Synchronous and asynchronous are complicated ideas in JavaScript, particularly for rookies. Two or extra issues are synchronous once they occur on the similar time (in sync), and asynchronous once they don’t (no longer in sync).
Despite the fact that those definitions are simple to absorb, it’s in truth extra sophisticated than it seems to be. We wish to remember what precisely are in sync, and what aren’t.
You’d almost certainly name a standard
serve as in JavaScript synchronous, proper? And if it’s one thing like setTimeout()
or AJAX that you just’re operating with, you are going to confer with it as being asynchronous, sure? What if I inform you that each are asynchronous in some way?
To provide an explanation for the why, we wish to flip to Mr X for assist.
Learn Additionally: 15 DOM Manipulation JavaScript Methods for Web Developers
State of affairs 1 – Mr X is making an attempt synchronicity
Right here’s the setup:
- Mr X is any person who can resolution tricky questions, and perform any asked process.
- The one method to touch him is thru a telephone name.
- No matter query or process you were given, as a way to ask Mr X’s assist to hold it out; you name him.
- Mr X will give you the solution or completes the duty immediately, and allows you to know it’s finished.
- You set down the receiver feeling content material and cross out for a film.
What you’ve simply performed was once a synchronous (backward and forward) verbal exchange with Mr X.
He listened as you have been asking him your query, and also you listened when he was once answering it.
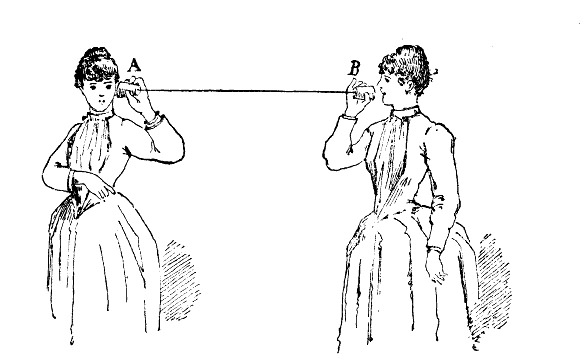
State of affairs 2 – Mr X isn’t proud of synchronicity
Since Mr X is so environment friendly, he begins receiving many extra calls. So what occurs while you name him however he’s already busy chatting with any person else? You gained’t be capable of ask him your query – no longer until he’s loose to obtain your name. All you are going to listen is a hectic tone.
So what can Mr X do to battle this?
As an alternative of taking calls immediately:
- Mr X hires a brand new man, Mr M and offers him an answering gadget for the callers to depart messages.
- Mr M’s activity is to cross on a message from the answering gadget to Mr X as soon as he is aware of Mr X has utterly completed processing all earlier messages and is already loose to take a brand new one.
- So now while you name him, as an alternative of having a hectic tone, you get to depart a message for Mr X, then look forward to him to name you again (no film time but).
- As soon as Mr X is completed with the entire queued up messages he won ahead of yours, he’ll glance into your factor, and name you again to come up with a solution.
Now right here lies the query: have been the movements thus far synchronous or asynchronous?
It’s combined. While you left your message, Mr X wasn’t listening in to it, so the forth verbal exchange was once asynchronous.
However, when he spoke back, you have been there listening, which makes the go back verbal exchange synchronous.
I’m hoping via now you’ve received a greater working out of the way synchronicity is perceived relating to verbal exchange. Time to usher in JavaScript.
JavaScript – An Asynchronous Programming Language
When any person labels JavaScript asynchronous, what they’re regarding on the whole is how you’ll go away a message for it, and no longer have your name blocked with a hectic tone.
The serve as calls are by no means direct in JavaScript, they’re actually finished by way of messages.
JavaScript makes use of a message queue the place incoming messages (or occasions) are held. An event-loop (a message dispatcher) sequentially dispatches the ones messages to a name stack the place the corresponding purposes of the messages are stacked as frames (serve as arguements & variables) for execution.
The decision stack holds the body of the preliminary serve as being referred to as, and some other frames for purposes referred to as by way of nested calls on best of it .
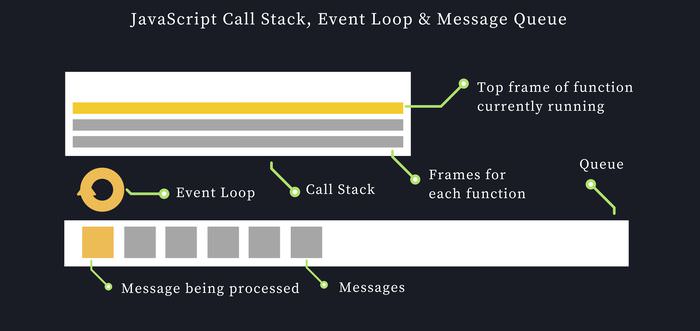
When a message joins the queue, it waits till the decision stack is empty of all frames from the former message, and when it’s, the event-loop dequeues the former message, and provides the corresponding frames of the present message to the decision stack.
The message waits once more till the decision stack turns into empty of its personal corresponding frames (i.e. the executions of the entire stacked purposes are over), then is dequeued.
Imagine the next code:
serve as foo(){} serve as bar(){ foo(); } serve as baz(){ bar(); } baz();
The serve as being run is baz()
(on the ultimate row of the code snippet), for which a message is added to the queue, and when the event-loop choices it up, the decision stack begins stacking frames for baz()
, bar()
, and foo()
on the related issues of execution.
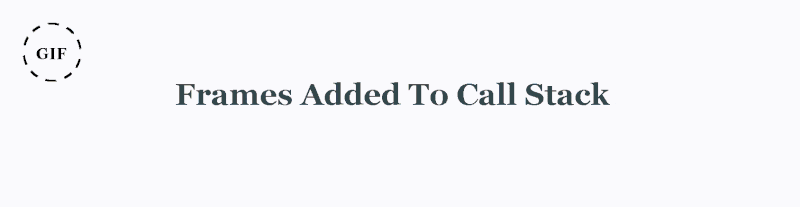
As soon as the execution of the purposes is whole one after the other, their frames are got rid of from the decision stack, whilst the message is nonetheless ready within the queue, till baz()
is popped from the stack.
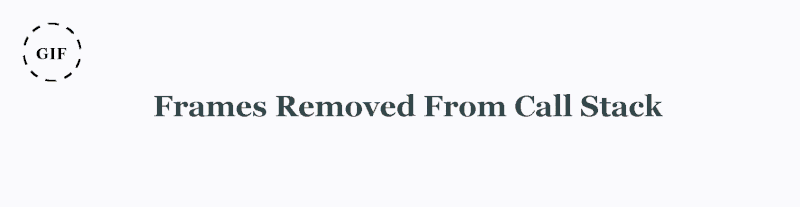
Be mindful, the serve as calls are by no means direct in JavaScript, they’re finished by way of messages. So on every occasion you listen any person say that JavaScript itself is an asynchronous programming language, suppose that they’re speaking about its integrated “answering gadget”, and the way you’re loose to depart messages.
However what concerning the explicit asynchronous strategies?
Up to now I’ve no longer touched on APIs similar to setTimeout()
and AJAX, the ones are those which can be particularly known as asynchronous. Why is that?
It’s necessary to grasp what precisely is being synchronous or asynchronous. JavaScript, with the assistance of occasions and the event-loop, would possibly apply asynchronous processing of messages, however that doesn’t imply the whole thing in JavaScript is asynchronous.
Be mindful, I advised you the message didn’t go away till the decision stack was once empty of its corresponding frames, similar to you didn’t go away for a film till you were given your resolution — that’s being synchronous, you’re there ready till the duty is whole, and also you get the solution.
Ready isn’t ultimate in all eventualities. What if after leaving a message, as an alternative of ready, you’ll go away for the film? What if a serve as can retire (emptying the decision stack), and its message will also be dequeued even ahead of the duty of the serve as is whole? What if you’ll have code accomplished asynchronously?
That is the place APIs similar to setTimeout()
and AJAX come into the image, and what they do is… hang on, I will’t give an explanation for this with out going again to Mr X, which we’ll see in the second one a part of this text. Keep tuned.
The submit Understanding Synchronous and Asynchronous JavaScript – Part 1 seemed first on Hongkiat.
WordPress Website Development Source: https://www.hongkiat.com/blog/synchronous-asynchronous-javascript/