Whilst development some plugins, I figured developing dynamic programs in WordPress Admin is way more straightforward with React parts in comparison to the use of PHP and jQuery like again within the previous days. On the other hand, integrating React parts with WordPress Admin could be a bit difficult, particularly relating to styling and accessibility. This led me to create Kubrick UI.
Kubrick UI is a React-based library providing pre-built, customizable parts that seamlessly combine with the WordPress admin house. It improves each visible consistency and accessibility, making it more straightforward so that you can create blank, dynamic interfaces in WordPress Admin, equivalent to making a Customized Settings Pages.
Sooner than we pass additional, I’d suppose that you just’re already conversant in how WordPress plugins paintings. You’re additionally conversant in JavaScript, React, and easy methods to set up Node.js applications with NPM as we gained’t dig into those basics on this educational. Differently, take a look at our articles underneath that can assist you stand up to hurry.
Should you’re waiting, we will now get began with our instructional on easy methods to create our WordPress Settings web page.
Mission Construction
First, we’re going to create and arrange the information required:
. |-- bundle.json |-- settings-page.php |-- src |-- index.js |-- App.js |-- kinds.scss
We’ve got the src
listing containing the supply information, stylesheet, and JavaScript information, which can comprise the app parts and the kinds. We additionally created settings-page.php
, which incorporates the WordPress plugin header in order that we will load our code as a plugin in WordPress. Finally, now we have bundle.json
so we will set up some NPM applications.
NPM Programs
Subsequent, we’re going to set up the @syntatis/kubrick
bundle for our UI parts, in addition to a couple of different applications that it is determined by and a few that we want to construct the web page: @wordpress/api-fetch
, @wordpress/dom-ready
, react
, and react-dom
.
npm i @syntatis/kubrick @wordpress/api-fetch @wordpress/dom-ready react react-dom
And the @wordpress/scripts
bundle as a construction dependency, to permit us to assemble the supply information simply.
npm i @wordpress/scripts -D
Operating the Scripts
Inside the bundle.json
, we upload a few customized scripts, as follows:
{ "scripts": { "construct": "wp-scripts construct", "get started": "wp-scripts get started" } }
The construct
script will let us assemble the information inside the src
listing into information that we can load at the Settings Web page. Throughout construction, we’re going to run the get started
script.
npm run get started
After operating the script, you must in finding the compiled information within the construct
listing:
. |-- index.asset.php |-- index.css |-- index.js
Create the Settings Web page
There are a number of steps we’re going to do and tie in combination to create the Settings Web page.
First, we’re going to replace our settings-page.php
record to sign up our settings web page in WordPress, and sign up the settings and the ideas for the web page.
add_action('admin_menu', 'add_submenu'); serve as add_submenu() { add_submenu_page( 'options-general.php', // Guardian slug. 'Kubrick Settings', 'Kubrick', 'manage_options', 'kubrick-setting', serve as () { ?>'string', 'sanitize_callback' => 'sanitize_text_field', 'default' => 'footer text', 'show_in_rest' => true, ] ); } add_action('admin_init', 'register_settings'); add_action('rest_api_init', 'register_settings');
Right here, we’re including a submenu web page beneath the Settings menu in WordPress Admin. We additionally sign up the settings and ideas for the web page. The register_setting
serve as is used to sign up the atmosphere, and the show_in_rest
parameter is ready to true
, which is necessary to make the atmosphere and the choice to be had within the WordPress /wp/v2/settings
REST API.
The following factor we’re going to do is enqueue the stylesheet and JavaScript information that we’ve got compiled within the construct
listing. We’re going to do that by means of including an motion hook to the admin_enqueue_scripts
motion.
add_action('admin_enqueue_scripts', serve as () { $property = come with plugin_dir_path(__FILE__) . 'construct/index.asset.php'; wp_enqueue_script( 'kubrick-setting', plugin_dir_url(__FILE__) . 'construct/index.js', $property['dependencies'], $property['version'], true ); wp_enqueue_style( 'kubrick-setting', plugin_dir_url(__FILE__) . 'construct/index.css', [], $property['version'] ); });
Should you load WordPress Admin, you must now see the brand new submenu beneath Settings. At the web page of this submenu, we render a div
with the ID root
the place we’re going to render our React software.
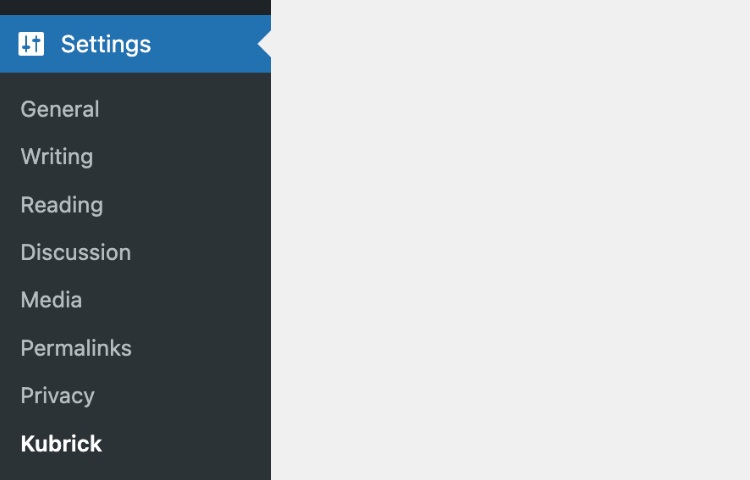
At this level, there’s not anything to look at the web page simply but. We can want to create a React part and render it at the web page.
Making a React part
To create the React software, we first upload the App serve as part in our App.js
record. We additionally import the index.css
from the @syntatis/kubrick
bundle inside this record to use the elemental kinds to one of the vital parts.
import '@syntatis/kubrick/dist/index.css'; export const App = () => { go backHi International from App
; };
Within the index.js
, we load and render our App
part with React.
import domReady from '@wordpress/dom-ready'; import { createRoot } from 'react-dom/consumer'; import { App } from './App'; domReady( () => { const container = report.querySelector( '#root' ); if ( container ) { createRoot( container ).render(); } } );
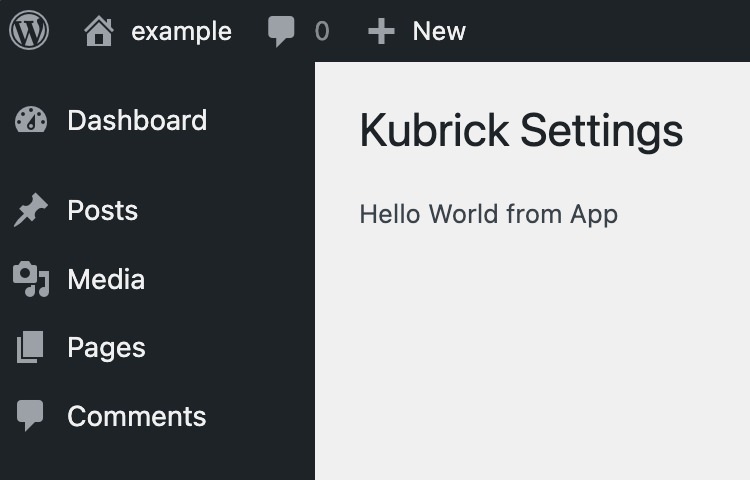
The usage of the UI parts
On this instance, we’d like so as to add a textual content enter at the Settings Web page which can permit the consumer to set the textual content that shall be displayed within the admin footer.
Kubrick UI recently provides round 18 parts. To create the instance discussed, we will use the TextField
part to create an enter box for the “Admin Footer Textual content” atmosphere, permitting customers to switch the textual content displayed within the WordPress admin footer. The Button part is used to put up the shape and save the settings. We additionally use the Realize
part to turn comments to the consumer, equivalent to when the settings are effectively stored or if an error happens throughout the method. The code fetches the present settings on web page load and updates them by means of an API name when the shape is submitted.
import { useEffect, useState } from 'react'; import apiFetch from '@wordpress/api-fetch'; import { Button, TextField, Realize } from '@syntatis/kubrick'; import '@syntatis/kubrick/dist/index.css'; export const App = () => { const [status, setStatus] = useState(null); const [statusMessage, setStatusMessage] = useState(null); const [values, setValues] = useState(); // Load the preliminary settings when the part mounts. useEffect(() => { apiFetch({ trail: '/wp/v2/settings' }) .then((information) => { setValues({ admin_footer_text: information?.admin_footer_text, }); }) .catch((error) => { setStatus('error'); setStatusMessage('An error took place. Please attempt to reload the web page.'); console.error(error); }); }, []); // Deal with the shape submission. const handleSubmit = (e) => { e.preventDefault(); const information = new FormData(e.goal); apiFetch({ trail: '/wp/v2/settings', approach: 'POST', information: { admin_footer_text: information.get('admin_footer_text'), }, }) .then((information) => { setStatus('luck'); setStatusMessage('Settings stored.'); setValues(information); }) .catch((error) => { setStatus('error'); setStatusMessage('An error took place. Please check out once more.'); console.error(error); }); }; if (!values) { go back; } go back ( <> {standing &&> ); }; export default App;setStatus(null)}>{statusMessage} }
Conclusion
We’ve simply created a easy customized settings web page in WordPress the use of React parts and the Kubrick UI library.
Our Settings Web page right here isn’t highest, and there are nonetheless many stuff lets enhance. For instance, lets upload extra parts to make the web page extra obtainable or upload extra options to make the web page extra user-friendly. Shall we additionally upload extra error dealing with or upload extra comments to the consumer when the settings are stored. Since we’re operating with React, you’ll be able to additionally make the web page extra interactive and visually interesting.
I’m hoping this educational is helping you get began with making a customized settings web page in WordPress the use of React parts. You’ll be able to in finding the supply code for this educational on GitHub, and be at liberty to make use of it as a place to begin to your personal initiatives.
The put up The way to Create a WordPress Settings Web page with React seemed first on Hongkiat.
WordPress Website Development Source: https://www.hongkiat.com/blog/wordpress-settings-page-with-react/