With code reuse comes the issue of dependency control, traditionally a relatively handbook and error-prone procedure. Thankfully, contemporary years have observed maximum programming languages resolve this drawback by way of introducing more than a few equipment for dependency control. Within the PHP ecosystem, Composer turn out to be the usual due to its ease of use, flexibility, and large adoption.
Even if Composer used to be constructed for dependency control, you’ll additionally use it for duties like:
- Autoloading: Mechanically load categories and purposes from exterior libraries to lead them to readily out there out of your code and simplify the method of integrating exterior code into your undertaking.
- Code era: Generate boilerplate code on your undertaking, akin to configuration recordsdata or different usually used code snippets to hurry up the advance procedure and make sure consistency.
- Scripting: A integrated scripting gadget can automate not unusual duties, akin to working exams or producing documentation out of your code base. It will assist streamline your construction workflow and cut back handbook paintings.
This step by step instructional guides you via developing your individual Composer bundle and publishing it to Packagist, a repository for PHP programs that builders around the globe can use in their very own tasks.
How does Composer paintings?
First, let’s ensure that we perceive Composer. In easy phrases, Composer works by way of the use of a composer.json document that incorporates the definitions for the dependencies to your PHP undertaking. It appears to be like up the ones programs from a centralized repository after which routinely downloads and installs the ones dependencies the use of the bundle repo.
Assuming you have already got Composer put in to your PHP operating atmosphere, right here’s what its dependency obtain and set up procedure looks as if:
- Outline the desired dependencies on your undertaking in a composer.json document to your undertaking’s root listing. This document contains details about required libraries and their variations and another configuration settings or dependencies for the ones libraries.
- Get to the bottom of dependencies in Composer with instructions like
set up
to put in the desired dependencies;replace
to replace present dependencies; andrequire
so as to add new dependencies to the composer.json document. While you run a command, Composer reads the composer.json document to resolve required dependencies, assessments the bundle repo for the newest model of every dependency suitable on your PHP atmosphere, after which assessments for any conflicts or model constraints. - Composer downloads and installs required dependencies, together with libraries, into the dealer listing of your undertaking. Composer creates a composer.lock document recording the precise variations of the dependencies put in.
- Composer units up an autoloader that quite a bit categories and purposes from the put in dependencies routinely. This makes it simple to make use of the put in libraries to your undertaking with no need to manually come with every document.
Lengthy tale brief, Composer simplifies managing dependencies in PHP tasks, making it simple to put in, replace, and use exterior libraries and frameworks.
Growing and publishing a Composer bundle
This instructional presentations you tips on how to create a easy PHP library known as tempconv that converts Celsius temperature to Fahrenheit and vice versa and wraps up by way of publishing it as Composer bundle.
Must haves
You’ll want a few issues in position sooner than you start:
- PHP and Composer put in correctly to your gadget On the time of writing, the newest Composer model is v2.6.6, however those directions will have to paintings with any v2 variant.
- A GitHub account to create a repository for website hosting your code.
- A Packagist account to submit your library.
Create a undertaking repository
Create your individual GitHub repository for this instructional, the place you’ll push your completed library code and recordsdata.
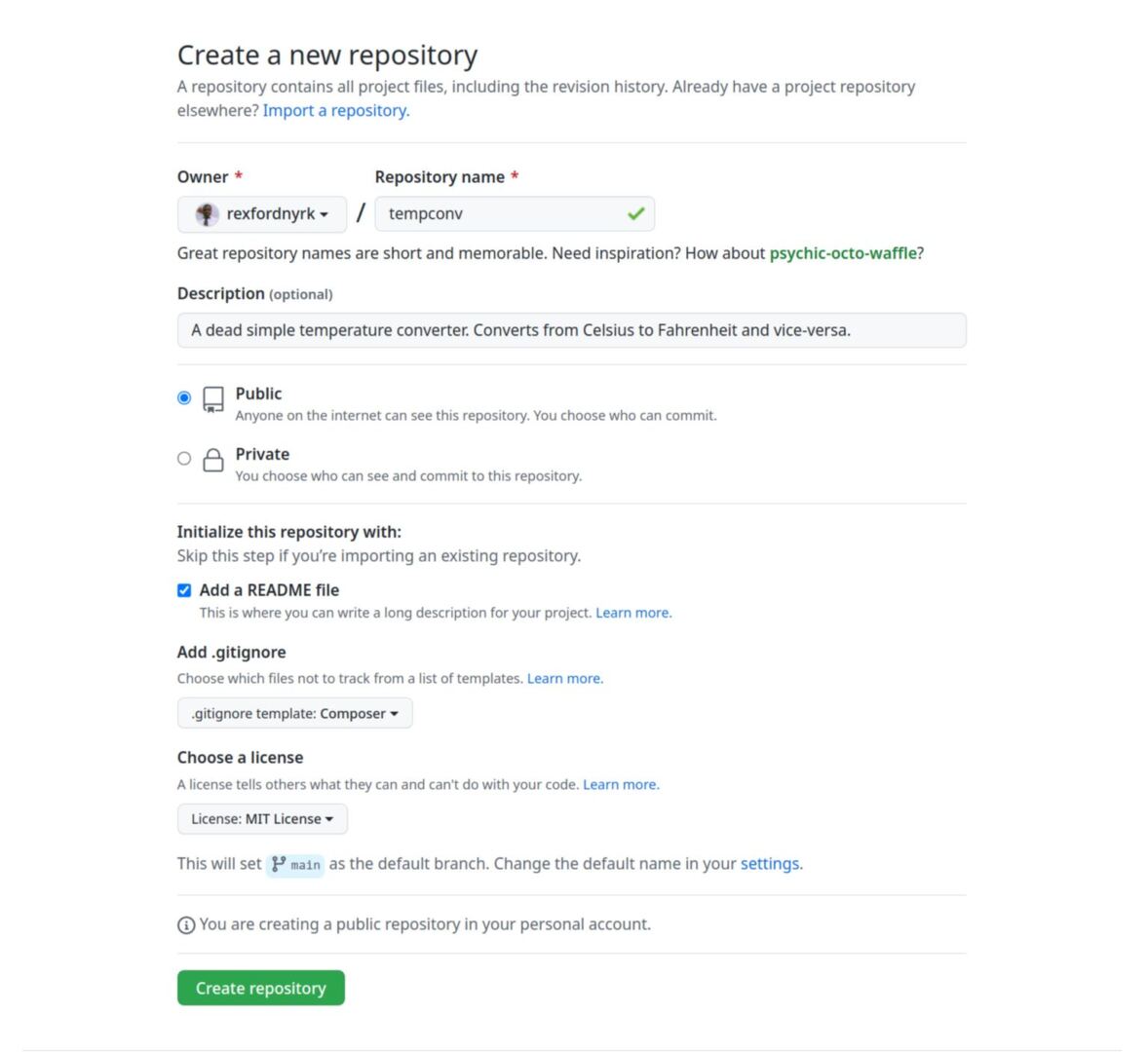
This instructional makes use of the undertaking title tempconv. Within the Description box, supply temporary information about the applying. Take a look at the field so as to add a README document, make a choice the Composer template for the Upload .gitignore choice, and make a choice a license of your selection—this instructional makes use of MIT License. After all, click on Create repository.
Clone the repository
Clone the repository you simply created for your native system. Don’t omit to exchange the URL with your individual, each right here and during this instructional:
$ git clone https://github.com/rexfordnyrk/tempconv.git
This creates a listing known as tempconv to your present operating listing. At this level, it most effective incorporates README.md, LICENSE, and .gitignore recordsdata, however you’ll create your bundle recordsdata right here as smartly.
Growing your PHP library
For your undertaking listing, upload a document named TemperatureConverter.php with the next code:
This magnificence has a unmarried manner known as convert
that takes the temperature and the unit as arguments and returns the transformed temperature. It throws an exception if the unit is invalid.
That’s sufficient for now. In a real-world state of affairs, you could possibly most likely write unit exams to make sure your code works as anticipated after adjustments or updates.
Growing your composer bundle.
Along with your library code in position, it’s time to make it a Composer bundle. You’ll generate a composer.json document on your bundle the use of a step by step wizard, and we’ll quilt some perfect practices for organizing your code as a bundle sooner than pushing it for your repository.
Producing a composer.json bundle document
Whilst it's essential manually write the content material of a composer.json document within the root of your undertaking listing, it’s higher to generate it the use of the next Composer command:
$ composer init
This command runs you via a small step by step wizard. The solutions you supply to activates just like the bundle title, description, writer data, and license kind will generate your bundle’s composer.json document.
Composer’s documentation outlines the predicted values, in addition to different choices you'll use to outline your undertaking’s composer.json.
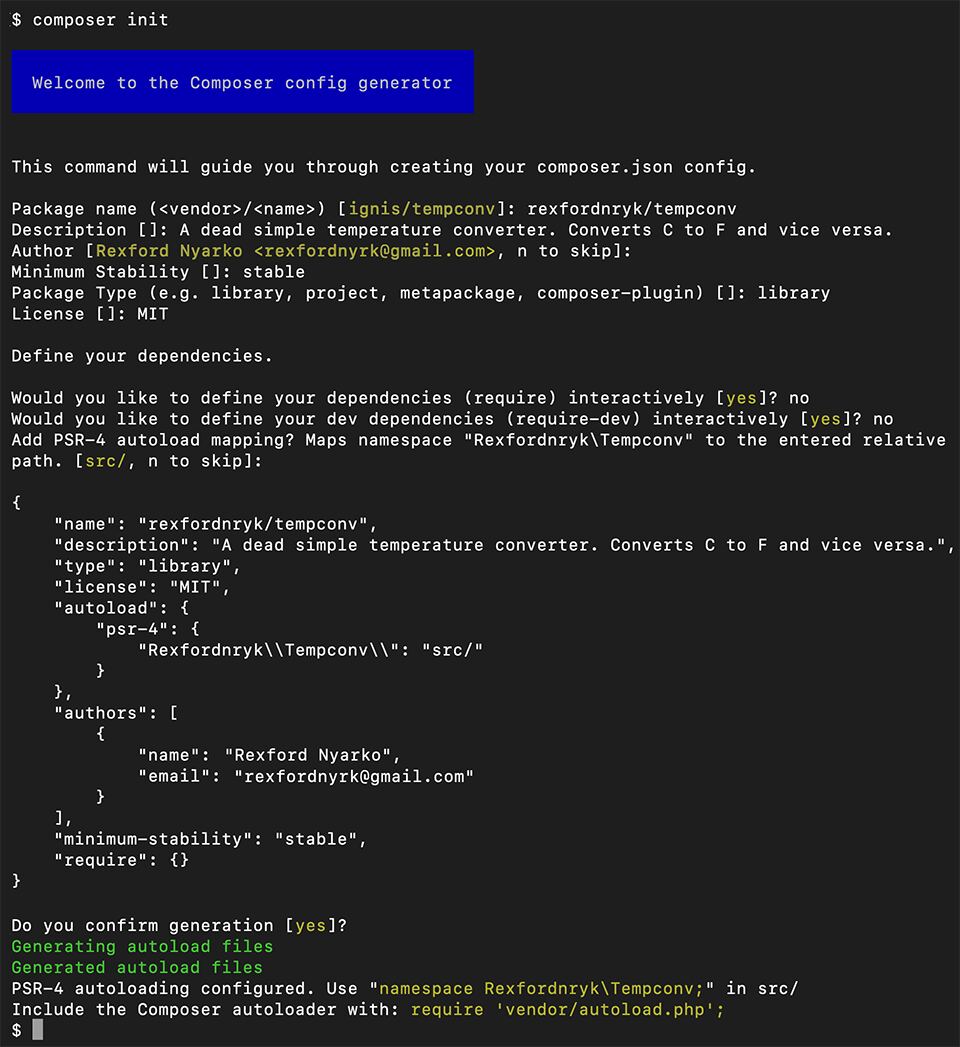
Realize that the wizard supplies predefined solutions to the questions the place acceptable, akin to deriving your title and e-mail from git. Be at liberty to modify them in case you like.
This library isn’t depending on another programs for capability, so you'll resolution No to questions specifying dependencies.
The wizard presentations you a preview of the generated document content material and asks you to verify it to finish the wizard.
Organizing bundle recordsdata
Finishing the wizard creates two directories along with the composer.json document:
- src on your supply code
- dealer on your downloaded dependencies
Transfer the TemperatureConverter.php document into the src listing. In case your library has dependencies, run composer set up
to generate the autoloader and set up the bundle dependencies.
Importing code to GitHub
Upload your adjustments and new recordsdata to git:
$ git upload -A
Devote adjustments made to the native repository and push it to the far flung repo on GitHub so you'll simply submit the undertaking within the subsequent segment:
$ git devote -am "Preliminary Free up" && git push
Create a unencumber model on your library
Along with your code to your repo, you'll create a unencumber of your library with a model quantity so different builders can stay observe of your solid and demanding updates.
Navigate for your repo on GitHub, and click on Releases underneath the About segment. At the Releases web page, the place you’re anticipated to don't have anything at this time, click on Create a brand new unencumber.
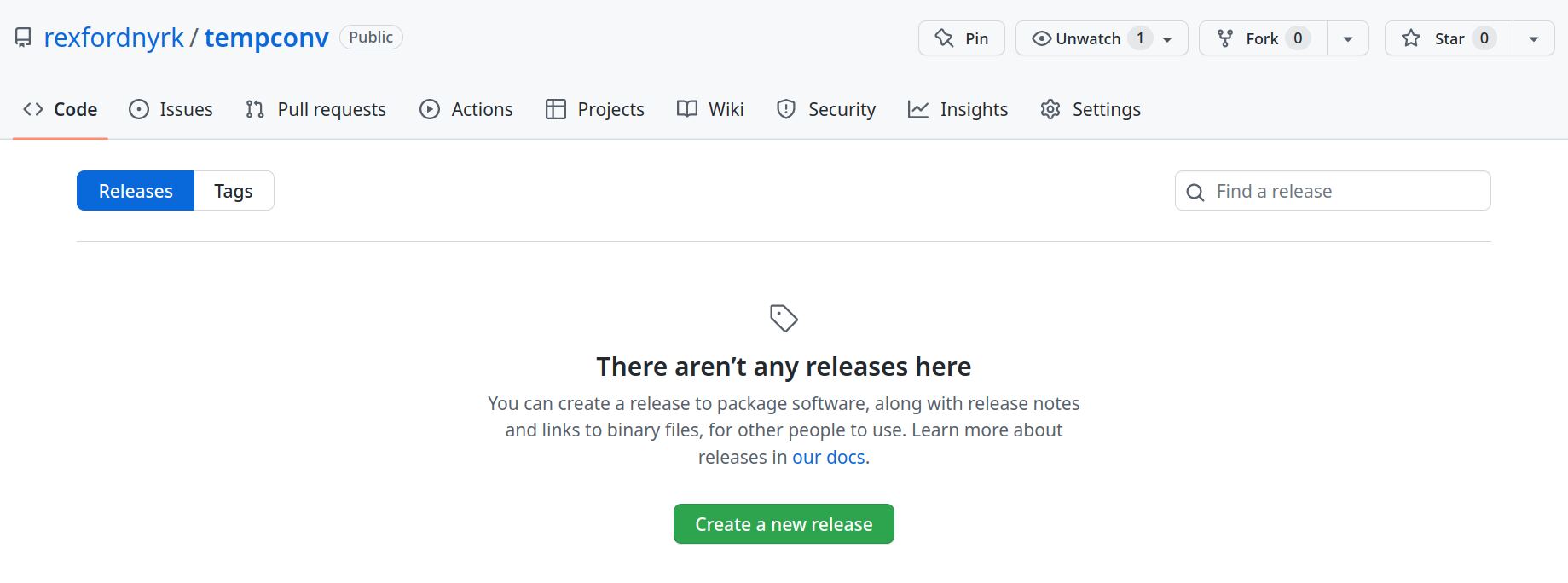
Fill out a couple of information about your unencumber, just like the tag model and unencumber name. The tag model will have to be a novel identifier for this unencumber (instance: v1.0.0), and the discharge name will have to describe the adjustments integrated within the unencumber (instance: Preliminary unencumber).
Optionally, you'll upload an outline of the discharge. If you wish to add a document, akin to a compiled binary or a supply code archive, drag and drop the document into the Connect binaries by way of shedding them right here or settling on them house, however that’s now not wanted for this instructional.
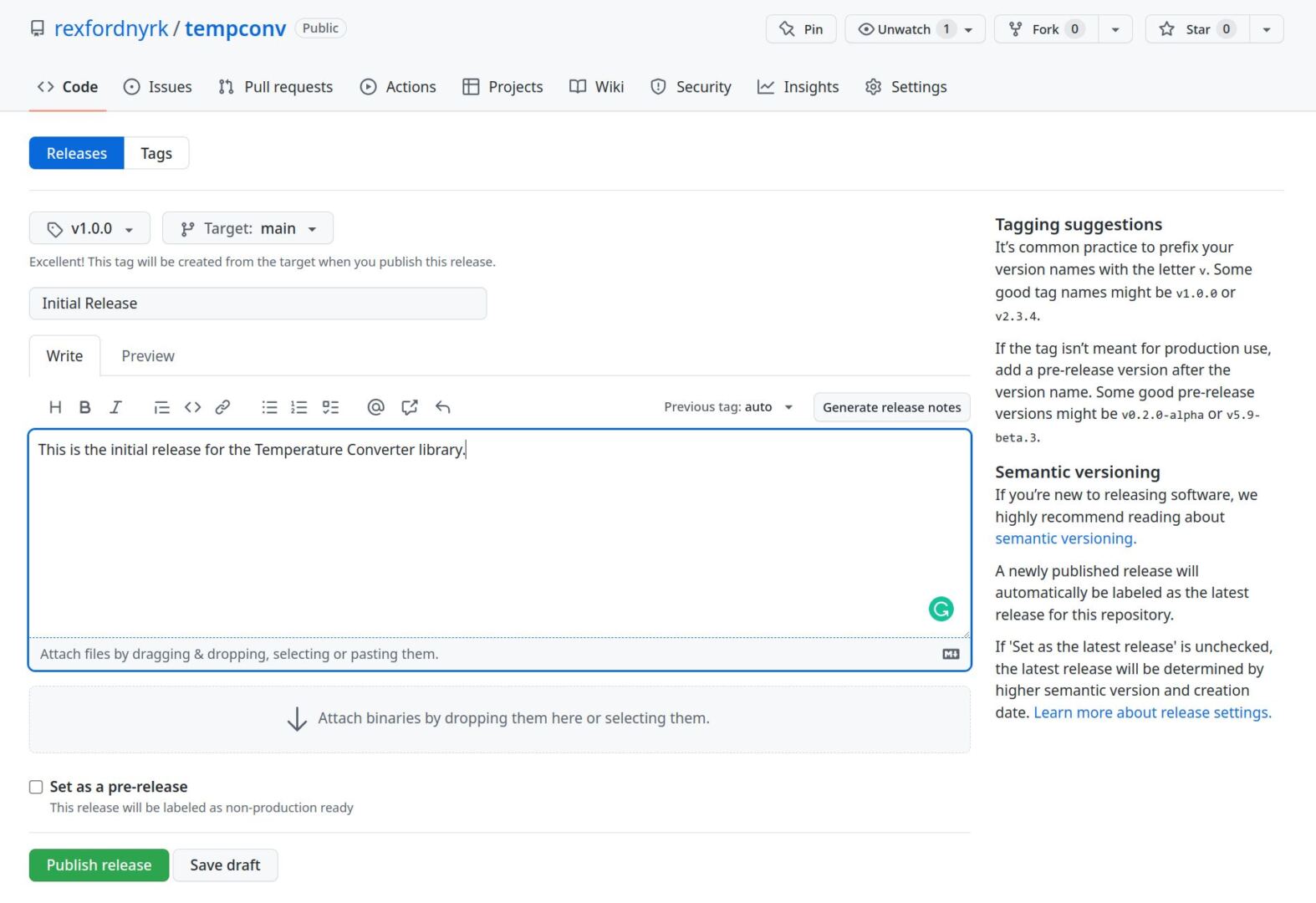
Click on Post unencumber to create the discharge.
Your undertaking will have to now be to be had at the Releases web page of your repository. Customers can obtain any recordsdata you hooked up and examine the discharge notes. Moreover, in case you added a tag for the discharge, different builders can use that tag to take a look at the precise code that used to be integrated within the unencumber.
Your library is able to proportion with the arena. Let’s submit it as a bundle on Packagist.
Getting began with Packagist
Packagist is the principle bundle repository for PHP. It supplies a central location for builders to submit and proportion their PHP programs, and for different builders to find and use the ones programs in their very own tasks. Let’s get your bundle printed!
Navigate to the Packagist site, click on Login, and make a choice GitHub because the login manner to make use of your GitHub account for authentication.
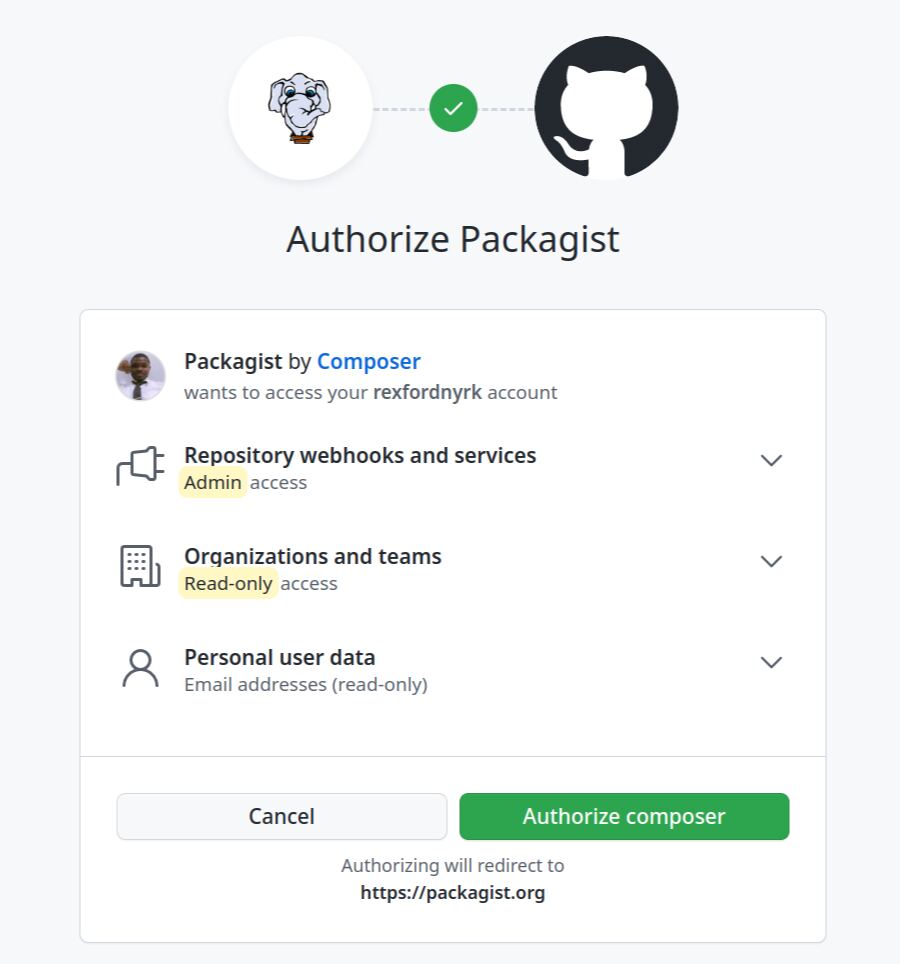
Click on Authorize to grant Packagist permission to get right of entry to your account.
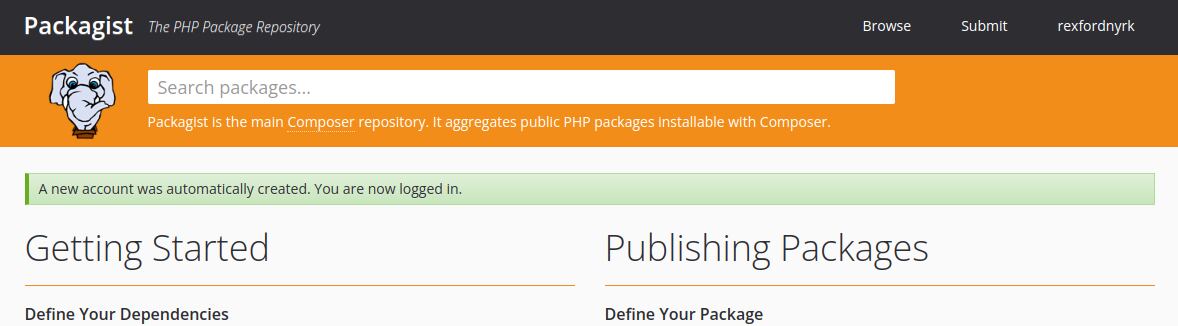
Filing your Composer bundle on Packagist
To submit a bundle on Packagist, publish the GitHub repository containing the composer.json document that describes your bundle and its dependencies. Click on Post on Packagist’s website online, supply your repo’s URL at the web page that looks, and click on Take a look at to validate it.
If the repository is legitimate, Packagist detects the title of the bundle, and the Take a look at button turns into Post.
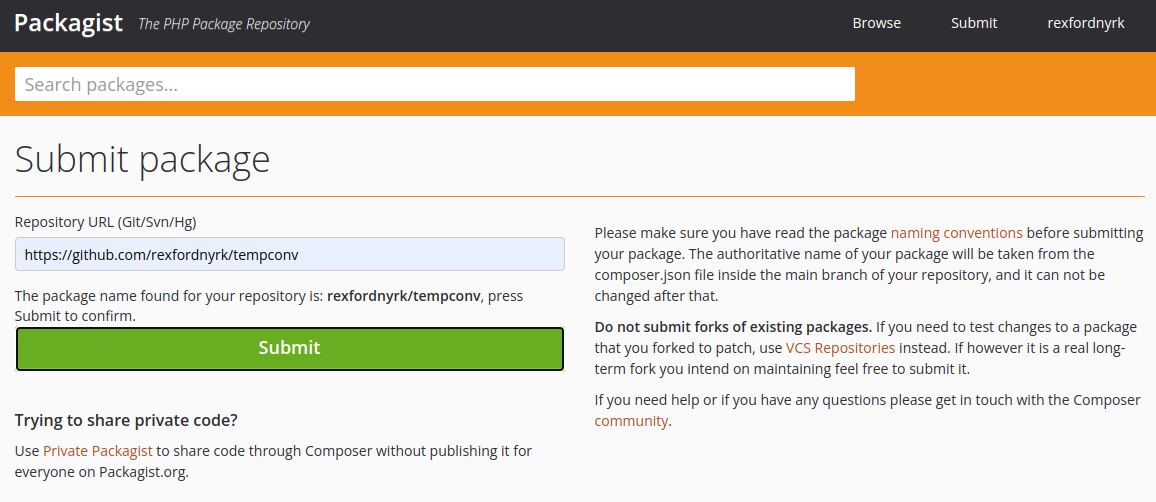
Click on Post, and Packagist takes a second to arrange and submit your undertaking.
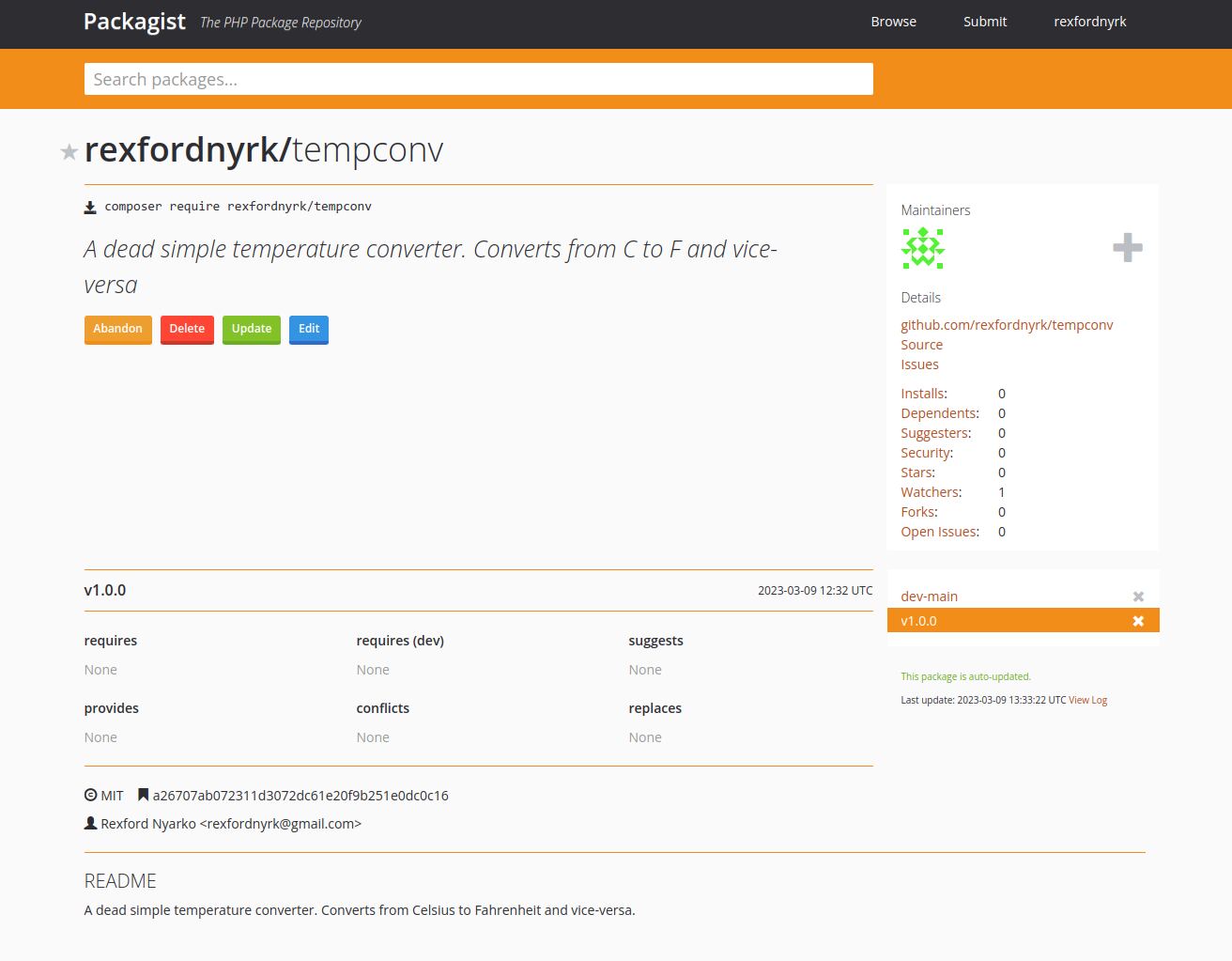
That’s it! Your bundle is now to be had on Packagist for different builders to make use of as a dependency in their very own tasks.
The usage of your Composer bundle in different tasks
You'll be able to use your printed bundle in different tasks by way of including it as a dependency within the composer.json document. You'll be able to manually create and edit the document, however it’s higher to make use of the Composer command as follows:
$ composer require rexfordnyrk/tempconv
Composer creates the composer.json document routinely if it doesn’t exist already, then it unearths, downloads, and autoloads the bundle from Packagist. The output will have to glance one thing like this:
Create a demo.php document to comprise your demo software code to paintings along with your library.
You'll be able to then use the TemperatureConverter
magnificence to your software code like this:
convert(20, 'C');
echo "20°C is identical to $fahrenheit °Fn";
$celsius = $converter->convert(68, 'F');
echo "68°F is identical to $celsius °Cn";
This code makes use of the TemperatureConverter
magnificence to transform temperatures 20 Celsius and 68 Fahrenheit and output the consequences. The imported autoloader.php document used to be created by way of Composer to load all of the dependencies you require. That looks after loading the category routinely when essential.
After all, run this code to your terminal:
$ php demo.php
You will have an output very similar to this:
$ php demo.php
20°C is identical to 68 °F
68°F is identical to twenty °C
Abstract
You’ve simply created a easy PHP library that converts temperature from Celsius to Fahrenheit and vice versa in an object-oriented magnificence that may be reused in different packages. You noticed tips on how to use Composer to create a bundle from the category with the Composer Init command, and also you discovered some fundamental code group perfect practices on your library. You printed your library on Packagist, so that you and different builders may use it as a dependency in different tasks.
After all, while you’re finished growing your software, you’ll wish to host it. Take your PHP construction to the following degree with Internet Utility Internet hosting at Kinsta. You'll be able to deploy your Composer-based PHP software in mins. Simply upload your software by the use of your undertaking’s git repository, and Kinsta handles the remaining. It routinely detects the Composer document and builds your software routinely. Get began risk-free as of late!
The submit The way to create your individual Composer bundle gave the impression first on Kinsta®.
WP Hosting