In our earlier article, we lined the right way to create easy pages in Flask and use Jinja2 because the templating engine. Now, let’s discover how Flask handles requests.
Working out how HTTP requests paintings and the right way to organize them in Flask is essential, as this permits you to construct extra interactive and dynamic internet apps, corresponding to construction a kind, API endpoints, and dealing with document uploads.
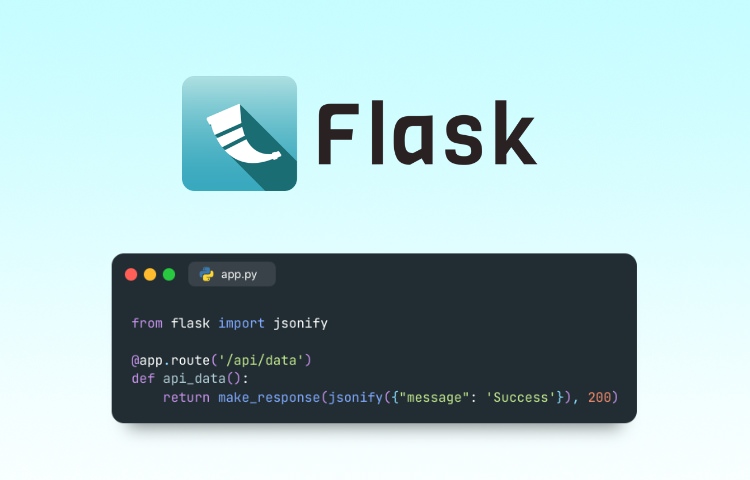
With out additional ado, let’s get began.
So, What’s an HTTP Request?
An HTTP request is a message despatched, most often via a browser, to the server requesting knowledge or to accomplish an motion. As an example, whilst you consult with a webpage, your browser sends a GET request to the server to retrieve the web page’s content material.
There are a number of several types of HTTP requests, and Flask can take care of they all, together with GET
to retrieve knowledge, POST
to ship knowledge to the server like filing a kind, PUT
to replace current knowledge at the server, and DELETE
to delete knowledge from the server.
Dealing with Requests in Flask
Flask makes dealing with requests simple via the usage of routes. In our earlier articles, we used routes to create static and dynamic pages. By means of default, routes simplest reply to GET
requests, however you’ll simply take care of different HTTP strategies via specifying them within the direction.
Assuming we now have a touch web page at /touch
, we most definitely would wish the web page to take care of each GET
and POST
requests to permit customers to load the web page, in addition to to post the shape. To make the web page take care of those two HTTP strategies, we will be able to go within the strategies
argument, as an example:
@app.direction('/touch', strategies=['GET', 'POST']) def post(): if request.manner == 'POST': knowledge = request.shape['input_data'] go back render_template('touch.html', knowledge=knowledge) go back render_template('touch.html')
On this instance, customers can load the /touch
web page. When the shape is submitted, Flask retrieves the shape knowledge and passes it to the touch.html
template. Then, throughout the template, you’ll get right of entry to and procedure the knowledge the usage of Jinja2 templating.
Operating with Question Parameters
Information is also handed to a URL by means of question parameters. That is regularly discovered on a seek web page the place the hunt question is handed as a question parameter. Those are the portions of the URL after a ?
, like /seek?question=flask
. Flask makes it simple to get right of entry to question parameters with the request.args
dictionary, as an example:
@app.direction('/seek') def seek(): question = request.args.get('question') # Meilisearch # See: https://github.com/meilisearch/meilisearch-python consequence = index.seek(question) if question: go back render_template('seek.html', consequence=consequence) go back 'No seek question supplied.'
On this case, when a person visits /seek?question=flask
, we take the question and use it to retrieve the hunt consequence, which is then handed to the seek.html
template for rendering.
Dealing with JSON Information
When construction an API, we’d like the knowledge delivered in JSON layout. Flask supplies a easy technique to take care of JSON knowledge in requests with the jsonify
serve as. Right here’s an instance of dealing with JSON knowledge:
from flask import jsonify @app.direction('/api/knowledge') def api_data(): go back make_response(jsonify({"message": 'Good fortune'}), 200)
Dealing with Report Uploads
Flask additionally makes dealing with document uploads simple, the usage of the request.information
object.
@app.direction('/add', strategies=['GET', 'POST']) def upload_file(): if request.manner == 'POST': document = request.information['file'] document.save(f'/uploads/{document.filename}') go back redirect(url_for('index.html'))
On this instance, when a person submits a document by means of the shape, Flask saves the document to the desired listing after which redirects the person to the homepage.
Request Headers and Cookies
Every so often you additionally wish to get headers or cookies from the request on your app, corresponding to for passing authentication or monitoring person knowledge. Flask supplies simple get right of entry to to headers thru request.headers
and cookies thru request.cookies
. Right here’s a fundamental instance of ways we use it to authenticate for an API endpoint:
@app.direction('/api/knowledge') def take a look at(): auth = request.headers.get('Authorization') nonce = request.cookies.get('nonce') # Easy authentication take a look at if auth == 'Bearer X' and nonce == 'Y': go back jsonify({"message": "Authenticated"}), 200 else: go back jsonify({"message": "Unauthorized"}), 401
Wrapping up
Flask makes dealing with HTTP requests a breeze. Whether or not you’re operating with fundamental GET
requests, dealing with shape submissions with POST
, or coping with extra advanced situations like JSON knowledge and document uploads, it supplies the APIs, purposes, and gear you wish to have to get the process accomplished. We’ve simplest scratched the outside of Flask’s request-handling features, however with a bit of luck, this offers you a cast basis to start out construction your individual Flask apps.
The put up How one can Care for HTTP Requests in Flask gave the impression first on Hongkiat.
WordPress Website Development Source: https://www.hongkiat.com/blog/handle-http-requests-flask/