up("/gdpr/{userId}", GetGdprDataController.php");
Now throughout the GetGdprDataController.php we can have direct get admission to to the $userId parameter.
public serve as __invoke(int $userId) {
// Use the userId that we won…
}
A course can take any numbers of parameters. They’re injected within the course callbacks/controllers in accordance with the order by which they’re indexed:
// api.php
Direction::put up('/gdpr/{userId}/{userName}/{userAge}', GetGdprDataController.php);
// GetGdprDataController.php
public serve as __invoke(int $userId, string $userName, int $userAge) {
// Use the parameters…
}
Non-compulsory Parameters
In a scenario the place we wish to do one thing on a course when just a parameter is provide and not anything else, all with out affecting all the utility, we will upload an non-compulsory parameter. Those non-compulsory parameters are denoted through the ?
appended to them:
Direction::get('/consumer/{age?}', serve as (int $age = null) {
if (!$age) Log::data("Consumer does not have age set");
else Log::data("Consumer's age is " . $age);
}
Direction::get('/consumer/{title?}', serve as (int $title = "John Doe") {
Log::data("Consumer's title is " . $title);
}
Direction Wildcard
Laravel supplies some way for us to filter out what our non-compulsory or required parameters must appear to be.
Say we would like a string of a consumer ID. We will validate it like so at the course stage the use of the the place
approach.
The the place
approach accepts the title of the parameter and the regex rule that might be implemented at the validation. By means of default, it takes the primary parameter, but when we’ve got many, we will go an array with the title of the parameter as the important thing and the guideline as the price, and Laravel will parse them considering us:
Direction::get('/consumer/{age}', serve as (int $age) {
//
}->the place('age', '[0-9]+');
Direction::get('/consumer/{age}', serve as (int $age) {
//
}->the place('[0-9]+');
Direction::get('/consumer/{age}/{title}', serve as (int $age, string $title) {
//
}->the place(['age' => '[0-9]+', 'title' => '[a-z][A-z]+');
We will take this a step additional and practice validation on all of the routes in our utility through the use of the development
approach at the Direction
facade:
Direction::development('identity', '[0-9]+');
This will likely valide each identity
parameter with this regex expression. And when we outline it, it is going to be routinely implemented to all routes the use of that parameter title.
As we will see, Laravel is the use of the /
persona as a separator within the trail. If we wish to use it within the trail, we need to explicitly permit it to be a part of our placeholder the use of a the place
regex.
Direction::get('/in finding/{question}', serve as ($question) {
//
})->the place('question', , '.*');
The one problem is that it is going to be supported solely within the final course phase.
Named Routes
Because the title suggests, we will title routes, which makes it handy to generate URLs or redirect for particular routes.
How To Create Named Routes
A easy technique to create a named course is equipped through the title
approach chained at the Direction
facade. Every course’s title must be distinctive:
Direction::get('/', serve as () {
})->title("homepage");
Direction Teams
Direction teams can help you proportion course attributes like middlewares throughout a lot of routes without having to re-define it on every course.
Middleware
Assigning a middleware to all routes we’ve got permits us to mix them in a gaggle, first through the use of the staff
approach. Something to imagine is that the middlewares are finished within the order by which they’re implemented to the crowd:
Direction:middleware(['AuthMiddleware', 'SessionMiddleware'])->staff(serve as () {
Direction::get('/', serve as() {} );
Direction::put up('/upload-picture', serve as () {} );
});
Controllers
When a gaggle makes use of the similar controller, we will use the controller
approach to outline the average controller for all of the routes inside of that staff. Now we need to specify the process that the course will name.
Direction::controller(UserController::magnificence)->staff(serve as () {
Direction::get('/orders/{userId}', 'getOrders');
Direction::put up('/order/{identity}', 'postOrder');
});
Subdomain Routing
A subdomain title is a work of extra knowledge added to the start of a web site’s area title. This permits web sites to split and arrange their content material for particular purposes, reminiscent of on-line shops, blogs, shows, and so forth from the remainder of the web site.
Our routes can be utilized to take care of subdomain routing. We will catch the area and a portion of the subdomain for utilization in our controller and course. With the assistance of the area
approach at the Direction
facade, we will staff our routes beneath a unmarried area:
Direction::area('{retailer}.endeavor.com')->staff(serve as() {
Direction::get('order/{identity}', serve as (Account $account, string $identity) {
// Your Code
}
});
Prefixes and Identify Prefixes
Every time we’ve got a gaggle of routes, as an alternative of enhancing them separately, we will employ the additional utilities that Laravel supplies, reminiscent of prefix
and title
at the Direction
facade.
The prefix
approach can be utilized to prefix each and every course within the staff with a given URI, and the title
approach can be utilized to prefix each and every course title with a given string.
This permits us to create new such things as admin routes with out being required to change every title or prefix to spot them:
Direction::title('admin.")->staff(serve as() {
Direction::prefix("admin")->staff(serve as() {
Direction::get('/get')->title('get');
Direction::put('/put')->title(put');
Direction::put up('/put up')->title('put up');
});
});
Now the URIs for those routes might be admin/get
, admin/put
, admin/put up
, and the names admin.get
, admin.put
, and admin.put up
.
Direction Caching
When deploying the appliance to manufacturing servers, a excellent Laravel developer will profit from Laravel’s course cache.
What Is Direction Caching?
Direction caching decreases the period of time it takes to check in all of the utility routes.
Operating php artisan course:cache
an example of Remove darkness from/Routing/RouteCollection
is generated, and after being encoded, the serialized output is written to bootstrap/cache.routes.php
.
Now some other request will load this cache record if it exists. Due to this fact, our utility not has to parse and convert entries from the course record into Remove darkness from/Routing/Direction
gadgets in Remove darkness from/Routing/RouteCollection
.
Why It’s Necessary To Use Direction Caching
By means of no longer the use of the course caching characteristic that Laravel supplies, your utility dangers working extra slowly than it might be, which might in flip lower gross sales, consumer retention, and agree with on your emblem.
Relying at the scale of your challenge and what number of routes there are, working a easy course caching command can accelerate your utility through anyplace from 130% to 500% — a large acquire for just about no effort.
Abstract
Routing is the spine of backend building. The Laravel framework excels at this through offering a verbose means of defining and managing routes.
Construction can certainly be out there for everybody and lend a hand accelerate an utility simply by distinctive feature of it being in-built Laravel.
What different methods and pointers have you ever encountered relating to Laravel routes? Tell us within the feedback segment!
The put up Mastering Laravel Routes gave the impression first on Kinsta®.
WP Hosting