WordPress is without doubt one of the global’s most well liked content material control methods (CMSs), serving to small and big companies arrange and create more than a few types of website online content material. However WordPress has advanced previous simply supporting conventional weblog content material — and that’s in large part due to the WordPress REST API.
The WordPress REST API connects WordPress and different exterior internet programs. It facilitates extra obtainable communique and is helping you construct immersive, enticing internet reports that combine seamlessly with the CMS platform.
This API makes use of endpoints to retrieve and manipulate WordPress content material as JSON items. With those endpoints, you’ll be able to create, learn, replace, and delete (CRUD) WordPress content material remotely with out logging into the WordPress Admin account, bringing flexibility and lengthening WordPress’s capability past its core options.
This information explores what the WordPress REST API is, its advantages, the way it extends WordPress’s base features, and learn how to create, sign in, and get right of entry to a customized endpoint.
Necessities
To practice this educational, you wish to have:
- Wisdom of REST API
- A WordPress website setup (you’ll be able to arrange one simply with the MyKinsta dashboard)
- Wisdom of the usage of WordPress and PHP
Working out the WordPress REST API
The WordPress REST API is an impressive interface that lets you have interaction with WordPress websites programmatically the usage of usual HTTP strategies.
Its default features come with getting access to and manipulating more than a few varieties of WordPress information, equivalent to posts, pages, feedback, customers, and taxonomies, in a structured JSON layout. You’ll be able to additionally remotely carry out CRUD movements on content material.
Then again, the WordPress REST API’s true price lies in its extensibility via customized endpoints. You’ll be able to create customized endpoints to tailor the API to express wishes, equivalent to integrating further functionalities, third-party services and products, or distinctive information buildings. This pliability empowers you to construct extremely custom designed and feature-rich programs on most sensible of WordPress.
How you can plan your customized API endpoint
Making plans the construction and objective of your customized endpoints is vital for environment friendly API building. Customized endpoints adapted for your explicit wishes require cautious attention to make sure optimum capability. Strategic making plans facilitates scalability and suppleness, future-proofing endpoints to deal with evolving industry necessities.
Making plans your customized API endpoints sooner than implementation helps:
- Readability of endpoint serve as — Making plans your endpoint clarifies the endpoint’s explicit serve as, its anticipated information varieties, and utilization.
- Consistency and building potency — Making plans additionally guarantees consistency in the usage of the endpoints, reaction varieties, and formatting, which improves interplay with the API. Moreover, figuring out the API’s objective allows correct implementation, decreasing building time and the chance of mistakes.
- Scalability and suppleness — Defining the wishes of your endpoint is helping future-proof it to deal with converting industry wishes and necessities with out requiring an entire redesign.
- Safety—Right kind endpoint making plans is helping resolve the will for authentication to get right of entry to or manipulate information. Getting content material by way of the API occasionally comes to no consumer authentication. Nonetheless, for content material containing delicate or unauthorized information, it’s crucial to outline safety necessities and enforce measures like authorization and get right of entry to controls to lend a hand make certain information safety.
The hands-on sections that practice provide an explanation for how you’ll be able to create a customized endpoint this is to be had at site-domain/wp-json/customized/v2/testimonials
to retrieve buyer testimonials from a WordPress database website.
After sending a request, the endpoint returns a JSON object containing details about the testimonials to your WordPress website as outlined on your callback serve as.
Let’s dive in!
Create a customized submit sort on your endpoint
First, you wish to have to create a customized submit sort.
- Navigate to Theme Record Editor from the Look segment of your WordPress Admin dashboard.
- Open your theme’s serve as.php document and upload the next code:
serve as create_custom_testimonial_type() { register_post_type('testimonials', array( 'labels' => array( 'identify' => 'Testimonials', 'singular_name' => 'Testimonial', ), 'public' => true, 'has_archive' => true, 'show_in_rest' => true, // This allows REST API improve )); } add_action('init', 'create_custom_testimonial_type');
This code creates a customized “testimonials” submit sort and allows the WordPress REST API improve (
'show_in_rest' => true
). Theadd_action hook
calls thecreate_testimonial_type
callback serve as and launches it all through execution.You’ll be able to customise the labels and arguments by means of taking away or including them to cater for your wishes.
- Click on Replace document to save lots of your adjustments.
Making a custom_testimonial
submit sort.Refresh the dashboard to peer the Testimonials possibility added for your WordPress dashboard.
The newly created testimonial submit sort. - Create a brand new submit containing testimonials by means of clicking Testimonials > Upload New Submit. You’ll be able to use the Pullquote block. Relying on the way you provide your testimonial, you’ll be able to additionally use different blocks.
Listed below are two pattern testimonials created the usage of the Pullquote blocks:
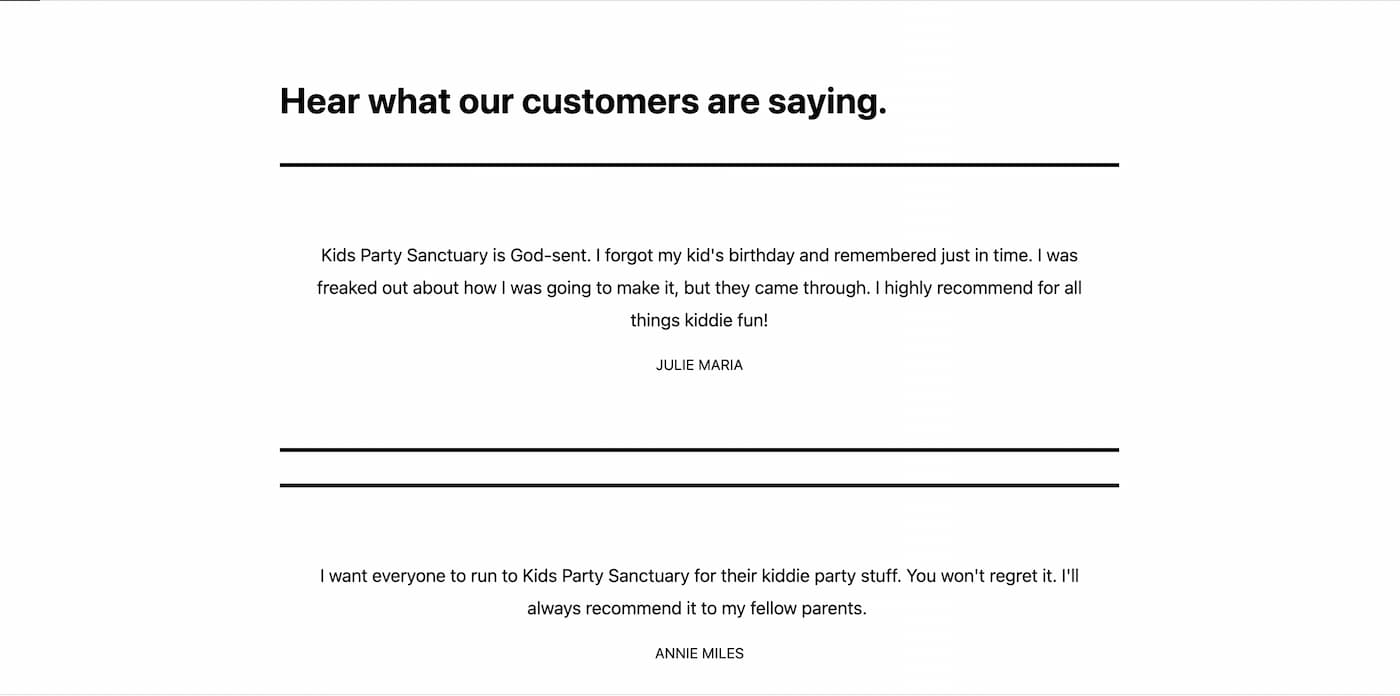
Sign up a customized endpoint in WordPress
Registering a customized endpoint makes it to be had for intake by way of the REST API. This comes to the usage of the register_rest_route
serve as, calling it at the rest_api_init
hook, and offering a callback means that can be invoked when the direction is named.
Paste the next code into your theme’s serve as.php document:
add_action( 'rest_api_init', 'register_testimonial_rest_route' );
serve as register_testimonial_rest_route(){
register_rest_route(
'customized/v2',
'/testimonials',
array(
'strategies' => 'GET',
'callback' => 'get_testimonials',
)
);
}
This register_rest_route()
takes in 3 parameters:
- Path Namespace (
$route_namespace
) — That is the primary a part of the URL section and must practice the seller/model quantity trend. The seller represents the seller or theme slug. The namespace is helping differentiate endpoints and is helping consumers touch improve on your customized endpoint. This educational makes use of thecustomized/v2
namespace. - The bottom URL (
$direction
) — This follows the namespace and is a URL mapped to one way. You’ll be able to sign in multiple unmarried endpoint on your direction. For this educational, you employ the/testimonials
direction, which tells the endpoint to retrieve testimonials. - The endpoint’s choices (
$args
) — Right here, that is an array containing the HTTP means used when calling the direction and the callback serve as the endpoint will invoke while you ship a request. We’ll cross over this callback serve as within the subsequent segment.
After all, word your endpoint deal with. The layout of an endpoint is site-address/wp-json/namespace/direction
. So, on this instance, the endpoint can be https://www.staging.kidspartysanctuary.co.united kingdom/wp-json/customized/v2/testimonials
.
Put in force the callback serve as for the endpoint
After developing the customized submit sort and registering your customized endpoint, your next step is to put in writing your callback serve as. This callback serve as is invoked each and every time the endpoint is accessed.
- Claim your
get_testimonials
callback serve as the usage of the code underneath:serve as get_testimonials(){ }
- Initialize an empty testimonials array to retailer the retrieved WordPress testimonial information:
$testimonials = array();
- Arrange an array named
$args
with question parameters for aWP_Query
name.$args = array( 'post_type' => 'testimonials', //specifies you need to question the customized submit sort 'testimonial', 'nopaging' => true, // no pagination, however retrieve all testimonials without delay ),
- Create an example of the
WP_Query
elegance that takes within the$args
array, plays a question in accordance with the desired parameters and retail outlets the WordPress question’s leads to the$question
variable.$question = new WP_Query($args)
- Write a conditional observation to test if there are any testimonial posts. Then, create a
whilst
loop to iterate in the course of the posts and go back the testimonials submit’sidentify
andcontent material
.if ( $query->have_posts() ) { whilst ( $query->have_posts() ) { $query->the_post(); $testimonial_data = array( /*an array that retail outlets the identify and content material of each and every submit*/ 'identify' => get_the_title(), 'content material' => get_the_content(), // Upload different fields as wanted ); $testimonials[] = $testimonial_data; } wp_reset_postdata(); /* restores $submit international to the present submit to keep away from any conflicts in next queries*/ } go back rest_ensure_response( $testimonials ); /*guarantees reaction is as it should be set as a reaction object for consistency*/
Right here’s the whole code:
serve as get_testimonials() { $testimonials = array(); $args = array( 'post_type' => 'testimonials', 'nopaging' => true, ); $question = new WP_Query( $args ); if ( $query->have_posts() ) { whilst ( $query->have_posts() ) { $query->the_post(); $testimonial_data = array( 'identify' => get_the_title(), 'content material' => get_the_content(), // Upload different fields as wanted ); $testimonials[] = $testimonial_data; } wp_reset_postdata(); } go back rest_ensure_response( $testimonials ); }
- Check your endpoint the usage of Postman to make sure if you’ll be able to get right of entry to your information.
Postman appearing a a hit reaction. You’ll be able to additionally check this the usage of a browser. Get entry to the endpoint by means of getting into the URL
site-domain/wp-json/customized/v2/testimonials
on your browser’s deal with bar.The browser outcome that looks when the endpoint is accessed.
Abstract
This educational has defined learn how to enforce a WordPress API customized endpoint. To allow customers to get right of entry to and have interaction together with your WordPress database information, you simply want to sign in the direction that implements a callback serve as.
Wish to know the way to maximise WordPress for what you are promoting? Kinsta gives a lot of complex choices as a part of our controlled WordPress Web hosting carrier to lend a hand serve your distinctive industry wishes. A notable possibility is the Kinsta MU (must-use) plugin, which manages and implements caching on your website to scale back latency and toughen efficiency. Check out Kinsta these days!
What are your perspectives on growing customized WordPress API endpoints? Have you ever ever designed any? We’d love to listen to about your reports. Percentage them within the feedback segment underneath.
The submit Customizing WordPress for builders: growing customized REST API endpoints seemed first on Kinsta®.
WP Hosting