Laravel’s templating engines reinforce React, Vue, and different libraries. But, builders love the Laravel Blade engine’s powerful skill to create modular and reusable perspectives temporarily. Blade allows you to without difficulty prolong layouts, outline sections, and use regulate buildings to generate data-driven content material.
This hands-on article explores what Blade is, the way it works, and the way it complements your Laravel programs.
The whole thing You Want To Use Laravel Blade
Laravel Blade is the default templating engine for the Laravel framework. It allows you to use variables, loops, conditional statements, and different PHP options immediately on your HTML code. To create Blade information, merely outline blade perspectives through growing information with the .blade.php
extension within the assets/perspectives listing of your Laravel task, then construction your most well-liked dynamic pages in those information.
Why Must You Use Blade?
One major good thing about Blade is its modular code group. Blade is helping arrange your code into reusable modules that you’ll simply upload, take away, or replace with out affecting the remainder of your software.
Code encapsulation is every other of Blade’s benefits. Blade is helping encapsulate purposes, making trying out, debugging, and code repairs extra manageable. This method advantages better programs since unorganized programs can temporarily transform difficult to regulate.
Blade’s templating engine is performant and the quickest PHP framework we examined. The engine compiles all of your blade perspectives into simple PHP code after which caches them till you alter them. This method promotes sooner rendering and total higher efficiency.
How To Use Laravel Blade
On this instructional, we create a Laravel software to enjoy Blade templates in motion. Discover ways to outline and prolong blade layouts, go information between blade perspectives, use the quite a lot of regulate buildings to be had, and create your individual blades.
Necessities
To observe this instructional, be sure to have the next:
- Prior familiarity with PHP
- Composer put in. Test that Composer is in your device through working
composer -V
Take a look at the entire instructional code for steering whilst operating.
How To Create the Laravel Utility
To create a pattern Laravel software, run:
composer create-project laravel/laravel my-app
Apply the set of directions in your terminal to complete making the app.
Subsequent, move into the app listing and serve it with this command:
cd my-app
php artisan serve
Click on the hyperlink within the terminal to open the Laravel Welcome web page in your browser.
How To Outline the Format
Layouts help you configure sections of your internet software to proportion throughout a couple of pages. For instance, in case your software has a constant navbar and footer throughout pages, it’s extra environment friendly to create it as soon as than construct it once more for each and every web page.
Earlier than operating thru step by step directions, check out the next skeleton demonstration.
The code for growing internet sites with out layouts is proven underneath. It makes you rewrite your navbar and footer code for each and every web page.
Content material for web page 1
Content material for web page 2
As an alternative, you’ll simply assemble your software the use of layouts to proportion the similar elements in one code block:
{slot}
Whenever you’ve outlined your structure, you’ll apply it to any web page you need:
Content material for web page n
In older Laravel variations, you needed to create layouts the use of template inheritance. On the other hand, when the corporate added the part characteristic, it turned into a lot more straightforward to create powerful layouts.
To create a brand new structure with Laravel Blade, first run this command to create the structure’s part:
php artisan make:part Format
This command generates a brand new structure.blade.php record positioned in a brand new folder referred to as elements within the assets/perspectives/ listing. Open that record and paste this code:
{{ $identify ?? 'Instance Web site' }}
{{ $slot }}
This code creates a structure part that has a easy navbar and footer. The slot
serve as defines the place to go the principle content material each time you prolong your structure part.
How To Lengthen the Format
You’ll be able to simply import an element in a blade view the use of the tag. This technique additionally applies to the structure part you created previous.
To peer how extending the structure seems to be, open the default assets/perspectives/welcome.blade.php record and exchange the record’s contents with this code:
Hi International!
Lorem ipsum dolor sit down amet consectetur adipiscing elit. Hic, aut?
This method updates the welcome web page to make use of the structure part whilst passing a heading part and a paragraph with some mock textual content as its content material. Reload the hyperlink you opened previous to view the adjustments.
To your structure definition, realize that the code rendered a identify information that defaults to “Instance Web site” if the code doesn’t go the identify information. You’ll be able to go this information as named slots on your perspectives by way of the
code — on this case,
. Replace the welcome.blade.php record with the code underneath and reload the web page:
House | Instance Web site
Hi International!
Lorem ipsum dolor sit down amet consectetur adipiscing elit. Hic, aut?
Subsequent, upload some styling to give a boost to your app’s look. Create a brand new app.css record throughout the /public/css listing, then paste the code from this record.
With a lot of these updates, you must see the next output whilst you preview your software at http://127.0.0.1:8000/.
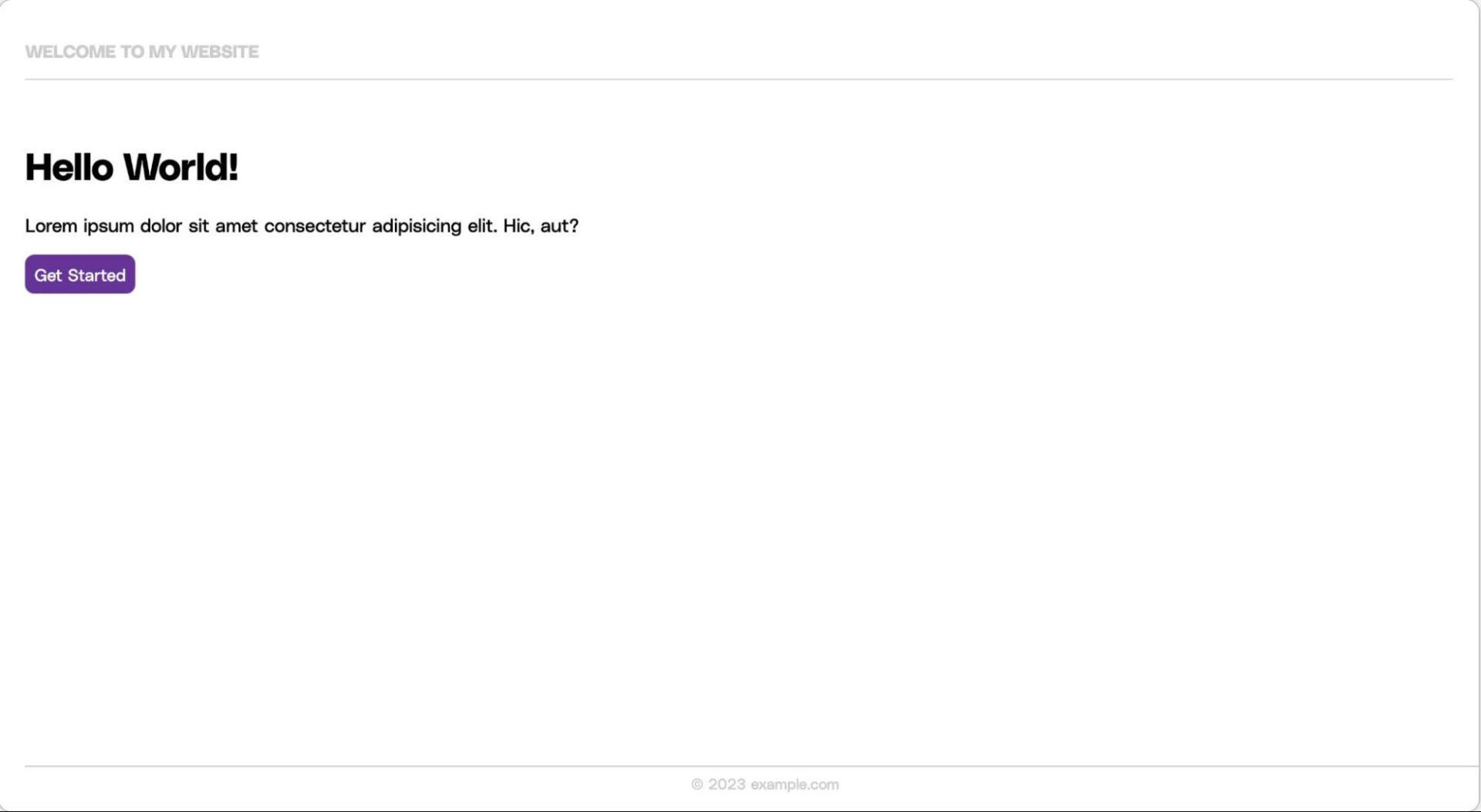
How To Come with Backend Knowledge
Within the earlier instance, you readily shared information between elements and perspectives by way of slots. On the other hand, there are higher approaches for loading information from a database or different far off supply.
If that’s the case, load and go the knowledge immediately out of your router definition and get admission to it such as you accessed a named slot within the earlier instance. To take action, open the routes/internet.php record and exchange its contents with this code:
$identify]);
});
Within the code above, you up to date your internet path to go the identify "John Doe"
to the welcome view. Now, get admission to this price on your blade perspectives like this:
Hi, {{ $identify }}
You might use this way to load information from a database. For example, assume you will have a to-dos desk containing a to-do listing. Use the DB facade to load those to-dos and go them in your view:
get();
go back view('welcome', ['todos' => $todos]);
});
On the other hand, when you don’t have a database, replace the internet access path to go an array of static to-dos. Open the routes/internet.php record and replace the default (/)
direction with the code underneath:
Course::get('/', serve as () {
$todos = ['Learn Laravel', 'Learn Blade', 'Build Cool Stuff'];
go back view('welcome', ['todos' => $todos]);
});
Change the content material of the welcome.blade.php record with the code underneath to get admission to the to-dos as an encoded JSON array.
House | Instance Web site
{{ json_encode($todos) }}
How To Use Keep watch over Shortcuts
The Blade templating engine additionally helps a couple of directives to render quite a lot of information sorts conditionally. For instance, to iterate throughout the returned to-dos array you had handed in your welcome view, practice a foreach
loop through pasting the next code within the welcome.blade.php record:
House | Instance Web site
@foreach ($todos as $todo)
- {{ $todo }}
@endforeach
This variation must render your to-dos in an unordered listing, just like the screenshot underneath.
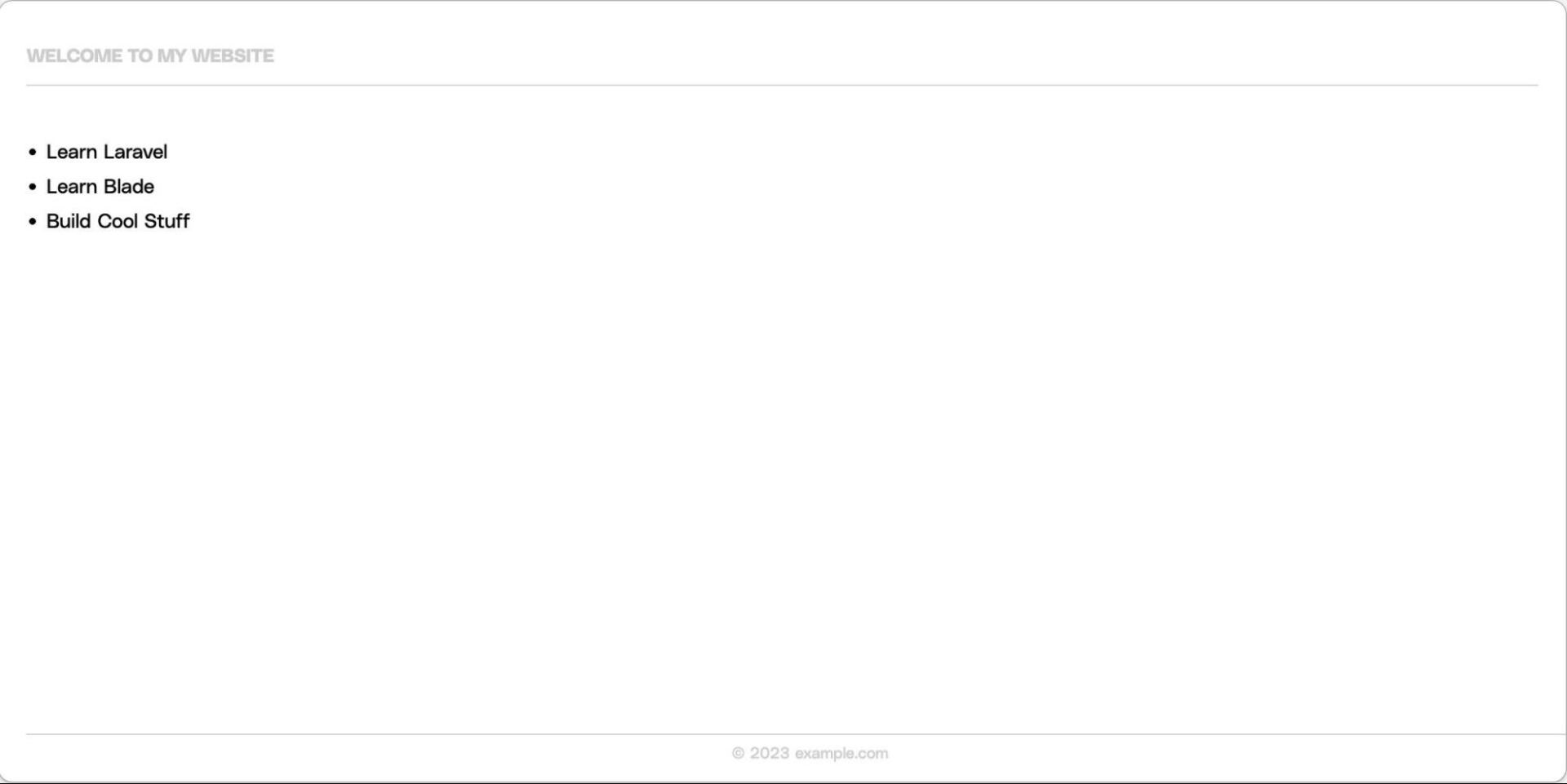
To build a block of conditional statements, use the @if
, @elseif
, @else
, and @endif
directives. For instance:
@if (depend($todos) === 1)
I've one activity!
@elseif (depend($todos) > 1)
I've a couple of duties!
@else
I do not have any duties!
@endif
Change the content material of the welcome.blade.php record with the code above. The quite a lot of if
–else
directives depend the to-do pieces and show a customized message for various situations. For the reason that you will have multiple activity on your to-dos array, you must see the output “I’ve a couple of duties!” within the browser.
You’ll be able to to find extra supported directives in Laravel’s documentation.
How To Make a Customized Extension
You’ll be able to write a customized directive, too, very similar to the former instance. To discover this system, create a customized text-truncating directive.
First, make a brand new provider supplier through working:
php artisan make:supplier TruncateTextServiceProvider
This command generates a brand new provider supplier record at app/Suppliers/TruncateTextServiceProvider.php. Open this record and exchange its contents with:
";
});
}
}
The code imports the Blade facade and defines a brand new customized directive referred to as @truncate
. The directive accepts two arguments: $textual content
and $duration
. It makes use of the Str::restrict()
way to truncate the textual content to the required duration.
In the end, sign in the provider supplier through including it in your suppliers’ array within the config/app.php configuration record:
'suppliers' => [
// Other service providers
AppProvidersTruncateTextServiceProvider::class,
],
Use the customized directive on your Blade templates (welcome.blade.php) through invoking it by way of the @truncate
syntax.
@truncate('Lorem ipsum dolor sit down amet', 10)
Abstract
This text explored how Laravel Blade allows you to streamline your building procedure whilst growing modular and reusable perspectives for internet programs. On the other hand, your Laravel building adventure shouldn’t finish there.
The infrastructure website hosting your software is as crucial in your luck as your native building procedure. To take your Laravel software to the following stage, you wish to have a competent website hosting platform that may deal with its calls for.
Kinsta optimizes website hosting answers for efficiency, safety, and scalability, enabling you to develop your software in the most productive imaginable setting. Deploy your Laravel app on Kinsta to enjoy it for your self.
The publish Figuring out Laravel Blade and How To Use It gave the impression first on Kinsta®.
WP Hosting