Laravel is a well-liked PHP framework for construction trendy and dynamic internet programs in lately’s fast moving and ever-evolving internet construction panorama. Certainly one of its core options is Laravel Eloquent, an object-relational mapper (ORM) that permits builders to successfully carry out create, learn, replace, and delete (CRUD) operations on a database.
This instructional demonstrates the way to carry out those operations for your Laravel utility the usage of Laravel’s Eloquent ORM and the way to deploy your Laravel CRUD utility the usage of MyKinsta.
CRUD Capability in Laravel
CRUD operations are the spine of any database-driven utility. They assist you to carry out probably the most elementary and crucial database operations, similar to developing new information, studying present ones, and updating and deleting them. Those operations are a very powerful to the capability of any Laravel utility that interacts with a database.
Eloquent supplies a easy and intuitive approach to engage with the database by means of lowering the complexities of database control as a way to center of attention on construction your utility. Its integrated strategies and categories provide help to simply CRUD information within the database.
Must haves
To apply this instructional, be sure you have the next:
- XAMPP
- Composer
- A MyKinsta account
- An account on GitHub, GitLab, or Bitbucket to push your code
- Bootstrap model 5
Steps
- Set up Laravel and create a brand new utility
- Create a database
- Create a desk
- Create a controller
- Arrange the type
- Upload a direction
- Generate Blade recordsdata
- Deploy and check your CRUD utility
For steerage alongside the best way, take a look at the academic’s entire code.
Set up Laravel and Create a New Utility
Open your terminal the place you wish to have to create your Laravel utility and apply the stairs underneath.
- To put in Laravel, run:
composer international require laravel/installer
- To create a brand new Laravel utility, run:
laravel new crudposts
Create a Database
To create a brand new database in your utility:
- Get started the Apache and MySQL servers within the XAMPP regulate panel and talk over with
http://localhost/phpmyadmin
for your browser.
- Click on New at the left sidebar. You must see the next:
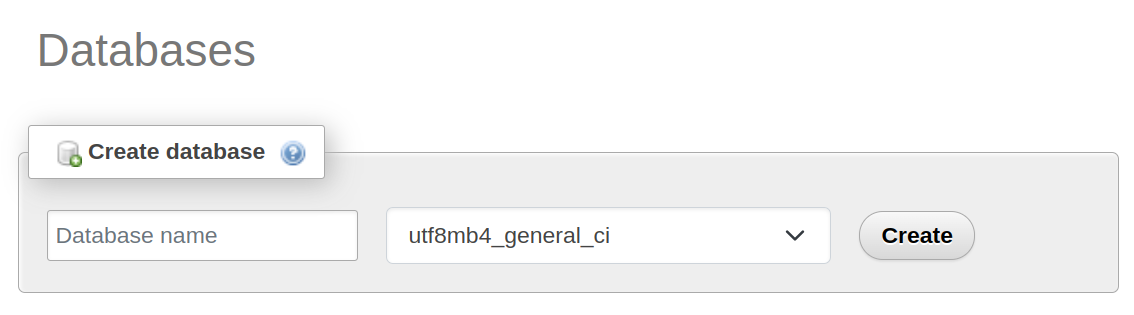
- Upload a database title and click on Create.
- Edit your utility’s .env report on the root of your Laravel utility. It comprises all of the atmosphere variables utilized by the applying. Find the variables prefixed with
DB_
and edit them along with your database credentials:
DB_CONNECTION=
DB_HOST=
DB_PORT=
DB_DATABASE=
DB_USERNAME=
DB_PASSWORD=
Create a Desk
The rows of information for your utility are saved in tables. You want only one desk for this utility, created the usage of Laravel migrations.
- To create a desk and generate a migration report the usage of Laravel’s command-line interface, Artisan, run:
php artisan make:migration create_posts_table
The command above creates a brand new report,
yyyy_mm_dd_hhmmss_create_posts_table.php, in database/migrations.
- Open yyyy_mm_dd_hhmmss_create_posts_table.php to outline the columns you wish to have inside of your database desk within the up serve as:
public serve as up()
{
Schema::create('posts', serve as (Blueprint $desk) {
$table->identification();
$table->string('identify');
$table->textual content('frame');
$table->timestamps();
});
}
This code defines what the posts desk comprises. It has 4 columns: identification
, identify
, frame
, and timestamps
.
- Run the migrations recordsdata within the database/migrations folder to create tables within the database:
php artisan migrate
The output looks as if this:
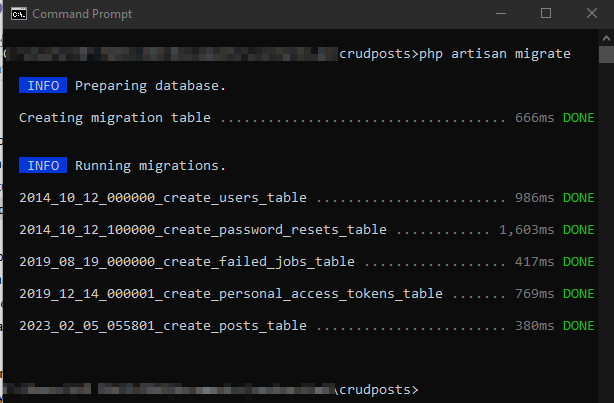
- Cross to the database you created previous to verify you’ve created the tables:

Create a Controller
The controller comprises all purposes to CRUD posts from the database.
Generate a controller report inside of your Laravel utility the usage of Artisan:
php artisan make:controller PostController --api
Operating this command creates a PostController.php report in app/Http/Controllers, with boilerplate code and empty serve as declarations index
, retailer
, display
, replace
, and smash
.
Create Purposes
Subsequent, create the purposes that retailer, index, replace, smash, create, display, and edit the information.
You’ll upload them to the report app/Http/Controller/PostController.php proven underneath.
The retailer
Serve as
The retailer
serve as provides a publish to the database.
Scroll to the retailer
serve as and upload the next code throughout the empty curly braces:
$request->validate([
'title' => 'required|max:255',
'body' => 'required',
]);
Publish::create($request->all());
go back redirect()->direction('posts.index')
->with('luck','Publish created effectively.');
This code takes an object containing the publish’s identify and frame, validates the information, provides a brand new publish to the database if the information is legitimate, and redirects the person to the homepage with a luck message.
The index
Serve as
The index
serve as fetches all of the posts from the database and sends the information to the posts.index web page.
The replace
Serve as
The <replace
serve as comprises the identification
of the publish to replace, the brand new publish identify
, and the frame
. After validating the information, it unearths the publish with the similar identification
. If discovered, the replace
serve as updates the publish within the database with the brand new identify
and frame
. Then, it redirects the person to the homepage with a luck message.
The smash
Serve as
The smash
serve as unearths a publish with the given identification
and deletes it from the database, then redirects the person to the homepage with a luck message.
The purposes above are the purposes used to CRUD posts from the database. On the other hand, you will have to outline extra purposes throughout the controller to render the vital pages in assets/perspectives/posts/.
The create
Serve as
The create
serve as renders the assets/perspectives/posts/create.blade.php web page, which comprises the shape for including posts to the database.
The display
Serve as
The display
serve as unearths a publish with the given identification
within the database and renders the assets/perspectives/posts/display.blade.php report with the publish.
The edit
Serve as
The edit
serve as unearths a publish with the given identification
within the database and renders the assets/perspectives/posts/edit.blade.php report with the publish main points inside of a kind.
Set Up the Style
The Publish
type interacts with the posts desk within the database.
- To create a type with Artisan, run:
php artisan make:type Publish
This code creates a Publish.php report throughout the App/Fashions folder.
- Create a
array. Upload the next code throughout the Publish
magnificence and underneath theuse HasFactory;
line
secure $fillable = [
'title',
'body',
];
This code creates a fillable
array that permits you to upload pieces to the database out of your Laravel utility.
- Attach the
Publish
type to the PostController.php report. Open PostController.php and upload the road underneath underneathuse IlluminateHttpRequest;
. It looks as if:
use IlluminateHttpRequest;
use AppModelsPost;
The PostController.php report must now appear to be this:
'required',
]);
Publish::create($request->all());
go back redirect()->direction('posts.index')
->with('luck', 'Publish created effectively.');
/**
* Replace the required useful resource in garage.
*
* @param IlluminateHttpRequest $request
* @param int $identification
* @go back IlluminateHttpResponse
*/
public serve as replace(Request $request, $identification)
max:255',
'body' => 'required',
]);
$publish = Publish::in finding($identification);
$post->replace($request->all());
go back redirect()->direction('posts.index')
->with('luck', 'Publish up to date effectively.');
/**
* Take away the required useful resource from garage.
*
* @param int $identification
* @go back IlluminateHttpResponse
*/
public serve as smash($identification)
{
$publish = Publish::in finding($identification);
$post->delete();
go back redirect()->direction('posts.index')
->with('luck', 'Publish deleted effectively');
}
// routes purposes
/**
* Display the shape for developing a brand new publish.
*
* @go back IlluminateHttpResponse
*/
public serve as create()
{
go back view('posts.create');
}
/**
* Show the required useful resource.
*
* @param int $identification
* @go back IlluminateHttpResponse
*/
public serve as display($identification)
{
$publish = Publish::in finding($identification);
go back view('posts.display', compact('publish'));
}
/**
* Display the shape for modifying the required publish.
*
* @param int $identification
* @go back IlluminateHttpResponse
*/
public serve as edit($identification)
{
$publish = Publish::in finding($identification);
go back view('posts.edit', compact('publish'));
}
}
Upload Routes
After developing the controller purposes and the Publish
type, you will have to upload routes in your controller purposes.
- Open routes/internet.php and delete the boilerplate direction that the applying generated. Exchange it with the code underneath to glue the controller purposes to their respective routes:
// returns the house web page with all posts
Path::get('/', PostController::magnificence .'@index')->title('posts.index');
// returns the shape for including a publish
Path::get('/posts/create', PostController::magnificence . '@create')->title('posts.create');
// provides a publish to the database
Path::publish('/posts', PostController::magnificence .'@retailer')->title('posts.retailer');
// returns a web page that presentations a complete publish
Path::get('/posts/{publish}', PostController::magnificence .'@display')->title('posts.display');
// returns the shape for modifying a publish
Path::get('/posts/{publish}/edit', PostController::magnificence .'@edit')->title('posts.edit');
// updates a publish
Path::put('/posts/{publish}', PostController::magnificence .'@replace')->title('posts.replace');
// deletes a publish
Path::delete('/posts/{publish}', PostController::magnificence .'@smash')->title('posts.smash');
- To glue the routes, open app/Http/Controllers/PostController.php and upload the road underneath underneath the road
use IlluminateSupportFacadesRoute;
:
use IlluminateSupportFacadesRoute;
use AppHttpControllersPostController;
The routes/internet.php report must now appear to be this:
title('posts.index');
// returns the shape for including a publish
Path::get('/posts/create', PostController::magnificence . '@create')->title('posts.create');
// provides a publish to the database
Path::publish('/posts', PostController::magnificence .'@retailer')->title('posts.retailer');
// returns a web page that presentations a complete publish
Path::get('/posts/{publish}', PostController::magnificence .'@display')->title('posts.display');
// returns the shape for modifying a publish
Path::get('/posts/{publish}/edit', PostController::magnificence .'@edit')->title('posts.edit');
// updates a publish
Path::put('/posts/{publish}', PostController::magnificence .'@replace')->title('posts.replace');
// deletes a publish
Path::delete('/posts/{publish}', PostController::magnificence .'@smash')->title('posts.smash');
Generate Blade Information
Now that you've got the routes, you'll be able to create the Laravel Blade recordsdata. Prior to the usage of Artisan to generate the Blade recordsdata, create the make:view
command, with which you'll be able to generate blade.php recordsdata.
- Run the next code within the CLI to create a MakeViewCommand command report throughout the app/Console/Instructions folder:
php artisan make:command MakeViewCommand
- Create a command for producing .blade.php recordsdata from the CLI by means of changing the code within the MakeViewCommand report with the next:
argument('view');
$trail = $this->viewPath($view);
$this->createDir($trail);
if (Document::exists($trail))
{
$this->error("Document {$trail} already exists!");
go back;
}
Document::put($trail, $trail);
$this->data("Document {$trail} created.");
}
/**
* Get the view complete trail.
*
* @param string $view
*
* @go back string
*/
public serve as viewPath($view)
{
$view = str_replace('.', '/', $view) . '.blade.php';
$trail = "assets/perspectives/{$view}";
go back $trail;
}
/**
* Create a view listing if it does no longer exist.
*
* @param $trail
*/
public serve as createDir($trail)
{
$dir = dirname($trail);
if (!file_exists($dir))
{
mkdir($dir, 0777, true);
}
}
}
Create a Homepage
Subsequent, create your homepage. The homepage is the index.blade.php report, which lists all of the posts.
- To create the homepage, run:
php artisan make:view posts.index
This creates a posts folder throughout the /assets/perspectives folder and an index.blade.php report beneath it. The ensuing trail is /assets/perspectives/posts/index.blade.php.
- Upload the next code throughout the index.blade.php report:
Posts
@foreach ($posts as $publish)
{{ $post->identify }}
{{ $post->frame }}
@endforeach
The code above creates a easy HTML web page that makes use of Bootstrap for styling. It establishes a navigation bar and a grid template that lists all of the posts within the database with main points and two motion buttons — edit and delete — the usage of the @foreach
Blade helper.
The Edit button takes a person to the Edit publish web page, the place they are able to edit the publish. The Delete button deletes the publish from the database the usage of {{ direction('posts.smash', $post->identification) }}
with a DELETE
means.
Notice: The navbar code for all of the recordsdata is equal to the former report.
- Create the create.blade.php web page. The Blade report known as create provides posts to the database. Use the next command to generate the report:
php artisan make:view posts.create
This generates a create.blade.php report throughout the /assets/perspectives/posts folder.
- Upload the next code to the create.blade.php report:
// identical as the former report. Upload the next after the nav tag and sooner than the final frame tag.
Upload a Publish
The code above creates a kind with identify
and frame
fields and a post
button for including a publish to the database during the {{ direction('posts.retailer') }}
motion with a POST
means.
- Create the Edit publish web page to edit posts within the database. Use the next command to generate the report:
php artisan make:view posts.edit
This creates an edit.blade.php report throughout the /assets/perspectives/posts folder.
- Upload the next code to the edit.blade.php report:
Replace Publish
The code above creates a kind with identify
and frame
fields and a post button for modifying a publish with the required identification
within the database during the {{ direction('posts.replace') }}
motion with a PUT
means.
- Then, restart your utility server the usage of the code underneath:
php artisan serve
Talk over with http://127.0.0.1:8000
for your browser to view your new weblog. Click on the Upload Publish button so as to add new posts.
Deploy and Take a look at Your CRUD Utility
Get ready your utility for deployment as follows.
- Make the deployment clean and simple by means of mentioning the general public folder. Upload a .htaccess report to the basis of the applying folder with the next code:
RewriteEngine On
RewriteRule ^(.*)$ public/\ [L]
- Power your app to make use of
HTTPS
by means of including the next code above your routes throughout the routes/internet.php report:
use IlluminateSupportFacadesURL;
URL::forceScheme('https');
- Push your code to a Git repository. Kinsta helps deployments from GitHub, GitLab, or Bitbucket.
Set Up a Challenge on MyKinsta
- Create a MyKinsta account if you happen to don’t have one already.
- Log in on your account and click on the Upload Provider button at the Dashboard to create a brand new utility.
- In the event you’re new to the app, attach on your GitHub, GitLab, or Bitbucket account and provides particular permissions.
- Fill out the shape, and upload the
APP_KEY
. You'll in finding its corresponding worth for your .env report.
- Choose your construct assets and whether or not you wish to have to make use of your utility construct trail or construct your utility with Dockerfile. For this demonstration, let MyKinsta construct the app in response to your repository.
- Specify the other processes you wish to have to run all over deployment. You'll depart it clean at this level.
- Finally, upload your fee means.
After confirming your fee means, MyKinsta deploys your utility and assigns you a URL as proven underneath:
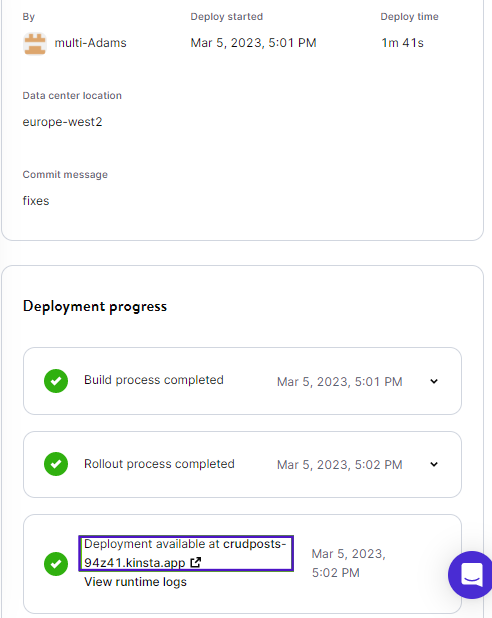
You'll talk over with the hyperlink, however you get a 500 | Server Error
web page for the reason that app wishes a legitimate database connection to paintings. The next segment resolves this drawback.
Create a Database by means of MyKinsta
- To create a database, move on your MyKinsta dashboard and click on Upload Provider.
- Choose Database and entire the shape along with your most popular database title, kind, username, and password. Upload an information heart location and a database dimension that matches your utility.
- The following web page presentations the price abstract and your fee means. Click on Create Database to finish the method.
- After developing the database, MyKinsta redirects you on your services and products checklist. Click on the database you simply created and scroll right down to Exterior Connections. Reproduction the database credentials.
- Return to the applying’s Deployment web page and click on Settings. Then, scroll right down to Atmosphere Variables and click on Upload Atmosphere Variables. Upload the database credentials as atmosphere variables within the following order:
DB_CONNECTION=mysql
DB_HOST=Exterior hostname
DB_PORT=Exterior port
DB_DATABASE=Database title
DB_USERNAME=Username
DB_PASSWORD=Password
The applying atmosphere variables checklist must now appear to be this:
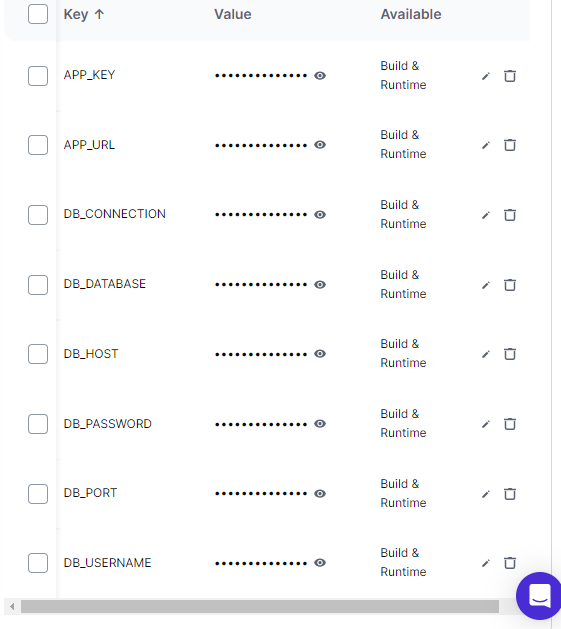
- Cross on your utility’s Deployments web page and manually deploy your utility by means of clicking Deploy Now to use those adjustments. To this point, you’ve created a database and attached it on your utility.
- In the end, to create the database tables for your MyKinsta database, attach the database on your native app by means of updating your .env report with the similar credentials you entered for your app at MyKinsta and run the next command:
php artisan migrate
This command runs all of the migration recordsdata. It creates all of the outlined tables for your MyKinsta utility.
Now, you'll be able to check your utility with the URL assigned after the primary deployment.
Abstract
Laravel is a complete framework for developing powerful and scalable internet programs that require CRUD capability. With its intuitive syntax and strong options, Laravel makes it simple to construct CRUD operations into your utility.
This text lined the basic ideas of CRUD operations and the way to put into effect them the usage of Laravel’s integrated options. It additionally defined:
- Tips on how to create a database in MyKinsta and attach it on your utility
- Tips on how to use Laravel’s migrations to outline the database desk, create the controller report and its purposes
- Outline a type and attach it to the controller. Laravel’s routing generates Blade recordsdata to create corresponding pages and bureaucracy and to deploy and check the applying the usage of MyKinsta
Now that you just’ve noticed how simple it's to accomplish CRUD operations in Laravel, take a look at MyKinsta for internet utility construction and webhosting.
The publish How To CRUD (Create, Learn, Replace, and Delete) With Laravel seemed first on Kinsta®.
WP Hosting