Unit checking out is the most important in instrument building, making sure your utility’s elements paintings as anticipated in isolation. By means of writing assessments for particular code gadgets, you’ll be able to determine and connect mistakes early in building, resulting in extra dependable and solid instrument.
In a continuing integration/steady supply (CI/CD) pipeline, you’ll be able to run those assessments mechanically after making codebase adjustments. This guarantees that new code doesn’t introduce mistakes or wreck present capability.
This newsletter highlights the significance of unit checking out in Laravel packages, detailing find out how to write unit assessments for a Laravel utility deployed the usage of Kinsta’s Software Internet hosting carrier.
Advent to PHPUnit
PHPUnit is a broadly used checking out framework throughout the PHP ecosystem designed for unit checking out. It has a powerful suite of gear for developing and operating assessments, making it a crucial useful resource for making sure your codebase’s reliability and high quality.
Laravel helps checking out with PHPUnit and ships with handy helper strategies that allow you to verify your utility.
Putting in place PHPUnit in a Laravel challenge comes to minimum configuration. Laravel supplies a preconfigured checking out setting, together with a phpunit.xml report and a devoted assessments listing on your verify recordsdata.
However, you’ll be able to alter the phpunit.xml report to outline customized choices for a adapted checking out revel in. You’ll additionally create a .env.checking out setting report within the challenge’s root folder as an alternative of the usage of the .env report.
Default assessments structure in Laravel
Laravel supplies a structured default listing structure. The basis listing of your Laravel challenge comprises a assessments listing with the Function and Unit subdirectories. This structure makes it easy to split other verify varieties and handle a blank and arranged checking out setting.
The phpunit.xml report in a Laravel challenge is the most important in orchestrating the checking out procedure, making sure consistency in verify runs, and permitting you to customise PHPUnit’s conduct in step with challenge necessities. It means that you can outline find out how to run assessments, together with defining the verify suites, specifying the verify setting, and putting in database connections.
This report additionally specifies that the consultation, cache, and e-mail will have to be set to the array driving force, making sure no knowledge consultation, cache, or e-mail knowledge persists when operating assessments.
You’ll carry out different types of checking out for your Laravel utility:
- Unit checking out — makes a speciality of person elements of your code, corresponding to categories, strategies, and purposes. Those assessments stay remoted from the Laravel utility and test that exact code gadgets paintings as anticipated. Observe that assessments outlined within the assessments/Unit listing don’t boot the Laravel utility, that means they are able to’t get right of entry to the database or different services and products the framework provides.
- Function checking out — validates the wider capability of your utility. Those assessments simulate HTTP requests and responses, letting you verify routes, controllers, and the mixing of more than a few elements. Function assessments lend a hand make certain that other portions of your utility paintings in combination as anticipated.
- Browser checking out — is going additional by means of automating browser interactions. The assessments use Laravel Nightfall, a browser automation and checking out instrument, to simulate person interactions, corresponding to filling out bureaucracy and clicking buttons. Browser assessments are the most important for validating your utility’s conduct and person revel in in real-world browsers.
Take a look at-driven building ideas
Take a look at-driven building (TDD) is a instrument building means emphasizing checking out ahead of enforcing code. This means follows a procedure referred to as the red-green-refactor cycle.
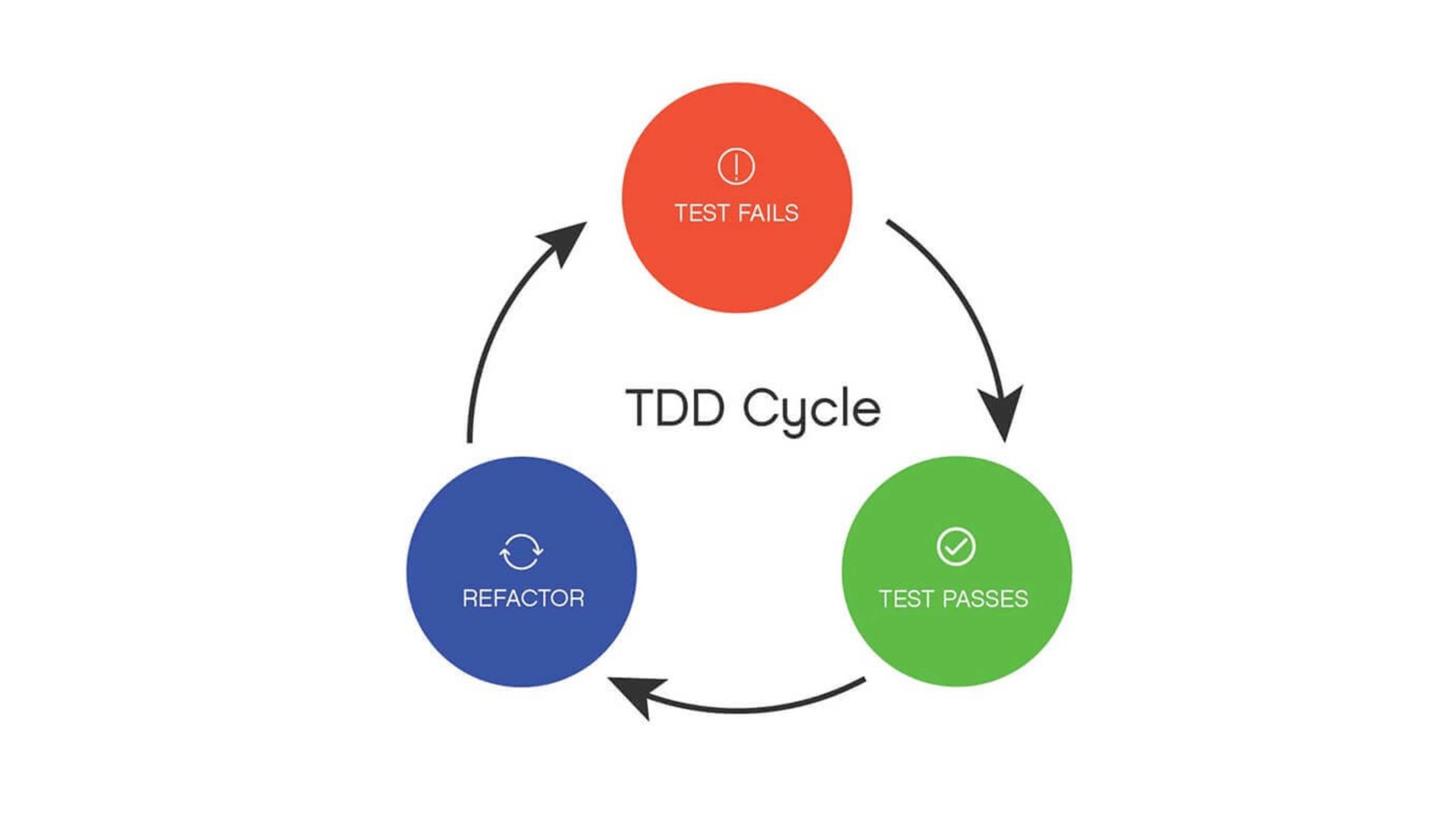
Here’s a proof of this cycle:
- Purple segment — Write a brand new verify to outline capability or an growth on an present one ahead of enforcing the true code. The verify will have to fail (as “crimson” indicates) as a result of there’s no corresponding code to make it go.
- Inexperienced segment — Write simply sufficient code to make the failing verify go, turning it from crimson to inexperienced. The code received’t be optimum, however it fulfills the necessities of the corresponding verify case.
- Refactor segment — Refactor the code to support its clarity, maintainability, and function with out converting its conduct. At this degree, you’ll be able to conveniently make adjustments to the code with out being worried about any regression problems, as the present verify circumstances catch them.
TDD has a number of advantages:
- Early worm detection — TDD is helping catch insects early within the building procedure, serving to scale back the fee and time of adjusting issues later within the building cycle.
- Progressed design — TDD encourages modular and loosely coupled code for higher instrument design. It encourages you to take into accounts the interface and part interactions ahead of implementation.
- Self belief in refactoring — You’ll optimistically refactor code, understanding that present assessments temporarily determine any regressions offered all through refactoring.
- Residing documentation — Take a look at circumstances function residing documentation by means of offering examples of ways the code will have to behave. This documentation is at all times up-to-the-minute since failing assessments point out problems within the code.
In Laravel building, you observe TDD ideas by means of writing assessments for elements like controllers, fashions, and services and products ahead of enforcing them.
Laravel’s checking out setting, together with PHPUnit, supplies handy strategies and assertions to facilitate TDD, making sure you’ll be able to create significant assessments and apply the red-green-refactor cycle successfully.
Elementary examples of unit checking out
This phase explains find out how to write a easy verify to test your type’s capability.
Must haves
To apply alongside, you wish to have the next:
- Meet the must haves indexed within the Laravel weblog information.
- A Laravel utility. This instructional makes use of the applying created within the information connected above. You’ll learn it and create the weblog utility, however in case you best want the supply code to enforce the assessments, apply the stairs beneath.
- Xdebug put in and configured with policy mode enabled.
Arrange the challenge
- Execute this command in a terminal window to clone the challenge.
git clone https://github.com/VirtuaCreative/kinsta-laravel-blog.git
- Transfer into the challenge folder and execute the
composer set up
command to put in challenge dependencies. - Rename the env.instance report to .env.
- Execute the
php artisan key:generate
command to generate an app key.
Create and run assessments
To start, be sure to have the challenge code for your gadget. The type you’ll be checking out is the Submit
type outlined within the app/Http/Fashions/Submit.php report. This type encompasses a number of fillable attributes, corresponding to name
, description
, and symbol
.
Your activity comes to crafting easy unit assessments for this type. One verifies that the attributes are correctly set, whilst any other examines mass project by means of making an attempt to assign a non-fillable characteristic.
- Execute the
php artisan make:verify PostModelFunctionalityTest --unit
command to create a brand new verify case. The--unit
possibility specifies that this can be a unit verify and saves it within the assessments/Unit listing. - Open the assessments/Unit/PostModelFunctionalityTest.php report and change the
test_example
serve as with this code:public serve as test_attributes_are_set_correctly() { // create a brand new put up example with attributes $put up = new Submit([ 'title' => 'Sample Post Title', 'description' => 'Sample Post Description', 'image' => 'sample_image.jpg', ]); // test in case you set the attributes appropriately $this->assertEquals('Pattern Submit Name', $post->name); $this->assertEquals('Pattern Submit Description', $post->description); $this->assertEquals('sample_image.jpg', $post->symbol); } public serve as test_non_fillable_attributes_are_not_set() { // Try to create a put up with further attributes (non-fillable) $put up = new Submit([ 'title' => 'Sample Post Title', 'description' => 'Sample Post Description', 'image' => 'sample_image.jpg', 'author' => 'John Doe', ]); // test that the non-fillable characteristic isn't set at the put up example $this->assertArrayNotHasKey('writer', $post->getAttributes()); }
This code defines two verify strategies.
The primary creates a
Submit
example with specified attributes and, the usage of theassertEquals
statement manner asserts that you just set thename
,description
, andsymbol
attributes appropriately.The second one manner makes an attempt to create a
Submit
example with an extra non-fillable characteristic (writer
) and asserts that this characteristic isn’t set at the type example the usage of theassertArrayNotHasKey
statement manner. - Make sure you upload the next
use
commentary in the similar report:use AppModelsPost;
- Run the
php artisan config:transparent
command to transparent the configuration cache. - To run those assessments, execute the next command:
php artisan verify assessments/Unit/PostModelFunctionalityTest.php
All assessments will have to go, and the terminal will have to show the effects and overall time to run the assessments.
Debug assessments
If assessments fail, you’ll be able to debug them by means of following those steps:
- Evaluate the mistake message within the terminal. Laravel supplies detailed error messages that pinpoint the issue. Sparsely learn the mistake message to know why the verify failed.
- Check up on the assessments and code you might be checking out to spot discrepancies.
- Make sure you correctly arrange the knowledge and dependencies required for the verify.
- Use debugging gear like Laravel’s dd() serve as to investigate cross-check variables and knowledge at particular issues to your verify code.
- After you have known the problem, make the important adjustments and rerun the assessments till they go.
Checks and databases
Laravel supplies a handy technique to arrange a checking out setting the usage of an in-memory SQLite database, which is rapid and doesn’t persist knowledge between verify runs. To configure the checking out database setting and write assessments that engage with the database, apply the stairs beneath:
- Open the phpunit.xml report and uncomment the next strains of code:
- Execute the
php artisan make:verify PostCreationTest --unit
command to create a brand new verify case. - Open the assessments/Unit/PostCreationTest.php report and change the
test_example
manner with the code beneath:public serve as testPostCreation() { // Create a brand new put up and reserve it to the database $put up = Submit::create([ 'title' => 'Sample Post Title', 'description' => 'Sample Post Description', 'image' => 'sample_image.jpg', ]); // Retrieve the put up from the database and assert its life $createdPost = Submit::in finding($post->identification); $this->assertNotNull($createdPost); $this->assertEquals('Pattern Submit Name', $createdPost->name); }
- Make sure you upload the next
use
commentary:use AppModelsPost;
These days, the
PostCreationTest
elegance extends thePHPUnitFrameworkTestCase
base elegance. The bottom elegance is repeatedly used for unit assessments when running with PHPUnit without delay, out of doors of Laravel, or when writing assessments for an element no longer tightly coupled with Laravel. On the other hand, you wish to have to get right of entry to the database, that means you should alter thePostCreationTest
elegance to increase theTestsTestCase
elegance.The latter elegance tailors the
PHPUnitFrameworkTestCase
elegance to Laravel packages. It supplies further capability and Laravel-specific setup, corresponding to database seeding and verify setting configuration. - Make sure you change the
use PHPUnitFrameworkTestCase;
commentary withuse TestsTestCase;
.Keep in mind that you put the checking out setting to make use of an in-memory SQLite database. So, you should migrate the database ahead of operating the assessments. Use theIlluminateFoundationTestingRefreshDatabase
trait to try this. This trait migrates the database if the schema isn’t up-to-the-minute and resets the database after every verify to make certain that the knowledge from the former verify does no longer intrude with next assessments. - Upload the next
use
commentary to the assessments/Unit/PostCreationTest.php report to include this trait to your code:use IlluminateFoundationTestingRefreshDatabase;
- Subsequent, upload the next line of code simply ahead of the
testPostCreation
manner:use RefreshDatabase;
- Run the
php artisan config:transparent
command to transparent the configuration cache. - To run this verify, execute the next command:
php artisan verify assessments/Unit/PostCreationTest.php
The assessments will have to go, and the terminal will have to show the verify effects and the full checking out time.
Function checking out
Whilst unit assessments test person utility elements in isolation, function assessments test better parts of the code, corresponding to how a number of items engage. Function checking out is important for a number of causes:
- Finish-to-end validation — Confirms that all of the function works seamlessly, together with the interactions amongst more than a few elements like controllers, fashions, perspectives, or even the database.
- Finish-to-end checking out — Covers all of the person waft from preliminary request to ultimate reaction, which is able to discover problems that unit assessments may pass over. This skill makes them precious for checking out person trips and sophisticated situations.
- Person revel in assurance — Mimics person interactions, serving to test a constant person revel in and that the function purposes as meant.
- Regression detection — Catches regressions and code-breaking adjustments when introducing new code. If an present function begins failing in a function verify, it indicators that one thing broke.
Now, create a function verify for the PostController
within the app/Http/Controllers/PostController.php report. You focal point at the retailer
manner, validating the incoming knowledge and developing and storing posts within the database.
The verify simulates a person developing a brand new put up thru a internet interface, making sure that the code shops the put up within the database and redirects the person to the Posts Index web page after introduction. To do that, apply those steps:
- Execute the
php artisan make:verify PostControllerTest
command to create a brand new verify case within the assessments/Options listing. - Open the assessments/Function/PostControllerTest.php report and change the
test_example
manner with this code:use RefreshDatabase; // Refresh the database after every verify public serve as test_create_post() { // Simulate a person developing a brand new put up in the course of the internet interface $reaction = $this->put up(direction('posts.retailer'), [ 'title' => 'New Post Title', 'description' => 'New Post Description', 'image' => $this->create_test_image(), ]); // Assert that the put up is effectively saved within the database $this->assertCount(1, Submit::all()); // Assert that the person is redirected to the Posts Index web page after put up introduction $response->assertRedirect(direction('posts.index')); } // Helper serve as to create a verify symbol for the put up personal serve as create_test_image() { // Create a ridicule symbol report the usage of Laravel's UploadedFile elegance $report = UploadedFile::faux()->symbol('test_image.jpg'); // Go back the trail to the brief symbol report go back $report; }
The
test_create_post
serve as simulates a person developing a brand new put up by means of creating aPOST
request to theposts.retailer
direction with particular attributes, together with a ridicule symbol generated the usage of Laravel’sUploadedFile
elegance.The verify then asserts that the code effectively saved the put up within the database by means of checking the rely of
Submit::all()
. It verifies that the code redirects the person to the Posts Index web page after put up introduction.This verify guarantees that the post-creation capability works and the applying appropriately handles the database interactions and redirects after post-submission.
- Upload the next
use
statements to the similar report:use AppModelsPost; use IlluminateHttpUploadedFile;
- Run the command
php artisan config:transparent
to transparent the configuration cache. - To run this verify, execute this command:
php artisan verify assessments/Function/PostControllerTest.php
The verify will have to go, and the terminal will have to display the verify effects and the full time to run the verify.
Ascertain verify policy
Take a look at policy refers to how a lot of the codebase your unit, function, or browser assessments test, expressed as a share. It is helping you determine untested spaces to your codebase and the under-tested spaces probably containing insects.
Gear like PHPUnit’s code policy function and Laravel’s integrated policy file generate experiences appearing which portions of your codebase your assessments quilt. This procedure supplies crucial details about your assessments’ high quality and is helping you focal point on spaces that may require further checking out.
Generate a file
- Delete the assessments/Function/ExampleTest.php and assessments/Unit/ExampleTest.php recordsdata, as you haven’t changed them, and they’d reason mistakes.
- Execute the
php artisan verify --coverage
command in a terminal window. You will have to obtain an output like the next:php artisan verify –coverage. It presentations the full selection of assessments that handed and the time to execute the effects. It additionally lists every part to your codebase and its code policy share.” width=”1001″ peak=”471″ /> Executing the command
php artisan verify --coverage
.The code policy file presentations the verify effects, the full selection of assessments handed, and the time to execute the effects. It additionally lists every part to your codebase and its code policy share. The odds constitute the percentage of the code your assessments quilt. For instance,Fashions/Submit
has 100% policy, that means that all of the type’s strategies and contours of code are lined. The code policy file additionally shows the General Protection — the whole code policy for all of the codebase. On this case, the assessments quilt best 65.3% of the code. - To specify a minimal policy threshold, execute the
php artisan verify --coverage --min=85
command. This command units a minimal threshold of 85%. You will have to obtain the next output:
Take a look at with a minimal threshold of 85%. The verify suites fail since the code doesn’t meet the set minimal threshold of 85%.
Whilst attaining upper code policy — frequently 100% — is the purpose, it’s extra essential to check your utility’s crucial and sophisticated portions totally.
Abstract
By means of embracing the most productive practices defined on this article, corresponding to writing significant and complete assessments, adhering to the red-green-refactor cycle in TDD, and leveraging the checking out options equipped by means of Laravel and PHPUnit, you’ll be able to create powerful and top of the range packages.
Moreover, you have got the approach to host your Laravel utility with Kinsta’s swift, safe, and constant infrastructure. Moreover, you’ll be able to make the most of the Kinsta API to start up deployments inside of your CI/CD pipelines thru platforms corresponding to GitHub Movements, CircleCI, and extra.
The put up Don’t skip the unit checking out and construct higher Laravel apps seemed first on Kinsta®.
WP Hosting