Over time, we now have explored Gutenberg from many views. Now we have dissected the editor’s functionalities, when put next them with the ones of different web page developers, constructed static and dynamic customized blocks, and a lot more.
With the newest variations of WordPress, new options and equipment permit you to create advanced layouts extra simply by means of enriching and embellishing the capability of the block editor with out the wish to construct customized blocks.
Because of the advent of block patterns and developer equipment such because the Block Bindings API, there are fewer use circumstances for customized blocks. You’ll be able to now create advanced block buildings, inject metadata values into your content material, and automate a just right a part of your workflow with out leaving the editor’s interface. In brief, these days, WordPress permits you to create advanced websites with as few clicks as ever prior to.
Including customized controls and equipment to the editor would possibly assist in making the content material advent procedure smoother. As an example, it’s possible you’ll wish to upload a panel to the Put up sidebar so as to add capability or create a customized sidebar to control more than one meta fields.
Let’s get began!
Learn how to create a block editor plugin with out making a customized block
WordPress supplies a handy command-line device that lets you set up a neighborhood Node.js construction surroundings with all of the important information and dependencies to create a customized block. Simply kind npx @wordpress/create-block
within the command line device, wait a couple of seconds, and you might be accomplished.
Alternatively, scaffolding a customized block isn’t important while you best wish to upload a sidebar or a easy settings panel. On this case, you want to create a Gutenberg plugin.
WordPress does now not supply a application to automate the method of making a plugin for Gutenberg, so you want to do it manually. However don’t worry an excessive amount of. The method is somewhat easy, and we can force you thru it. Those are the stairs to observe:
1. Obtain and set up a neighborhood construction surroundings
First issues first: Even though you’ll be able to broaden your Gutenberg plugin in a far flung surroundings, it can be extra handy so that you can set up a construction WordPress web site in the community. You’ll be able to use any surroundings in response to PHP and MySQL. Some of the many possible choices to be had in the market, we suggest DevKinsta. It’s unfastened, absolutely featured, simple to make use of, and 100% suitable with Kinsta internet hosting.
After getting your construction website arrange, you are prepared to create a Gutenberg block editor plugin.
2. Obtain and set up Node.js and npm
Obtain Node.js from nodejs.org and set up it for your pc. This may occasionally additionally set up npm, the Node package deal supervisor.
If you’ve accomplished this, release your command-line device and run node -v
and npm -v
. You must see the put in variations of Node.js and npm.
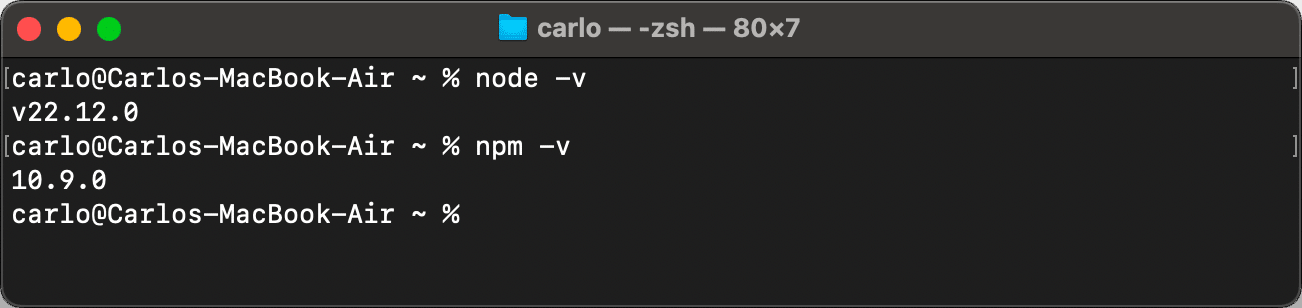
3. Create your plugin’s folder
Create a brand new folder underneath wp-content/plugins
and rename it my-sidebar-plugin
or one thing identical. Simply take into account that this identify must replicate your plugin’s identify.
Open the terminal, navigate to the plugin’s folder, and initialize an npm undertaking with the next command:
npm init -y
This may occasionally create a fundamental package deal.json
report.
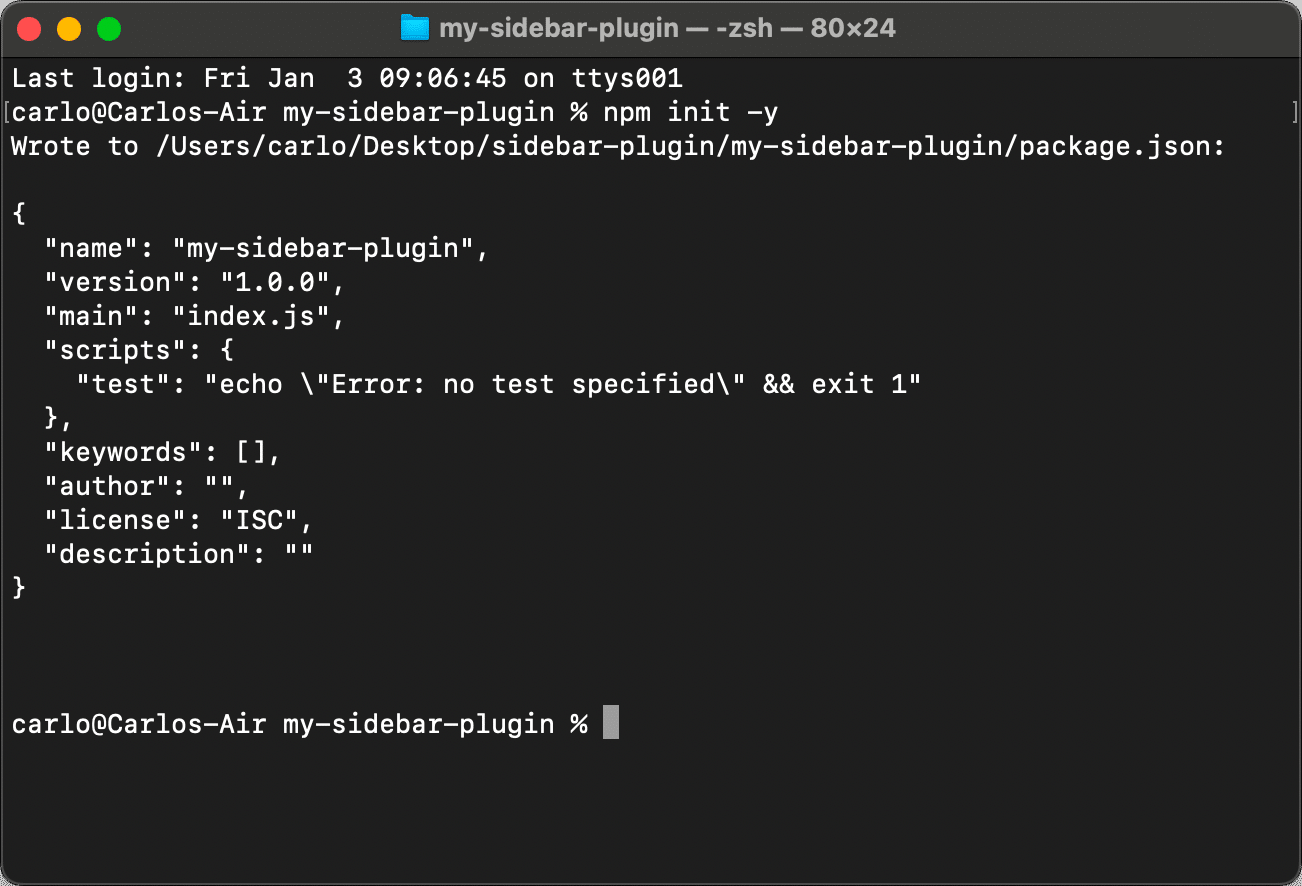
4. Set up dependencies
For your command-line device, kind the next command:
npm set up @wordpress/plugins @wordpress/scripts --save-dev
@wordpress/plugins
installs theplugins
module for WordPress.@wordpress/scripts
installs a choice of reusable scripts for WordPress construction.
A brand new node_modules
folder must had been added in your undertaking.
Now, open your package deal.json
and replace the scripts
as follows:
{
"identify": "my-sidebar-plugin",
"model": "1.0.0",
"major": "index.js",
"scripts": {
"construct": "wp-scripts construct",
"get started": "wp-scripts get started"
},
"key phrases": [],
"creator": "",
"license": "ISC",
"description": "",
"devDependencies": {
"@wordpress/plugins": "^7.14.0",
"@wordpress/scripts": "^30.7.0"
}
}
Now you’ll be able to take a look at the plugin’s folder:
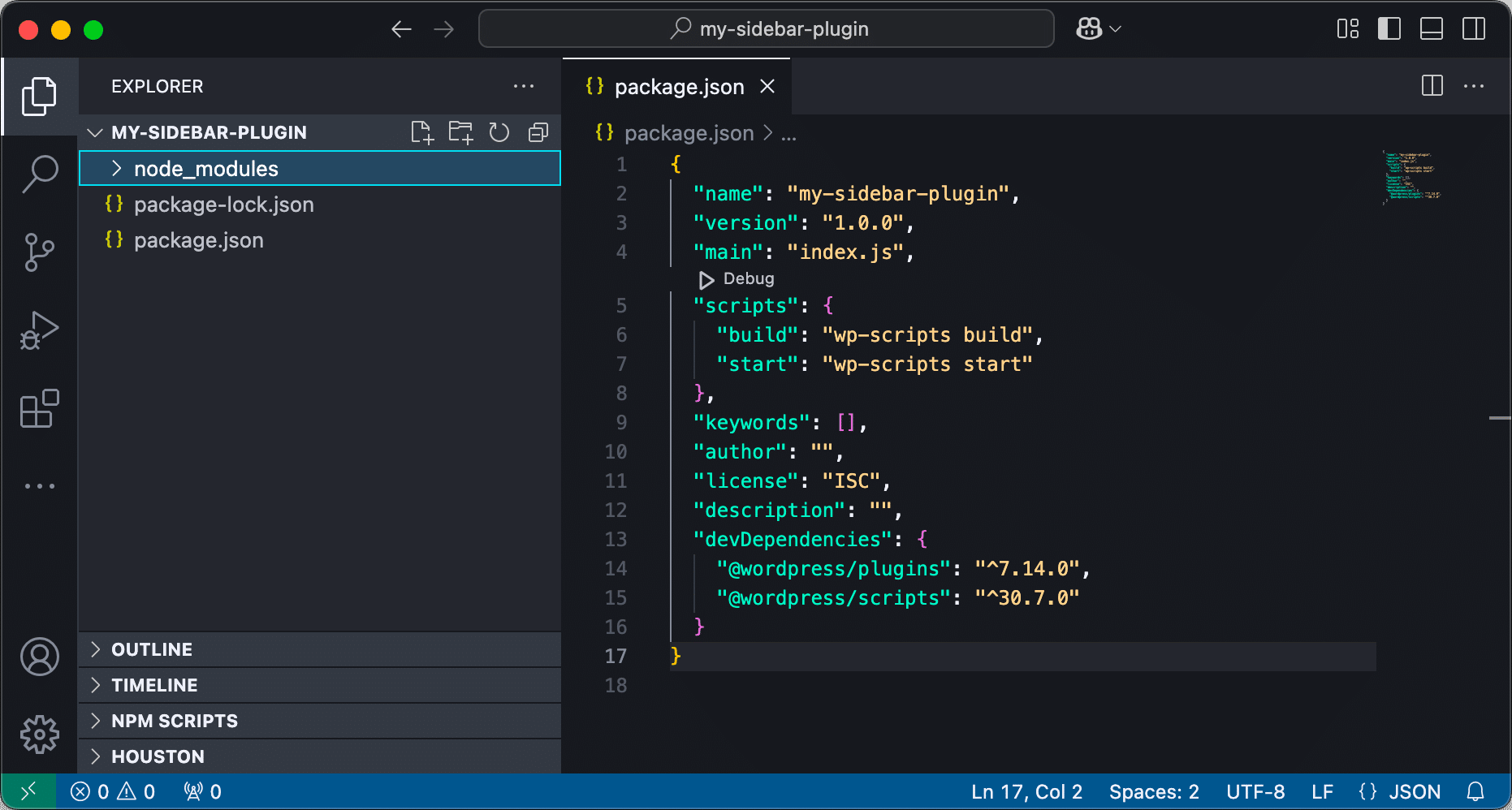
5. Create the plugin’s information
For your plugin’s listing, create a brand new .php
report and provides it the similar identify as your folder. In our instance, it’s my-sidebar-plugin.php
.
Open the report and paste the next code to sign up the plugin at the server:
Subsequent, create a src
folder on your plugin’s listing. In there, create a brand new index.js
report with the next code:
import { registerPlugin } from '@wordpress/plugins';
import { PluginSidebar } from '@wordpress/edit-post';
const MyPluginSidebar = () => (
Hi my buddies!
);
registerPlugin( 'my-sidebar-plugin', {
render: MyPluginSidebar,
} );
6. Assemble the code
All this is lacking is the construct. Return to the command line and run the next command:
npm run construct
This creates a construct
folder with the undertaking’s compressed information.
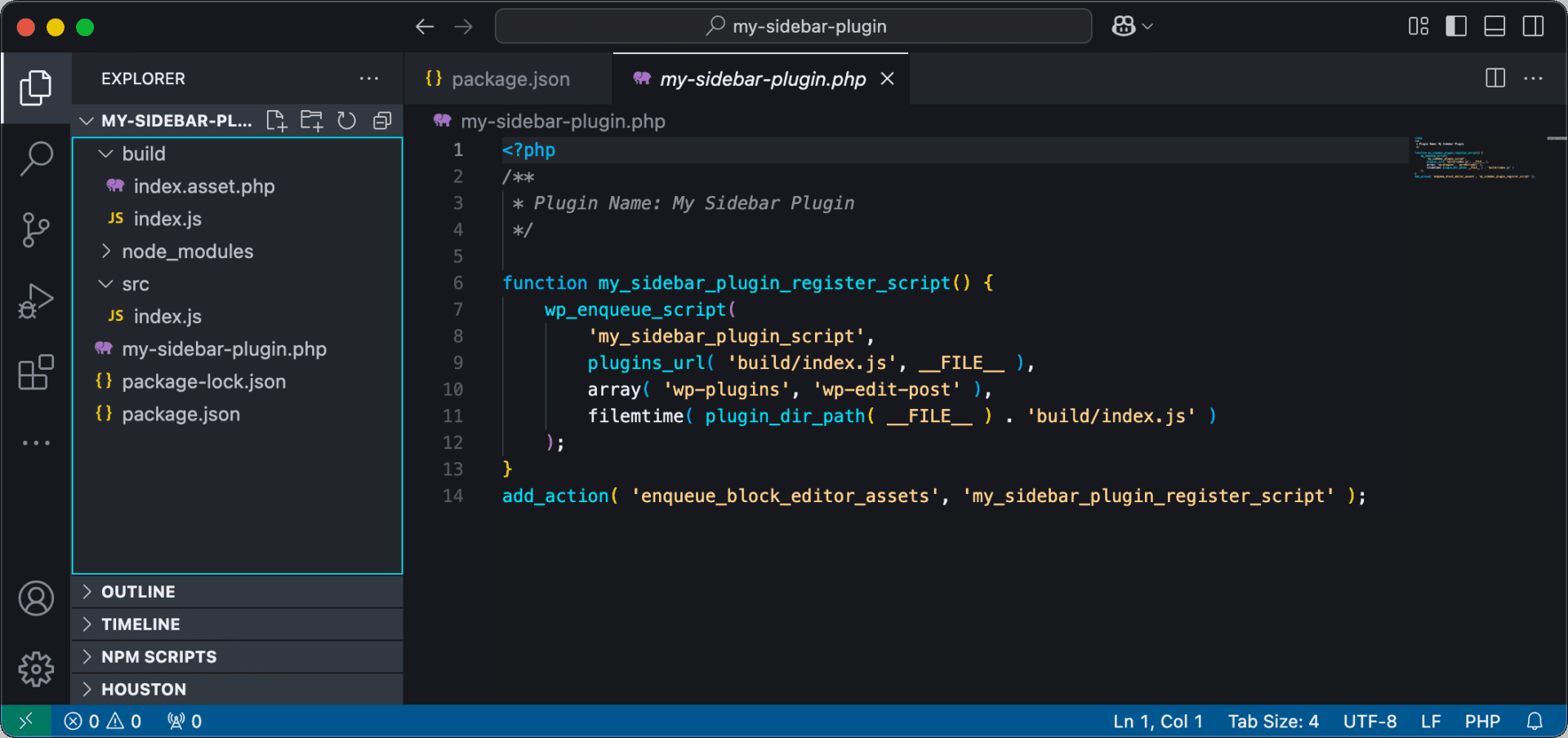
When accomplished, navigate to the Plugins display on your WordPress dashboard and turn on the plugin. Create a brand new publish or web page and click on the plug icon within the most sensible proper nook to show your customized sidebar.
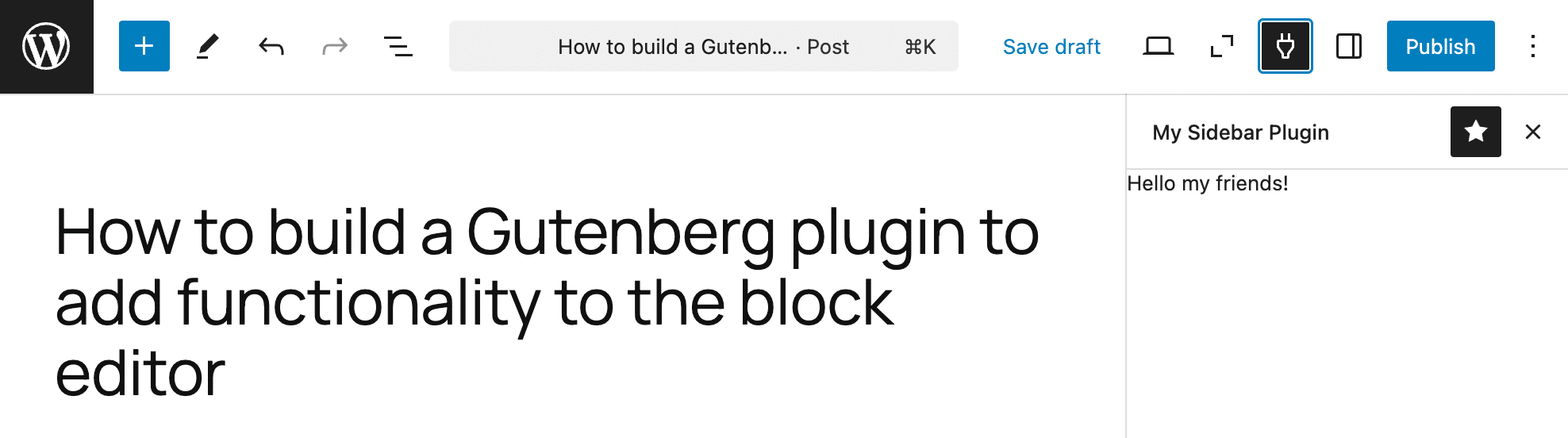
7. Broaden and construct
Now we have positioned the index.js
report within the src
folder. The use of a src
folder is a not unusual conference in WordPress plugin and theme construction, making your code more straightforward for different builders to grasp.
Via putting your JS code within the src
folder, you'll be able to use the npm get started
or npm run get started
command to start out a construction surroundings that screens the scripts and routinely recompiles the code when important. When you're accomplished with construction, the npm construct
or npm run construct
command will bring together the JavaScript code into the construct
folder, which can comprise the code optimized for manufacturing.
Now, let’s put what we discovered into apply and regulate the plugin we now have created within the earlier phase so as to add a brand new panel to the publish editor sidebar to control customized fields.
Learn how to create an extra sidebar to control publish meta fields
Our objective is to create a customized sidebar that comprises a panel with a unmarried textual content box for including and enhancing a customized meta box.
Ahead of going into it, we must point out that lets use a customized meta field to succeed in the similar consequence. With WordPress 6.7, meta bins have won an improve and are actually absolutely suitable with the block editor, so it's possible you'll surprise why arrange meta fields from a customized sidebar as a substitute of a meta field. The reason being {that a} sidebar permits you to make the most of integrated parts. This is helping you construct extra pleasant interfaces with tough controls that are supposed to even be acquainted to customers.
That being mentioned, here's the method for making a customized sidebar that lets you arrange customized fields from throughout the editor.
my-sidebar-plugin.php
Step 1: Check in publish meta
First, you want to sign up the meta box. Upload the next code to the primary report of the plugin:
serve as my_sidebar_plugin_register_meta() {
register_post_meta(
'publish',
'meta_fields_book_title',
array(
'show_in_rest' => true,
'kind' => 'string',
'unmarried' => true,
'sanitize_callback' => 'sanitize_text_field',
'label' => __( 'Ebook name', 'my-sidebar-plugin' ),
'auth_callback' => serve as() {
go back current_user_can( 'edit_posts' );
}
)
);
}
add_action( 'init', 'my_sidebar_plugin_register_meta' );
The register_post_meta
serve as accepts 3 arguments:
- The publish kind to sign up a meta key for. Atmosphere an empty string would sign up the meta key throughout all current publish varieties.
- The meta key to sign up. Observe that we aren't the usage of an underscore at first of the meta key. Prefixing the meta key with an underscore would conceal the customized box, so it's possible you'll need to use it in a meta field. Alternatively, hiding the customized box would save you the meta box from getting used by the use of the Block Bindings API within the publish content material.
- An array of arguments. Observe that you simply should set
show_in_rest
totrue
. This exposes the meta box to the Leisure API and permits us to bind the meta box to dam attributes. For the opposite attributes, see the serve as reference.
Step 2: Check in meta field
To verify backward compatibility in your plugin, you want to sign up a customized meta field to permit customers to control your customized fields, even though the usage of the vintage editor. Upload the next code in your plugin’s PHP report:
/**
* Check in meta field
*
* @hyperlink https://developer.wordpress.org/reference/purposes/add_meta_box/
*
*/
serve as my_sidebar_plugin_register_meta_box(){
add_meta_box(
'book_meta_box', // Distinctive ID
__( 'Ebook main points' ), // Field name
'my_sidebar_plugin_meta_box_callback', // Content material callback
array( 'publish' ), // Put up varieties
'complex', // context
'default', // precedence
array('__back_compat_meta_box' => true) // conceal the meta field in Gutenberg
);
}
add_action( 'add_meta_boxes', 'my_sidebar_plugin_register_meta_box' );
Now claim the callback that builds the shape:
/**
* Construct meta field shape
*
* @hyperlink https://developer.wordpress.org/reference/purposes/wp_nonce_field/
* @hyperlink https://developer.wordpress.org/reference/purposes/get_post_meta/
*
*/
serve as my_sidebar_plugin_meta_box_callback( $publish ){
wp_nonce_field( 'my_sidebar_plugin_save_meta_box_data', 'my_sidebar_plugin_meta_box_nonce' );
$name = get_post_meta( $post->ID, 'meta_fields_book_title', true );
?>
Subsequent, write the serve as to avoid wasting your meta fields into the database:
/**
* Save metadata
*
* @hyperlink https://developer.wordpress.org/reference/purposes/wp_verify_nonce/
* @hyperlink https://developer.wordpress.org/reference/purposes/current_user_can/
* @hyperlink https://developer.wordpress.org/reference/purposes/sanitize_text_field/
* @hyperlink https://developer.wordpress.org/reference/purposes/update_post_meta/
*
*/
serve as my_sidebar_plugin_save_meta_box_data( $post_id ) {
if ( ! isset( $_POST['my_sidebar_plugin_meta_box_nonce'] ) )
go back;
if ( ! wp_verify_nonce( $_POST['my_sidebar_plugin_meta_box_nonce'], 'my_sidebar_plugin_save_meta_box_data' ) )
go back;
if ( outlined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE )
go back;
if ( ! current_user_can( 'edit_post', $post_id ) )
go back;
if ( ! isset( $_POST['meta_fields_book_title'] ) )
go back;
$name = sanitize_text_field( $_POST['meta_fields_book_title'] );
update_post_meta( $post_id, 'meta_fields_book_title', $name );
}
add_action( 'save_post', 'my_sidebar_plugin_save_meta_box_data' );
We received’t dive deeper into this code since it's past the scope of this text, however you'll be able to in finding all of the data you want by means of following the hyperlinks within the serve as headers.
Ultimate, we wish to enqueue our plugin’s index.js
report:
serve as my_sidebar_plugin_register_script() {
wp_enqueue_script(
'my_sidebar_plugin_script',
plugins_url( 'construct/index.js', __FILE__ ),
array( 'wp-plugins', 'wp-edit-post' ),
filemtime( plugin_dir_path( __FILE__ ) . 'construct/index.js' )
);
}
add_action( 'enqueue_block_editor_assets', 'my_sidebar_plugin_register_script' );
That’s fascinated by the PHP report. Subsequent, we wish to write the JS code.
index.js
Your index.js
is positioned within the src
folder, which is the place you retailer your JS information right through the improvement section.
Open your index.js
and upload the next import
declarations:
import { __ } from '@wordpress/i18n';
import { registerPlugin } from '@wordpress/plugins';
import { PluginSidebar } from '@wordpress/editor';
import { PanelBody, PanelRow, TextControl } from '@wordpress/parts';
import { useSelect } from '@wordpress/information';
import { useEntityProp } from '@wordpress/core-data';
You wish to have those assets to construct the sidebar with the specified controls.
Subsequent, you want to construct the sidebar element:
const MyPluginSidebar = () => {
const postType = useSelect(
( choose ) => choose( 'core/editor' ).getCurrentPostType(),
[]
);
const [ meta, setMeta ] = useEntityProp( 'postType', postType, 'meta' );
const bookTitle = meta ? meta[ 'meta_fields_book_title' ] : '';
const updateBookTitleMetaValue = ( newValue ) => {
setMeta( { ...meta, meta_fields_book_title: newValue } );
};
if ( postType === 'publish' ) {
go back (
);
}
};
registerPlugin( 'my-sidebar-plugin', {
render: MyPluginSidebar,
} );
The registerPlugin
serve as registers the plugin and renders the element named MyPluginSidebar
.
The serve as MyPluginSidebar
broadcasts a couple of constants and returns the JSX code of the element.
useSelect
is a customized hook for retrieving props from registered selectors. We used it to get the present publish kind. See additionally this weblog publish from the WordPress Developer Weblog.useEntityProp
returns an array of meta fields and a setter serve as to set new meta values. See additionally the on-line reference.updateBookTitleMetaValue
is an match handler that saves thebookTitle
meta box worth.
We used a couple of integrated parts to construct our sidebar:
PluginSidebar
permits you to upload pieces to the toolbar of both the Put up or Web page editor monitors. (See the element’s reference.)PanelBody
creates a collapsible container that may be toggled open or closed. (See the element’s reference.)PanelRow
is a generic container for rows inside aPanelBody
. (See the element’s reference.)TextControl
is a single-line box that can be utilized without cost textual content access. (See the element’s reference.)
Now run the npm run construct
command, turn on the plugin, and create a brand new publish. A brand new e book icon must seem within the most sensible sidebar. Clicking on that icon displays your plugin sidebar.
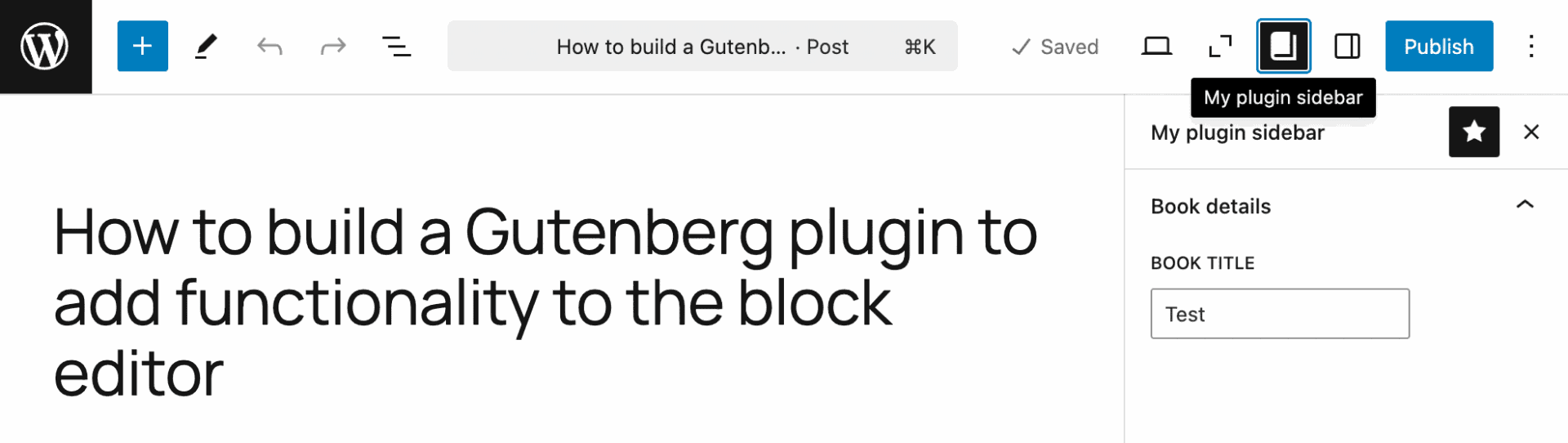
What when you don’t desire a new sidebar however need to show your customized box within the integrated publish sidebar? You simply wish to exchange PluginSidebar
with PluginDocumentSettingPanel
. That is your new index.js
report:
import { __ } from '@wordpress/i18n';
import { registerPlugin } from '@wordpress/plugins';
import { PluginDocumentSettingPanel } from '@wordpress/edit-post';
import { PanelBody, PanelRow, TextControl } from '@wordpress/parts';
import { useSelect } from '@wordpress/information';
import { useEntityProp } from '@wordpress/core-data';
const MyPluginSidebar = () => {
const postType = useSelect(
( choose ) => choose( 'core/editor' ).getCurrentPostType(),
[]
);
const [ meta, setMeta ] = useEntityProp( 'postType', postType, 'meta' );
const bookTitle = meta ? meta[ 'meta_fields_book_title' ] : '';
const updateBookTitleMetaValue = ( newValue ) => {
setMeta( { ...meta, meta_fields_book_title: newValue } );
};
if ( postType === 'publish' ) {
go back (
);
}
};
registerPlugin( 'my-sidebar-plugin', {
render: MyPluginSidebar,
} );
The next symbol displays the end result.
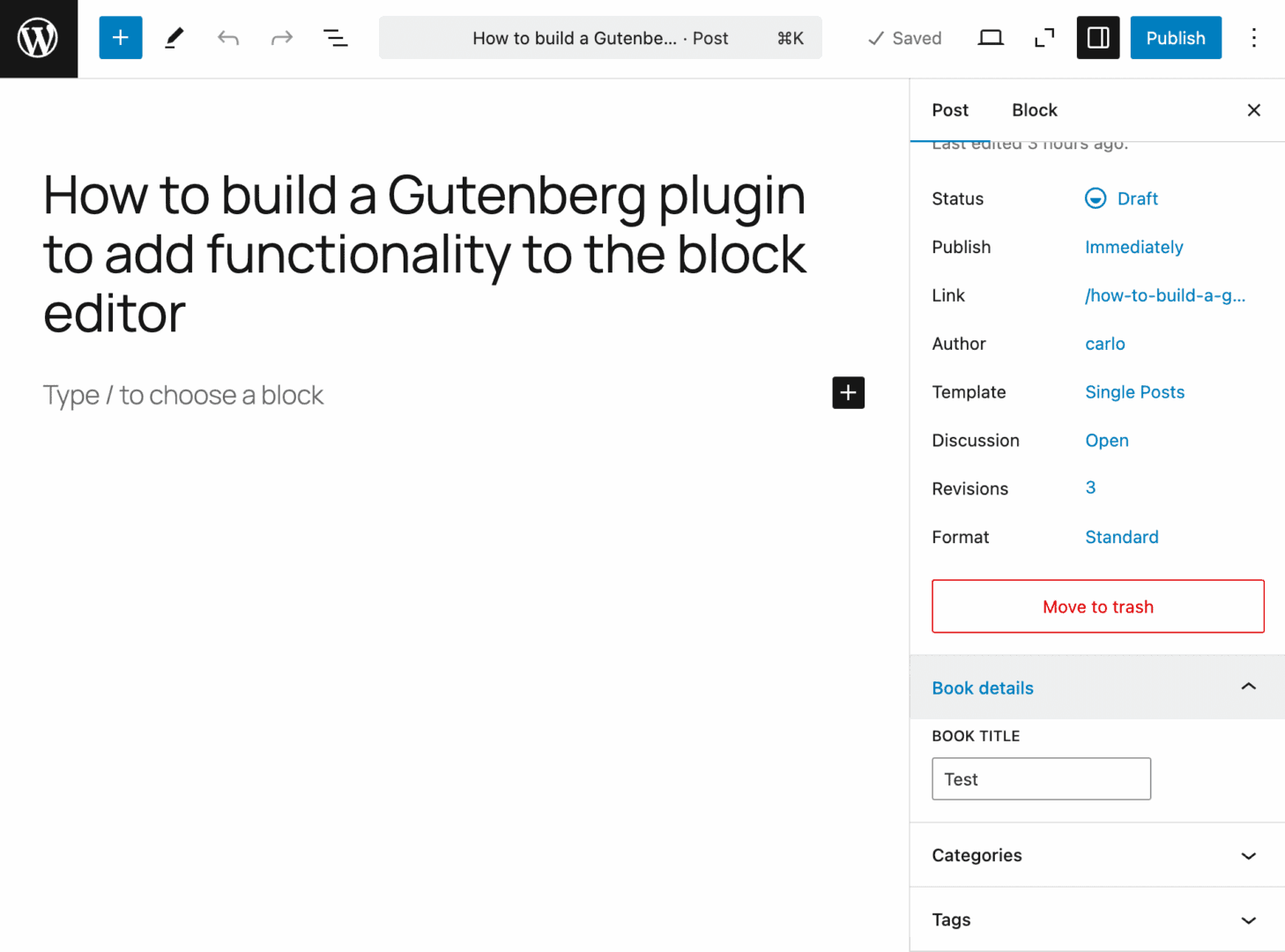
A use case: a block development override to automate your workflow
You'll be able to now upload a worth for the customized box, and it'll be to be had during the Block Bindings API to be used with block attributes. As an example, you'll be able to upload a Paragraph block in your content material and bind the customized box to the paragraph’s content material
characteristic.
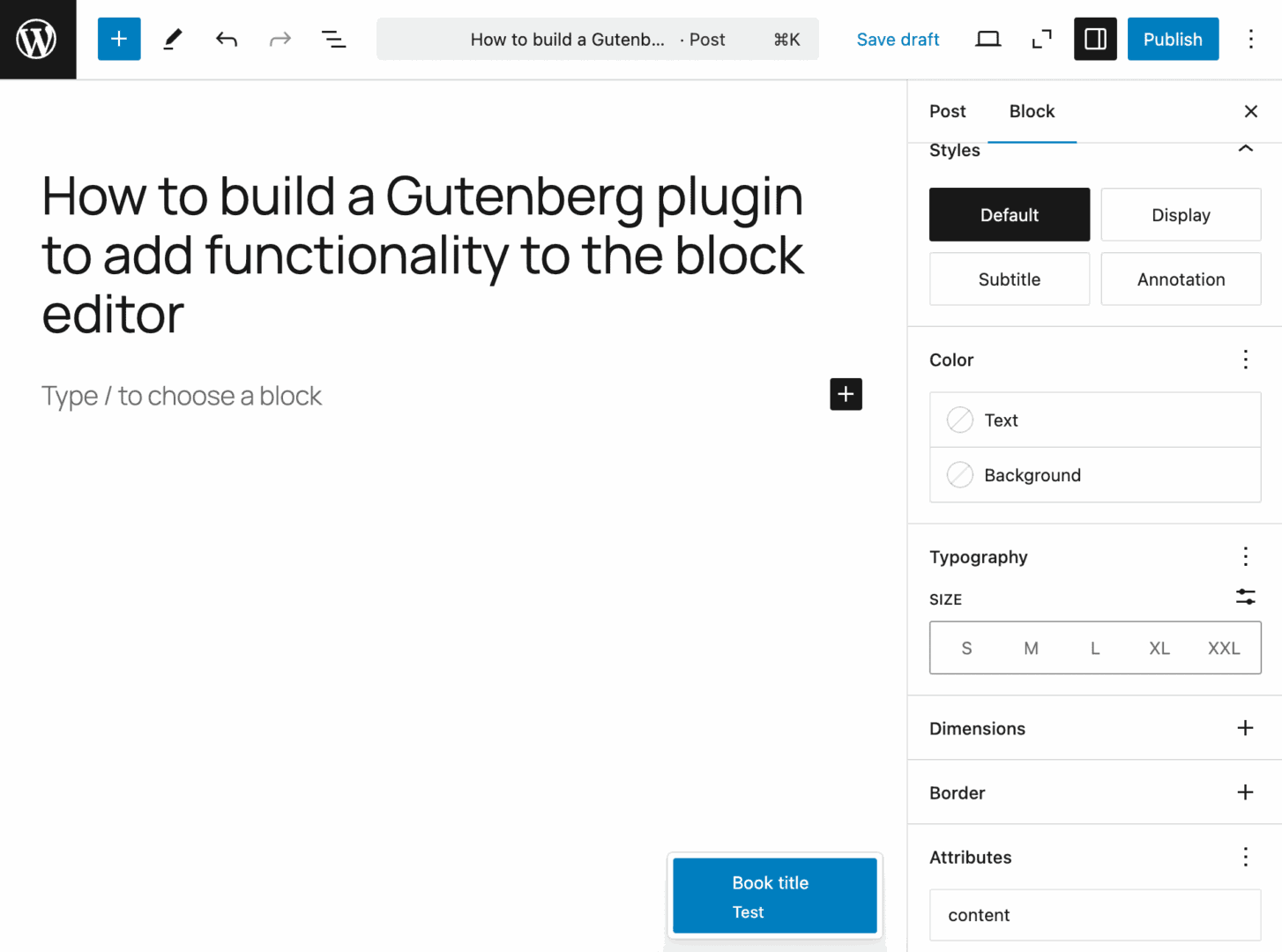
You're unfastened to modify the price of your customized box, and those adjustments shall be routinely implemented to the content material of your paragraph.
If you're questioning if there’s extra that you'll be able to do with customized fields and Block Bindings, the solution is sure! Block patterns and Block Bindings API permit you to automate all the content material advent procedure.
To have a clue, create a development with a minimum of a heading or a paragraph. On this instance, we create a block development with a Columns block, an Symbol, a Heading, and a few Row blocks, together with two paragraphs every.
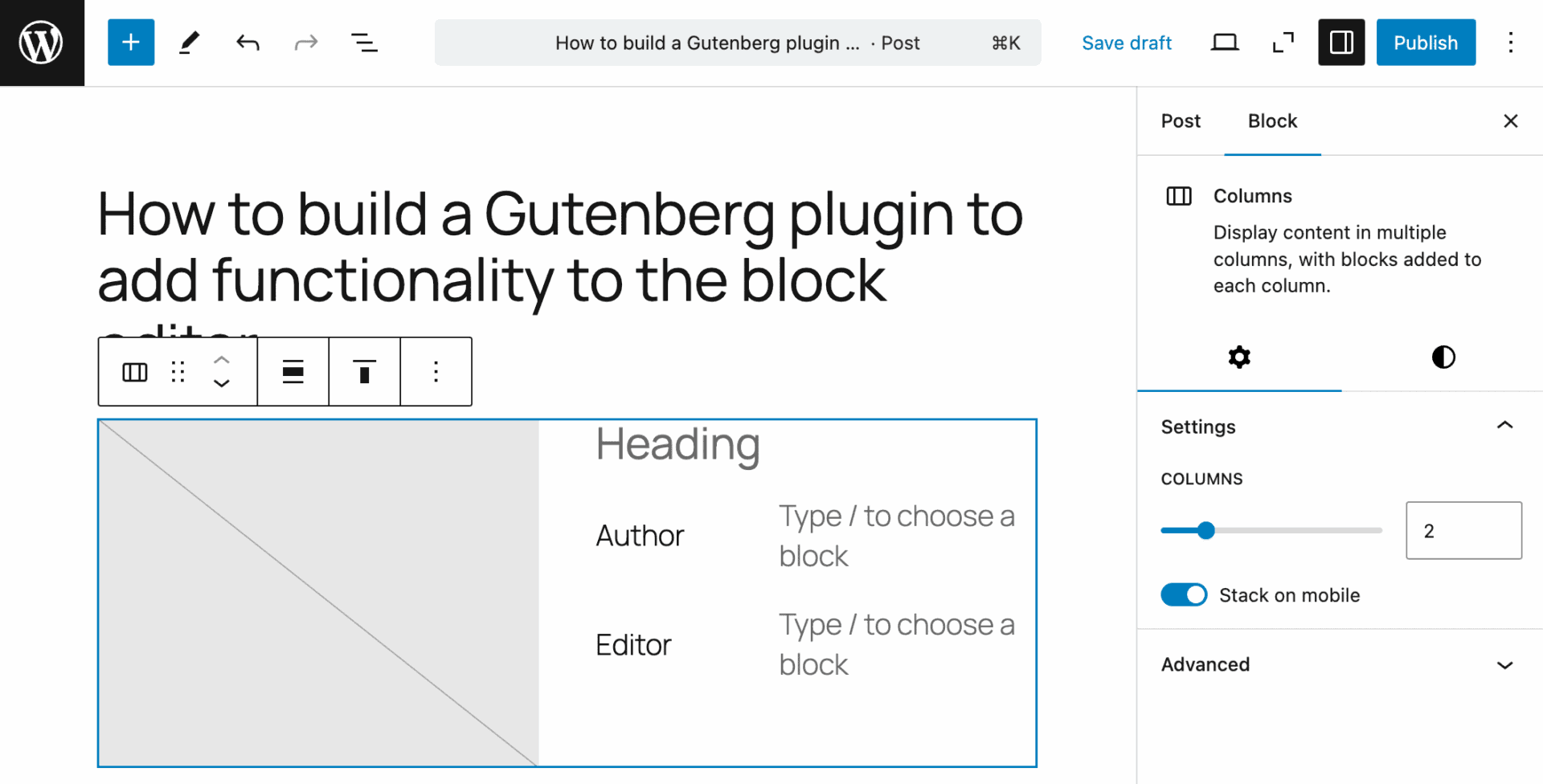
As soon as you might be satisfied along with your format, choose the wrapping parts and create a synced development.
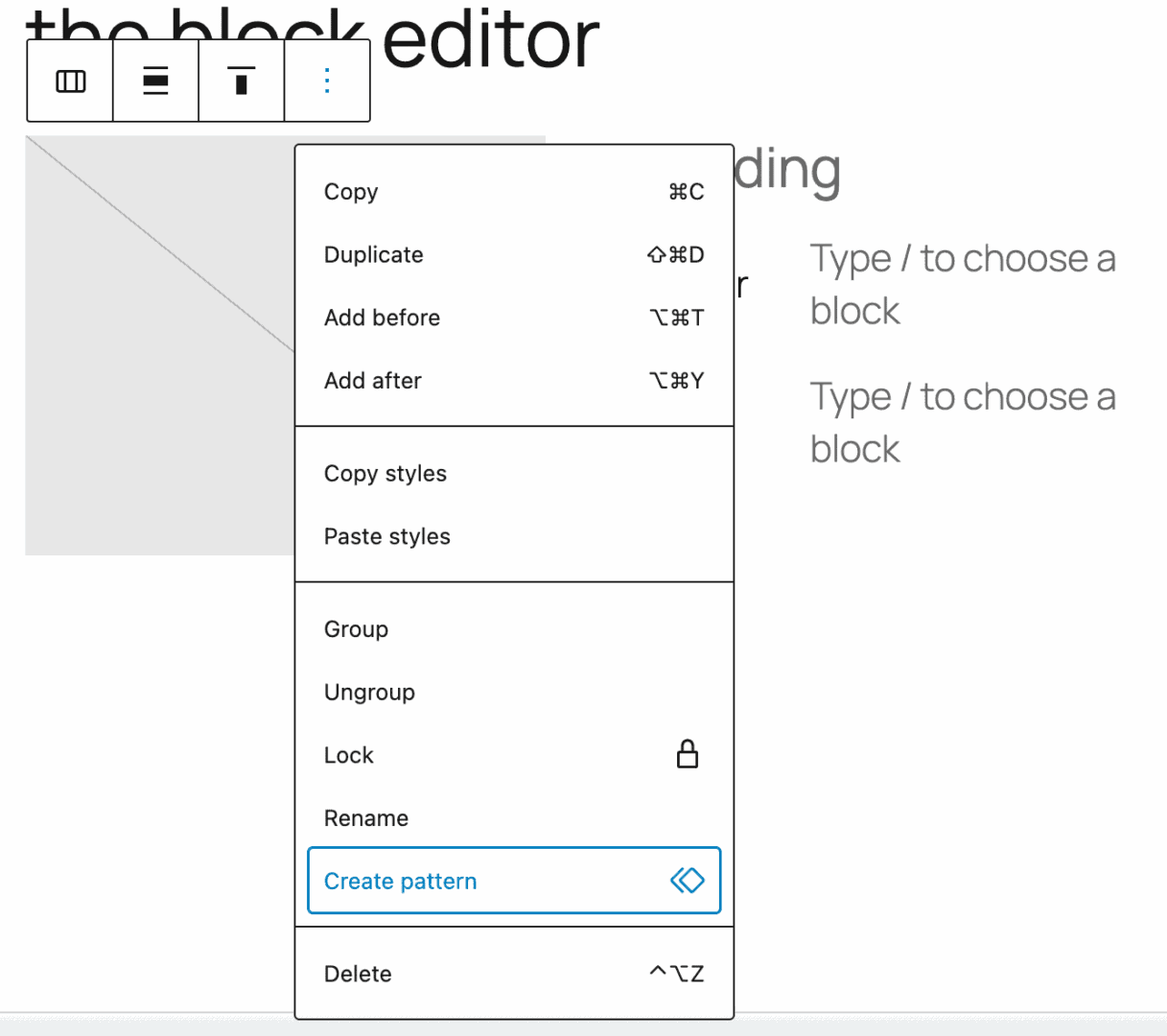
Upload a reputation and a class for the block development, and you'll want to sync it.
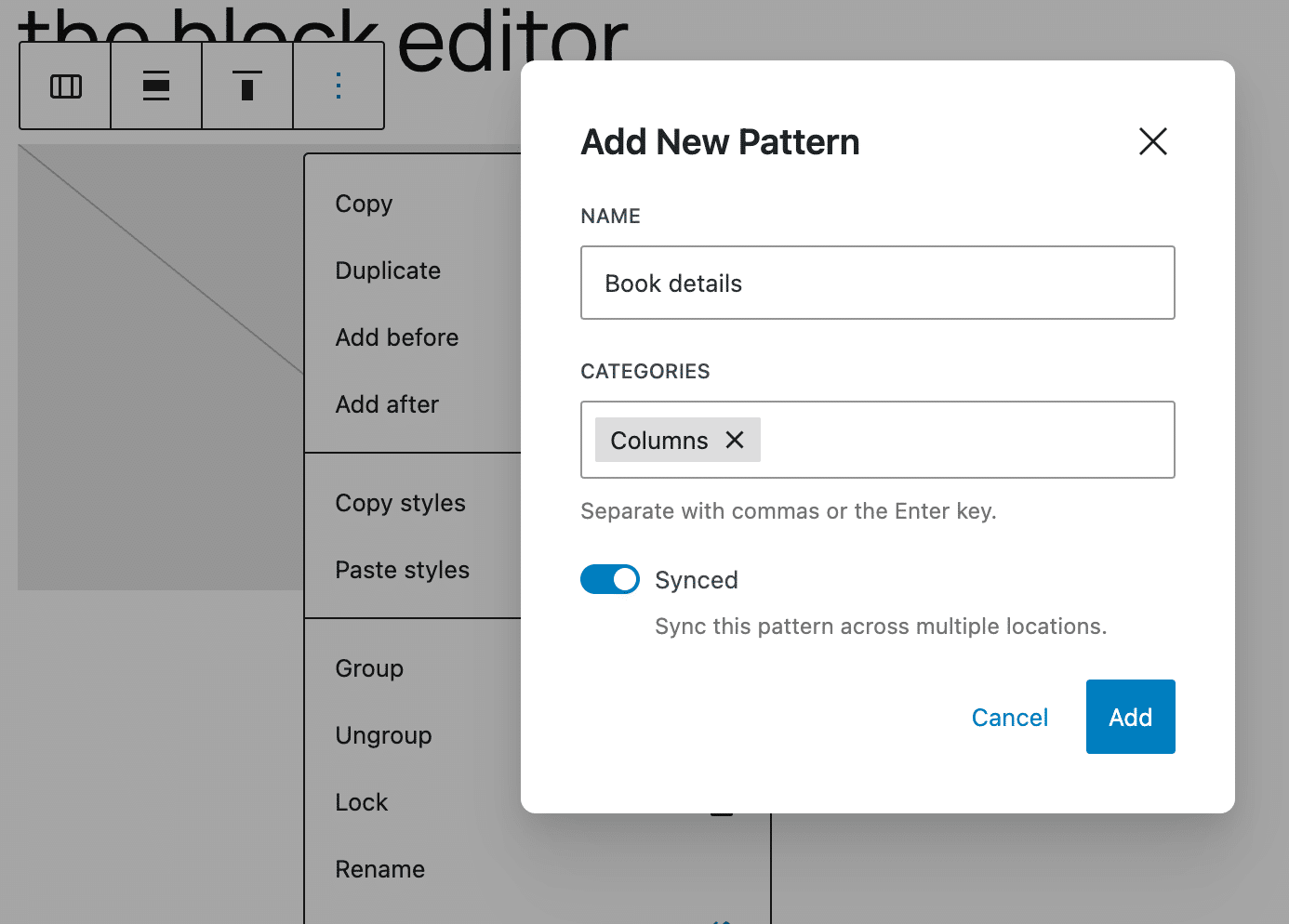
Subsequent, when you created the development within the Put up editor, choose it and click on on Edit authentic within the block toolbar. You'll be able to additionally navigate to the Patterns phase of the Web page editor and in finding the development underneath My Patterns or within the development class you might have set prior to.
Open the Code Editor and in finding the block you wish to have to bind in your customized box. Within the block delimiter, upload the next code:
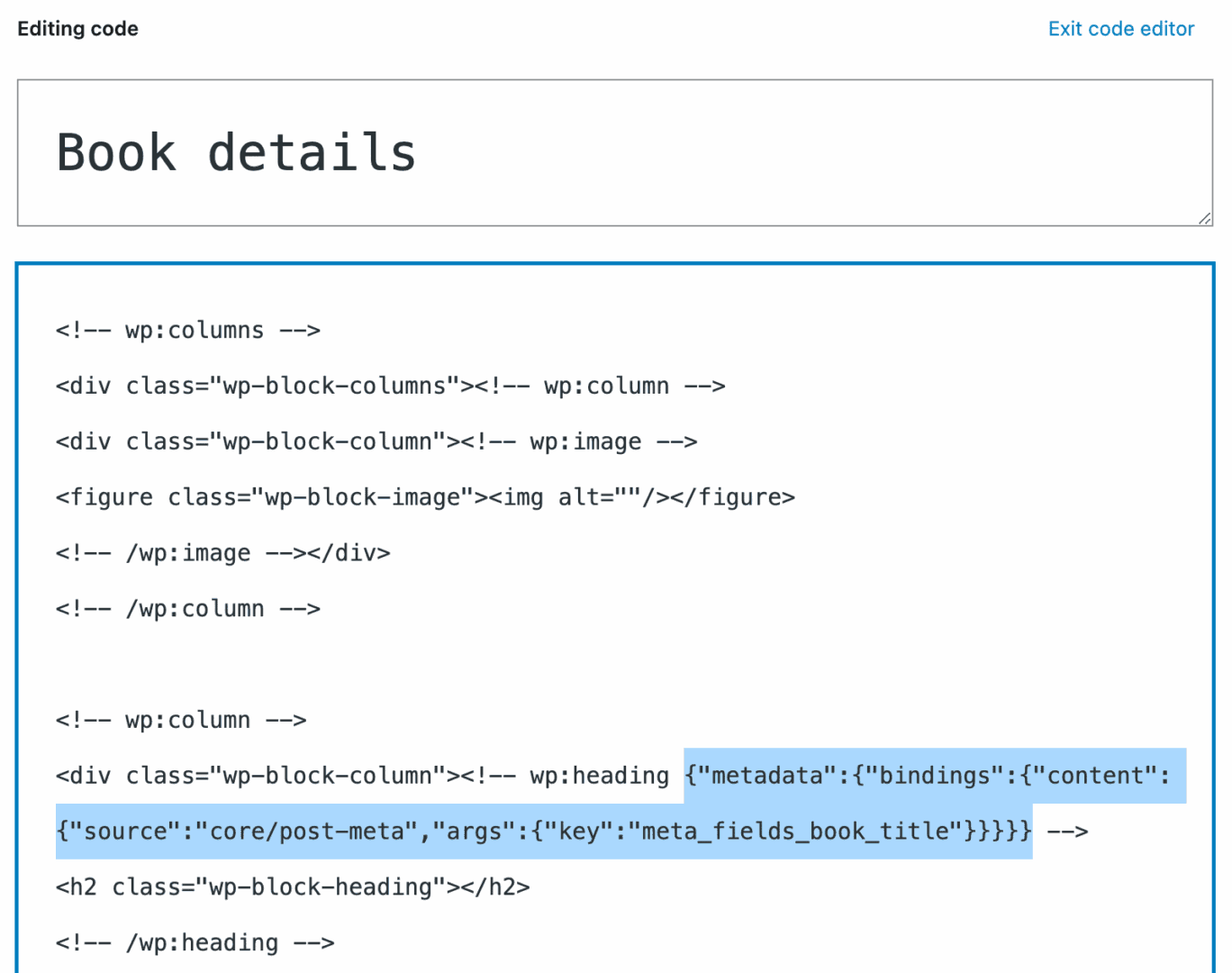
Save the development and create a brand new publish. Upload the development in your content material and set a worth for the customized box. You must see that worth routinely implemented in your development.
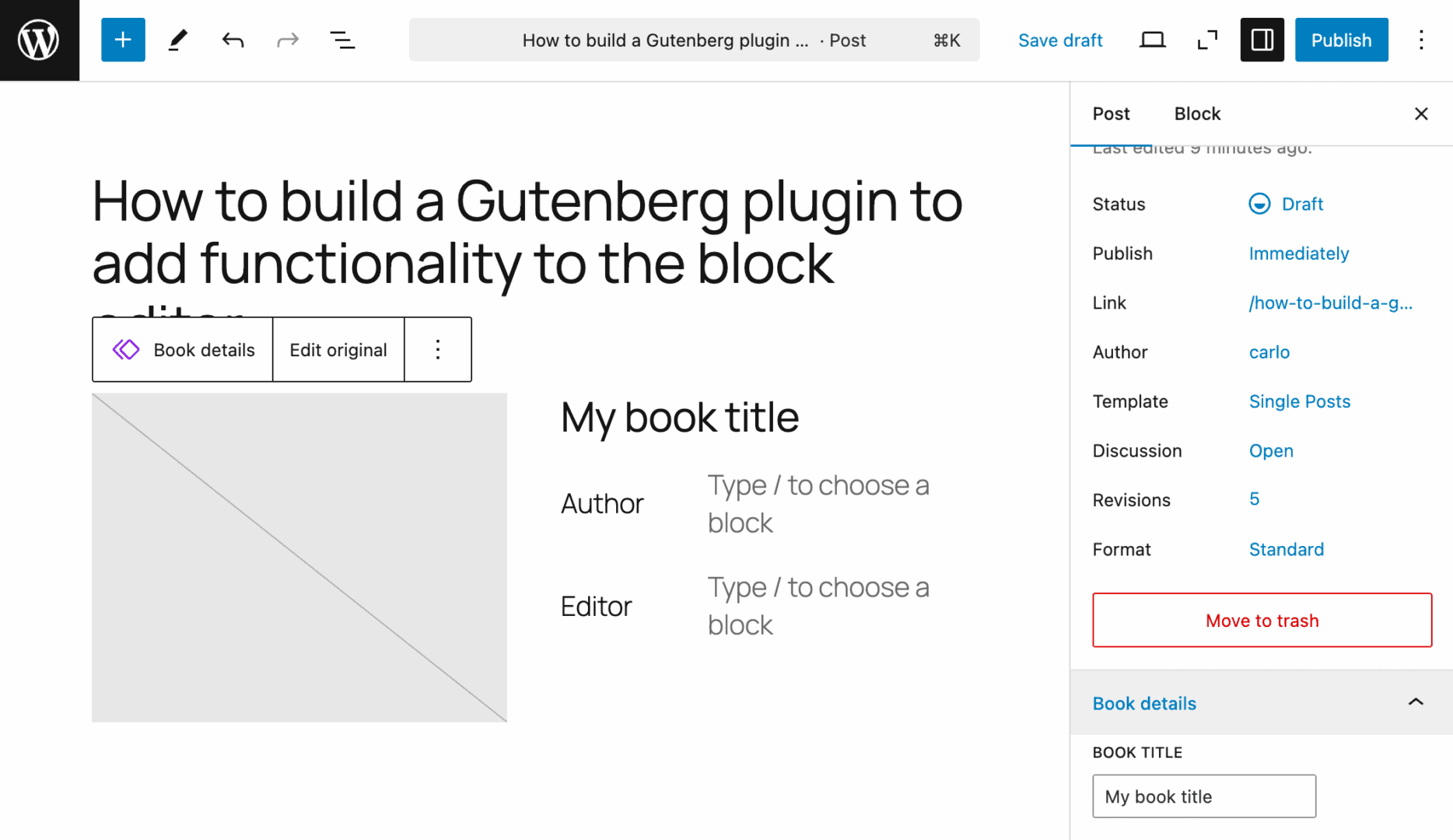
Now, you'll be able to mess around with this plugin. Because of customized fields and the Block Bindings API, you'll be able to upload extra fields and controls to routinely populate your layouts.
Abstract
Growing a customized block can also be difficult. However do you want to construct a block when you'll be able to do extra with a block development?
With the developments in block patterns and the advent of tough developer options, such because the Block Bindings API, developing customized blocks to construct refined and useful web pages is not important. A easy plugin and a block development can successfully automate a good portion of your workflow.
This instructional demonstrated the way to upload capability to the WordPress publish editor thru a plugin. Alternatively, what we now have coated on this publish best scratches the skin of what you'll be able to accomplish with the powerful options WordPress now provides.
Have you ever already explored those options and added capability to the WordPress editor? If this is the case, be happy to proportion your studies and insights within the feedback phase underneath.
The publish Learn how to construct a Gutenberg plugin so as to add capability to the block editor seemed first on Kinsta®.
WP Hosting