Extracting textual content from pictures has been a well-liked downside in device engineering for lengthy. Optical Personality Popularity (OCR) has been a pioneer generation used broadly to resolve this downside. With its talent to grow to be pictures containing textual content into machine-readable knowledge, OCR has revolutionized more than a few industries, from file processing automation to language translation.
Whilst business OCR answers exist, construction your personal OCR API in Python, a flexible and robust programming language, gives a number of benefits, together with customization, regulate over knowledge privateness, and the potential of value financial savings.
This information will stroll you thru growing your personal OCR API the use of Python. It explores the important libraries, tactics, and concerns for growing an efficient OCR API, empowering you to harness the facility of OCR to your packages.
Necessities
To apply alongside, you want a fundamental figuring out of Python & Flask and a neighborhood replica of Python put in in your machine.
Developing the OCR API
On this information, you discover ways to construct a Flask utility that permits customers to add pictures thru a POST endpoint, which then lots the use of Pillow, and processes the use of the PyTesseract wrapper (for the Tesseract OCR engine). In the end, it returns the extracted textual content because the reaction to the request.
You’ll be able to additional customise this API to supply choices comparable to template-based classification (extracting line pieces from invoices, inputs in tax paperwork, and so on.) or OCR engine alternatives (you’ll be able to in finding extra OCR engines right here).
To start out off, create a brand new listing to your mission. Then, arrange a brand new digital setting within the folder via operating the next instructions:
python3 -m venv env
supply env/bin/turn on
Subsequent, set up Flask, PyTesseract, Gunicorn, and Pillow via operating the next command:
pip3 set up pytesseract flask pillow gunicorn
As soon as those are put in, you want to put in the Tesseract OCR engine in your host mechanical device. The set up directions for Tesseract will range consistent with your host running machine. You’ll be able to in finding the proper directions right here.
As an example, on MacOS, you’ll be able to set up Tesseract the use of Homebrew via operating the next command:
brew set up tesseract
As soon as that is finished, the PyTesseract wrapper will have the ability to keep up a correspondence with the OCR engine and procedure OCR requests.
Now, you are prepared to jot down the Flask utility. Create a brand new listing named ocrapi and a brand new report on this listing with the title major.py. Save the next contents in it:
from flask import Flask, request, jsonify
from PIL import Symbol
import pytesseract
app = Flask(__name__)
@app.direction('/ocr', strategies=['POST'])
def ocr_process():
if request.way == 'POST':
image_file = request.information['image']
image_data = Symbol.open(image_file)
# Carry out OCR the use of PyTesseract
textual content = pytesseract.image_to_string(image_data)
reaction = {
'standing': 'good fortune',
'textual content': textual content
}
go back jsonify(reaction)
The code above creates a fundamental Flask app that has one endpoint—/ocr
. Whilst you ship a POST request to this endpoint with a picture report, it extracts the report, makes use of the pytesseract
wrapper to accomplish OCR the use of its code_to_string()
way, and sends again the extracted textual content as a part of the reaction.
Create a wsgi.py report in the similar ocrapi listing and save the next contents in it:
from ocrapi.major import app as utility
if __name__ == "__main__":
utility.run()
You’ll be able to now run the app the use of the next command:
gunicorn ocrapi.wsgi
Your fundamental OCR API is in a position, and it’s time to check it!
Trying out the OCR API In the community
You’ll be able to use the integrated cURL CLI to ship requests on your API or transfer to an in depth API trying out instrument comparable to Postman. To check the API, it is important to obtain a pattern picture that has some textual content. You’ll be able to use this straightforward one, or this scribbled one for now.
Obtain both of those to the mission listing and provides it a uncomplicated title, comparable to simple-image.png or scribbled-image.png, relying at the picture you select.
Subsequent, open your terminal and navigate on your mission’s listing. Run the next command to check the API:
curl -X POST -F “[email protected]” localhost:5000/ocr
This sends a request on your OCR API and returns a equivalent reaction:
{
"standing": "good fortune",
"textual content": "This appears love it was once written in a hucrynn"
}
This confirms that your OCR API has been arrange appropriately. You’ll be able to additionally take a look at with the straightforward picture, and right here’s what the reaction must seem like:
{
"standing": "good fortune",
"textual content": "This appears love it was once written with a gentle handnn"
}
This additionally demonstrates the accuracy of the Tesseract OCR engine. You’ll be able to now continue to host your OCR API at the Kinsta Utility Webhosting so it may be accessed on-line.
Deploying Your OCR API
To deploy your app to Kinsta, you first wish to push your mission code to a Git supplier (Bitbucket, GitHub, or GitLab).
Earlier than you push your code, you want to arrange Tesseract one by one in your host machine so that you can use the PyTesseract wrapper with it. So that you can use the wrapper at the Kinsta utility platform (or another setting, typically), it is important to set it up there as neatly.
For those who had been running with far off compute cases (comparable to AWS EC2), it’s good to SSH into the compute example and run the proper command for putting in the bundle on it.
Then again, utility platforms don’t give you direct get right of entry to to the host. It is important to use an answer like Nixpacks, Buildpacks, or Dockerfiles to arrange the preliminary necessities of your utility’s environments (which is able to come with putting in place the Tesseract bundle in the neighborhood) after which set up the applying.
Upload a nixpacks.toml report on your mission’s listing with the next contents:
# nixpacks.toml
suppliers = ["python"]
[phases.setup]
nixPkgs = ["...", "tesseract"]
[phases.build]
cmds = ["echo building!", "pip install -r requirements.txt", "..."]
[start]
cmd = "gunicorn ocrapi.wsgi"
This may increasingly instruct the construct platform to
- Use the Python runtime to construct and run your utility
- Arrange the Tesseract bundle on your utility’s container.
- Get started the app the use of
gunicorn
.
Additionally, run the next command to generate a necessities.txt report that the applying platform can use to put in the requirement Python applications all the way through construct:
pip3 freeze > necessities.txt
As soon as your Git repository is in a position, apply those steps to deploy your OCR API to Kinsta:
- Log in to or create an account to view your MyKinsta dashboard.
- Authorize Kinsta together with your Git supplier.
- At the left sidebar, click on Programs after which click on Upload Utility.
- Choose the repository and the department you want to deploy from.
- Choose one of the most to be had knowledge heart places from the listing of 35 choices. Kinsta routinely detects the construct settings to your packages thru your Nixpack report — so depart the beginning command box clean.
- Make a choice your utility assets, comparable to RAM and disk house.
- Click on Create utility.
As soon as the deployment is entire, replica the deployed app’s hyperlink and run the next command in your CLI:
curl -x POST -F “[email protected]” /ocr
This must go back the similar reaction as you won in the neighborhood:
{"standing":"good fortune","textual content":"This appears love it was once written with a gentle handnn"}
You’ll be able to additionally use Postman to check the API.
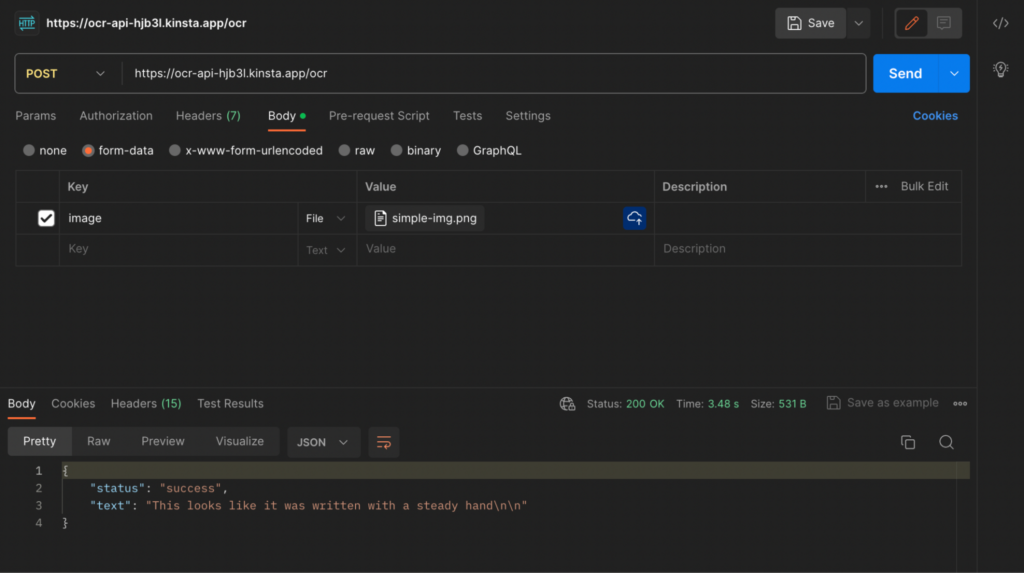
This completes the advance of a fundamental OCR API. You’ll be able to get right of entry to the entire code for this mission on GitHub.
Abstract
You presently have a running self-hosted OCR API that you’ll be able to customise on your liking! This API can extract textual content from pictures, offering a treasured instrument for knowledge extraction, file digitization, and different packages.
As you still broaden and refine your OCR API, imagine exploring complex options like multi-language beef up, picture pre-processing tactics, and integrating with cloud garage products and services for storing and gaining access to pictures.
What function do you assume is indispensable for a self-hosted OCR API? Tell us within the feedback underneath!
The submit How To Construct Your Personal OCR API in Python seemed first on Kinsta®.
WP Hosting