At the present time, React is likely one of the hottest JavaScript libraries. It may be used to create dynamic and responsive packages, permits for higher efficiency, and may also be simply prolonged. The underlying common sense is in accordance with parts that may be reused in several contexts, lowering the wish to write the similar code a number of instances. Briefly, with React you’ll be able to create environment friendly and robust packages.
So there hasn’t ever been a greater time to learn to create React packages.
Then again, with no cast working out of a few key JavaScript options, construction React packages could be tough and even inconceivable.
Because of this, now we have compiled a listing of JavaScript options and ideas that you wish to have to grasp earlier than getting began with React. The simpler you already know those ideas, the better it’s going to be so that you can construct skilled React packages.
That being stated, here’s what we will be able to talk about on this article:
JavaScript and ECMAScript
JavaScript is a well-liked scripting language used in conjunction with HTML and CSS to construct dynamic internet pages. Whilst HTML is used to create the construction of a internet web page and CSS to create the way and format of its parts, JavaScript is the language used so as to add habits to the web page, i.e. to create capability and interactivity.
The language has since been followed by means of the most important browsers and a report was once written to explain the best way JavaScript was once supposed to paintings: the ECMAScript same old.
As of 2015, an replace of the ECMAScript same old is launched every year, and thus new options are added to JavaScript annually.
ECMAScript 2015 was once the 6th unlock of the usual and is subsequently often referred to as ES6. Following variations are marked in development, so we discuss with ECMAScript 2016 as ES7, ECMAScript 2017 as ES8, and so forth.
Because of the frequency with which new options are added to the usual, some will not be supported in all browsers. So how may just you make certain that the newest JavaScript options you added in your JS app would paintings as anticipated throughout all internet browsers?
You have got 3 choices:
- Wait till all main browsers supply improve for the brand new options. However if you happen to completely want that incredible new JS characteristic on your app, this isn’t an choice.
- Use a Polyfill, which is “a work of code (most often JavaScript at the Internet) used to supply trendy capability on older browsers that don’t natively improve it” (see additionally mdn internet medical doctors).
- Use a JavaScript transpiler equivalent to Babel or Traceur, that convert ECMAScript 2015+ code right into a JavaScript model this is supported by means of all browsers.
Statements vs Expressions
Figuring out the adaptation between statements and expressions is very important when construction React packages. So, allow us to return to the elemental ideas of programming for a second.
A pc program is a listing of directions to be finished by means of a pc. Those directions are referred to as statements.
In contrast to statements, expressions are fragments of code that produce a worth. In a observation, an expression is a component that returns a worth and we most often see it at the proper aspect of an equivalent signal.
While:
JavaScript statements may also be blocks or traces of code that most often finish with semicolons or are enclosed in curly brackets.
Right here is an easy instance of a observation in JavaScript:
report.getElementById("hi").innerHTML = "Hi Global!";
The observation above writes "Hi Global!"
in a DOM part with identification="hi"
.
As we already discussed, expessions produce a worth or are themselves a worth. Believe the next instance:
msg = report.getElementById("hi").worth;
report.getElementById("hi").worth
is en expression because it returns a worth.
An extra instance will have to lend a hand explain the adaptation between expressions and statements:
const msg = "Hi Global!";
operate sayHello( msg ) {
console.log( msg );
}
Within the instance above,
- the primary line is a observation, the place
"Hi Global!"
is an expression, - the operate declaration is a observation, the place the parameter
msg
handed to the operate is an expression, - the road that prints the message within the console is a observation, the place once more the parameter
msg
is an expression.
Why Expressions Are Essential in React
When construction a React software, you’ll be able to inject JavaScript expressions into your JSX code. As an example, you’ll be able to move a variable, write an match handler or a situation. To try this, you wish to have to incorporate your JS code in curly brackets.
As an example, you’ll be able to move a variable:
const Message = () => {
const call = "Carlo";
go back Welcome {call}!
;
}
Briefly, the curly brackets inform your transpiler to procedure the code wrapped in brackets as JS code. The whole lot that comes earlier than the outlet
tag and after the final
tag is standard JavaScript code. The whole lot throughout the opening
and shutting
tags is processed as JSX code.
Here’s some other instance:
const Message = () => {
const call = "Ann";
const heading = Welcome {call}
;
go back (
{heading}
That is your dashboard.
);
}
You’ll additionally move an object:
render(){
const individual = {
call: 'Carlo',
avatar: 'https://en.gravatar.com/userimage/954861/fc68a728946aac04f8531c3a8742ac22',
description: 'Content material Author'
}
go back (
Welcome {individual.call}
Description: {individual.description}.
);
}
And beneath is a extra complete instance:
render(){
const individual = {
call: 'Carlo',
avatar: 'https://en.gravatar.com/userimage/954861/fc68a728946aac04f8531c3a8742ac22?measurement=authentic',
description: 'Content material Author',
theme: {
boxShadow: '0 4px 8px 0 rgba(0,0,0,0.2)', width: '200px'
}
}
go back (
{individual.call}
{individual.description}.
);
}
Understand the double curly brackets within the taste
attributes within the parts img
and div
. We used double brackets to move two gadgets containing card and symbol types.
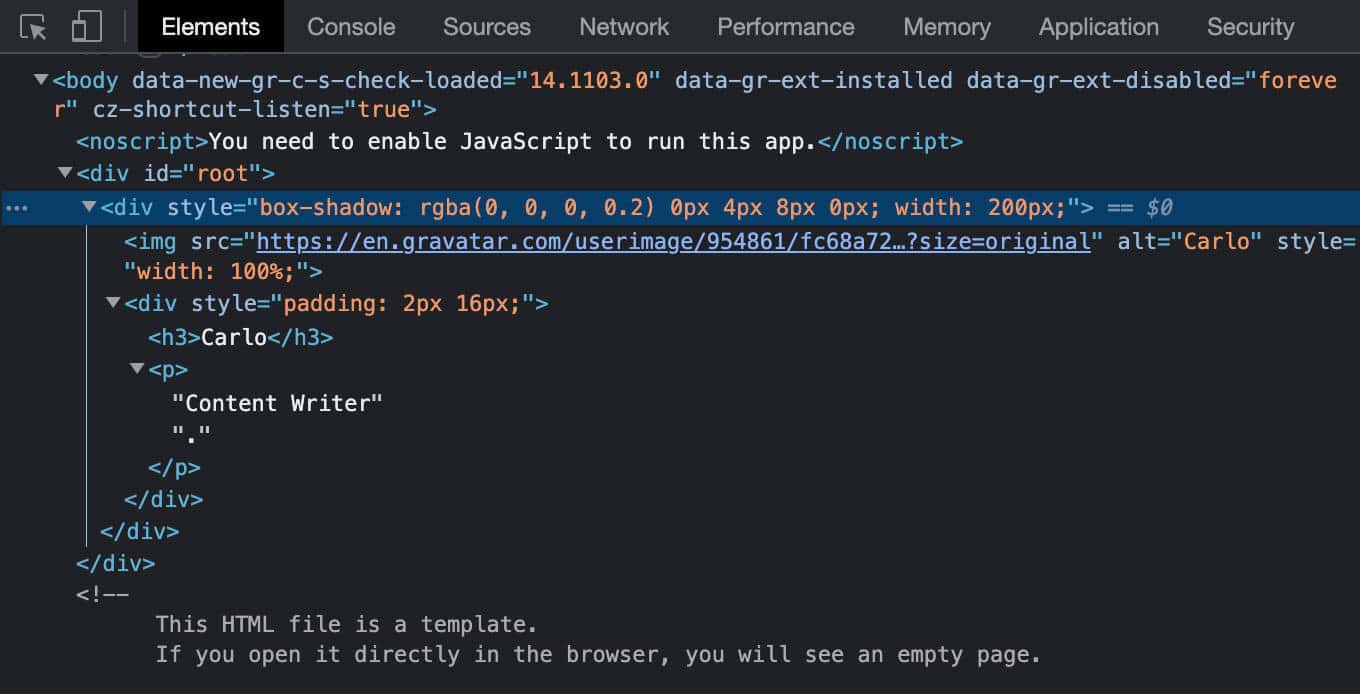
You will have spotted that during all of the examples above, we integrated JavaScript expressions in JSX.
Immutability in React
Mutability and Immutability are two key ideas in object-oriented and purposeful programming.
Immutability signifies that a worth can’t be modified after it’s been created. Mutability method, in fact, the other.
In Javascript, primitive values are immutable, that means that after a primitive worth is created, it can’t be modified. Conversely, arrays and gadgets are mutable as a result of their houses and parts may also be modified with out reassigning a brand new worth.
There are a number of causes for the usage of immutable gadgets in JavaScript:
- Advanced efficiency
- Diminished reminiscence intake
- Thread-safety
- More straightforward coding and debugging
Following the trend of immutability, as soon as a variable or object is assigned, it can’t be re-assigned or modified. When you wish to have to switch knowledge, you will have to create a replica of it and alter its content material, leaving the unique content material unchanged.
Immutability may be a key idea in React.
The React documentation states:
The state of a category element is to be had as
this.state
. The state box should be an object. Don’t mutate the state at once. If you want to exchange the state, namesetState
with the brand new state.
On every occasion the state of an element adjustments, React calculates whether or not to re-render the element and replace the Digital DOM. If React didn’t have monitor of the former state, it might no longer resolve whether or not to re-render the element or no longer. React documentation supplies an very good instance of this.
What JavaScript options are we able to use to ensure the immutability of the state object in React? Let’s in finding out!
Stating Variables
You have got 3 ways to claim a variable in JavaScript: var
, let
, and const
.
The var
observation exists because the starting of JavaScript. It’s used to claim a function-scoped or globally-scoped variable, optionally initializing it to a worth.
Whilst you claim a variable the usage of var
, you’ll be able to re-declare and replace that variable each within the world and native scope. The next code is permitted:
// Claim a variable
var msg = "Hi!";
// Redeclare the similar variable
var msg = "Good-bye!"
// Replace the variable
msg = "Hi once more!"
var
declarations are processed earlier than any code is finished. Because of this, mentioning a variable anyplace within the code is similar to mentioning it on the most sensible. This habits is named hoisting.
It’s price noting that solely the variable declaration is hoisted, no longer the initialization, which solely occurs when the regulate go with the flow reaches the project observation. Till that time, the variable is undefined
:
console.log(msg); // undefined
var msg = "Hi!";
console.log(msg); // Hi!
The scope of a var
declared in a JS operate is the entire frame of that operate.
Which means that the variable isn’t outlined at block degree, however on the degree of all the operate. This ends up in numerous issues that may make your JavaScript code buggy and hard to deal with.
To mend those issues, ES6 offered the let
key phrase.
The
let
declaration pronounces a block-scoped native variable, optionally initializing it to a worth.
What are some great benefits of let
over var
? Listed here are some:
let
pronounces a variable to the scope of a block observation, whilstvar
pronounces a variable globally or in the community to a whole operate irrespective of block scope.- World
let
variables don’t seem to be houses of thewindow
object. You can’t get admission to them withwindow.variableName
. let
can solely be accessed after its declaration is reached. The variable isn’t initialized till the regulate go with the flow reaches the road of code the place it’s declared (let declarations are non-hoisted).- Redeclaring a variable with
let
throws aSyntaxError
.
Since variables declared the usage of var
can’t be block-scoped, if you happen to outline a variable the usage of var
in a loop or inside of an if
observation, it may be accessed from outdoor the block and this can result in buggy code.
The code within the first instance is finished with out mistakes. Now change var
with let
within the block of code noticed above:
console.log(msg);
let msg = "Hi!";
console.log(msg);
In the second one instance, the usage of let
as an alternative of var
produces an Uncaught ReferenceError
:

ES6 additionally introduces a 3rd key phrase: const
.
const
is lovely very similar to let
, however with a key distinction:
Believe the next instance:
const MAX_VALUE = 1000;
MAX_VALUE = 2000;
The above code would generate the next TypeError:
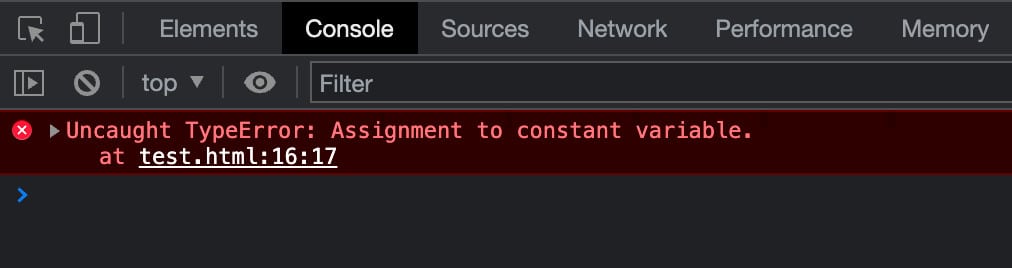
As well as:
Stating a const
with out giving it a worth would throw the next SyntaxError
(see additionally ES6 In Intensity: let and const):

But when a continuing is an array or an object, you’ll be able to edit houses or pieces inside of that array or object.
As an example, you’ll be able to exchange, upload, and take away array pieces:
// Claim a continuing array
const towns = ["London", "New York", "Sydney"];
// Trade an merchandise
towns[0] = "Madrid";
// Upload an merchandise
towns.push("Paris");
// Take away an merchandise
towns.pop();
console.log(towns);
// Array(3)
// 0: "Madrid"
// 1: "New York"
// 2: "Sydney"
However you don’t seem to be allowed to reassign the array:
const towns = ["London", "New York", "Sydney"];
towns = ["Athens", "Barcelona", "Naples"];
The code above would lead to a TypeError.

You’ll upload, reassign, and take away object houses and techniques:
// Claim a continuing obj
const put up = {
identification: 1,
call: 'JavaScript is superior',
excerpt: 'JavaScript is an excellent scripting language',
content material: 'JavaScript is a scripting language that lets you create dynamically updating content material.'
};
// upload a brand new assets
put up.slug = "javascript-is-awesome";
// Reassign assets
put up.identification = 5;
// Delete a assets
delete put up.excerpt;
console.log(put up);
// {identification: 5, call: 'JavaScript is superior', content material: 'JavaScript is a scripting language that lets you create dynamically updating content material.', slug: 'javascript-is-awesome'}
However you don’t seem to be allowed to reassign the article itself. The next code would undergo an Uncaught TypeError
:
// Claim a continuing obj
const put up = {
identification: 1,
call: 'JavaScript is superior',
excerpt: 'JavaScript is an excellent scripting language'
};
put up = {
identification: 1,
call: 'React is strong',
excerpt: 'React allows you to construct person interfaces'
};
Object.freeze()
We now agree that the usage of const
does no longer all the time ensure sturdy immutability (particularly when running with gadgets and arrays). So, how are you able to put into effect the immutability trend to your React packages?
First, when you need to stop the weather of an array or houses of an object from being changed, you’ll be able to use the static system Object.freeze()
.
Freezing an object prevents extensions and makes current houses non-writable and non-configurable. A frozen object can now not be modified: new houses can’t be added, current houses can’t be got rid of, their enumerability, configurability, writability, or worth can’t be modified, and the article’s prototype can’t be re-assigned.
freeze()
returns the similar object that was once handed in.
Any try to upload, exchange or take away a assets will fail, both silently or by means of throwing a TypeError
, maximum regularly in strict mode.
You’ll use Object.freeze()
this manner:
'use strict'
// Claim a continuing obj
const put up = {
identification: 1,
call: 'JavaScript is superior',
excerpt: 'JavaScript is an excellent scripting language'
};
// Freeze the article
Object.freeze(put up);
For those who now attempt to upload a assets, you are going to obtain an Uncaught TypeError
:
// Upload a brand new assets
put up.slug = "javascript-is-awesome"; // Uncaught TypeError
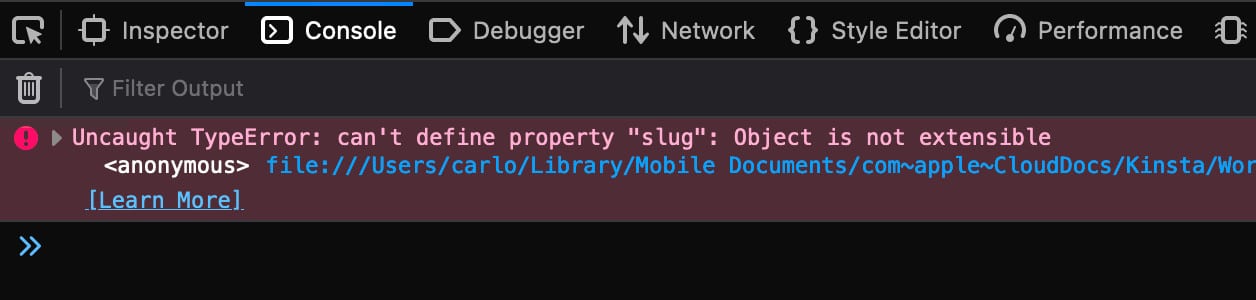
Whilst you attempt to reassign a assets, you get some other roughly TypeError
:
// Reassign assets
put up.identification = 5; // Uncaught TypeError
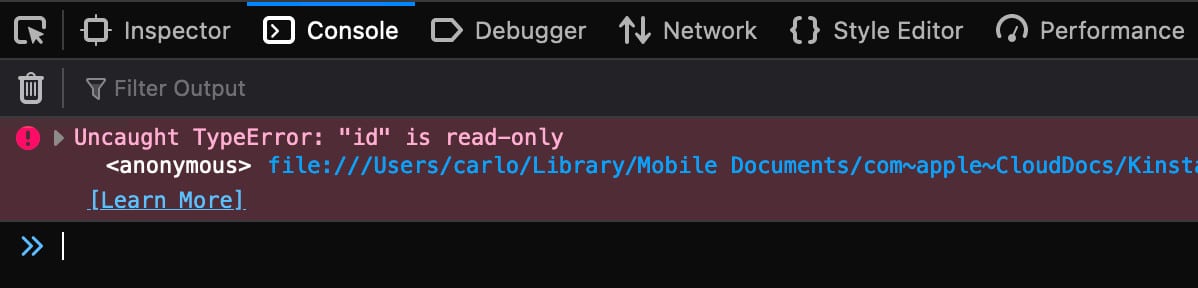
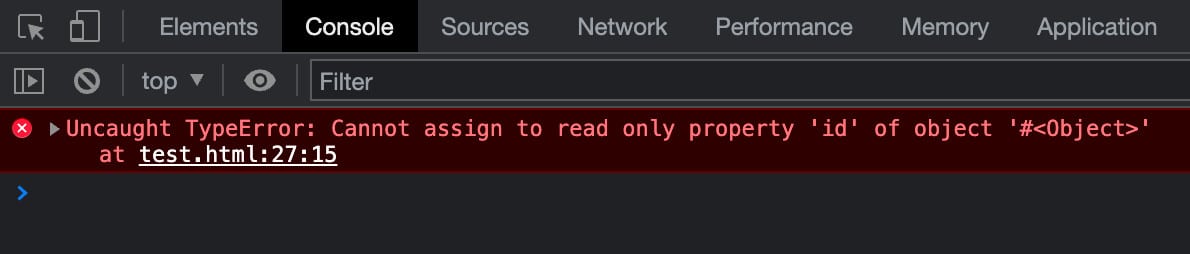
You’ll additionally attempt to delete a assets. The outcome will probably be some other TypeError
:
// Delete a assets
delete put up.excerpt; // Uncaught TypeError
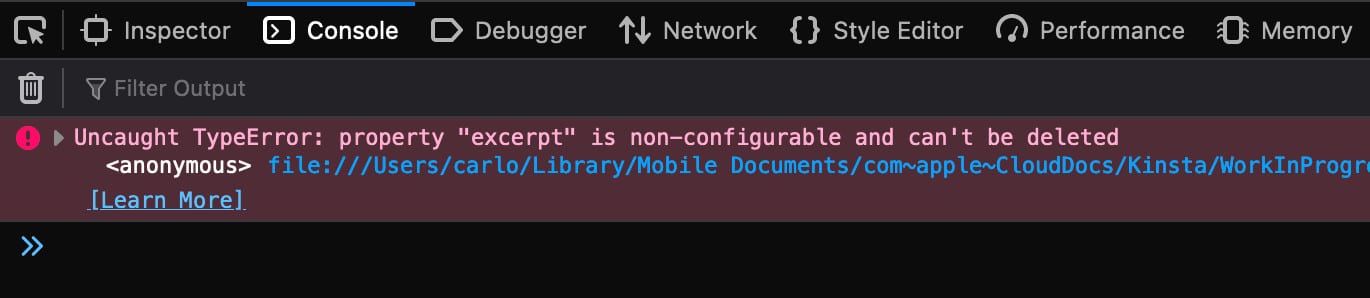
Template Literals
When you wish to have to mix strings with the output of expressions in JavaScript, you most often use the addition operator +
. Then again, you’ll be able to additionally use a JavaScript characteristic that lets you come with expressions inside of strings with out the usage of the addition operator: Template Literals.
Template Literals are a unique roughly strings delimited with backtick (`
) characters.
In Template Literals you’ll be able to come with placeholders, that are embedded expressions delimited by means of a buck persona and wrapped in curly brackets.
Here’s an instance:
const align = 'left';
console.log(`This string is ${ align }-aligned`);
The strings and placeholders get handed to a default operate that plays string interpolation to change the placeholders and concatenate the portions right into a unmarried string. You’ll additionally change the default operate with a customized operate.
You’ll use Template Literals for:
Multi-line strings: newline characters are a part of the template literal.
console.log(`Twinkle, twinkle, little bat!
How I'm wondering what you’re at!`);
String interpolation: With out Template Literals, you’ll be able to solely use the addition operator to mix the output of expressions with strings. See the next instance:
const a = 3;
const b = 7;
console.log("The results of " + a + " + " + b + " is " + (a + b));
It’s slightly complicated, isn’t it? However you’ll be able to write that code in a extra readable and maintainable approach the usage of Template Literals:
const a = 3;
const b = 7;
console.log(`The results of ${ a } + ${ b } is ${ a + b }`);
However remember the fact that there’s a distinction between the 2 syntaxes:
Template Literals lend themselves to a number of makes use of. Within the following instance, we use a ternary operator to assign a worth to a elegance
characteristic.
const web page = 'archive';
console.log(`elegance=${ web page === 'archive' ?
'archive' : 'unmarried' }`);
Beneath, we’re appearing a easy calculation:
const worth = 100;
const VAT = 0.22;
console.log(`Overall worth: ${ (worth * (1 + VAT)).toFixed(2) }`);
It’s also imaginable to nest Template Literals by means of together with them inside of an ${expression}
placeholder (however use nested templates with warning as a result of complicated string constructions is also arduous to learn and deal with).
Tagged templates: As we discussed above, it is usually imaginable to outline a customized operate to accomplish string concatenation. This sort of Template Literal is named Tagged Template.
Tags let you parse template literals with a operate. The primary argument of a tag operate comprises an array of string values. The remainder arguments are associated with the expressions.
Tags let you parse template literals with a customized operate. The primary argument of this operate is an array of the strings integrated within the Template Literal, the opposite arguments are the expressions.
You’ll create a customized operate to accomplish any type of operation at the template arguments and go back the manipulated string. Here’s a very fundamental instance of tagged template:
const call = "Carlo";
const function = "scholar";
const group = "North Pole College";
const age = 25;
operate customFunc(strings, ...tags) {
console.log(strings); // ['My name is ', ", I'm ", ', and I am ', ' at ', '', raw: Array(5)]
console.log(tags); // ['Carlo', 25, 'student', 'North Pole University']
let string = '';
for ( let i = 0; i < strings.duration - 1; i++ ){
console.log(i + "" + strings[i] + "" + tags[i]);
string += strings[i] + tags[i];
}
go back string.toUpperCase();
}
const output = customFunc`My call is ${call}, I am ${age}, and I'm ${function} at ${group}`;
console.log(output);
The code above prints the strings
and tags
array parts then capitalizes the string characters earlier than printing the output within the browser console.
Arrow Purposes
Arrow purposes are an alternative choice to nameless purposes (purposes with out names) in JavaScript however with some variations and barriers.
The next declarations are all legitimate Arrow Purposes examples:
// Arrow operate with out parameters
const myFunction = () => expression;
// Arrow operate with one parameter
const myFunction = param => expression;
// Arrow operate with one parameter
const myFunction = (param) => expression;
// Arrow operate with extra parameters
const myFunction = (param1, param2) => expression;
// Arrow operate with out parameters
const myFunction = () => {
statements
}
// Arrow operate with one parameter
const myFunction = param => {
statements
}
// Arrow operate with extra parameters
const myFunction = (param1, param2) => {
statements
}
Chances are you'll put out of your mind the spherical brackets if you happen to solely move one parameter to the operate. For those who move two or extra parameters, you should enclose them in brackets. Here's an instance of this:
const render = ( identification, identify, class ) => `${identification}: ${identify} - ${class}`;
console.log( render ( 5, 'Hi Global!', "JavaScript" ) );
One-line Arrow Purposes go back a worth by means of default. For those who use the multiple-line syntax, you'll have to manually go back a worth:
const render = ( identification, identify, class ) => {
console.log( `Submit identify: ${ identify }` );
go back `${ identification }: ${ identify } - ${ class }`;
}
console.log( `Submit main points: ${ render ( 5, 'Hi Global!', "JavaScript" ) }` );
One key distinction between standard purposes and Arrow Purposes to remember is that Arrow purposes don’t have their very own bindings to the key phrase this
. For those who attempt to use this
in an Arrow Serve as, it's going to cross outdoor the operate scope.
For a better description of Arrow purposes and examples of use, learn additionally mdn internet medical doctors.
Categories
Categories in JavaScript are a unique form of operate for growing gadgets that use the prototypical inheritance mechanism.
Consistent with mdn internet medical doctors,
Relating to inheritance, JavaScript solely has one assemble: gadgets. Every object has a non-public assets which holds a hyperlink to some other object referred to as its prototype. That prototype object has a prototype of its personal, and so forth till an object is reached with
null
as its prototype.
As with purposes, you could have two tactics of defining a category:
- A category expression
- A category declaration
You'll use the elegance
key phrase to outline a category inside of an expression, as proven within the following instance:
const Circle = elegance {
constructor(radius) {
this.radius = Quantity(radius);
}
space() {
go back Math.PI * Math.pow(this.radius, 2);
}
circumference() {
go back Math.PI * this.radius * 2;
}
}
console.log('Circumference: ' + new Circle(10).circumference()); // 62.83185307179586
console.log('House: ' + new Circle(10).space()); // 314.1592653589793
A category has a frame, that's the code integrated in curly brakets. Right here you’ll outline constructor and techniques, that are also referred to as elegance participants. The frame of the category is finished in strict mode even with out the usage of the 'strict mode'
directive.
The constructor
system is used for growing and initializing an object created with a category and is robotically finished when the category is instantiated. For those who don’t outline a constructor system to your elegance, JavaScript will robotically use a default constructor.
A category may also be prolonged the usage of the extends
key phrase.
elegance E-book {
constructor(identify, writer) {
this.booktitle = identify;
this.authorname = writer;
}
provide() {
go back this.booktitle + ' is a smart e-book from ' + this.authorname;
}
}
elegance BookDetails extends E-book {
constructor(identify, writer, cat) {
tremendous(identify, writer);
this.class = cat;
}
display() {
go back this.provide() + ', this is a ' + this.class + ' e-book';
}
}
const bookInfo = new BookDetails("The Fellowship of the Ring", "J. R. R. Tolkien", "Myth");
console.log(bookInfo.display());
A constructor can use the tremendous
key phrase to name the mum or dad constructor. For those who move an issue to the tremendous()
system, this argument can also be to be had within the mum or dad constructor elegance.
For a deeper dive into JavaScript categories and several other examples of utilization, see additionally the mdn internet medical doctors.
Categories are frequently used to create React parts. Typically, you’ll no longer create your personal categories however somewhat lengthen integrated React categories.
All categories in React have a render()
system that returns a React part:
elegance Animal extends React.Element {
render() {
go back Whats up, I'm a {this.props.call}!
;
}
}
Within the instance above, Animal
is a category element. Consider that
- The call of the element should start with a capital letter
- The element should come with the expression
extends React.Element
. This offers get admission to to the strategies of theReact.Element
. - The
render()
system returns the HTML and is needed.
After getting created your elegance element, you'll be able to render the HTML at the web page:
const root = ReactDOM.createRoot(report.getElementById('root'));
const part = ;
root.render(part);
The picture beneath displays the outcome at the web page (You'll see it in motion on CodePen).
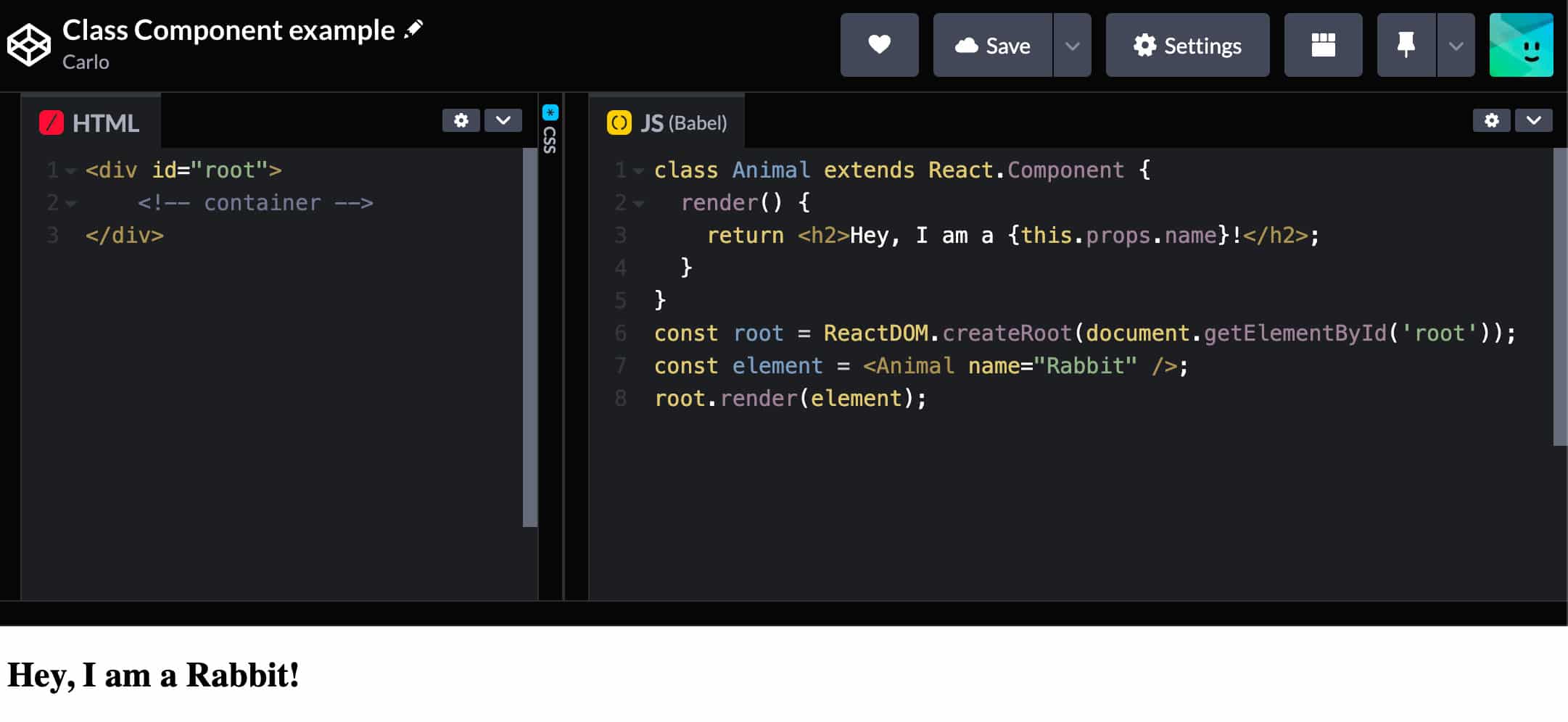
Observe, on the other hand, that the usage of elegance parts in React isn't advisable and it’s preferable defining parts as purposes.
The Key phrase ‘this’
In JavaScript, the this
key phrase is a generic placeholder most often used inside of gadgets, categories, and purposes, and it refers to other parts relying at the context or scope.
this
can be utilized within the world scope. For those who digit this
to your browser’s console, you get:
Window {window: Window, self: Window, report: report, call: '', location: Location, ...}
You'll get admission to any of the strategies and houses of the Window
object. So, if you happen to run this.location
to your browser’s console, you get the next output:
Location {ancestorOrigins: DOMStringList, href: 'https://kinsta.com/', starting place: 'https://kinsta.com', protocol: 'https:', host: 'kinsta.com', ...}
Whilst you use this
in an object, it refers back to the object itself. On this approach, you'll be able to discuss with the values of an object within the strategies of the article itself:
const put up = {
identification: 5,
getSlug: operate(){
go back `post-${this.identification}`;
},
identify: 'Superior put up',
class: 'JavaScript'
};
console.log( put up.getSlug );
Now let’s attempt to use this
in a operate:
const useThis = operate () {
go back this;
}
console.log( useThis() );
For those who don't seem to be in strict mode, you’ll get:
Window {window: Window, self: Window, report: report, call: '', location: Location, ...}
However if you happen to invoke strict mode, you get a special end result:
const doSomething = operate () {
'use strict';
go back this;
}
console.log( doSomething() );
On this case, the operate returns undefined
. That’s as a result of this
in a operate refers to its specific worth.
So the way to explicitly set this
in a operate?
First, you'll be able to manually assign houses and how one can the operate:
operate doSomething( put up ) {
this.identification = put up.identification;
this.identify = put up.identify;
console.log( `${this.identification} - ${this.identify}` );
}
new doSomething( { identification: 5, identify: 'Superior put up' } );
However you'll be able to additionally use name
, practice
, and bind
strategies, in addition to arrow purposes.
const doSomething = operate() {
console.log( `${this.identification} - ${this.identify}` );
}
doSomething.name( { identification: 5, identify: 'Superior put up' } );
The name()
system can be utilized on any operate and does precisely what it says: it calls the operate.
Moreover, name()
accepts every other parameter outlined within the operate:
const doSomething = operate( cat ) {
console.log( `${this.identification} - ${this.identify} - Class: ${cat}` );
}
doSomething.name( { identification: 5, identify: 'Superior put up' }, 'JavaScript' );
const doSomething = operate( cat1, cat2 ) {
console.log( `${this.identification} - ${this.identify} - Classes: ${cat1}, ${cat2}` );
}
doSomething.practice( { identification: 5, identify: 'Superior put up' }, ['JavaScript', 'React'] );
const put up = { identification: 5, identify: 'Superior put up', class: 'JavaScript' };
const doSomething = operate() {
go back `${this.identification} - ${this.identify} - ${this.class}`;
}
const bindRender = doSomething.bind( put up );
console.log( bindRender() );
A substitute for the choices mentioned above is the usage of arrow purposes.
Arrow operate expressions will have to solely be used for non-method purposes as a result of they don't have their very own
this
.
This makes arrow purposes in particular helpful with match handlers.
That’s as a result of “when the code is named from an inline match handler characteristic, its this
is ready to the DOM part on which the listener is positioned” (see mdn internet medical doctors).
However issues exchange with arrow purposes as a result of…
… arrow purposes determine
this
in accordance with the scope the arrow operate is outlined inside of, and thethis
worth does no longer exchange in accordance with how the operate is invoked.
Binding ‘this’ to Tournament Handlers in React
Relating to React, you could have a couple of tactics to make certain that the development handler does no longer lose its context:
1. The usage of bind()
throughout the render system:
import React, { Element } from 'react';
elegance MyComponent extends Element {
state = { message: 'Hi Global!' };
showMessage(){
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
go back( );
}
}
export default MyComponent;
2. Binding the context to the development handler within the constructor:
import React, { Element } from 'react';
elegance MyComponent extends Element {
state = { message: 'Hi Global!' };
constructor(props) {
tremendous(props);
this.showMessage = this.showMessage.bind( this );
}
showMessage(){
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
go back( );
}
}
export default MyComponent;
3. Outline the development handler the usage of arrow purposes:
import React, { Element } from 'react';
elegance MyComponent extends Element {
state = { message: 'Hi Global!' };
showMessage = () => {
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
go back( );
}
}
export default MyComponent;
4. The usage of arrow purposes within the render system:
import React, { Element } from 'react';
elegance MyComponent extends Element {
state = { message: 'Hi Global!' };
showMessage() {
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
go back( );
}
}
export default MyComponent;
Whichever system you select, while you click on the button, the browser console displays the next output:
This refers to: MyComponent {props: {…}, context: {…}, refs: {…}, updater: {…}, state: {…}, …}
The message is: Hi Global!
Ternary Operator
The conditional operator (or ternary operator) permits you to write easy conditional expressions in JavaScript. It takes 3 operands:
- a situation adopted by means of a query mark (
?
), - an expression to execute if the situation is truthy adopted by means of a semicolon (
:
), - a 2nd expression to execute if the situation is falsy.
const drink = personAge >= 18 ? "Wine" : "Juice";
It's also imaginable to chain a number of expressions:
const drink = personAge >= 18 ? "Wine" : personAge >= 6 ? "Juice" : "Milk";
Watch out, although, as a result of chaining a number of expressions can result in messy code this is tough to deal with.
The ternary operator is especially helpful in React, particularly to your JSX code, which solely accepts expressions in curly brackets.
As an example, you'll be able to use the ternary operator to set the price of an characteristic in accordance with a particular situation:
render(){
const individual = {
call: 'Carlo',
avatar: 'https://en.gravatar.com/...',
description: 'Content material Author',
theme: 'mild'
}
go back (
{individual.call}
{individual.description}.
);
}
Within the code above, we test the situation individual.theme === 'darkish'
to set the price of the taste
characteristic of the container div
.
Quick Circuit Analysis
The logical AND (&&
) operator evaluates operands from left to proper and returns true
if and provided that all operands are true
.
The logical AND is a short-circuit operator. Every operand is transformed to a boolean and, if the results of the conversion is false
, the AND operator stops and returns the unique worth of the falsy operand. If all values are true
, it returns the unique worth of the remaining operand.
Quick circuit analysis is a JavaScript characteristic regularly utilized in React because it permits you to output blocks of code in accordance with explicit stipulations. Right here is an instance:
{
displayExcerpt &&
put up.excerpt.rendered && (
{ put up.excerpt.rendered }
)
}
Within the code above, if displayExcerpt
AND put up.excerpt.rendered
evaluation to true
, React returns the general block of JSX.
To recap, “if the situation is true
, the part proper after &&
will seem within the output. Whether it is false
, React will forget about and skip it”.
Unfold Syntax
In JavaScript, unfold syntax permits you to extend an iterable part, equivalent to an array or object, into operate arguments, array literals, or object literals.
Within the following instance, we're unpacking an array in a operate name:
operate doSomething( x, y, z ){
go back `First: ${x} - 2nd: ${y} - 3rd: ${z} - Sum: ${x+y+z}`;
}
const numbers = [3, 4, 7];
console.log( doSomething( ...numbers ) );
You'll use the unfold syntax to replicate an array (even multidimensional arrays) or to concatenate arrays. Within the following examples, we concatenate two arrays in two other ways:
const firstArray = [1, 2, 3];
const secondArray = [4, 5, 6];
firstArray.push( ...secondArray );
console.log( firstArray );
Then again:
let firstArray = [1, 2, 3];
const secondArray = [4, 5, 6];
firstArray = [ ...firstArray, ...secondArray];
console.log( firstArray );
You'll additionally use the unfold syntax to clone or merge two gadgets:
const firstObj = { identification: '1', identify: 'JS is superior' };
const secondObj = { cat: 'React', description: 'React is straightforward' };
// clone object
const thirdObj = { ...firstObj };
// merge gadgets
const fourthObj = { ...firstObj, ...secondObj }
console.log( { ...thirdObj } );
console.log( { ...fourthObj } );
Destructuring Project
Some other syntactic construction you are going to frequently in finding utilized in React is the destructuring project syntax.
Within the following instance, we unpack values from an array:
const person = ['Carlo', 'Content writer', 'Kinsta'];
const [name, description, company] = person;
console.log( `${call} is ${description} at ${corporate}` );
And right here is an easy instance of destructuring project with an object:
const person = {
call: 'Carlo',
description: 'Content material creator',
corporate: 'Kinsta'
}
const { call, description, corporate } = person;
console.log( `${call} is ${description} at ${corporate}` );
However we will be able to do much more. Within the following instance, we unpack some houses of an object and assign the remainder houses to some other object the usage of the unfold syntax:
const person = {
call: 'Carlo',
circle of relatives: 'Daniele',
description: 'Content material creator',
corporate: 'Kinsta',
energy: 'swimming'
}
const { call, description, corporate, ...leisure } = person;
console.log( leisure ); // {circle of relatives: 'Daniele', energy: 'swimming'}
You'll additionally assign values to an array:
const person = [];
const object = { call: 'Carlo', corporate: 'Kinsta' };
( { call: person[0], corporate: person[1] } = object );
console.log( person ); // (2) ['Carlo', 'Kinsta']
Observe that the parentheses across the project observation are required when the usage of object literal destructuring project with no declaration.
For a better research of destructuring project, with a number of examples of use, please discuss with the mdn internet medical doctors.
filter out(), map(), and scale back()
JavaScript supplies a number of helpful strategies you’ll in finding frequently utilized in React.
filter out()
Within the following instance, we practice the filter out to the numbers
array to get an array whose parts are numbers more than 5:
const numbers = [2, 6, 8, 2, 5, 9, 23];
const end result = numbers.filter out( quantity => quantity > 5);
console.log(end result); // (4) [6, 8, 9, 23]
Within the following instance, we get an array of posts with the phrase ‘JavaScript’ integrated within the identify:
const posts = [
{id: 0, title: 'JavaScript is awesome', content: 'your content'},
{id: 1, title: 'WordPress is easy', content: 'your content'},
{id: 2, title: 'React is cool', content: 'your content'},
{id: 3, title: 'With JavaScript to the moon', content: 'your content'},
];
const jsPosts = posts.filter out( put up => put up.identify.contains( 'JavaScript' ) );
console.log( jsPosts );
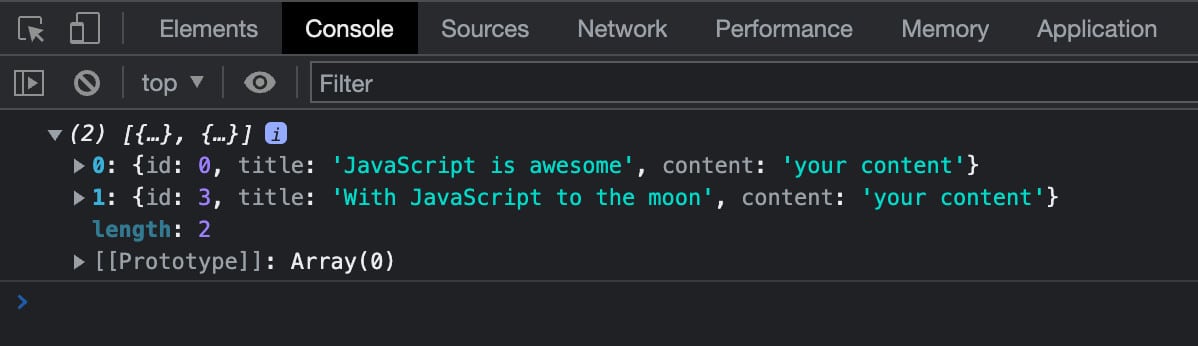
map()
const numbers = [2, 6, 8, 2, 5, 9, 23];
const end result = numbers.map( quantity => quantity * 5 );
console.log(end result); // (7) [10, 30, 40, 10, 25, 45, 115]
In a React element, you’ll frequently in finding the map()
system used to construct lists. Within the following instance, we’re mapping the WordPress posts
object to construct a listing of posts:
{ posts && posts.map( ( put up ) => {
go back (
-
{
put up.identify.rendered ?
put up.identify.rendered :
__( 'Default identify', 'author-plugin' )
}
)
})}
scale back()
scale back()
accepts two parameters:
- A callback operate to execute for every part within the array. It returns a worth that turns into the price of the accumulator parameter at the subsequent name. At the remaining name, the operate returns the price that would be the go back worth of
scale back()
. - An preliminary worth that's the first worth of the accumulator handed to the callback operate.
The callback operate takes a couple of parameters:
- An accumulator: The price returned from the former name to the callback operate. At the first name, it’s set to an preliminary worth if specified. Differently, it takes the price of the primary merchandise of the array.
- The price of the present part: The price is ready to the primary part of the array (
array[0]
) if an preliminary worth has been set, another way it takes the price of the second one part (array[1]
). - The present index is the index place of the present part.
An instance will make the whole lot clearer.
const numbers = [1, 2, 3, 4, 5];
const initialValue = 0;
const sumElements = numbers.scale back(
( accumulator, currentValue ) => accumulator + currentValue,
initialValue
);
console.log( numbers ); // (5) [1, 2, 3, 4, 5]
console.log( sumElements ); // 15
Let’s in finding out intimately what occurs at every iteration. Return to the former instance and alter the initialValue
:
const numbers = [1, 2, 3, 4, 5];
const initialValue = 5;
const sumElements = numbers.scale back(
( accumulator, currentValue, index ) => {
console.log('Accumulator: ' + accumulator + ' - currentValue: ' + currentValue + ' - index: ' + index);
go back accumulator + currentValue;
},
initialValue
);
console.log( sumElements );
The next symbol displays the output within the browser console:
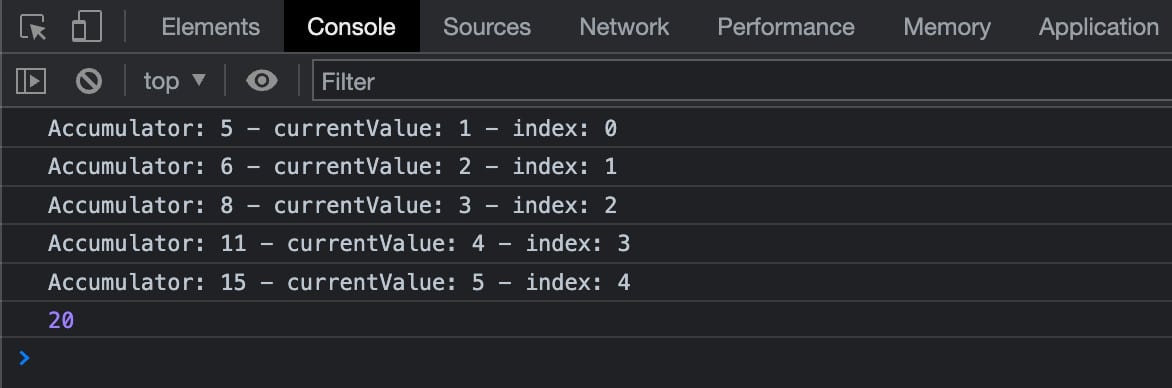
Now allow us to in finding out what occurs with out the initialValue
parameter:
const numbers = [1, 2, 3, 4, 5];
const sumElements = numbers.scale back(
( accumulator, currentValue, index ) => {
console.log( 'Accumulator: ' + accumulator + ' - currentValue: ' + currentValue + ' - index: ' + index );
go back accumulator + currentValue;
}
);
console.log( sumElements );
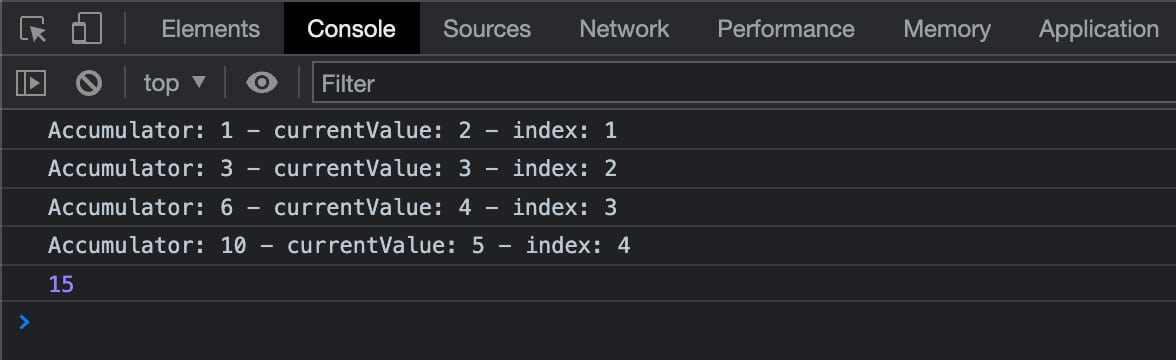
Extra examples and use circumstances are mentioned at the mdn internet medical doctors site.
Exports and Imports
As of ECMAScript 2015 (ES6), it's imaginable to export values from a JavaScript module and import them into some other script. You’ll be the usage of imports and exports widely to your React packages and subsequently it is very important have a excellent working out of ways they paintings.
The next code creates a purposeful element. The primary line imports the React library:
import React from 'react';
operate MyComponent() {
const individual = {
call: 'Carlo',
avatar: 'https://en.gravatar.com/userimage/954861/fc68a728946aac04f8531c3a8742ac22?measurement=authentic',
description: 'Content material Author',
theme: 'darkish'
}
go back (
{ individual.call }
{ individual.description }.
);
}
export default MyComponent;
We used the import
key phrase adopted by means of the call we need to assign to what we're uploading, adopted by means of the call of the bundle we need to set up as it's referred to within the bundle.json report.
Observe that within the MyComponent()
operate above, we used one of the JavaScript options mentioned within the earlier sections. We integrated assets values in curly brackets and assigned the price of the taste
assets the usage of the conditional operator syntax.
The script ends with the export of our customized element.
Now that we all know slightly extra about imports and exports, let’s take a better take a look at how they paintings.
Export
Each React module will have two various kinds of export: named export and default export.
As an example, you'll be able to export a number of options without delay with a unmarried export
observation:
export { MyComponent, MyVariable };
You'll additionally export particular person options (operate
, elegance
, const
, let
):
export operate MyComponent() { ... };
export let myVariable = x + y;
However you'll be able to solely have a unmarried default export:
export default MyComponent;
You'll additionally use default export for particular person options:
export default operate() { ... }
export default elegance { ... }
Import
As soon as the element has been exported, it may be imported into some other report, e.g. an index.js report, together with different modules:
import React from 'react';
import ReactDOM from 'react-dom/shopper';
import './index.css';
import MyComponent from './MyComponent';
const root = ReactDOM.createRoot( report.getElementById( 'root' ) );
root.render(
);
Within the code above, we used the import declaration in numerous tactics.
Within the first two traces, we assigned a reputation to the imported assets, within the 3rd line we didn't assign a reputation however merely imported the ./index.css report. The remaining import
observation imports the ./MyComponent report and assigns a reputation.
Let’s in finding out the variations between the ones imports.
In general, there are 4 kinds of imports:
Named import
import { MyFunction, MyVariable } from "./my-module";
Default import
import MyComponent from "./MyComponent";
Namespace import
import * as call from "my-module";
Aspect impact import
import "module-name";
After getting added a couple of types to your index.css, your card will have to seem like within the symbol beneath, the place you'll be able to additionally see the corresponding HTML code:
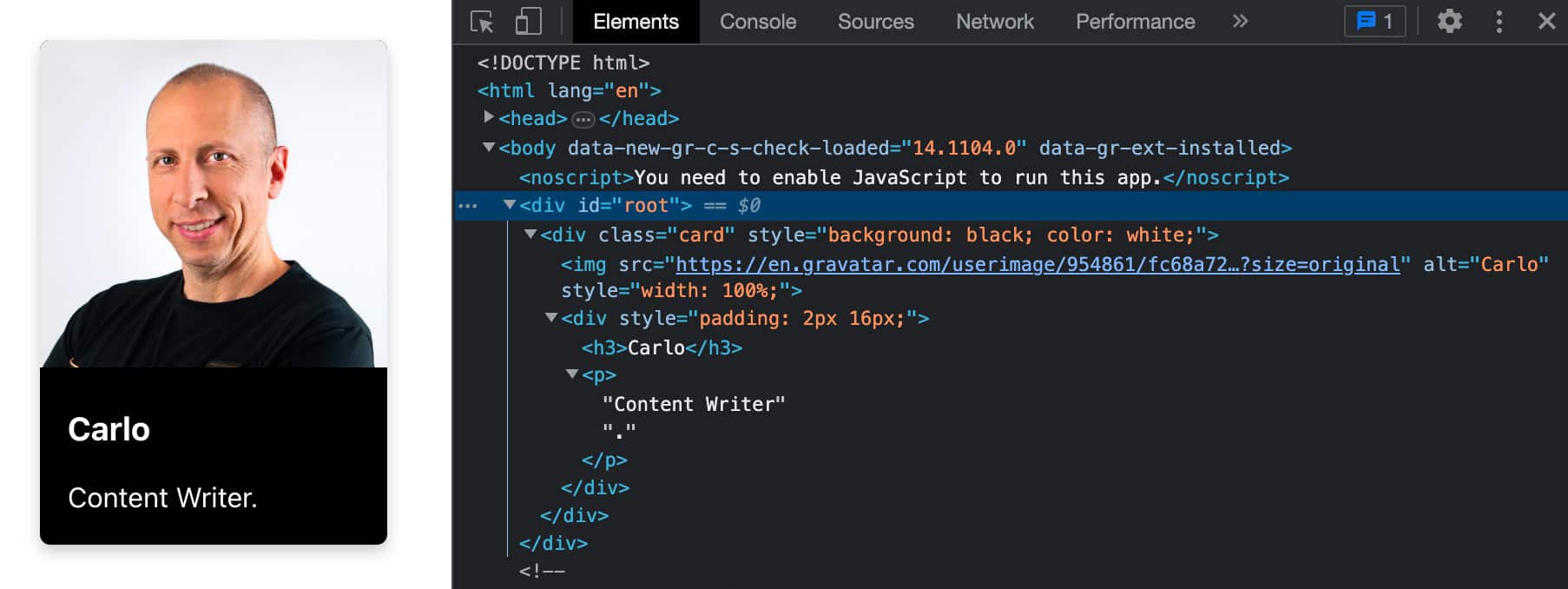
Observe that import
declarations can solely be utilized in modules on the most sensible degree (no longer inside of purposes, categories, and many others.).
For a extra complete evaluation of import
and export
statements, you might also need to test the next assets:
- export (mdn internet medical doctors)
- import (mdn internet medical doctors)
- Uploading and Exporting Elements (React dev)
- When will have to I take advantage of curly braces for ES6 import? (Stack Overflow)
Abstract
React is likely one of the maximum in style JavaScript libraries lately and is likely one of the maximum asked abilities on the planet of internet building.
With React, it's imaginable to create dynamic internet packages and complicated interfaces. Developing huge, dynamic, and interactive packages may also be simple because of its reusable parts.
However React is a JavaScript library, and a excellent working out of the primary options of JavaScript is very important to begin your adventure with React. That’s why we’ve accumulated in a single position one of the JavaScript options you’ll in finding maximum frequently utilized in React. Mastering the ones options offers you a leg up to your React studying adventure.
And in terms of internet building, making the transfer from JS/React to WordPress takes little or no effort.
Now it’s your flip, what JavaScript options do you suppose are most precious in React building? Have we ignored the rest essential that you'd have favored to look on our checklist? Proportion your ideas with us within the feedback beneath.
The put up JavaScript Options You Want to Know to Grasp React seemed first on Kinsta®.
WP Hosting