As the usage of chatbots and digital assistants continues to develop, many companies and builders are in search of techniques to create their very own AI-powered chatbots. ChatGPT is one such chatbot, created via OpenAI, this is in a position to enticing in human-like conversations and answering a variety of questions.
What You’re Going To Construct
On this educational, you are going to discover ways to construct a ChatGPT clone software the usage of React and the OpenAI API. If you need to check out your hand at a amusing and attractive mission over the weekend, this can be a nice alternative to dive into React and OpenAI.
You’re going to additionally discover ways to deploy immediately out of your GitHub repository to Kinsta’s Software Website hosting platform, which supplies a unfastened .kinsta.app area to make your mission move reside all of a sudden. And with Kinsta’s unfastened trial and Passion Tier, you’ll simply get began with none price.
Right here’s a reside demo of the ChatGPT clone software.
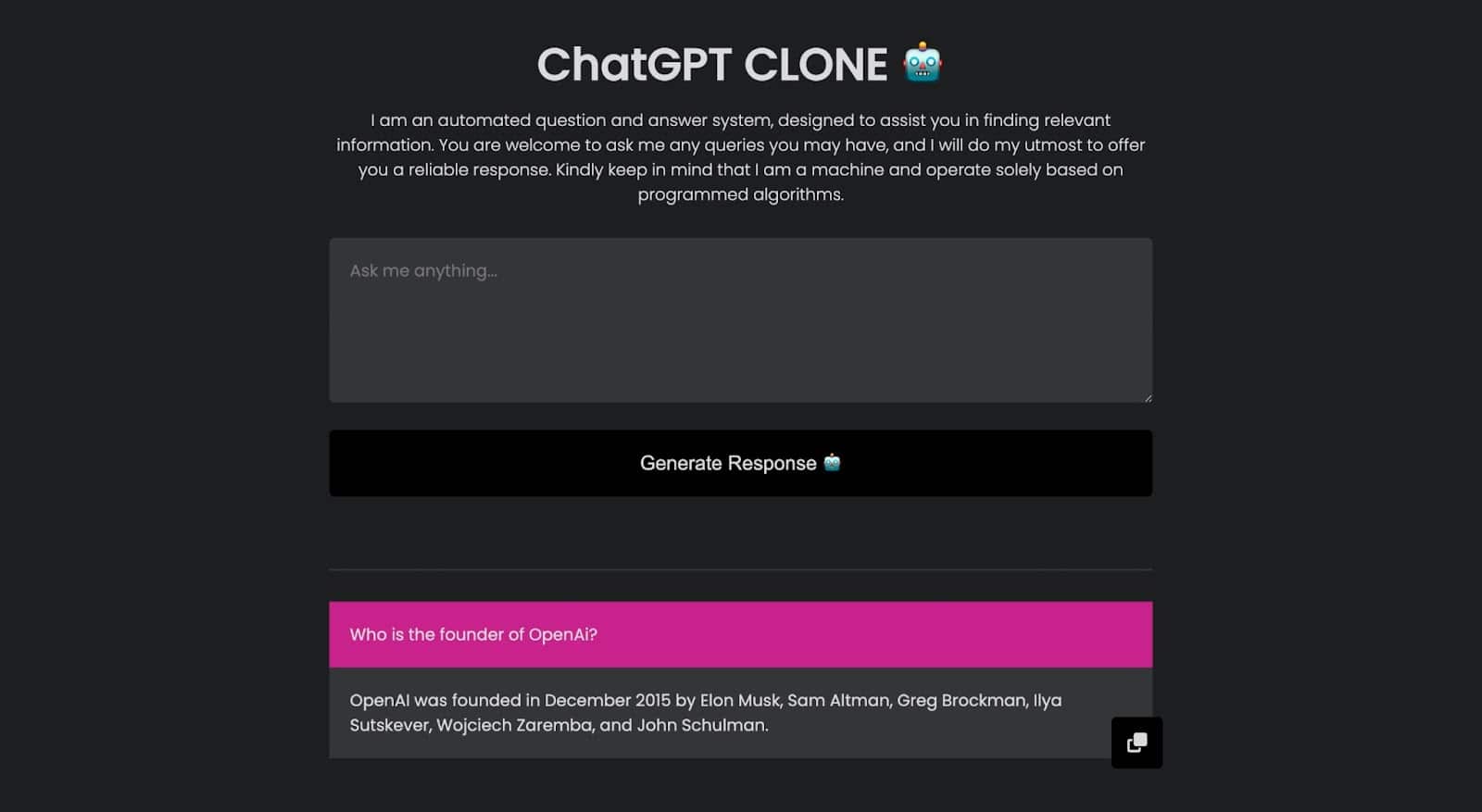
For those who’d love to check up on this mission extra carefully, you’ll get admission to its GitHub repository.
On the other hand, you must additionally clone the React software starter mission, which comes with basic parts comparable to kinds, Font Superior CDN hyperlink, OpenAI bundle, and elementary construction, to lend a hand you in getting began.
Necessities/Must haves
This educational is designed to be a “follow-along” revel in. Due to this fact, it’s beneficial that you’ve the next to code along very easily:
- Basic working out of HTML, CSS, and JavaScript
- Some familiarity with React
- Node.js and npm (Node Package deal Supervisor) or yarn put in in your laptop
What’s OpenAI API?
The OpenAI API is a cloud-based platform that grants builders get admission to to OpenAI’s language fashions, comparable to GPT-3, by means of an API. It lets in builders so as to add herbal language processing options like textual content crowning glory, sentiment research, summarization, and translation to their programs with out growing and coaching their fashions.
To make use of the OpenAI API, builders will have to create an account at the OpenAI site and acquire an API key. The API key’s used to authenticate API requests and observe utilization.
As soon as the API key’s got, builders can use the API to put up textual content to the language fashion and obtain responses.
Why React?
React is a widespread JavaScript library for development consumer interfaces. In step with the 2022 Stack Overflow developer survey, it’s the second one maximum often used internet generation, with 42.62% of the marketplace percentage.
React lets in builders to create declarative parts representing other portions of the consumer interface. Those parts are outlined the usage of a syntax referred to as JSX, which is a mix of JavaScript and HTML.
Because of its huge ecosystem of part libraries and kits, builders can simply paintings with and combine APIs such because the OpenAI API, to construct advanced chat interfaces and that’s what makes it a very good selection for development a ChatGPT clone software.
How To Set Up Your React Construction Atmosphere
One of the best ways to put in React or create a React mission is to put in it with create-react-app. One prerequisite is to have Node.js put in in your device. To substantiate that you’ve Node put in, run the next command to your terminal.
node -v
If it brings up a model, then it exists. To make use of npx, you’ll want to ensure that your Node model isn’t lower than v14.0.0, and your NPM model isn’t lower than v5.6; else, you must want to replace it via operating npm replace -g
. While you’ve found out npm, you’ll now arrange a React mission via operating the command underneath:
npx create-react-app chatgpt-clone
Be aware: “chatgpt-clone” is the appliance identify we’re growing, however you’ll exchange it to any identify of your selection.
The set up procedure might take a couple of mins. As soon as a success, you’ll navigate to the listing and set up the Node.js OpenAI bundle, which supplies handy get admission to to the OpenAI API from Node.js the usage of the command underneath:
npm set up openai
You’ll now run npm get started
to look your software survive localhost:3000.
When a React mission is created the usage of the create-react-app
command, it mechanically scaffolds a folder construction. The foremost folder that considerations you is the src
folder, which is the place building takes position. This folder accommodates many recordsdata via default however you must best be involved in the App.js, index.js and index.css recordsdata.
- App.js: The App.js report is the primary part in a React software. It most often represents the top-level part that renders all different parts within the software.
- index.js: This report is the access level of your React software. It’s the first report loaded when the app is opened and is liable for rendering the App.js part to the browser.
- index.css: This report is liable for defining the entire styling and format of your React software.
How To Construct a ChatGPT Clone With React and OpenAI API
The ChatGPT clone software will consist of 2 parts to make the appliance more straightforward to grasp and take care of. Those two parts are:
- Shape Segment: This part features a textual content space box and a button for customers to engage with the chatbot.
- Solution Segment: The questions and corresponding solutions can be saved in an array and displayed on this segment. You’re going to loop in the course of the array chronologically, appearing the newest first.
Surroundings Up the ChatGPT Clone Software
On this educational, allow us to get started via first development the appliance interface after which you’ll enforce capability so your software interacts with the OpenAI API. Get started via growing the 2 parts you are going to use on this educational. For right kind group, you are going to create a parts folder within the src folder the place all of the parts can be saved.
The Shape Segment Part
This can be a easy shape that is composed of a textarea
and a put up button
.
// parts/FormSection.jsx
const FormSection = () => {
go back (
)
}
export default FormSection;
That is what the shape is anticipated to appear to be whilst you import it into your App.js report:
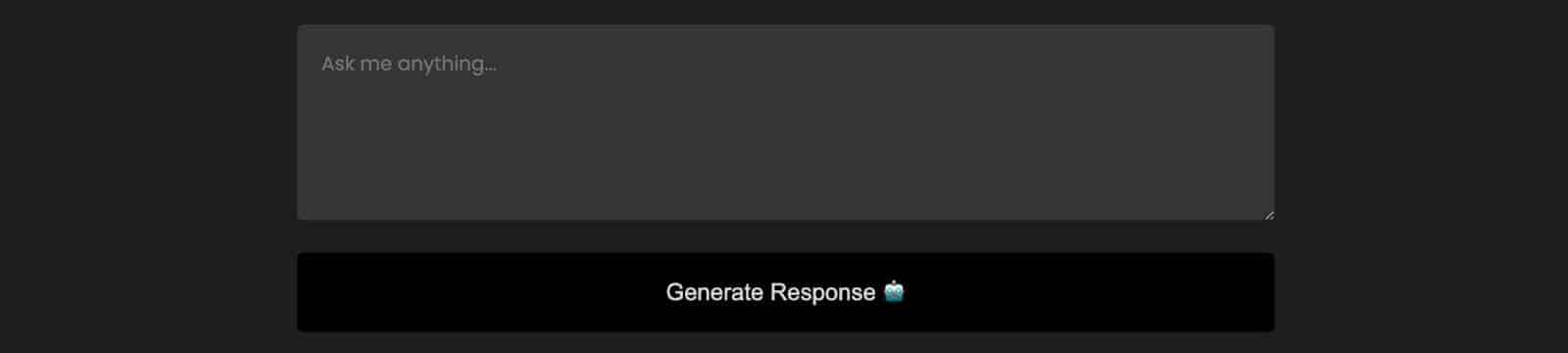
The Solution Segment Part
This segment is the place all questions and solutions can be displayed. That is what this segment will appear to be whilst you additionally import it into your App.js report.
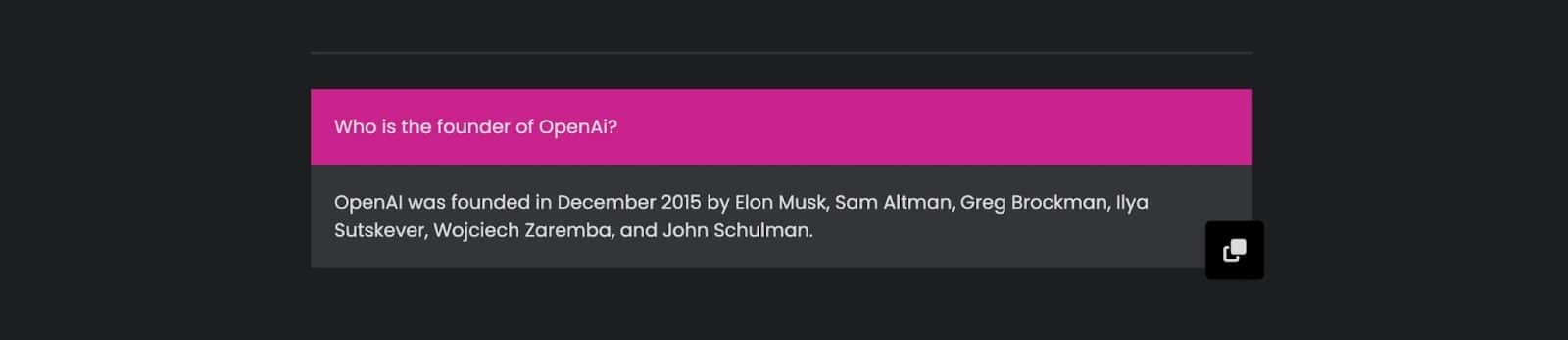
You’re going to fetch those questions and solutions from an array and loop to make your code more straightforward to learn and take care of.
// parts/AnswerSection.jsx
const AnswerSection = () => {
go back (
<>
Who's the founding father of OpenAi?
OpenAI used to be based in December 2015 via Elon Musk, Sam Altman, Greg Brockman, Ilya Sutskever, Wojciech Zaremba, and John Schulman.
>
)
}
export default AnswerSection;
The House Web page
You currently have each parts created, however not anything will seem whilst you run your software as a result of you want to import them into your App.js report. For this software, you are going to now not enforce any type of routing, which means the App.js report will function your software’s house part/web page.
You’ll upload some content material, just like the identify and outline of your software, earlier than uploading the parts.
// App.js
import FormSection from './parts/FormSection';
import AnswerSection from './parts/AnswerSection';
const App = () => {
go back (
ChatGPT CLONE 🤖
I'm an automatic query and solution machine, designed to lend a hand you
to find related knowledge. You're welcome to invite me any queries
you might have, and I will be able to do my utmost to give you a competent
reaction. Kindly remember the fact that I'm a device and perform only
in keeping with programmed algorithms.
);
};
export default App;
Within the code above, the 2 parts are imported and added to the appliance. Whilst you run your software, that is what your software will appear to be:
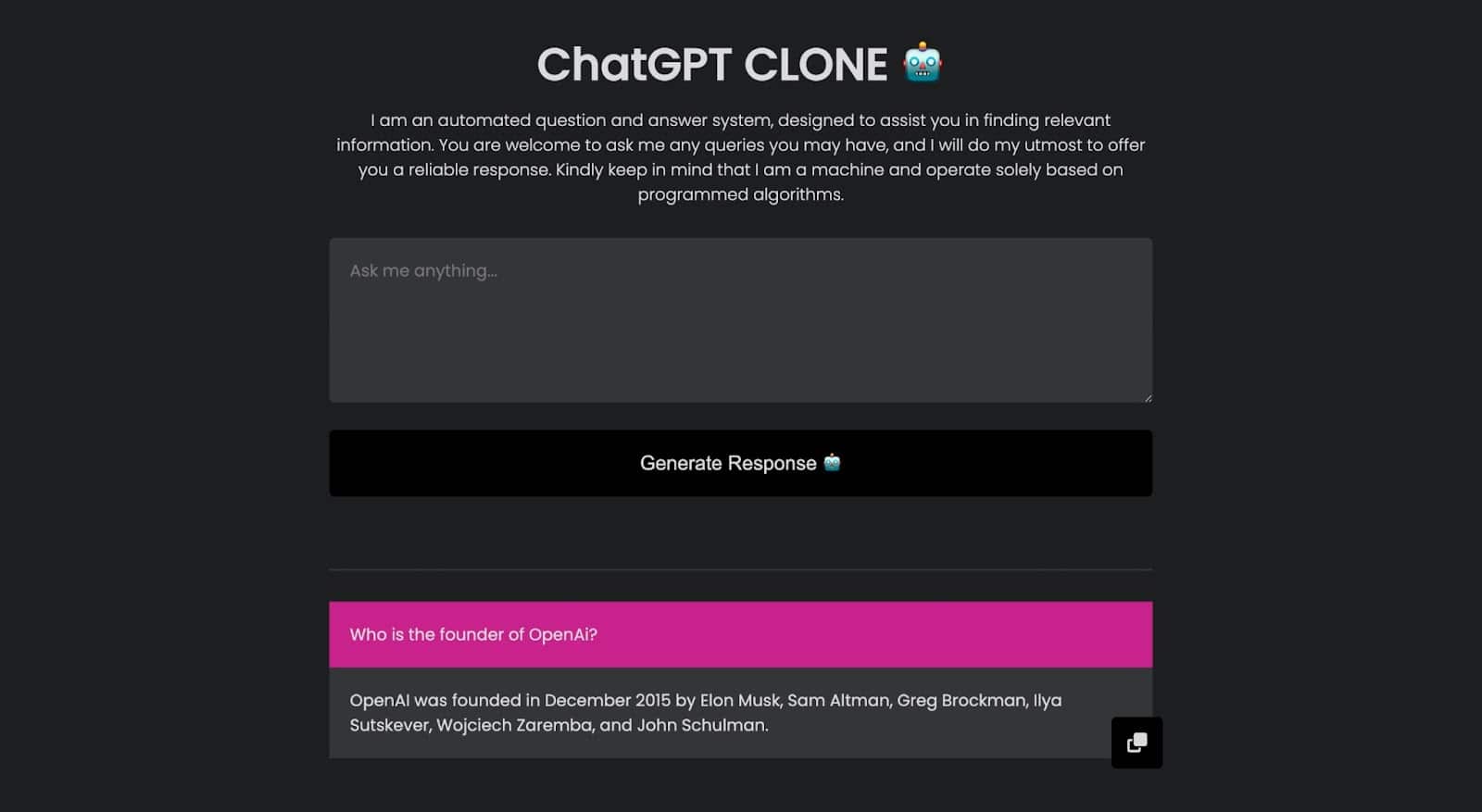
Including Capability and Integrating OpenAI API
You currently have the consumer interface of your software. The next move is to make the appliance useful so it may well have interaction with the OpenAI API and get responses. First, you want to get the worth out of your shape when submitted as a result of it is going to be used to question the OpenAI API.
Getting Information From Shape
In React, one of the best ways to retailer and replace information is to make use of states. In useful parts, the useState()
hook is used to paintings with states. You’ll create a state, assign the worth out of your shape to the state, and replace it on every occasion its worth adjustments. Let’s get started via uploading the useState()
hook into the FormSection.jsx part after which making a state to retailer and replace newQuestions
.
// parts/FormSection.jsx
import { useState } from 'react';
const FormSection = ({ generateResponse }) => {
const [newQuestion, setNewQuestion] = useState('');
go back (
// Shape to put up a brand new query
)
}
export default FormSection;
Subsequent, you’ll assign the worth of the textarea
box to the state and create an onChange()
tournament to replace the state on every occasion the enter worth adjustments:
In spite of everything, you’ll create an onClick()
tournament, to load a serve as on every occasion the put up button is clicked. This system can be created within the App.js report and handed as a props into the FormSection.jsx part with the newQuestion
and setNewQuestion
values as arguments.
You could have now created a state to retailer and replace the shape worth, added a technique which is handed as a props from the App.js report and treated click on tournament. That is what the general code will appear to be:
// parts/FormSection.jsx
import { useState } from 'react';
const FormSection = ({ generateResponse }) => {
const [newQuestion, setNewQuestion] = useState('');
go back (
)
}
export default FormSection;
The next move can be to create a technique within the App.js report to care for all the means of interacting with OpenAI API.
Interacting With OpenAI API
To have interaction with OpenAI API and acquire API keys in a React software, you will have to create an OpenAI API account. You’ll join an account at the OpenAI site the usage of your google account or e mail. To generate an API key, click on Private on the top-right nook of the site; some choices will seem; click on View API keys.
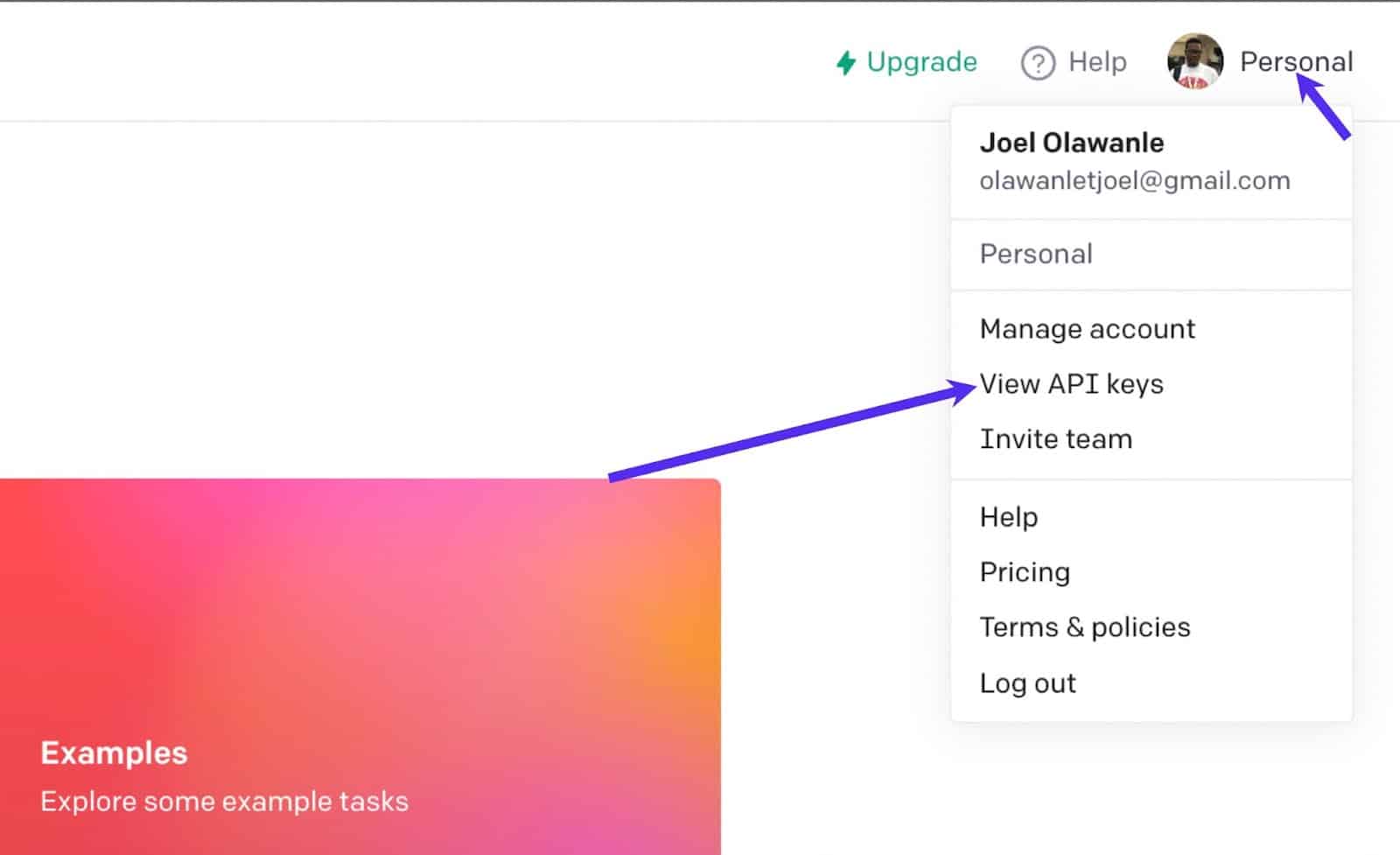
Click on the Create new secret key button, reproduction the important thing someplace as you possibly can use it on this software to engage with OpenAI. You’ll now continue to initialize OpenAI via uploading the openai bundle (you already put in) at the side of the configuration way. Then create a configuration together with your generated key and use it to initialize OpenAI.
// src/App.js
import { Configuration, OpenAIApi } from 'openai';
import FormSection from './parts/FormSection';
import AnswerSection from './parts/AnswerSection';
const App = () => {
const configuration = new Configuration({
apiKey: procedure.env.REACT_APP_OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
go back (
// Render FormSection and AnswerSection
);
};
export default App;
Within the code above, the OpenAI API key’s saved as an surroundings variable within the .env report. You’ll create a .env report within the root folder of your software and retailer the important thing to the variable REACT_APP_OPENAI_API_KEY
.
// .env
REACT_APP_OPENAI_API_KEY = sk-xxxxxxxxxx…
You’ll now continue to create the generateResponse
way within the App.js report, and move within the two parameters anticipated from the shape you already created to care for the request and get reaction from the API.
// src/App.js
import FormSection from './parts/FormSection';
import AnswerSection from './parts/AnswerSection';
const App = () => {
const generateResponse = (newQuestion, setNewQuestion) => {
// Arrange OpenAI API and care for reaction
};
go back (
// Render FormSection and AnswerSection
);
};
export default App;
You’ll now ship a request to the OpenAI API. The OpenAI API can carry out many operations, comparable to query and solution (Q&A), Grammar correction, translation and so much extra. For every of those operations, the choices are other. For instance, the engine worth for Q&A is text-davinci-00
, whilst for SQL translate is code-davinci-002
. Be happy to test the OpenAI instance documentation for the quite a lot of examples and their choices.
For this educational, we’re operating best with the Q&A, that is what the choice seems like:
{
fashion: "text-davinci-003",
suggested: "Who's Obama?",
temperature: 0,
max_tokens: 100,
top_p: 1,
frequency_penalty: 0.0,
presence_penalty: 0.0,
forestall: [""],
}
Be aware: I modified the suggested worth.
The suggested is the query this is despatched from the shape. This implies it is very important obtain it from the shape enter you’re passing into the generateResponse
way as a parameter. To try this, you are going to outline the choices after which use the unfold operator to create an entire possibility that comes with the suggested:
// src/App.js
import { Configuration, OpenAIApi } from 'openai';
import FormSection from './parts/FormSection';
import AnswerSection from './parts/AnswerSection';
const App = () => {
const configuration = new Configuration({
apiKey: procedure.env.REACT_APP_OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
const generateResponse = async (newQuestion, setNewQuestion) => {
let choices = {
fashion: 'text-davinci-003',
temperature: 0,
max_tokens: 100,
top_p: 1,
frequency_penalty: 0.0,
presence_penalty: 0.0,
forestall: ['/'],
};
let completeOptions = {
...choices,
suggested: newQuestion,
};
};
go back (
// Render FormSection and AnswerSection
);
};
export default App;
At this level, what’s left is to ship a request by means of the createCompletion
solution to OpenAI to get a reaction.
// src/App.js
import { Configuration, OpenAIApi } from 'openai';
import FormSection from './parts/FormSection';
import AnswerSection from './parts/AnswerSection';
import { useState } from 'react';
const App = () => {
const configuration = new Configuration({
apiKey: procedure.env.REACT_APP_OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
const [storedValues, setStoredValues] = useState([]);
const generateResponse = async (newQuestion, setNewQuestion) => {
let choices = {
fashion: 'text-davinci-003',
temperature: 0,
max_tokens: 100,
top_p: 1,
frequency_penalty: 0.0,
presence_penalty: 0.0,
forestall: ['/'],
};
let completeOptions = {
...choices,
suggested: newQuestion,
};
const reaction = look ahead to openai.createCompletion(completeOptions);
console.log(reaction.information.possible choices[0].textual content);
};
go back (
// Render FormSection and AnswerSection
);
};
export default App;
Within the code above, the solution’s textual content can be displayed in your console. Be happy to check your software via asking any query. The general step can be to create a state that may grasp the array of questions and solutions after which ship this array as a prop into the AnswerSection part. That is what the App.js ultimate code will appear to be:
// src/App.js
import { Configuration, OpenAIApi } from 'openai';
import FormSection from './parts/FormSection';
import AnswerSection from './parts/AnswerSection';
import { useState } from 'react';
const App = () => {
const configuration = new Configuration({
apiKey: procedure.env.REACT_APP_OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
const [storedValues, setStoredValues] = useState([]);
const generateResponse = async (newQuestion, setNewQuestion) => {
let choices = {
fashion: 'text-davinci-003',
temperature: 0,
max_tokens: 100,
top_p: 1,
frequency_penalty: 0.0,
presence_penalty: 0.0,
forestall: ['/'],
};
let completeOptions = {
...choices,
suggested: newQuestion,
};
const reaction = look ahead to openai.createCompletion(completeOptions);
if (reaction.information.possible choices) {
setStoredValues([
{
question: newQuestion,
answer: response.data.choices[0].textual content,
},
...storedValues,
]);
setNewQuestion('');
}
};
go back (
ChatGPT CLONE 🤖
I'm an automatic query and solution machine, designed to lend a hand you
to find related knowledge. You're welcome to invite me any
queries you might have, and I will be able to do my utmost to give you a
dependable reaction. Kindly remember the fact that I'm a device and
perform only in keeping with programmed algorithms.
);
};
export default App;
You’ll now edit the AnswerSection part, so it receives the props worth from App.js and use the JavaScript Map()
solution to glance in the course of the storedValues
array:
// parts/AnswerSection.jsx
const AnswerSection = ({ storedValues }) => {
go back (
<>
{storedValues.map((worth, index) => {
go back (
{worth.query}
{worth.solution}
);
})}
>
)
}
export default AnswerSection;
Whilst you run your software and check it via asking questions, the solution will show underneath. However you are going to understand the reproduction button doesn’t paintings. It is very important upload an onClick()
tournament to the button, so it triggers a solution to care for the capability. You’ll use the navigator.clipboard.writeText()
solution to care for the capability. That is what the AnswerSection part will now appear to be:
// parts/AnswerSection.jsx
const AnswerSection = ({ storedValues }) => {
const copyText = (textual content) => {
navigator.clipboard.writeText(textual content);
};
go back (
<>
{storedValues.map((worth, index) => {
go back (
{worth.query}
{worth.solution}
copyText(worth.solution)}
>
);
})}
>
)
}
export default AnswerSection;
Whilst you run your software, your ChatGPT clone software will paintings completely. You’ll now deploy your software to get admission to it on-line and percentage it with buddies.
How To Deploy Your React Software to Kinsta
It’s now not sufficient to construct this software and go away it in your native computer systems. You’ll wish to percentage it on-line, so others can get admission to it. Let’s see how to do that the usage of GitHub and Kinsta.
Push Your Code to GitHub
To push your code to GitHub, you’ll use Git instructions, which is a competent and environment friendly method to set up code adjustments, collaborate on initiatives, and take care of model historical past.
Step one to push your codes can be to create a brand new repository via logging in for your GitHub account, clicking at the + button within the peak correct nook of the display screen, and settling on New repository from the dropdown menu.
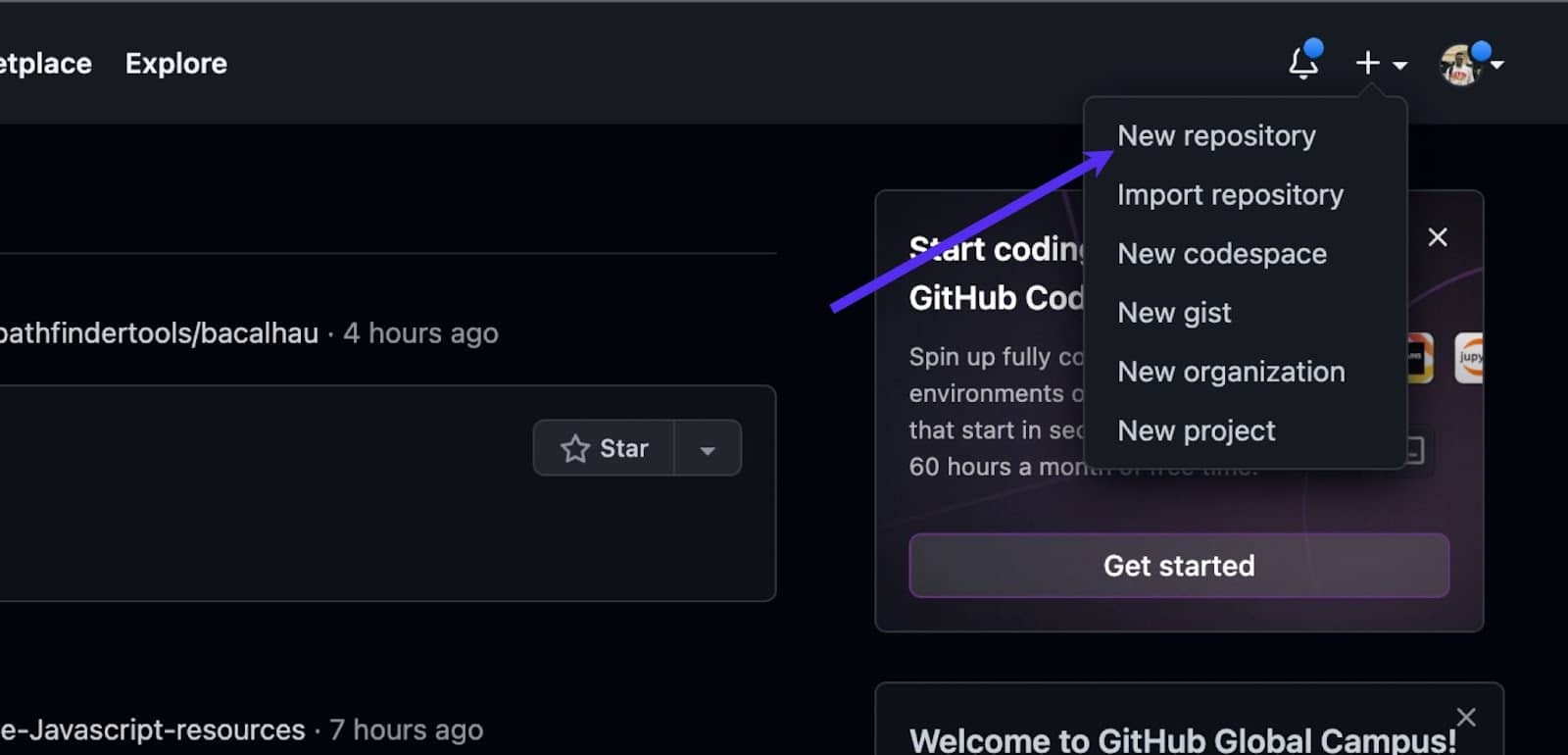
Give your repository a reputation, upload an outline (non-compulsory), and make a selection whether or not you need it to be public or personal. Click on Create repository to create it.
As soon as your repository is created, make sure you download the repository URL from the primary web page of your repository, which it is very important push your code to GitHub.
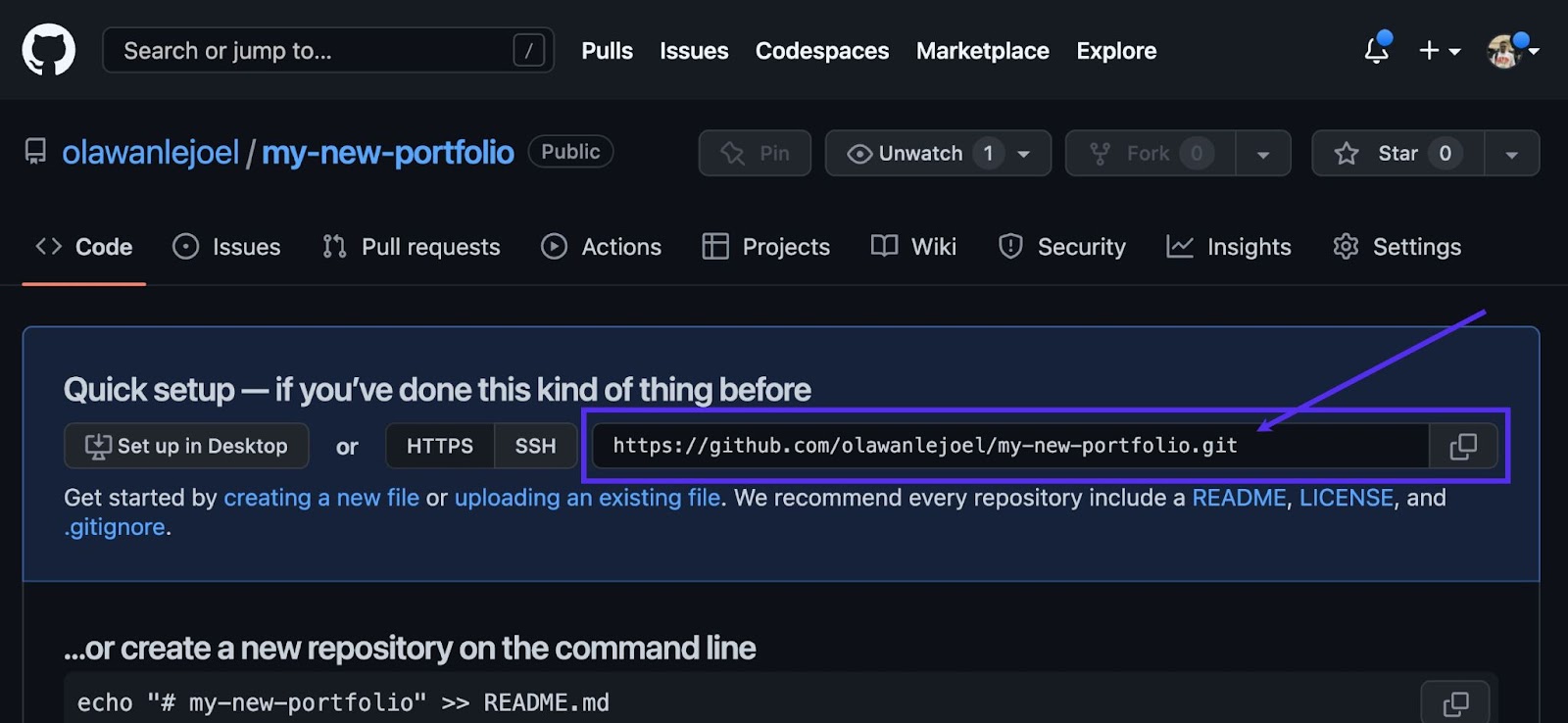
Open your terminal or command suggested and navigate to the listing that accommodates your mission. Run the next instructions separately to push your code for your GitHub repository:
git init
git upload .
git devote -m "my first devote"
git far flung upload beginning [repository URL]
git push -u beginning grasp
git init
initializes an area Git repository, git upload .
provides all recordsdata within the present listing and its subdirectories to the brand new Git repository. git devote -m "my first devote"
commits the adjustments to the repository with a short lived message. git far flung upload beginning [repository URL]
units the repository URL because the far flung repository and git push -u beginning grasp
pushes the code to the far flung repository (beginning) within the grasp department.
Deploy Your ChatGPT Clone React Software to Kinsta
To deploy your repository to Kinsta, persist with those steps:
- Log in to or create your Kinsta account at the My Kinsta dashboard.
- Click on Programs at the left sidebar after which click on Upload provider.
- Choose Software from the dropdown menu to deploy a React software to Kinsta.
- Choose the repository you want to deploy from the modal that looks. You probably have a couple of branches, you’ll make a selection the only you need to deploy and assign a reputation to the appliance. Choose a information heart location a few of the 25 to be had, and Kinsta will mechanically stumble on a get started command.
- In spite of everything, it’s now not secure to push out API keys to public hosts like GitHub, it used to be added as an atmosphere variable in the community. When webhosting, you’ll additionally upload it as an surroundings variable the usage of the similar variable identify and the important thing as its worth.
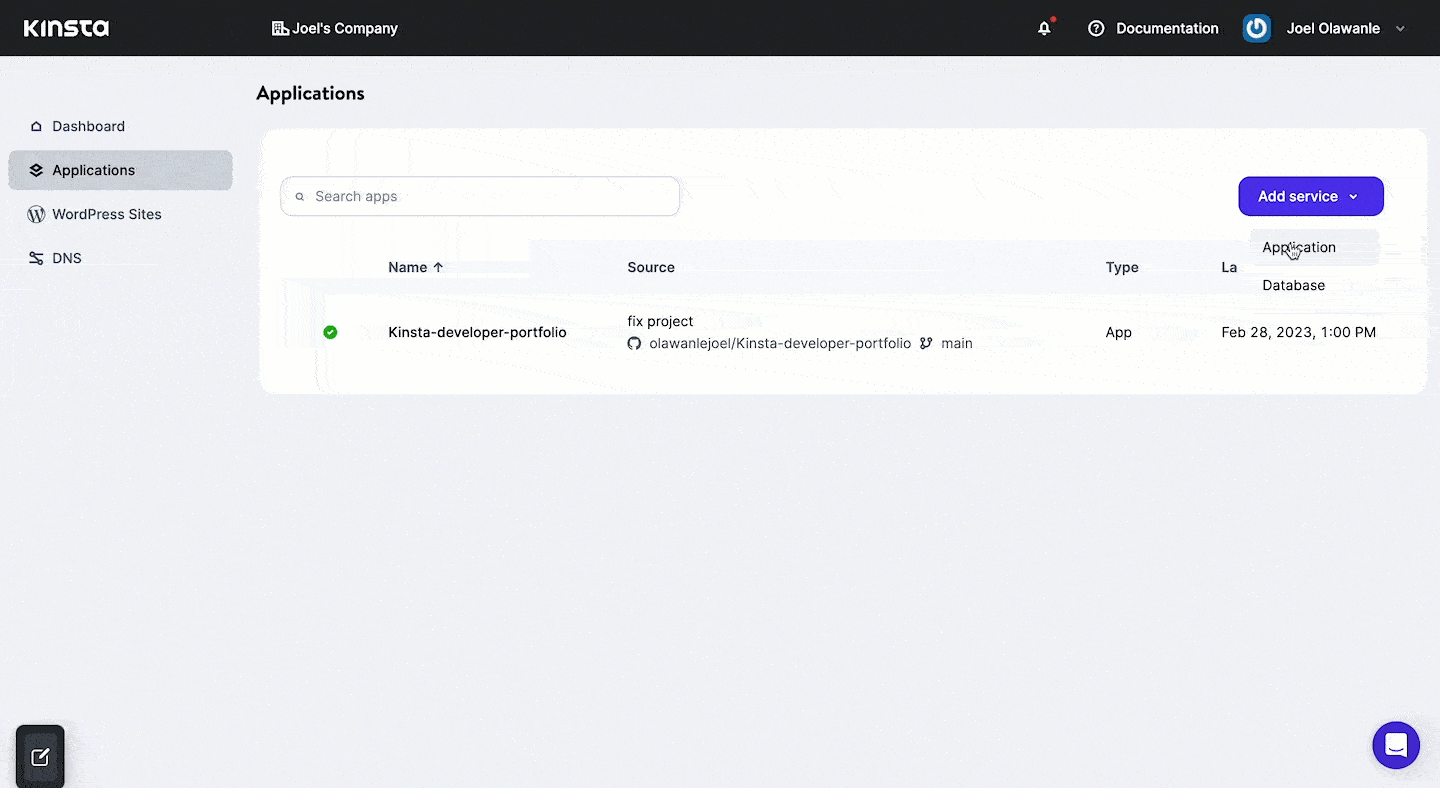
Your software will get started deploying, and inside of a couple of mins, a hyperlink can be supplied to get admission to the deployed model of your software. On this case, that is https://chatgpt-clone-g9q10.kinsta.app/Be aware: You’ll permit automated deployment, so Kinsta will re-deploy your software on every occasion you convert your codebase and push it to GitHub.
Abstract
The OpenAI API can be utilized to construct a variety of possible programs, from buyer reinforce and private assistants to language translation and content material advent.
On this educational, you will have discovered the right way to construct a ChatGPT clone software with React and OpenAI. You’ll combine this software/function into different programs to supply human-like conversational stories to customers.
There’s extra to what may also be performed with OpenAI API and the right way to give a boost to this clone software. For instance, you’ll enforce native garage in order that earlier questions and solutions don’t disappear even after you refresh your browser.
With Kinsta’s unfastened trial and Passion Tier, you’ll simply get began with none price on our Software Website hosting. So why now not give it a try to see what you’ll create?
Proportion your mission and stories within the remark underneath.
The put up How To Construct and Deploy a ChatGPT Clone Software With React and OpenAI API gave the impression first on Kinsta®.
WP Hosting