As a Google Chrome consumer, you’ve most certainly used some extensions in that browser. Have you ever ever questioned how they’re constructed or if that you must construct one?
This newsletter guides you throughout the procedure of constructing a Chrome extension, in particular person who makes use of React and the Kinsta API to control plugins on WordPress websites hosted with Kinsta.
What’s a Chrome extension?
A Chrome extension is a program put in within the Chrome browser and complements its capability. Extensions can vary from easy icon buttons within the toolbar to totally built-in options that engage deeply together with your surfing enjoy.
Learn how to create a Chrome extension
Making a Chrome extension is very similar to growing a internet utility, nevertheless it calls for a JSON-formatted report referred to as manifest.json. This report acts because the spine of the extension, dictating its settings, permissions, and functionalities you want to come with.
To start out, create a folder that can grasp your whole extension information. Subsequent, create a manifest.json report within the folder.
A elementary manifest.json report for a Chrome extension contains key houses that outline the extension’s elementary settings. Under is an instance of a manifest.json report that comes with the important fields to make it paintings:
{
"manifest_version": 3,
"title": "My Chrome extension",
"model": "1.0",
"description": "Here's a description for my Chrome extension."
}
You’ll be able to load and take a look at this as an unpacked extension to Chrome. Navigate to chrome://extensions
to your browser and toggle Developer mode, then click on the Load Unpacked button. This may occasionally open a report browser, and you’ll make a selection the listing you created on your extension.
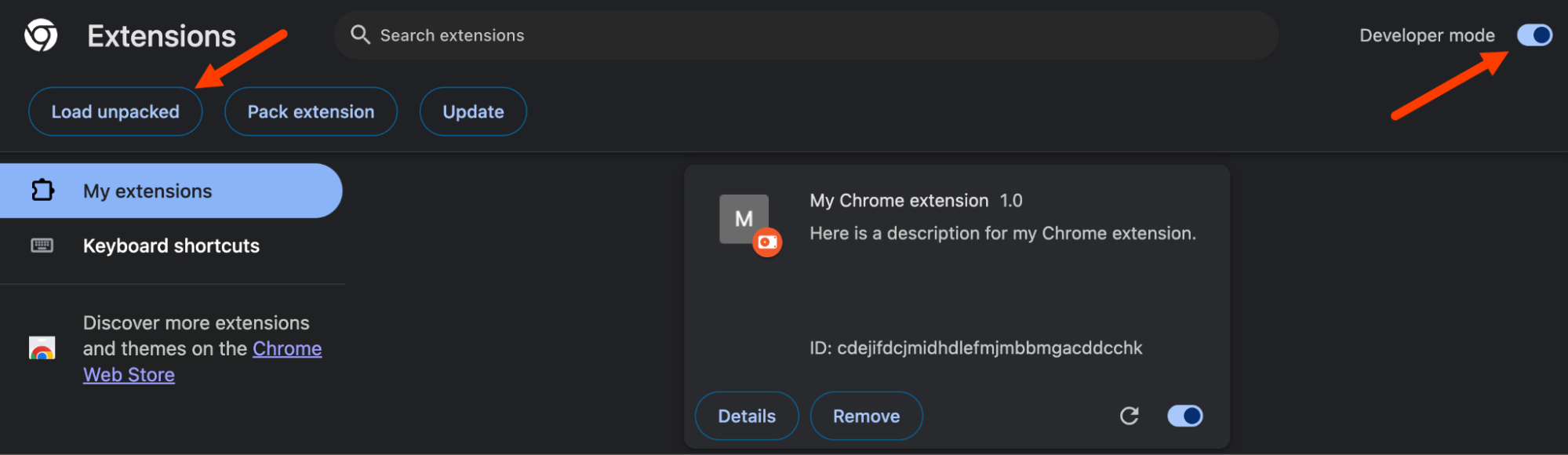
Whilst you click on the extension icon, not anything will occur as a result of you haven’t created a consumer interface.
Create a consumer interface (popup) on your Chrome extension
Like with each and every internet utility, the consumer interface (UI) of your extension makes use of HTML to construction the content material, CSS to taste it, and JavaScript so as to add interactivity.
Let’s create a elementary UI the usage of all of those information. Get started by means of growing an HTML report (popup.html). This report defines the construction of your UI parts, reminiscent of textual content, headings, photographs, and buttons. Upload the next code:
Hi Global
Hi Global!
My first Chrome Extension
The code above creates a heading, paragraph, and button. The CSS and JavaScript information also are connected. Now, upload some types within the popup.css report:
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
frame {
font-family: Arial, sans-serif;
background-color: aliceblue;
padding: 20px;
}
Subsequent, within the popup.js report, upload an tournament listener to the button in order that when it’s clicked, an alert is displayed:
const sayHelloBtn = file.getElementById('sayHello');
sayHelloBtn.addEventListener('click on', async () => {
let tab = watch for chrome.tabs.question({ energetic: true });
chrome.scripting.executeScript({
goal: { tabId: tab[0].identity },
serve as: () => alert('Hi from the extension!'),
});
});
This JavaScript code retrieves the present energetic tab and makes use of the Chrome Scripting API to execute a script that shows an alert with a greeting message when the Say Hi button is clicked. This introduces elementary interactivity in your Chrome extension.
With those steps, you may have arrange a easy popup UI on your Chrome extension that comes with elementary textual content, styling, and capability.
In spite of everything, you want to permit the popup report within the manifest.json report by means of including some permissions:
{
. . . ,
"motion": {
"default_popup": "popup.html"
},
"permissions": [
"scripting",
"tabs"
],
"host_permissions": [
"http://*/*",
"https://*/*"
]
}
Within the configuration above, the default_popup
key specifies that popup.html would be the default UI when the consumer interacts with the extension. The permissions
array contains scripting
and tabs
, which might be an important for the extension to have interaction with the tabs and use the browser’s scripting options.
The host_permissions
array specifies which internet sites your extension can engage with. The patterns http://*/*
and https://*/*
point out that your extension can engage with all internet sites accessed over HTTP and HTTPS protocols.
With those settings to your manifest.json report, your Chrome extension is correctly configured to show a popup and execute scripts.
Reload your Chrome extension
With those adjustments effected to your native folder, you want to replace the unpacked folder loaded to Chrome. To try this, open the Chrome extensions web page, in finding your extension, and click on the reload icon.
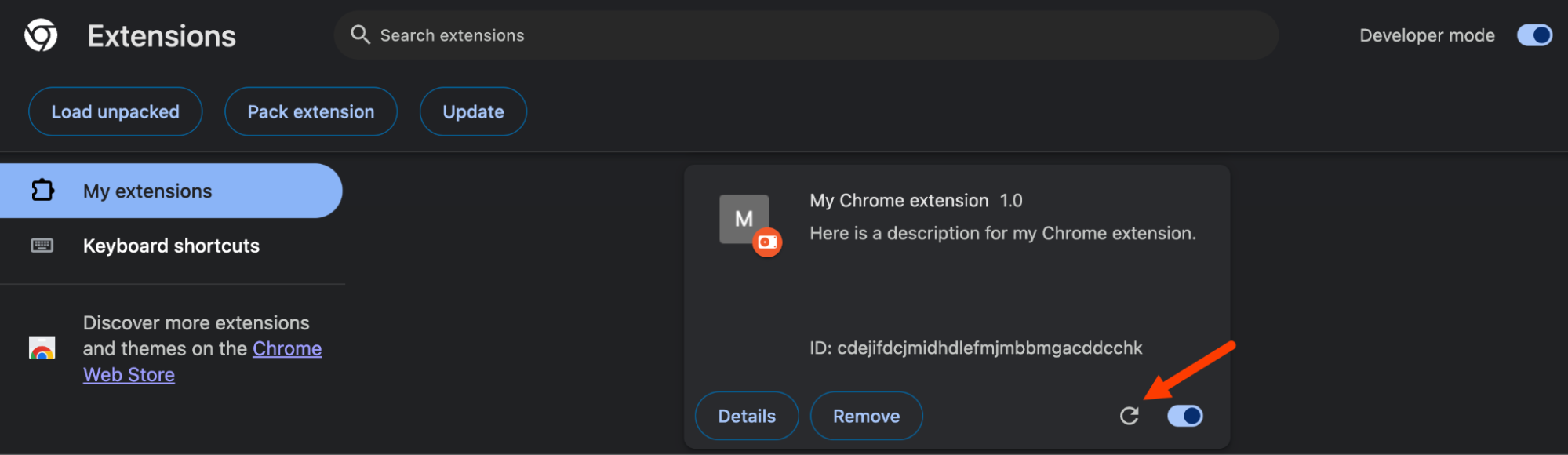
You’ll be able to then click on the extension icon, and a popup will seem. Whilst you click on the Say Hi button, an alert will seem.
You currently have a elementary wisdom of start construction a Chrome extension. There’s extra that may be accomplished. You’ll be able to manipulate your website’s UI, make API requests, retrieve information from URLs to accomplish particular operations, and extra.
Learn how to create a Chrome extension with React
As we discussed previous, making a Chrome extension is very similar to construction a internet utility. You’ll be able to use widespread internet frameworks like React.
For React, the manifest.json report is created within the public folder. This folder is used for static property you don’t want to be processed by means of Webpack (or identical bundlers that React would possibly use underneath the hood in gear like Create React App).
Whilst you construct your React utility, the construct procedure copies all contents of the public folder into the dist folder. This is create a Chrome extension with React:
- Create a brand new React utility. You’ll be able to use the native building surroundings Vite by means of working the next command to your terminal:
npm create vite@newest
Subsequent, give your challenge a reputation and make a selection React because the framework. As soon as that is accomplished, navigate into the challenge folder and set up the dependencies:
cd
npm set up
- On your React challenge’s public folder, create a manifest.json report. Upload the next configurations:
{
"manifest_version": 3,
"title": "React Chrome extension",
"description": "Chrome extension constructed with React",
"model": "0.1.0",
"motion": {
"default_popup": "index.html"
},
"permissions": [
"tabs"
],
"host_permissions": [
"http://*/*",
"https://*/*"
]
}
The configuration for a Chrome extension contains an motion
object that units index.html because the default popup when the extension icon is clicked. That is the static HTML report generated while you construct your React utility.
- Increase the React utility. Be happy to make API requests, taste them as you want, use React Hooks, and extra.
- If you find yourself accomplished construction the UI of the extension, run the construct command in React (
npm run construct
). Your entire property, together with your manifest.json report, React-generated index.html, and others, are moved into the dist or construct folder. - In spite of everything, load your extension into Chrome. Navigate to
chrome://extensions/
and reload your extension.
Making a Chrome extension to control your website’s plugins with Kinsta API
That is what the Chrome extension you’ll construct will seem like:
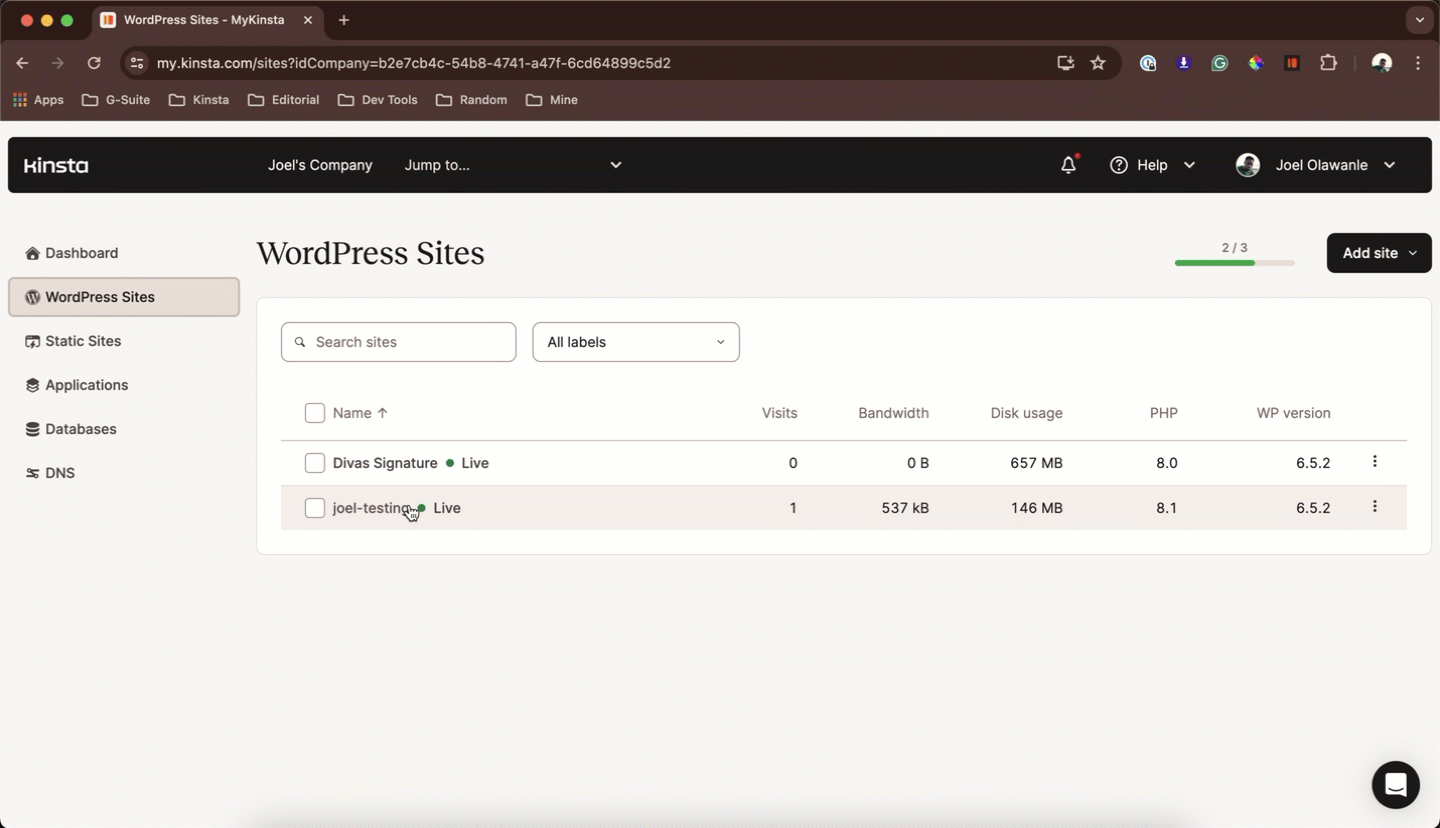
When clicked, the extension shows an inventory of websites with out of date plugins to your MyKinsta account. You’ll be able to see an inventory of the plugins and click on the View in MyKinsta button to navigate to the website’s Issues & Plugins web page, the place you’ll replace every plugin.
Let’s discover create the Chrome extension.
Working out the Kinsta API
The Kinsta API is a formidable device that lets you engage programmatically with Kinsta services and products like hosted WordPress websites. It might assist automate more than a few duties associated with WordPress control, together with website introduction, retrieving website knowledge, getting a website’s standing, surfing and restoring backups, and extra.
To make use of Kinsta’s API, you should have an account with a minimum of one WordPress website, utility, or database in MyKinsta. You should additionally generate an API key to authenticate and get entry to your account.
To generate an API key:
- Pass in your MyKinsta dashboard.
- Navigate to the API Keys web page (Your title > Corporate settings > API Keys).
- Click on Create API Key.
- Select an expiration or set a customized get started date and choice of hours for the important thing to run out.
- Give the important thing a singular title.
- Click on Generate.
After growing an API key, replica it and retailer it someplace secure (the usage of a password supervisor is beneficial). You’ll be able to generate more than one API keys, which will likely be indexed at the API Keys web page. If you want to revoke an API key, click on the Revoke button.
Set up your website’s plugins with Kinsta API and React
Let’s get started by means of growing a consumer interface in React, which is able to then be remodeled right into a Chrome extension. This information assumes elementary familiarity with React and API interplay.
Putting in place the surroundings
Originally, within the App.jsx report, outline a relentless for the Kinsta API URL to steer clear of redundancy to your code:
const KinstaAPIUrl = 'https://api.kinsta.com/v2';
For safety, retailer delicate information reminiscent of your API key and Kinsta corporate ID in a .env.native report to stay them safe and from your supply code:
VITE_KINSTA_COMPANY_ID=YOUR_COMPANY_ID
VITE_KINSTA_API_KEY=YOUR_API_KEY
Fetch information with Kinsta API
Within the App.jsx report, you want to make a number of requests to the Kinsta API to retrieve details about websites and their plugins.
- Retrieve corporate websites: Start by means of fetching an inventory of websites related together with your Kinsta corporate account. Use the corporate ID in a GET request, which returns an array of website main points.
const getListOfCompanySites = async () => { const question = new URLSearchParams({ corporate: import.meta.env.VITE_KINSTA_COMPANY_ID, }).toString(); const resp = watch for fetch(`${KinstaAPIUrl}/websites?${question}`, { approach: 'GET', headers: { Authorization: `Bearer ${import.meta.env.VITE_KINSTA_API_KEY}`, }, }); const information = watch for resp.json(); const companySites = information.corporate.websites; go back companySites; }
- Fetch surroundings information for every website: For every website, retrieve the environments, which come with the surroundings ID important for additional requests. This comes to mapping over every website and making an API name to the
/websites/${siteId}/environments
endpoint.const companySites = watch for getListOfCompanySites(); // Get all environments for every website const sitesEnvironmentData = companySites.map(async (website) => { const siteId = website.identity; const resp = watch for fetch(`${KinstaAPIUrl}/websites/${siteId}/environments`, { approach: 'GET', headers: { Authorization: `Bearer ${import.meta.env.VITE_KINSTA_API_KEY}`, }, }); const information = watch for resp.json(); const environments = information.website.environments; go back { identity: siteId, title: website.display_name, environments: environments, }; });
- Retrieve plugins for every website surroundings: In spite of everything, use the surroundings ID to fetch plugins for every website. This step comes to a mapping serve as and an API name to the
/websites/environments/${environmentId}/plugins
endpoint for every surroundings.// Look forward to all of the guarantees to get to the bottom of const sitesData = watch for Promise.all(sitesEnvironmentData); // Get all plugins for every surroundings const sitesWithPlugin = sitesData.map(async (website) => { const environmentId = website.environments[0].identity; const resp = watch for fetch( `${KinstaAPIUrl}/websites/environments/${environmentId}/plugins`, { approach: 'GET', headers: { Authorization: `Bearer ${import.meta.env.VITE_KINSTA_API_KEY}`, }, } ); const information = watch for resp.json(); const plugins = information.surroundings.container_info; go back { env_id: environmentId, title: website.title, site_id: website.identity, plugins: plugins, }; });
You’ll be able to now put all of those requests in combination right into a serve as this is used to go back the general array of websites with elementary information about every website and its plugins:
const getSitesWithPluginData = async () => { const getListOfCompanySites = async () => { const question = new URLSearchParams({ corporate: import.meta.env.VITE_KINSTA_COMPANY_ID, }).toString(); const resp = watch for fetch(`${KinstaAPIUrl}/websites?${question}`, { approach: 'GET', headers: { Authorization: `Bearer ${import.meta.env.VITE_KINSTA_API_KEY}`, }, }); const information = watch for resp.json(); const companySites = information.corporate.websites; go back companySites; } const companySites = watch for getListOfCompanySites(); // Get all environments for every website const sitesEnvironmentData = companySites.map(async (website) => { const siteId = website.identity; const resp = watch for fetch(`${KinstaAPIUrl}/websites/${siteId}/environments`, { approach: 'GET', headers: { Authorization: `Bearer ${import.meta.env.VITE_KINSTA_API_KEY}`, }, }); const information = watch for resp.json(); const environments = information.website.environments; go back { identity: siteId, title: website.display_name, environments: environments, }; }); // Look forward to all of the guarantees to get to the bottom of const sitesData = watch for Promise.all(sitesEnvironmentData); // Get all plugins for every surroundings const sitesWithPlugin = sitesData.map(async (website) => { const environmentId = website.environments[0].identity; const resp = watch for fetch( `${KinstaAPIUrl}/websites/environments/${environmentId}/plugins`, { approach: 'GET', headers: { Authorization: `Bearer ${import.meta.env.VITE_KINSTA_API_KEY}`, }, } ); const information = watch for resp.json(); const plugins = information.surroundings.container_info; go back { env_id: environmentId, title: website.title, site_id: website.identity, plugins: plugins, }; }); // Look forward to all of the guarantees to get to the bottom of const sitesWithPluginData = watch for Promise.all(sitesWithPlugin); go back sitesWithPluginData; }
Showing website information
Create a state with the useState
hook to retailer websites with out of date plugin(s). The useEffect
hook may also name the getSitesWithPluginData()
approach and extract the website main points when the element is fastened.
Within the useEffect
hook, create a serve as that can loop via every website to filter websites with out of date plugins after which retailer them within the state:
const [sitesWithOutdatedPlugin, setSitesWithOutdatedPlugin] = useState([]);
const [isLoading, setIsLoading] = useState(true);
useEffect(() => {
const checkSitesWithPluginUpdate = async () => {
const sitesWithPluginData = watch for getSitesWithPluginData();
const sitesWithOutdatedPlugin = sitesWithPluginData.map((website) => {
const plugins = website.plugins.wp_plugins.information;
const outdatedPlugins = plugins.filter out((plugin) => plugin.replace === "to be had");
if (outdatedPlugins.duration > 0) {
const kinstaDashboardPluginPageURL = `https://my.kinsta.com/websites/plugins/${website.site_id}/${website.env_id}?idCompany=${import.meta.env.VITE_KINSTA_COMPANY_ID}`;
go back {
title: website.title,
plugins: outdatedPlugins,
url: kinstaDashboardPluginPageURL,
};
}
});
setSitesWithOutdatedPlugin(sitesWithOutdatedPlugin);
checkSitesWithPluginUpdate();
setIsLoading(false);
}, []);
Within the code above, you understand that the loading state could also be created and set to true
by means of default. This will likely be used to keep an eye on how information is displayed. When all of the information is loaded, we set it to false
.
Under is a markup to render the website information and plugins inside your UI.
import { useEffect, useState } from "react"
import KinstaLogo from './property/kinsta-logo.png'
import PluginPage from './elements/PluginsPage'
serve as App() {
// load the information from the API
go back (
className="title-section">
className="info-box">
Get fast details about your website plugins that want replace.
{isLoading ? (
Loading...
) : (
<>
The next websites have plugins that want to be up to date.
{sitesWithOutdatedPlugin.map((website, index) => {
go back (
);
})}
>
)}
)
}
export default App
The code features a header with an emblem and an informational paragraph. The content material of the UI is conditionally rendered in keeping with the isLoading
state. If information remains to be loading, it shows a loading message. As soon as information is loaded, it items the information concerning the websites and any plugins requiring updates.
You’ll additionally understand an element: PluginPage
(PluginPage.jsx). This element is designed to show person websites and their plugin main points. It features a capability to toggle the visibility of the plugin main points.
import { useState } from "react"
import { FaRegEye } from "react-icons/fa";
import { FaRegEyeSlash } from "react-icons/fa";
const PluginUse = (website) => {
const [viewPlugin, setViewPlugin] = useState(false);
go back (
<>
{viewPlugin && (
{website.plugins.map((plugin, index) => {
go back (
{plugin.title}
Present Model: {plugin.model}
Newest Model: {plugin.update_version}
);
})}
)}
>
)
}
export default PluginUse
Configure the manifest report
To grow to be your consumer interface and capability right into a Chrome extension, you want to configure the manifest.json report.
Create a manifest.json report within the public folder and paste the code beneath:
{
"manifest_version": 3,
"title": "Kinsta Plugins Supervisor - Because of Kinsta API",
"description": "This extension means that you can organize your WordPress website's plugin from Kinsta's MyKinsta dashboard by way of Kinsta API.",
"model": "0.1.0",
"icons": {
"48": "kinsta-icon.png"
},
"motion": {
"default_popup": "index.html"
},
"permissions": [
"tabs"
],
"host_permissions": [
"https://my.kinsta.com/*"
]
}
You should definitely upload the icon report in your public folder.
At this level, you’ll now run the construct command (npm run construct
) so your whole property, together with your manifest.json report, React-generated index.html, and different information, are moved into the dist or construct folder.
Subsequent, navigate to chrome://extensions/
and cargo this as an unpacked extension to Chrome. Click on the Load Unpacked button and make a selection the listing you created on your extension.
Prohibit extension to precise websites
You understand that this extension works at any time. We would like it to paintings best when a consumer is navigated to the MyKinsta dashboard.
To try this, let’s regulate the App.jsx report. Create a state to retailer the energetic tab:
const [activeTab, setActiveTab] = useState(null);
Subsequent, replace the useEffect
Hook to outline and invoke the getCurrentTab
serve as:
const getCurrentTab = async () => {
const queryOptions = { energetic: true, currentWindow: true };
const
= watch for chrome.tabs.question(queryOptions);
setActiveTab(tab);
}
getCurrentTab();
The above code makes use of chrome.tabs.question
with particular question choices to make sure it retrieves best the energetic tab within the present window. As soon as the tab is retrieved, it’s set because the energetic tab inside the extension’s state.
In spite of everything, put in force a conditional rendering good judgment to your element’s go back remark. This guarantees that the plugin control UI seems best when the consumer is at the MyKinsta dashboard:
go back (
{activeTab?.url.contains('my.kinsta.com') ? (
Get fast details about your website plugins that want replace.
{isLoading ? (
Loading...
) : (
<>
The next {sitesWithPluginUpdate} websites have plugins that want to be up to date.
{sitesWithOutdatedPlugin.map((website, index) => {
go back (
);
})}
>
)}
) : (
This extension is best to be had on Kinsta Dashboard.
)}
)
After making the adjustments, rebuild your utility and reload the Chrome extension. This may occasionally follow the brand new good judgment and restrictions.
Abstract
On this article, you may have discovered the fundamentals of constructing a Chrome extension and create one with React. You’ve additionally discovered create an extension that interacts with the Kinsta API.
As a Kinsta consumer, you’ll benefit from the large possible and versatility the Kinsta API brings because it is helping you increase customized answers to control your websites, packages, and databases.
What endpoint of the Kinsta API have you ever been the usage of so much, and the way have you ever used it? Proportion with us within the remark part!
The submit Learn how to construct a Chrome extension to control your website’s plugins with Kinsta API and React seemed first on Kinsta®.
WP Hosting