Within the Gutenberg generation, the design procedure isn’t strictly tied to WordPress topics. Out of the field, the CMS supplies customers with the entire design equipment had to construct an ideal web site format and the theme objectives to be one thing that provides much more development and design equipment.
Block templates are a characteristic that unlocks much more powers in web site development. In line with the Block Editor Guide:
A block template is outlined as an inventory of block pieces. Such blocks could have predefined attributes, placeholder content material, and be static or dynamic. Block templates permit specifying a default preliminary state for an editor consultation.
In different phrases, block templates are pre-built collections of blocks used to set a default state dynamically at the shopper.
Template information are PHP information similar to index.php, web page.php, and unmarried.php, and paintings the similar approach with each vintage and block topics, in keeping with the WordPress template hierarchy. In vintage topics, those information are written in PHP and HTML. In block topics, they’re fully made from blocks.
Block patterns wish to be manually added for your pages whilst block templates robotically give you the preliminary format and defaults while you or your workforce contributors create a brand new submit.
You’ll additionally bind explicit block templates for your customized submit sorts and lock some blocks or options to pressure customers to make use of your defaults or save you mistakes.
You will have a few tactics to create block templates. You’ll use the block API to claim an array of block sorts by the use of PHP, or you’ll create a customized block kind the use of the InnerBlocks
part.
Let’s dive in!
How To Construct a Block Template The usage of PHP
In case you’re an old-school developer, you’ll outline a customized block template the use of a plugin or your theme’s purposes.php. If you make a decision to head with a plugin, release your favourite code editor, create a brand new PHP report, and upload the next code:
template = array(
array( 'core/symbol' ),
array( 'core/heading' ),
array( 'core/paragraph' )
);
}
add_action( 'init', 'myplugin_register_my_block_template' );
Within the code above, get_post_type_object
retrieves a submit kind via title.
Save your report within the wp-content/plugins folder, navigate to the Plugins display to your WordPress dashboard, and turn on the My Block Templates plugin.
Now, while you create a brand new submit, the editor robotically launches your block template with a picture block, a heading, and a paragraph.
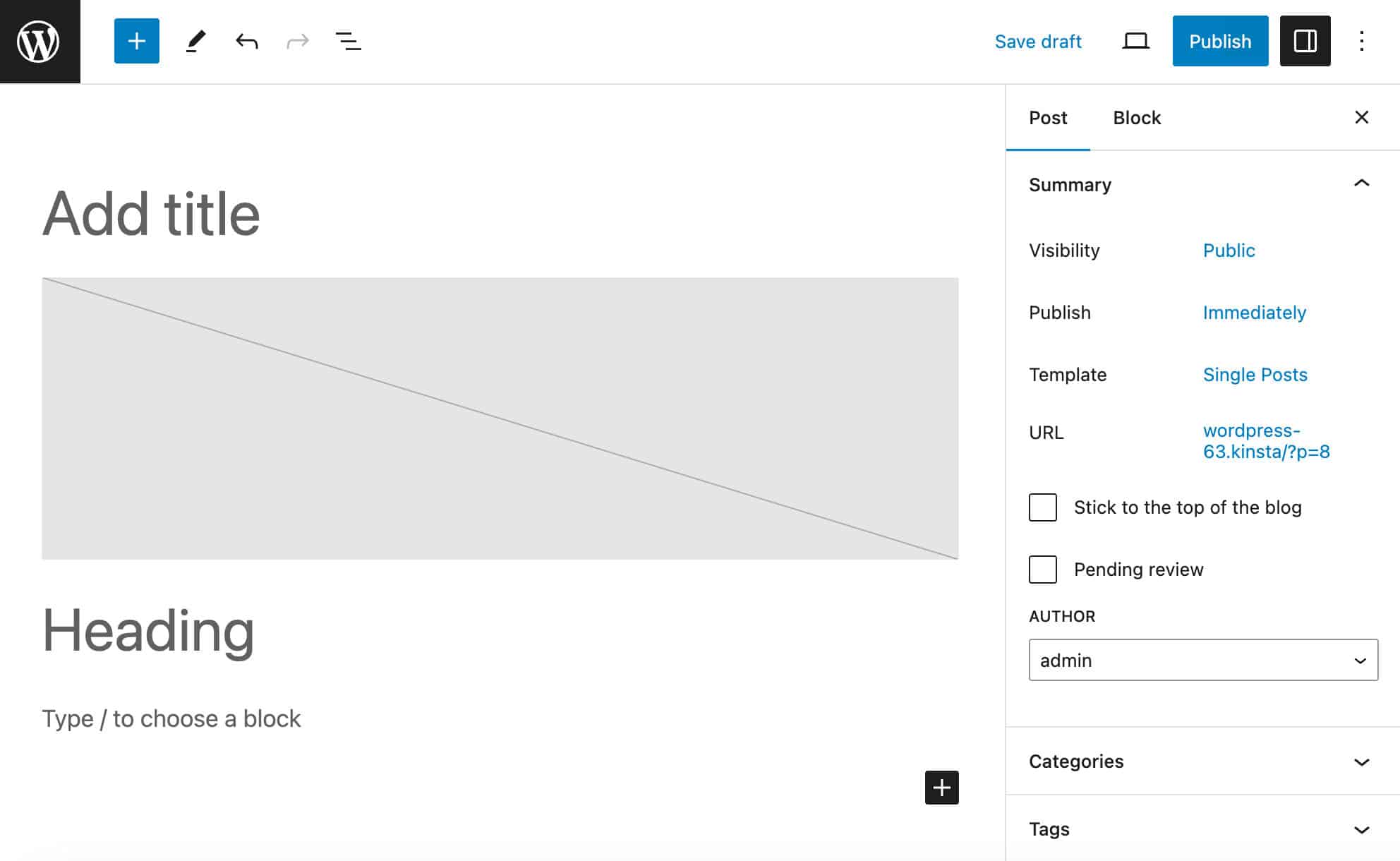
You’ll additionally upload an array of settings for every block and in addition create nested constructions of blocks. The next serve as builds a extra complex block template with internal blocks and settings:
serve as myplugin_register_my_block_template() {
$block_template = array(
array( 'core/symbol' ),
array( 'core/heading', array(
'placeholder' => 'Upload H2...',
'stage' => 2
) ),
array( 'core/paragraph', array(
'placeholder' => 'Upload paragraph...'
) ),
array( 'core/columns',
array(),
array(
array( 'core/column',
array(),
array(
array( 'core/symbol' )
)
),
array( 'core/column',
array(),
array(
array( 'core/heading', array(
'placeholder' => 'Upload H3...',
'stage' => 3
) ),
array( 'core/paragraph', array(
'placeholder' => 'Upload paragraph...'
) )
)
)
)
)
);
$post_type_object = get_post_type_object( 'submit' );
$post_type_object->template = $block_template;
}
add_action( 'init', 'myplugin_register_my_block_template' );
You’ll see the output of the code above within the following symbol:
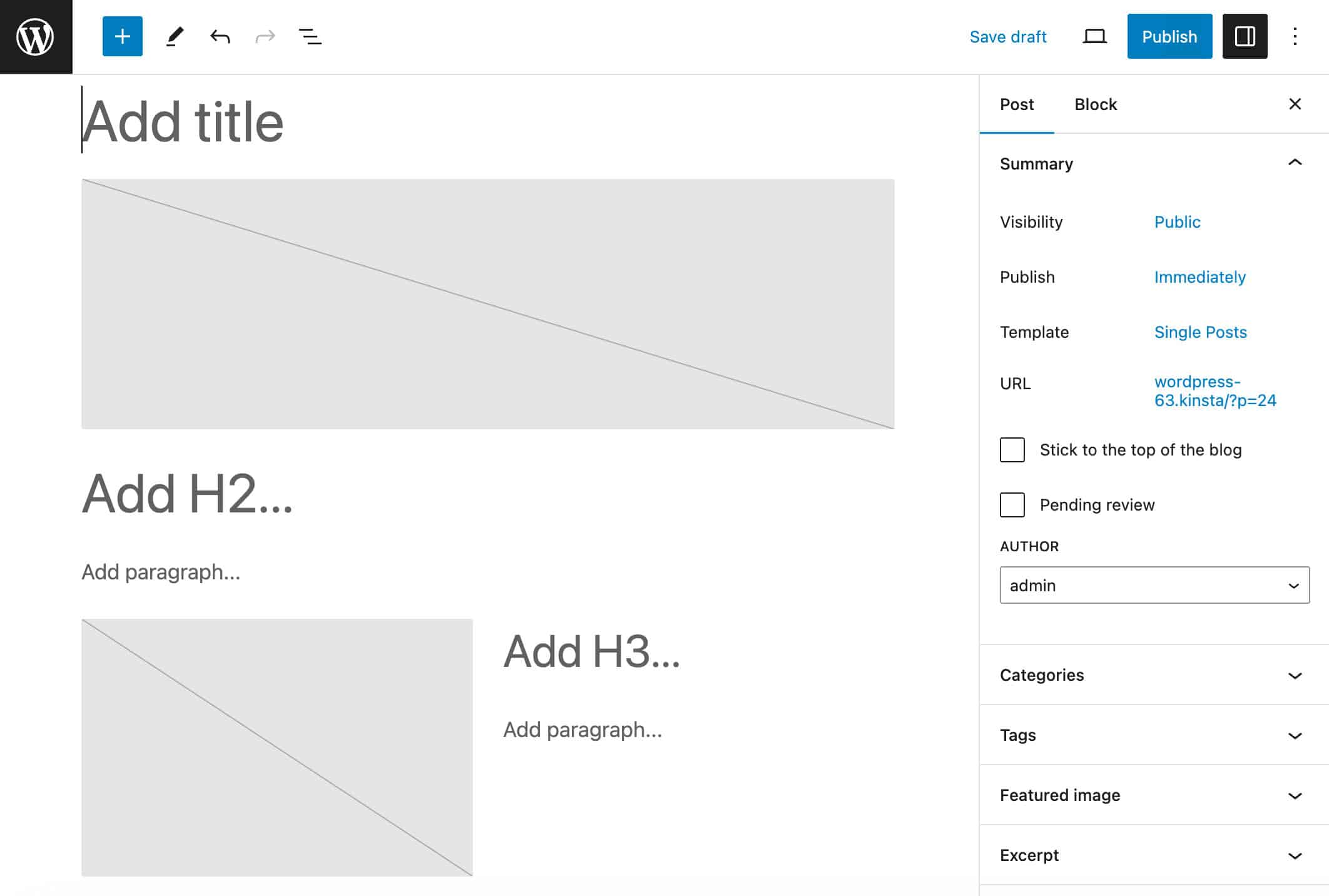
Thus far, we have now simplest used core blocks. However you’ll additionally come with customized blocks or block patterns to your block templates, as proven within the following instance:
serve as myplugin_register_my_block_template() {
$post_type_object = get_post_type_object( 'web page' );
$post_type_object->template = array(
array( 'core/sample', array(
'slug' => 'my-plugin/my-block-pattern'
) )
);
}
add_action( 'init', 'myplugin_register_my_block_template' );
There’s now not a lot distinction in case you make a decision to create a default block template for an already registered customized submit kind. Merely exchange the submit form of get_post_type_object
for your customized submit kind title, as proven within the following instance:
template = array(
array( 'core/symbol' ),
array( 'core/heading' ),
array( 'core/paragraph' )
);
}
add_action( 'init', 'myplugin_register_my_block_template' );
Now that you know the way to create block templates, we will transfer ahead and discover extra use instances. Let’s dive a little bit deeper.
Block Templates With Customized Put up Varieties
As we discussed previous, you’ll connect a block template to a customized submit kind. You’ll do this after your customized submit kind has already been registered, however you might like to outline a block template on customized submit kind registration.
On this case, you’ll use the template
and template_lock
arguments of the register_post_type
serve as:
serve as myplugin_register_book_post_type() {
$args = array(
'label' => esc_html__( 'Books' ),
'labels' => array(
'title' => esc_html__( 'Books' ),
'singular_name' => esc_html__( 'E book' ),
),
'public' => true,
'publicly_queryable' => true,
'show_ui' => true,
'show_in_rest' => true,
'rest_namespace' => 'wp/v2',
'has_archive' => true,
'show_in_menu' => true,
'show_in_nav_menus' => true,
'helps' => array( 'name', 'editor', 'thumbnail' ),
'template' => array(
array( 'core/paragraph', array(
'placeholder' => 'Upload paragraph...'
) ),
array( 'core/columns',
array(),
array(
array( 'core/column',
array(),
array(
array( 'core/symbol' )
)
),
array( 'core/column',
array(),
array(
array( 'core/heading', array(
'placeholder' => 'Upload H3...',
'stage' => 3
) ),
array( 'core/paragraph', array(
'placeholder' => 'Upload paragraph...'
) )
)
)
)
)
)
);
register_post_type( 'ebook', $args );
}
add_action( 'init', 'myplugin_register_book_post_type' );
And that’s it. The picture beneath displays the block template within the editor’s interface for a E book customized submit kind.
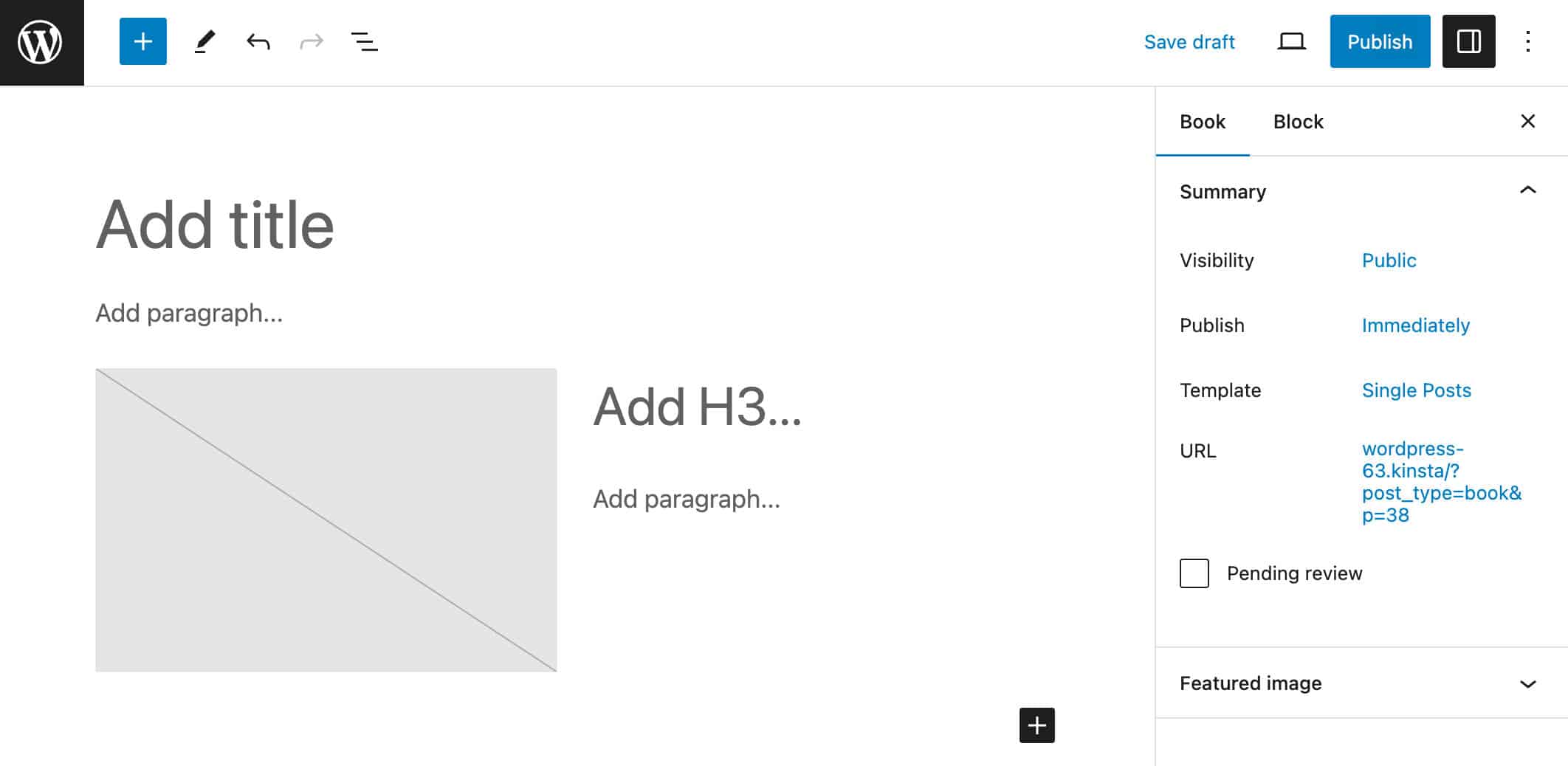
When you find yourself completed with the format, you might need to mess around with block settings to fine-tune the habits and look of your block template.
Tremendous Tuning the Block Template With Block Attributes
We outlined a block template as an inventory of blocks. Every merchandise within the listing must be an array containing the block title and an array of non-compulsory attributes. With nested arrays, you might need to upload a 3rd array for kids blocks.
A template with a Columns block can also be represented as follows:
$template = array( 'core/columns',
// attributes
array(),
// nested blocks
array(
array( 'core/column' ),
array( 'core/column' )
)
);
As discussed above, the second one array within the listing is an non-compulsory array of block attributes. Those attributes can help you customise the illusion of your template in order that you or your customers can focal point at the submit content material with out worrying in regards to the web page format and design.
To start out, you’ll use the block editor to create a construction of blocks you’ll use as a reference on your template.
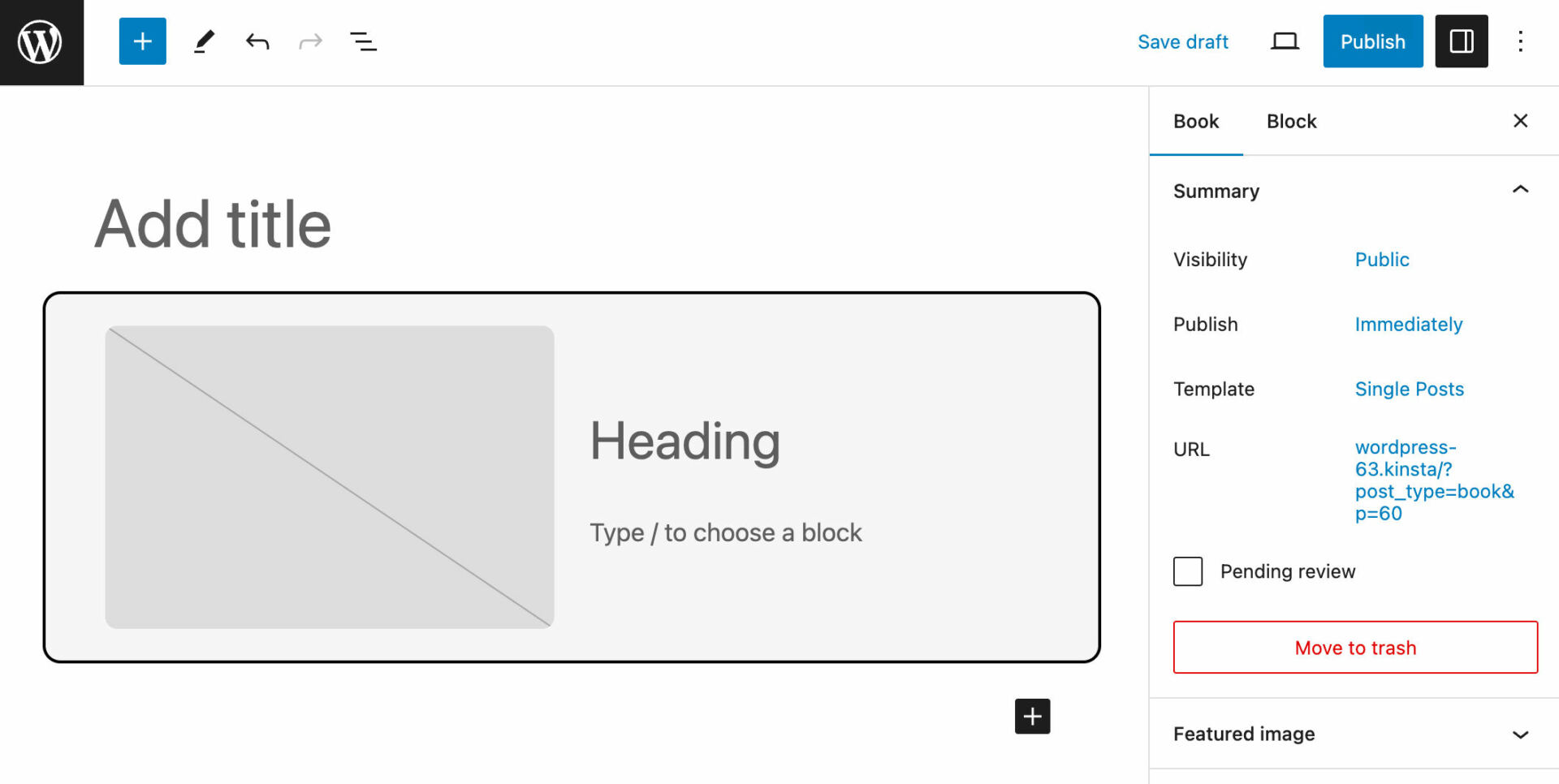
Upload your blocks, customise the format and kinds, then transfer to the code editor and in finding block delimiters.

Block delimiters retailer block settings and kinds in key/worth pairs. You’ll merely replica and paste keys and values from the block markup to populate your array of attributes:
$template = array( 'core/columns',
array(
'verticalAlignment' => 'middle',
'align' => 'broad',
'taste' => array(
'border' => array(
'width' => '2px',
'radius' => array(
'topLeft' => '12px',
'topRight' => '12px',
'bottomLeft' => '12px',
'bottomRight' => '12px'
)
)
),
'backgroundColor' => 'tertiary'
),
array(
array( 'core/column' ),
array( 'core/column' )
)
);
Repeat the method for every block within the template and you might be achieved.
$template = array(
array( 'core/paragraph', array(
'placeholder' => 'Upload paragraph...'
) ),
array( 'core/columns',
array(
'verticalAlignment' => 'middle',
'align' => 'broad',
'taste' => array(
'border' => array(
'width' => '2px',
'radius' => array(
'topLeft' => '12px',
'topRight' => '12px',
'bottomLeft' => '12px',
'bottomRight' => '12px'
)
)
),
'backgroundColor' => 'tertiary',
'lock' => array(
'take away' => true,
'transfer' => true
)
),
array(
array( 'core/column',
array( 'verticalAlignment' => 'middle' ),
array(
array( 'core/symbol',
array(
'taste' => array( 'border' => array( 'radius' => '8px' ) )
)
)
)
),
array( 'core/column',
array( 'verticalAlignment' => 'middle' ),
array(
array( 'core/heading', array(
'placeholder' => 'Upload H3...',
'stage' => 3
) ),
array( 'core/paragraph', array(
'placeholder' => 'Upload paragraph...'
) )
)
)
)
)
);
Locking Blocks
You’ll lock explicit blocks or the entire blocks integrated to your template the use of the template_lock
assets of the $post_type_object
.
Locking templates might be tremendous helpful in case you have a multi-author weblog and need to save you all or explicit customers from converting the format of your block template.
Within the following instance, we’re locking all blocks within the block template:
serve as myplugin_register_my_block_template() {
$post_type_object = get_post_type_object( 'submit' );
$post_type_object->template = array(
array( 'core/symbol' ),
array( 'core/heading' ),
array( 'core/paragraph' )
);
$post_type_object->template_lock = 'all';
}
add_action( 'init', 'myplugin_register_my_block_template' );
Locked blocks display a lock icon within the block toolbar and listing view:
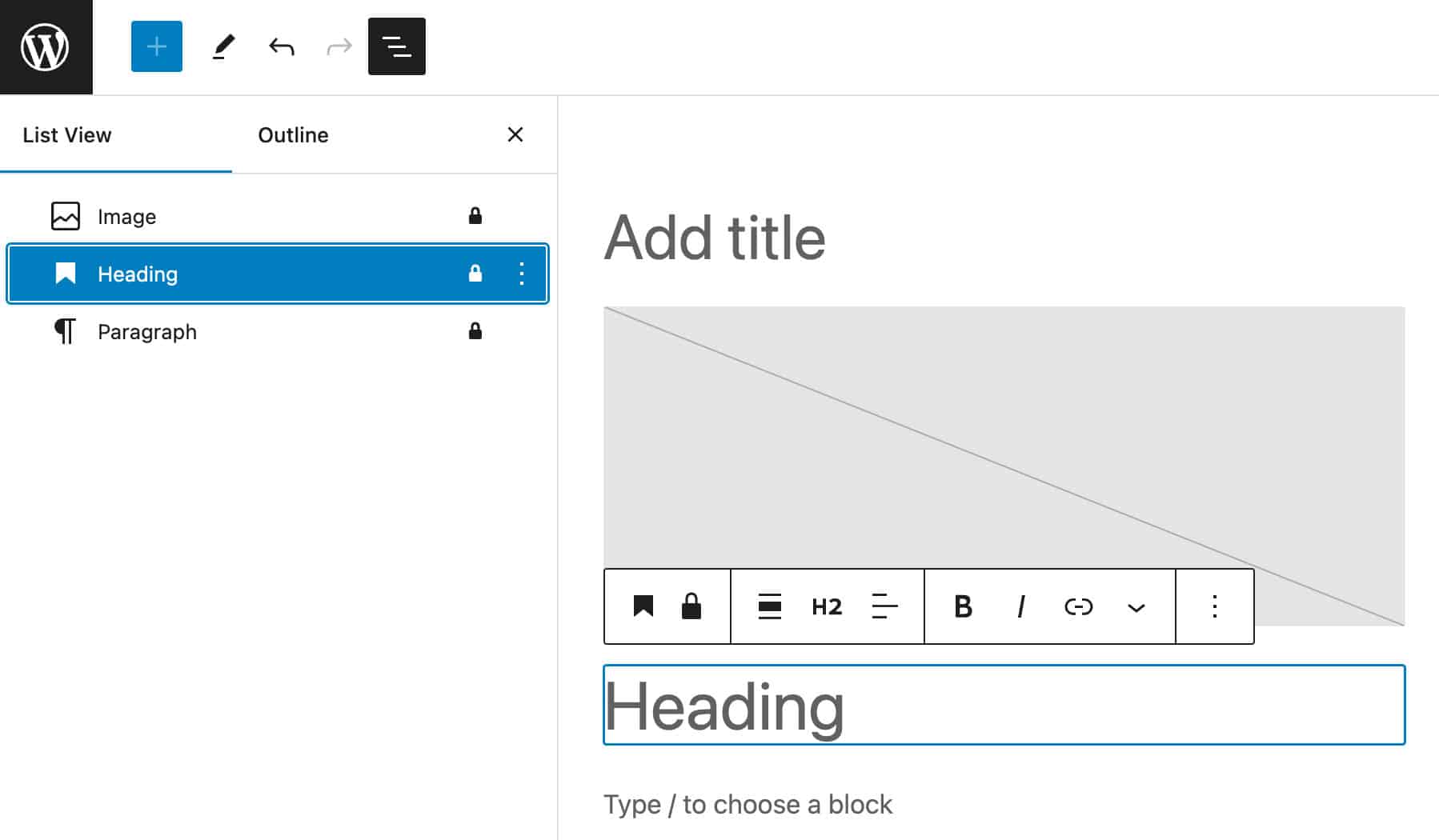
Customers can release blocks from the Choices menu to be had within the block toolbar.
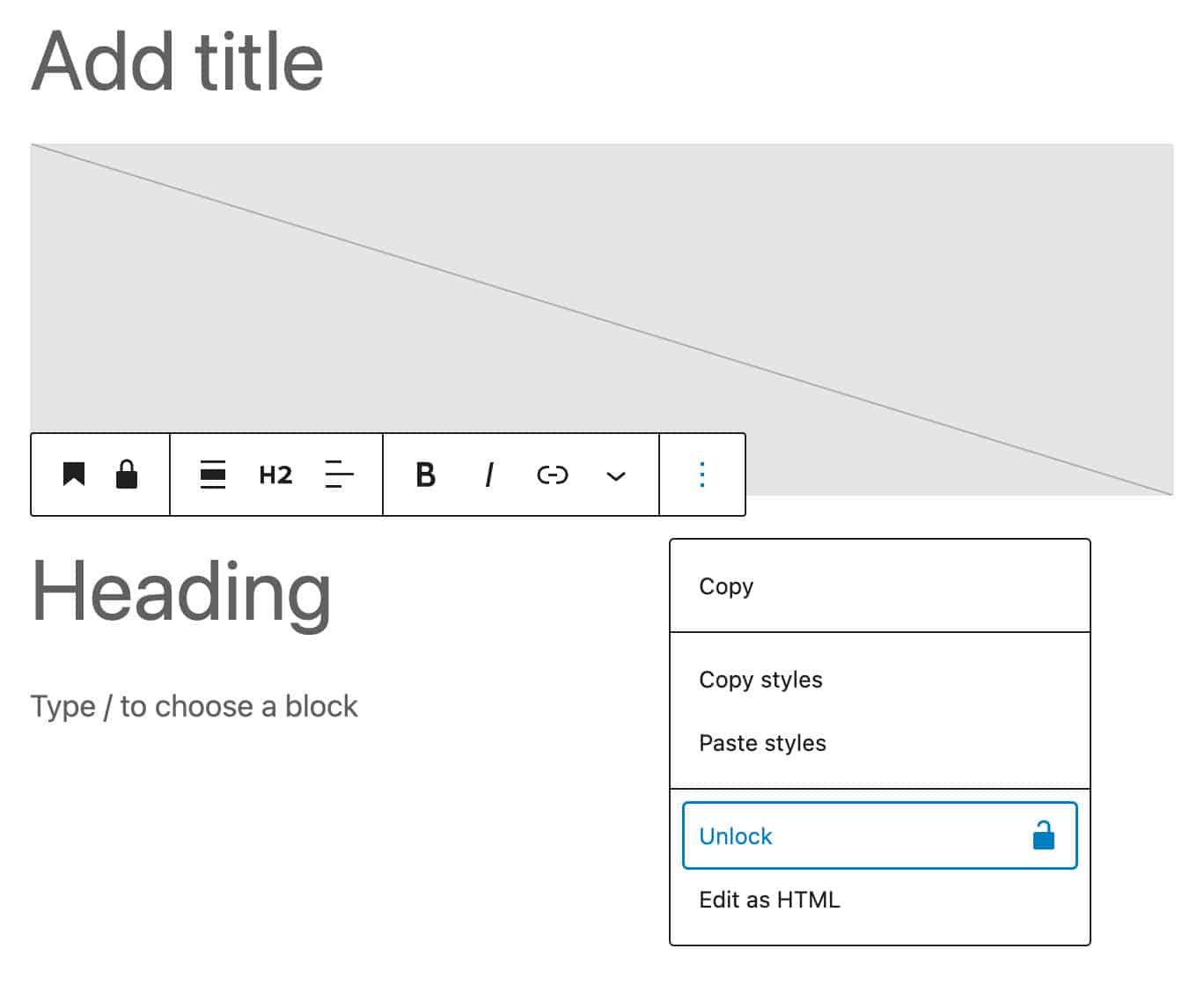
While you click on on Release, a modal popup lets you permit/disable motion, save you elimination, or each:
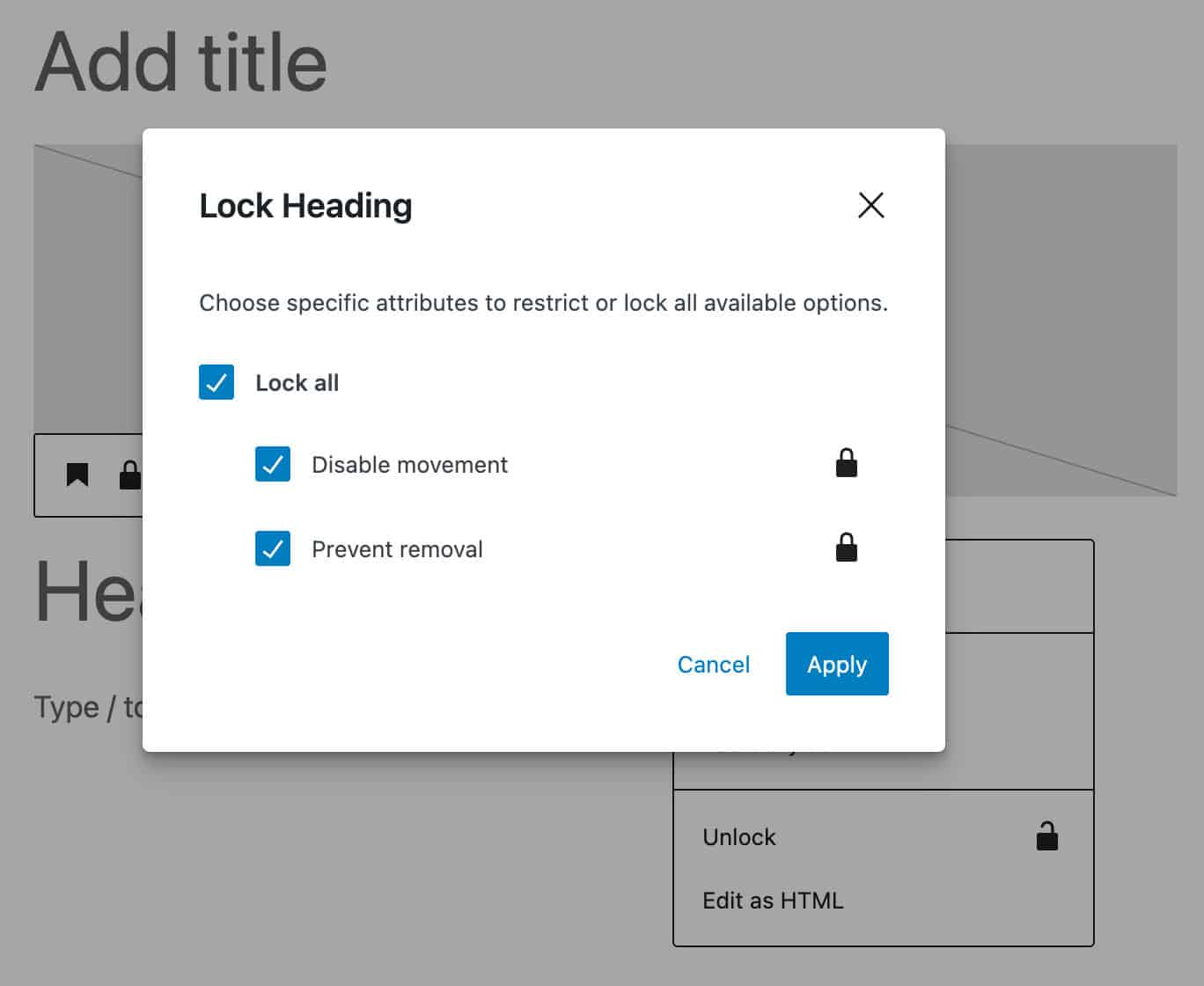
template_lock
can take one of the crucial following values:
all
– Prevents customers from including new blocks, shifting and disposing of present blocksinsert
– Prevents customers from including new blocks and disposing of present blockscontentOnly
– Customers can simplest edit the content material of the blocks integrated within the template. Word thatcontentOnly
can simplest be used on the sample or template stage and will have to be controlled with code. (See additionally Locking APIs).
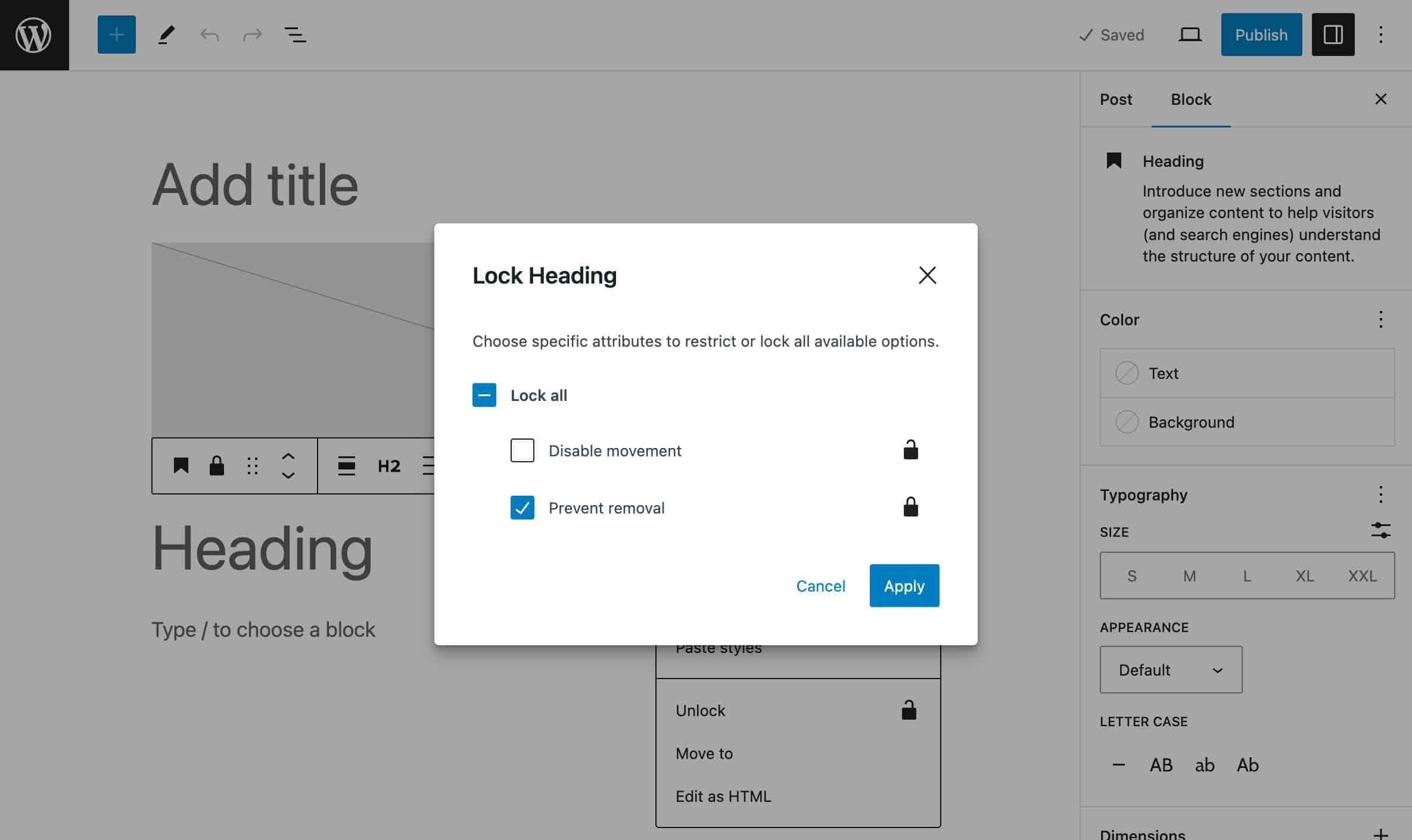
If you wish to lock explicit blocks, you’ll use the lock
characteristic on every block:
serve as myplugin_register_my_block_template() {
$post_type_object = get_post_type_object( 'submit' );
$post_type_object->template = array(
array( 'core/symbol' ),
array( 'core/heading' ),
array( 'core/paragraph', array(
'lock' => array(
'take away' => true,
'transfer' => true
)
) )
);
}
add_action( 'init', 'myplugin_register_my_block_template' );
The lock
characteristic can take one of the crucial following values:
take away
: Prevents customers from disposing of a block.transfer
: Prevents customers from shifting a block.
And you’ll additionally use lock
together with template_lock
to fine-tune the habits of the blocks integrated to your block template. Within the following instance, we’re locking all blocks however the heading:
serve as myplugin_register_my_block_template() {
$post_type_object = get_post_type_object( 'submit' );
$post_type_object->template = array(
array( 'core/symbol' ),
array( 'core/heading', array(
'lock' => array(
'take away' => false,
'transfer' => false
)
) ),
array( 'core/paragraph' )
);
$post_type_object->template_lock = 'all';
}
add_action( 'init', 'myplugin_register_my_block_template' );
The picture beneath displays the block template with locked and unlocked blocks:
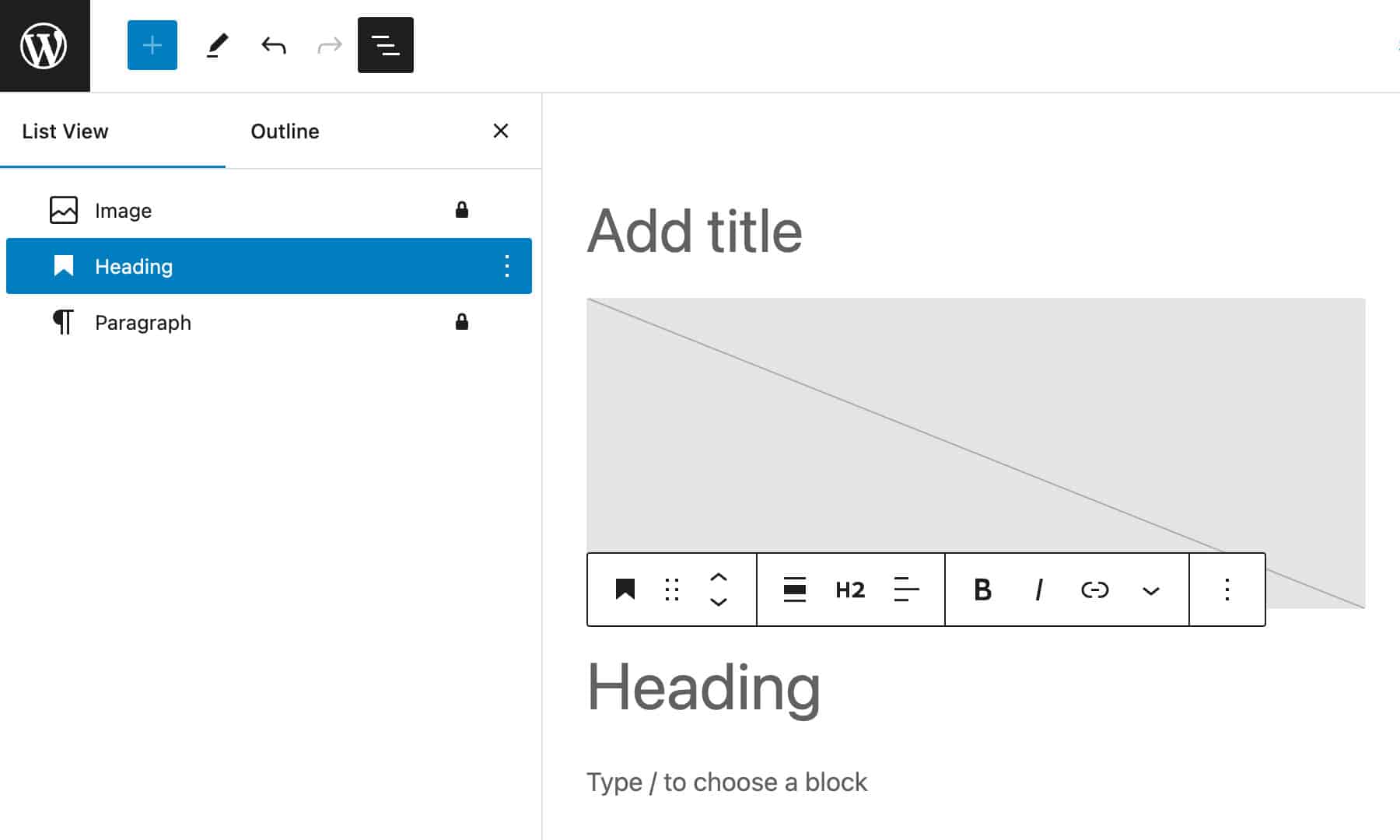
Block builders too can use the lock
characteristic within the block definition at the attributes
stage (see additionally Person block locking).
Fighting Customers From Unlocking Blocks
In case you examined the code mentioned to this point within the article, you’ll have learned that you’ll release the blocks integrated to your template (or every other block) from the editor’s interface. Through default, all customers with modifying get admission to can lock or release blocks, and doing so bypass your template settings.
You’ll keep an eye on whether or not blocks can also be locked or unlocked the use of the block_editor_settings_all
filter out.
The filter out will ship any atmosphere to the initialized Editor, this means that any editor atmosphere this is used to configure the editor at initialization can also be filtered via a PHP WordPress plugin ahead of being despatched.
The callback serve as you’ll use with this filter out takes two parameters:
$settings
: An array of editor settings.$context
: An example of the categoryWP_Block_Editor_Context
, an object that accommodates details about a block editor being rendered.
What it’s important to do is to filter out $settings['canLockBlocks']
atmosphere it to true
or false
as proven within the following instance:
add_filter( 'block_editor_settings_all',
serve as( $settings, $context ) {
if ( $context->submit && 'ebook' === $context->post->post_type ) {
$settings['canLockBlocks'] = false;
}
go back $settings;
}, 10, 2
);
You’ll exclude explicit consumer roles from locking/unlocking blocks via working a conditional test at the present consumer features.
Within the following instance, we’re checking if the present consumer can edit others’ posts (in different phrases, if the present consumer function is Editor or upper):
add_filter( 'block_editor_settings_all',
serve as( $settings, $context ) {
if ( $context->submit && 'ebook' === $context->post->post_type ) {
$settings['canLockBlocks'] = current_user_can( 'edit_others_posts' );
}
go back $settings;
}, 10, 2
);
The next pictures examine the editor’s preliminary state for an Admin and an Writer. First, Admin:
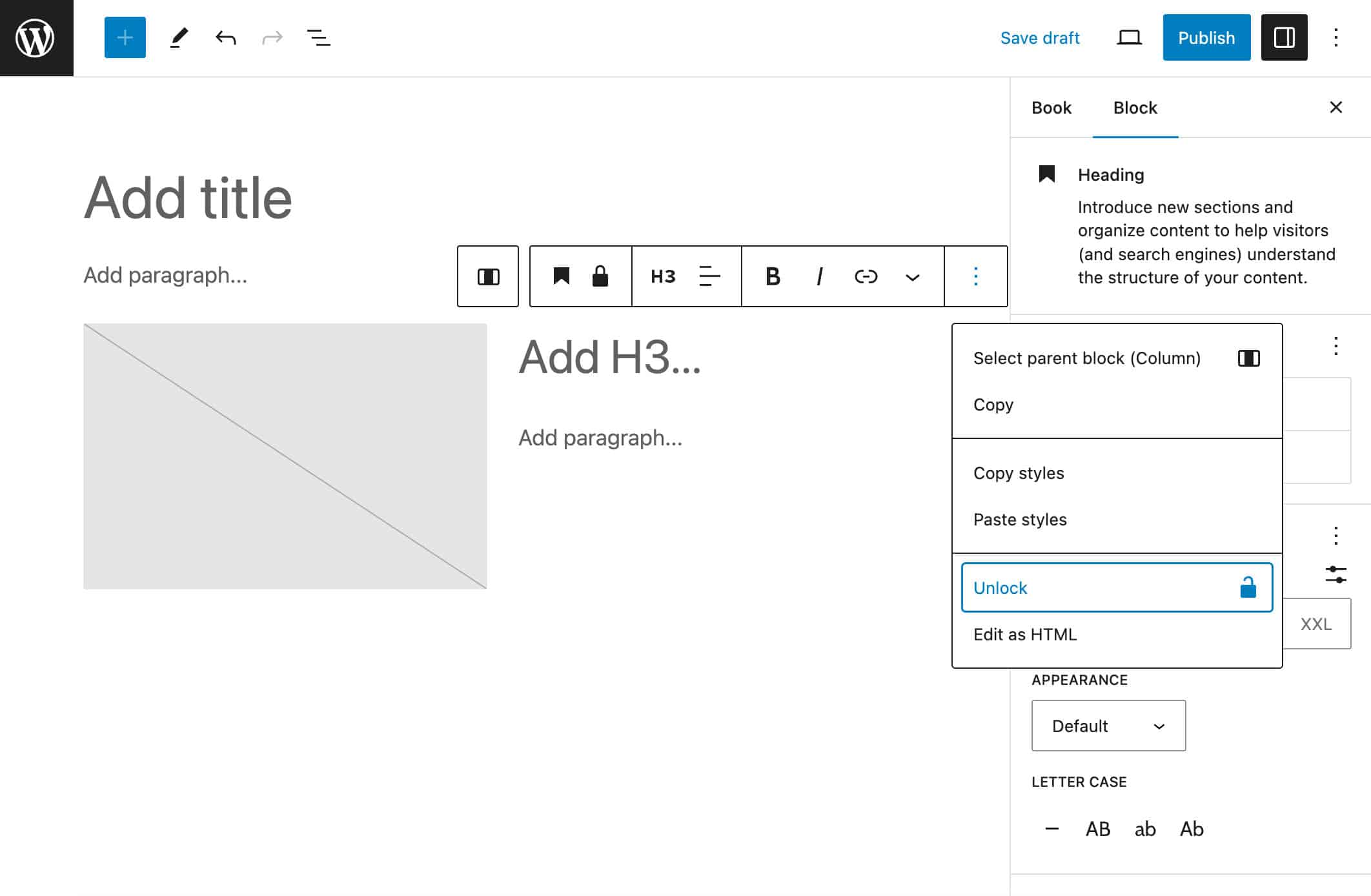
Now, Writer:
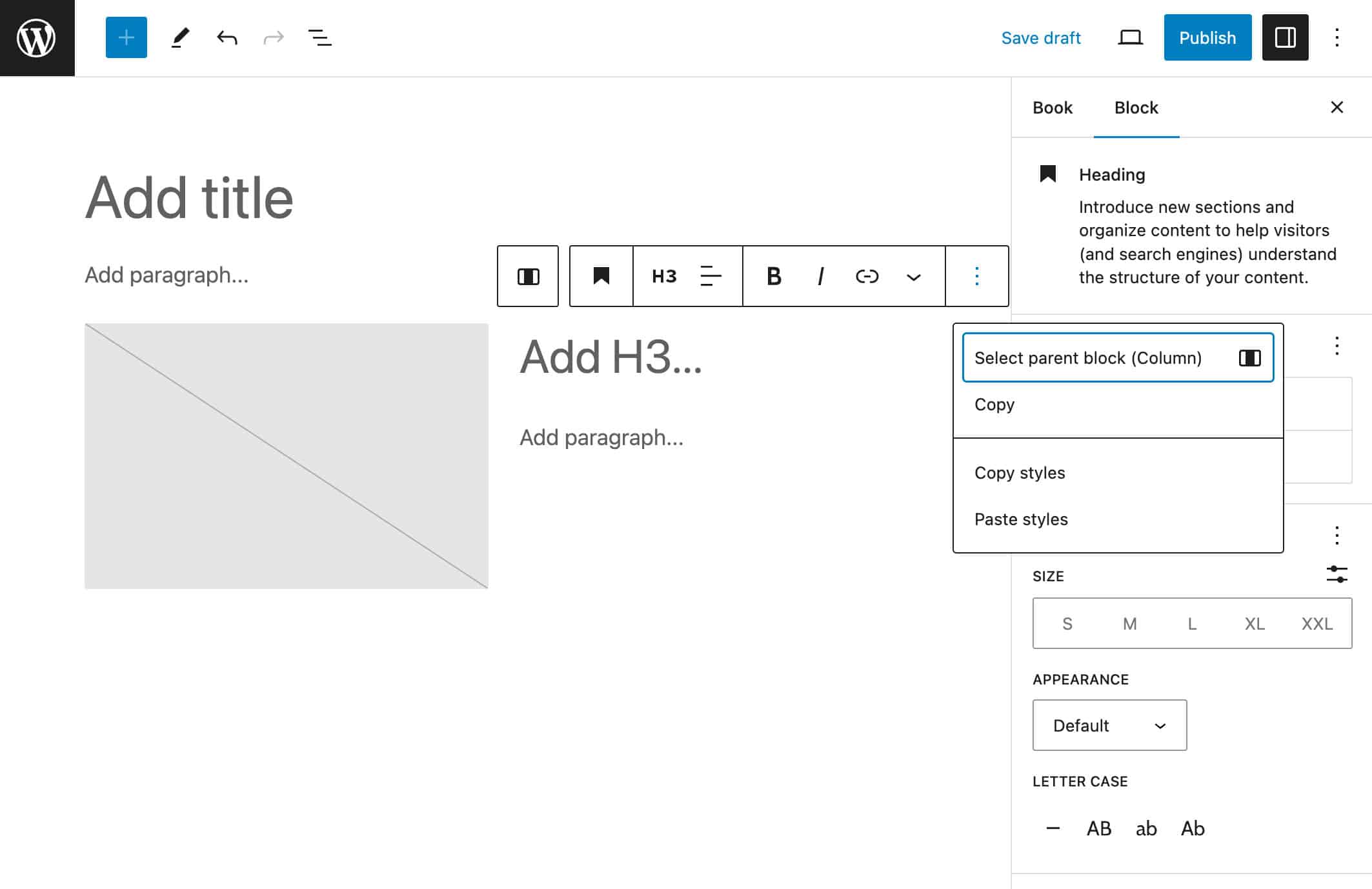
And you’ll test any situation at the present consumer. Within the following instance, we’re fighting a particular consumer from locking/unlocking blocks:
add_filter( 'block_editor_settings_all',
serve as( $settings, $context ) {
$consumer = wp_get_current_user();
if ( in_array( $user->user_email, [ '[email protected]' ], true ) ) {
$settings['canLockBlocks'] = false;
}
go back $settings;
}, 10, 2
);
You’ll mix extra stipulations to have granular keep an eye on over who is permitted to fasten/release blocks to your template and who isn’t. This is one facet of what’s referred to as Curated revel in.
However wait. Have you ever attempted to edit your submit content material the use of the code editor? Neatly, you can be stunned to look that customers now not allowed to release blocks from the UI can nonetheless exchange the content material from the code editor.
Disabling the Code Editor for Particular Person Roles
Through default, all customers who can edit content material can get admission to the code editor. This might override your locking settings and a few customers may break or delete your template format.
You’ll additionally use block_editor_settings_all
to filter out the codeEditingEnabled
atmosphere to stop explicit consumer roles to get admission to the code editor. This is the code:
add_filter( 'block_editor_settings_all',
serve as( $settings, $context ) {
if ( $context->submit && 'ebook' === $context->post->post_type ) {
$settings['canLockBlocks'] = current_user_can( 'edit_others_posts' );
$settings['codeEditingEnabled'] = current_user_can( 'edit_others_posts' );
}
go back $settings;
}, 10, 2
);
With this code in position, customers who don’t have edit_others_posts
capacity received’t be allowed to get admission to the code editor. The picture beneath displays the Choices toolbar for an Writer.
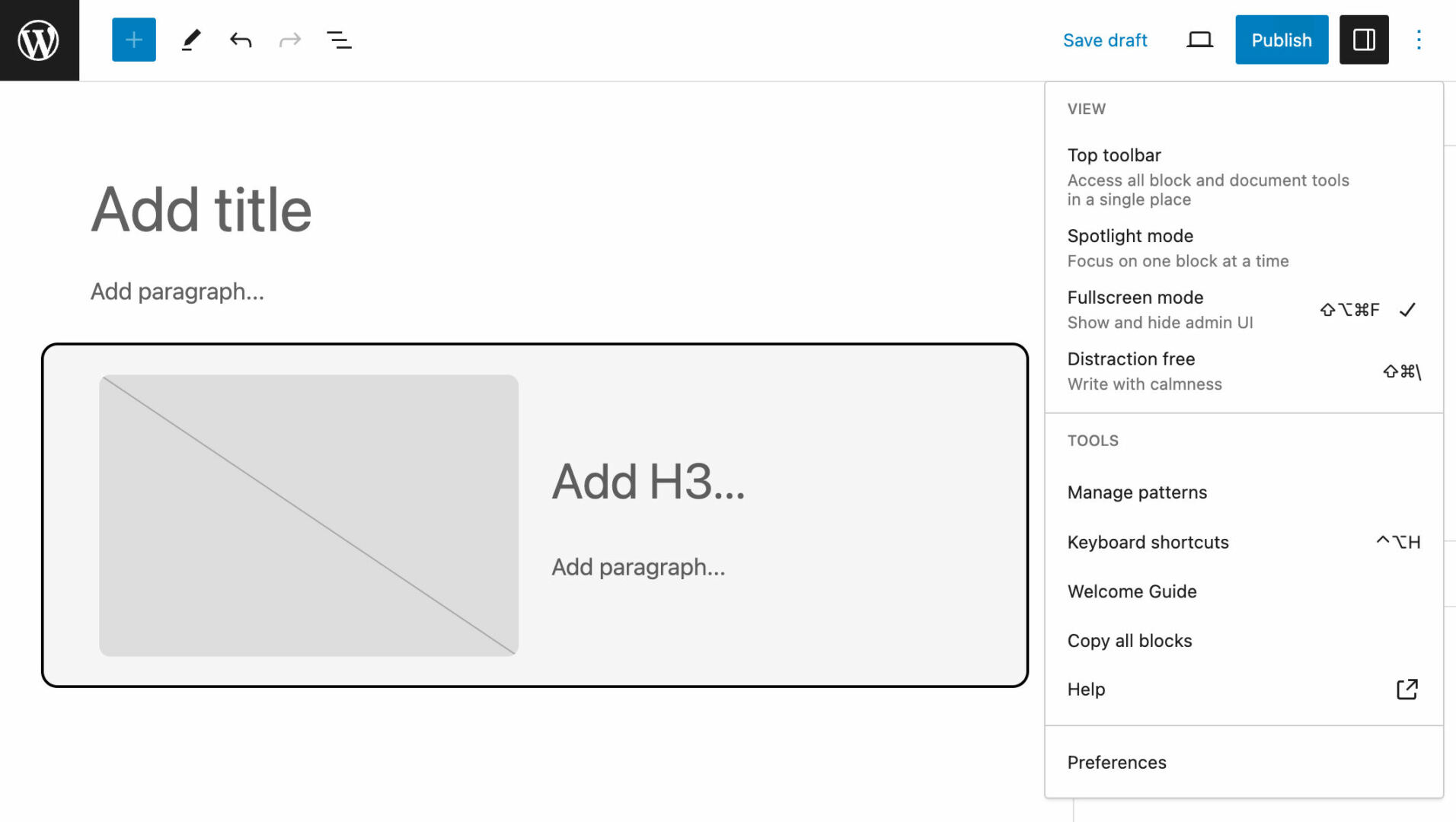
That’s what you want to understand to construct block templates by the use of PHP. Now, in case you are a Gutenberg block developer and like to paintings with JavaScript, you might like to go for a special means.
How To Construct a Template The usage of JavaScript
Including a block template to a submit works another way if you make a decision to head with JavaScript. You’ll nonetheless construct a template, however you want to create a customized block and use the InnerBlocks
part as mentioned in our Gutenberg block construction information.
InnerBlocks exports a couple of elements which can be utilized in block implementations to permit nested block content material. – Supply: InnerBlocks
How does it paintings?
You’ll use InnerBlocks
to your customized blocks the similar approach as every other Gutenberg part.
You first wish to come with it from a package deal in conjunction with different dependencies:
import { registerBlockType } from '@wordpress/blocks';
import { useBlockProps, InnerBlocks } from '@wordpress/block-editor';
Subsequent, you’ll outline InnerBlocks
houses. Within the following instance, we’ll claim a TEMPLATE
consistent we’ll then use to set the worth for the template
assets of the InnerBlocks
component:
const TEMPLATE = [
[ 'core/paragraph', { 'placeholder': 'Add paragraph...' } ],
[ 'core/columns', { verticalAlignment: 'center' },
[
[
'core/column',
{ templateLock: 'all' },
[
[ 'core/image' ]
]
],
[
'core/column',
{ templateLock: 'all' },
[
[
'core/heading',
{'placeholder': 'Add H3...', 'level': 3}
],
[
'core/paragraph',
{'placeholder': 'Add paragraph...'}
]
],
]
]
]
];
registerBlockType( metadata.title, {
name: 'My Template',
class: 'widgets',
edit: ( props ) => {
go back(
)
},
save() {
const blockProps = useBlockProps.save();
go back(
)
}
} );
This code is slightly easy. You must understand that we used the templateLock
characteristic two times, first on the block stage, then throughout the InnerBlocks
component. For all the listing of to be had props, see the InnerBlocks
reference and the Block Editor Guide.
Now take a look at it your self.
First, create an area WordPress set up the use of DevKinsta or every other native construction atmosphere.
Subsequent, release your command line instrument, navigate for your plugins folder, and run the next command:
npx @wordpress/create-block template-block
You’ll exchange the title of the block to no matter you need. If you would like keep an eye on any and each and every facet of your starter block, simply observe the instruction equipped in our definitive information to dam construction.
As soon as the set up is entire, run the next instructions:
cd template-block
npm get started
Release your WordPress admin dashboard and navigate to the Plugins display. To find and turn on your Template Block plugin.
To your favourite code editor, open the index.js report you in finding below the src folder. Reproduction and paste the code above, save your index.js, and again within the WordPress dashboard, create a brand new submit or web page.
Open the block inserter and scroll all the way down to the Widgets segment. There you must in finding your customized block.
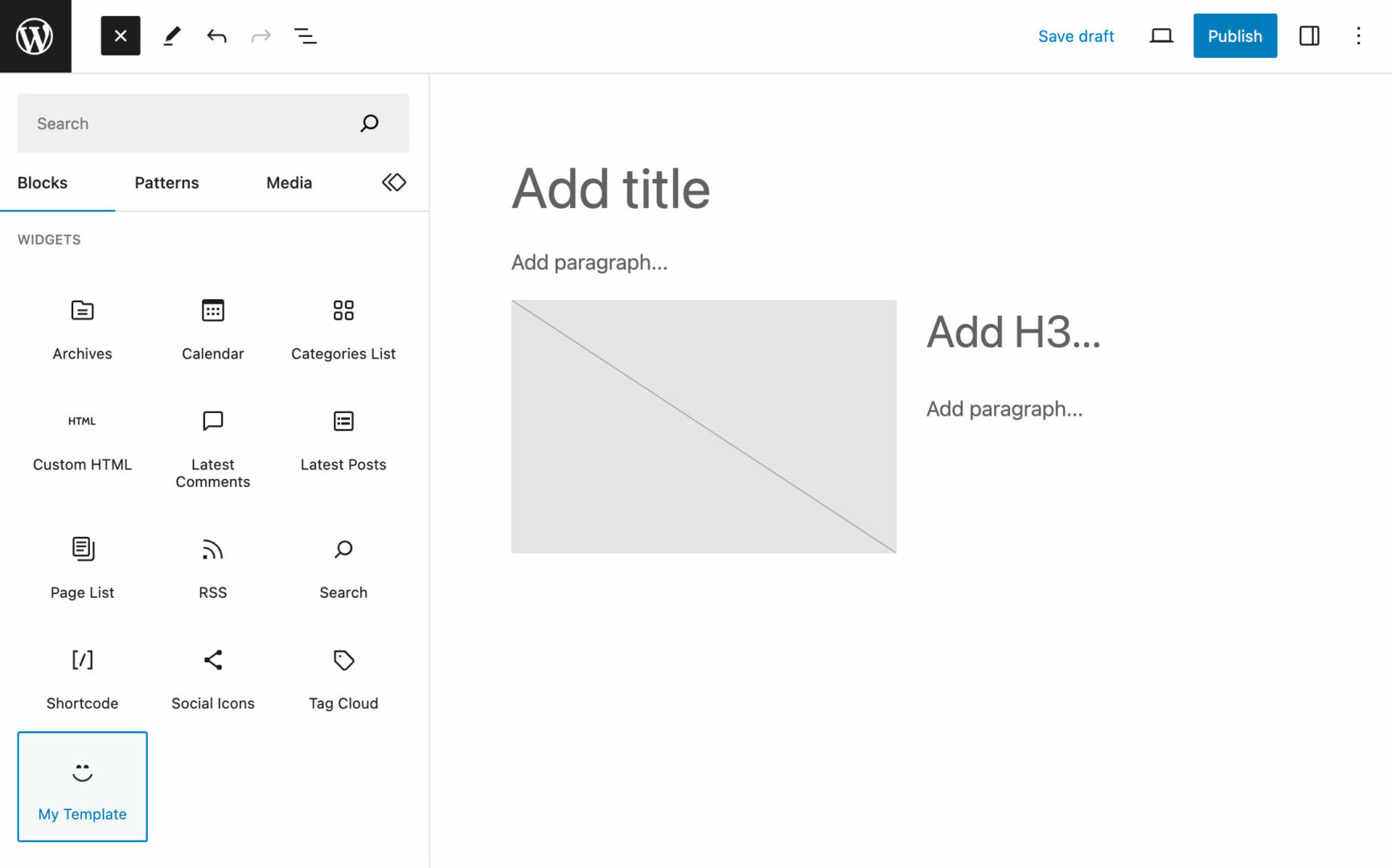
Upload it to the submit, customise the content material, and save the submit.
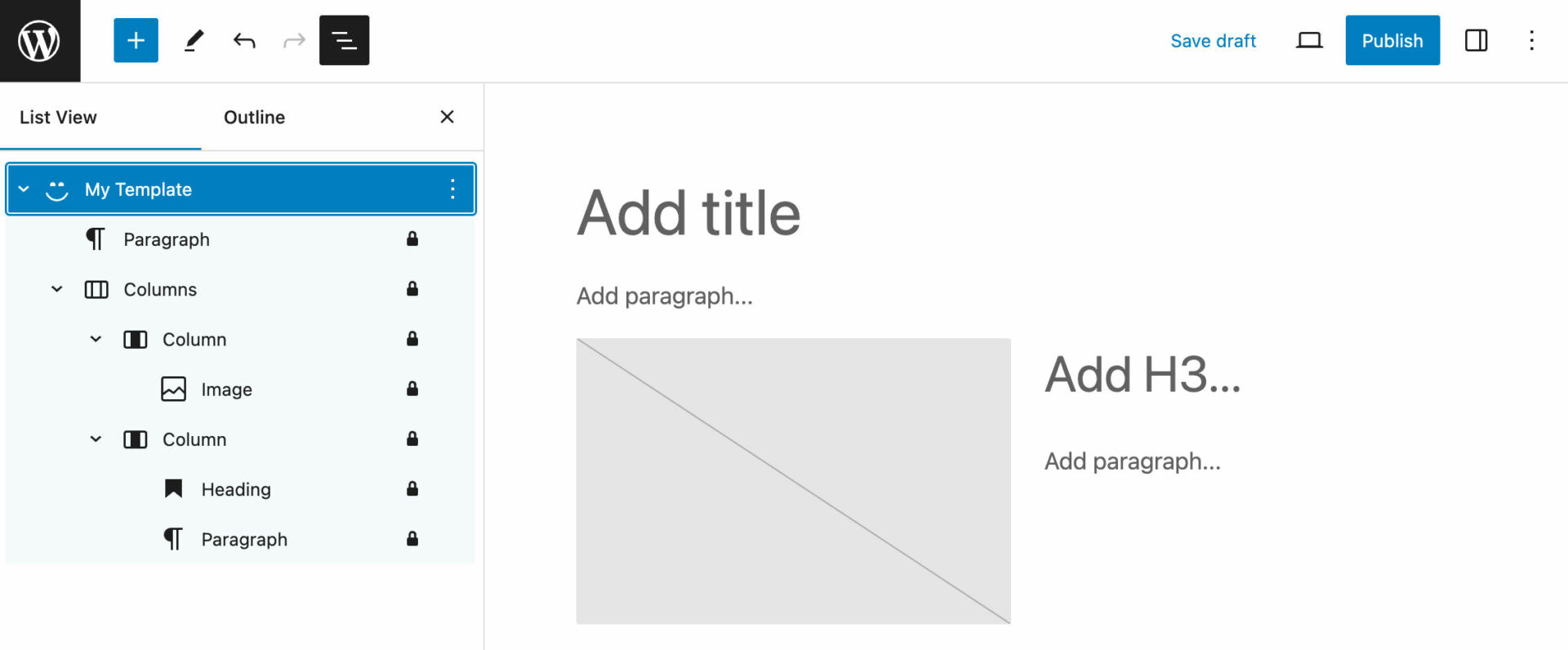
In case you transfer to the code editor, you’ll see the next markup:
Now preview the outcome to your favourite browser. In case your block look must be progressed, you’ll merely exchange the kinds to your taste.scss report.
As soon as you might be satisfied along with your customizations, prevent the method and run npm run construct
. All of the information of your undertaking will likely be compressed and to be had for manufacturing within the new construct folder.
That’s simple and strong, isn’t it?
Now you’ll create complex templates of blocks that you’ll come with to your content material with only some clicks.
Abstract
Including a block template for your submit or customized submit sorts can dramatically accelerate and enhance the advent and modifying revel in in your WordPress web site. Block templates are particularly helpful on multi-user internet sites, the place a couple of customers are enabled to create content material and you want them to stick to the similar structure.
This additionally lets you create uniform layouts with no need to manually upload a block sample every time you create a brand new submit. Bring to mind a assessment or recipe web site, the place every web page must appreciate the similar construction.
Through combining the information you’ll have won in growing static or dynamic customized blocks, block patterns, and block templates, it is possible for you to to at all times establish the most productive and efficient answer for development any form of WordPress web site.
As much as you currently. Have you ever already explored block templates? Which case use do you in finding them maximum are compatible for? Please, percentage your revel in within the feedback beneath.
The submit How To Construct WordPress Block Templates gave the impression first on Kinsta®.
WP Hosting