Feedback are notes that programmers advert to their code to give an explanation for what that code is meant to do. The compilers or interpreters that flip code into motion forget about feedback, however they may be able to be very important to managing device tasks.
Feedback lend a hand to give an explanation for your Python code to different programmers and will remind you of why you made the decisions you probably did. Feedback make debugging and revising code more straightforward by way of serving to long run programmers perceive the design alternatives at the back of device.
Even though feedback are basically for builders, writing efficient ones too can support in generating superb documentation in your code’s customers. With the assistance of record turbines like Sphinx for Python tasks, feedback on your code may give content material in your documentation.
Let’s glance below the hood of commenting in Python.
Feedback in Python
In step with the Python PEP 8 Taste Information, there are a number of issues to bear in mind whilst writing feedback:
- Feedback must all the time be whole and concise sentences.
- It’s higher to haven’t any remark in any respect than person who’s obscure or faulty.
- Feedback must be up to date incessantly to replicate adjustments on your code.
- Too many feedback will also be distracting and cut back code high quality. Feedback aren’t wanted the place the code’s objective is apparent.
In Python, a line is asserted as a remark when it starts with #
image. When the Python interpreter encounters #
on your code, it ignores the rest after that image and does now not produce any error. There are two techniques to claim single-line feedback: inline feedback and block feedback.
Inline Feedback
Inline feedback supply brief descriptions of variables and easy operations and are written at the similar line because the code observation:
border = x + 10 # Make offset of 10px
The remark explains the serve as of the code in the similar observation because the code.
Block Feedback
Block feedback are used to explain advanced common sense within the code. Block feedback in Python are built in a similar fashion to inline feedback — the one distinction is that block feedback are written on a separate line:
import csv
from itertools import groupby
# Get a listing of names in a chain from the csv report
with open('new-top-firstNames.csv') as f:
file_csv = csv.reader(f)
# Skip the header section: (sr, identify, perc)
header = subsequent(file_csv)
# Handiest identify from (quantity, identify, perc)
individuals = [ x[1] for x in file_csv]
# Type the listing by way of first letter as a result of
# The groupby serve as appears for sequential information.
individuals.type(key=lambda x:x[0])
information = groupby(individuals, key=lambda x:x[0])
# Get each and every identify as a listing
data_grouped = {}
for okay, v in information:
# Get information within the shape
# {'A' : ["Anthony", "Alex"], "B" : ["Benjamin"]}
data_grouped[k] = listing(v)
Be aware that once the usage of block feedback, the feedback are written above the code that they’re explaining. The Python PEP8 Taste Information dictates {that a} line of code must now not include greater than seventy-nine characters, and inline feedback regularly push traces over this period. For this reason block feedback are written to explain the code on separate traces.
Multi-Line Feedback
Python does now not natively give a boost to multi-line feedback, this means that there’s no particular provision for outlining them. In spite of this, feedback spanning more than one traces are regularly used.
You’ll create a multi-line remark out of a number of single-line feedback by way of prefacing every line with #
:
# interpreter
# ignores
# those traces
You’ll additionally use multi-line strings syntax. In Python, you’ll outline multi-line strings by way of enclosing them in """
, triple double quotes, or '''
, triple unmarried quotes:
print("Multi-Line Remark")
"""
This
String is
Multi line
"""
Within the code above, the multi-line string isn’t assigned to a variable, which makes the string paintings like a remark. At runtime, Python ignores the string, and it doesn’t get incorporated within the bytecode. Executing the above code produces the next output:
Multi-Line Remark
Particular Feedback
Along with making your code readable, feedback additionally serve some particular functions in Python, comparable to making plans long run code additions and producing documentation.
Python Docstring Feedback
In Python, docstrings are multi-line feedback that give an explanation for the best way to use a given serve as or magnificence. The documentation of your code is progressed by way of the introduction of high quality docstrings. Whilst operating with a serve as or magnificence and the usage of the integrated lend a hand(obj)
serve as, docstrings may well be useful in giving an summary of the item.
Python PEP 257 supplies an ordinary way of mentioning docstrings in Python, proven underneath:
from collections import namedtuple
Particular person = namedtuple('Particular person', ['name', 'age'])
def get_person(identify, age, d=False):
"""
Returns a namedtuple("identify", "age") object.
Additionally returns dict('identify', 'age') if arg `d` is True
Arguments:
identify – first identify, will have to be string
age – age of particular person, will have to be int
d – to go back Particular person as `dict` (default=False)
"""
p = Particular person(identify, age)
if d:
go back p._asdict()
go back p
Within the code above, the docstring supplied main points on how the related serve as works. With documentation turbines like Sphinx, this docstring can be utilized to provide customers of your venture an summary of the best way to use this system.
A docstring outlined slightly below the serve as or magnificence signature may also be returned by way of the usage of the integrated lend a hand()
serve as. The lend a hand()
serve as takes an object or serve as identify as a controversy, and returns the serve as’s docstrings as output. Within the instance above, lend a hand(get_person)
will also be referred to as to expose docstrings related to the get_person
serve as. In case you run the code above in an interactive shell the usage of the -i
flag, you’ll see how this docstring shall be parsed by way of Python. Run the above code by way of typing python -i report.py
.
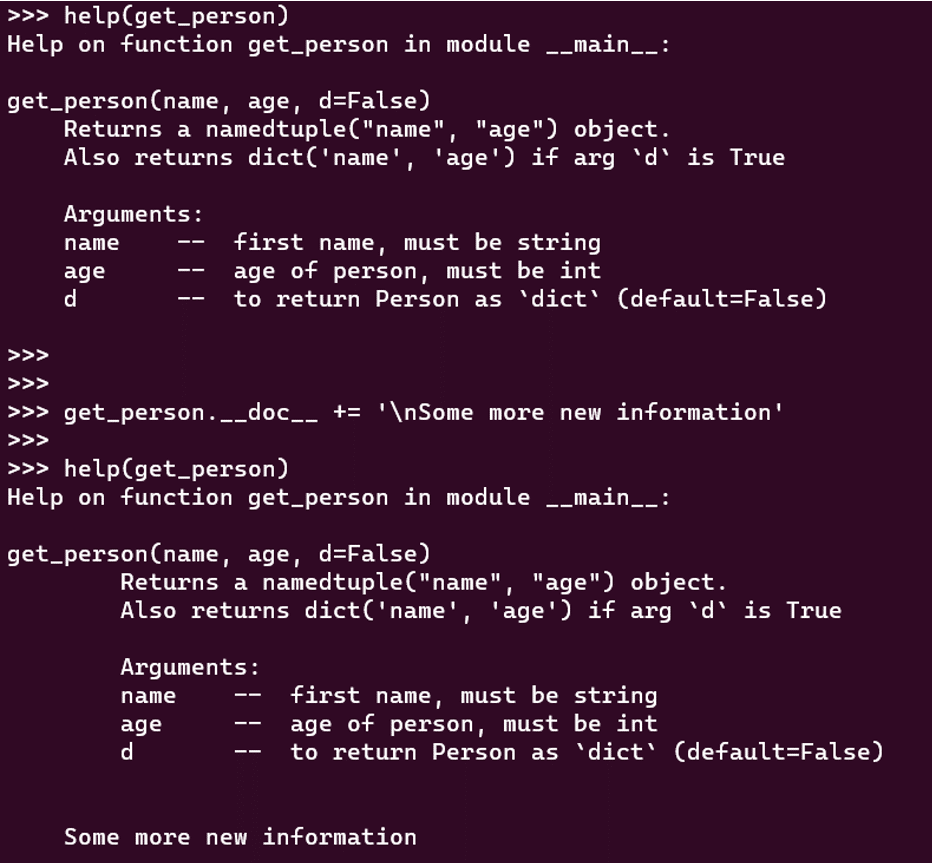
The lend a hand(get_person)
serve as name returns a docstring in your serve as. The output comprises get_person(identify, age, d=False)
, which is a serve as signature that Python provides routinely.
The get_person.__ doc__
characteristic may also be used to retrieve and alter docstrings programmatically. After including “Some extra new data” within the instance above, it seems that in the second one name to lend a hand(get_person)
. Nonetheless, it’s not likely that you’re going to wish to dynamically adjust docstrings at runtime like this.
TODO Feedback
When writing code, there are times whilst you’ll wish to spotlight sure traces or complete blocks for growth. Those duties are flagged by way of TODO feedback. TODO feedback turn out to be useful whilst you’re making plans updates or adjustments on your code, or if you want to tell the venture’s customers or collaborators that individual sections of the report’s code stay to be written.
TODO feedback must now not be written as pseudocode — they simply need to in brief give an explanation for the serve as of the yet-unwritten code.
TODO feedback and single-line block feedback are very equivalent, and the only real difference between them is that TODO feedback will have to start with a TODO prefix:
# TODO Get serialized information from the CSV report
# TODO Carry out calculations at the information
# TODO Go back to the person
It’s necessary to notice that even though many IDEs can spotlight those feedback for the programmer, the Python interpreter does now not view TODO feedback any otherwise from block feedback.
Best possible Practices When Writing Python Feedback
There are a selection of highest practices that are meant to be adopted when writing feedback to verify reliability and high quality. Under are some pointers for writing high quality feedback in Python.
Keep away from the Evident
Feedback that state the most obvious don’t upload any price on your code, and must be have shyed away from. For instance:
x = x + 4 # building up x by way of 4
That remark isn’t helpful, because it merely states what the code does with out explaining why it must be completed. Feedback ought to give an explanation for the “why” quite than the “what” of the code they’re describing.
Rewritten extra usefully, the instance above may appear to be this:
x = x + 4 # building up the border width
Stay Python Feedback Brief and Candy
Stay your feedback brief and simply understood. They must be written in usual prose, now not pseudocode, and must change the wish to learn the true code to get a basic evaluate of what it does. An excessive amount of element or advanced feedback don’t make a programmer’s activity any more straightforward. For instance:
# go back house by way of acting, Space of cylinder = (2*PI*r*h) + (2*PI*r*r)
def get_area(r,h):
go back (2*3.14*r*h) + (2*3.14*r*r)
The remark above supplies additional info than is important for the reader. As an alternative of specifying the core common sense, feedback must supply a basic abstract of the code. This remark will also be rewritten as:
# go back house of cylinder
def get_area(r,h):
go back (2*3.14*r*h) + (2*3.14*r*r)
Use Identifiers Sparsely
Identifiers must be used sparsely in feedback. Converting identifier names or circumstances can confuse the reader. Instance:
# go back magnificence() after editing argument
def func(cls, arg):
go back cls(arg+5)
The above remark mentions magnificence
and argument
, neither of that are discovered within the code. This remark will also be rewritten as:
# go back cls() after editing arg
def func(cls, arg):
go back cls(arg+5)
DRY and WET
Whilst you’re writing code, you wish to have to stick to the DRY (don’t repeat your self) idea and keep away from WET (write the entirety two times).
This may be true for feedback. Keep away from the usage of more than one statements to explain your code, and take a look at to merge feedback that give an explanation for the similar code right into a unmarried remark. Then again, it’s necessary to watch out whilst you’re merging feedback: careless merging of more than one feedback can lead to an enormous remark that violates taste guides and is tricky for the reader to apply.
Take into account that feedback must cut back the studying time of the code.
# serve as to do x paintings
def do_something(y):
# x paintings can't be completed if y is bigger than max_limit
if y < 400:
print('doing x paintings')
Within the code above, the feedback are unnecessarily fragmented, and will also be merged right into a unmarried remark:
# serve as to do x if arg:y is lower than max_limit
def do_something(y):
if y in vary(400):
print('doing x paintings')
Constant Indentation
Make sure that feedback are indented on the similar degree because the code they’re describing. Once they’re now not, they may be able to be tough to apply.
For instance, this remark isn't indented or situated correctly:
for i in vary(2,20, 2):
# simplest even numbers
if test(i):
# i must be verified by way of test()
carry out(x)
It may be rewritten as follows:
# simplest even numbers
for i in vary(2,20, 2):
# i must be verified by way of test()
if test(i):
carry out(x)
Abstract
Feedback are a very powerful part of writing comprehensible code. The funding you are making in writing a remark is person who your long run self — or different builders who wish to paintings in your code base — will recognize. Commenting additionally means that you can achieve deeper insights into your code.
On this instructional, you’ve discovered extra about feedback in Python, together with the quite a lot of sorts of Python feedback, when to make use of every of them, and the most productive practices to apply when growing them.
Writing just right feedback is a ability that must be studied and advanced. To observe writing feedback, believe going again and including feedback to a couple of your earlier tasks. For inspiration and to peer highest practices in motion, take a look at well-documented Python tasks on GitHub.
Whilst you’re able to make your personal Python tasks reside, Kinsta’s Software Internet hosting platform can get your code from GitHub to the cloud in seconds.
The put up Create Python Feedback the Proper Approach seemed first on Kinsta®.
WP Hosting