Within the dynamic panorama of generation, the place innovation frequently shapes the limits of what’s imaginable, synthetic intelligence (AI) by no means ceases to captivate our creativeness.
AI refers back to the simulation of human intelligence processes through laptop techniques. Those processes come with duties corresponding to studying, reasoning, problem-solving, belief, language working out, and decision-making.
Nowadays, folks and firms have advanced and educated a number of AI fashions to accomplish sure duties higher than people in real-time. A number of the myriad programs of AI, one in particular intriguing space is AI-powered symbol era.
What You’re Construction
This information explains how you can construct a React software that seamlessly integrates with the OpenAI DALL-E API by means of a Node.js backend and generates charming photographs according to textual activates.
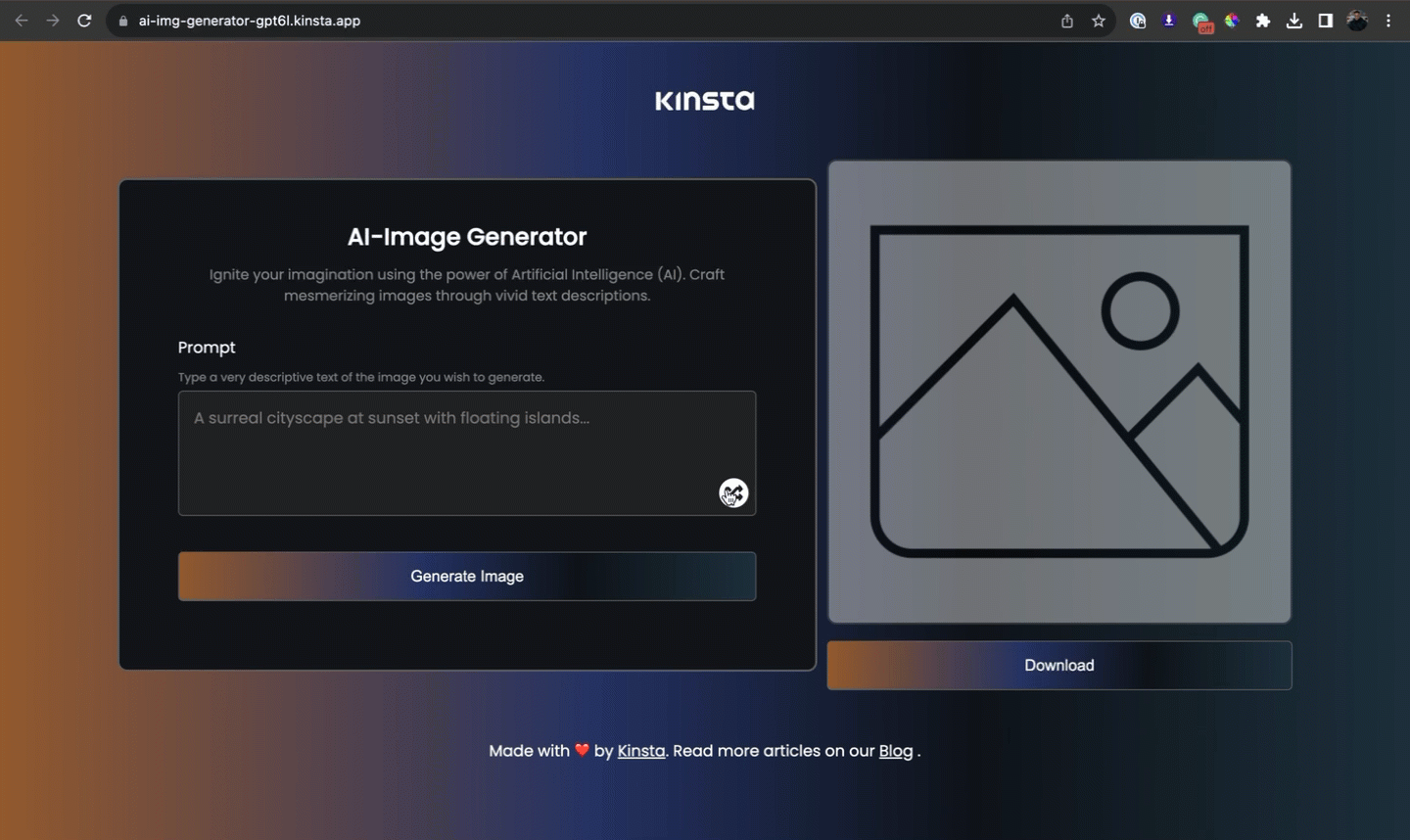
Must haves
To apply together with this undertaking, you’ll have:
- Elementary working out of HTML, CSS, and JavaScript
- Fundamental wisdom of React and Node.js
- Node.js and npm (Node Bundle Supervisor) or yarn put in for your laptop
What’s OpenAI DALL-E API?
OpenAI API is a cloud-based platform that grants builders get admission to to OpenAI’s pre-trained AI fashions, corresponding to DALL-E and GPT-3 (we used this type to construct a ChatGPT clone with the code in this Git repository). It lets in builders so as to add AI options corresponding to summarization, translation, symbol era, and amendment to their systems with out creating and coaching their fashions.
To make use of OpenAI API, create an account the use of your Google account or e mail at the OpenAI web site and procure an API key. To generate an API key, click on Private on the top-right nook of the web site, then make a choice View API keys.
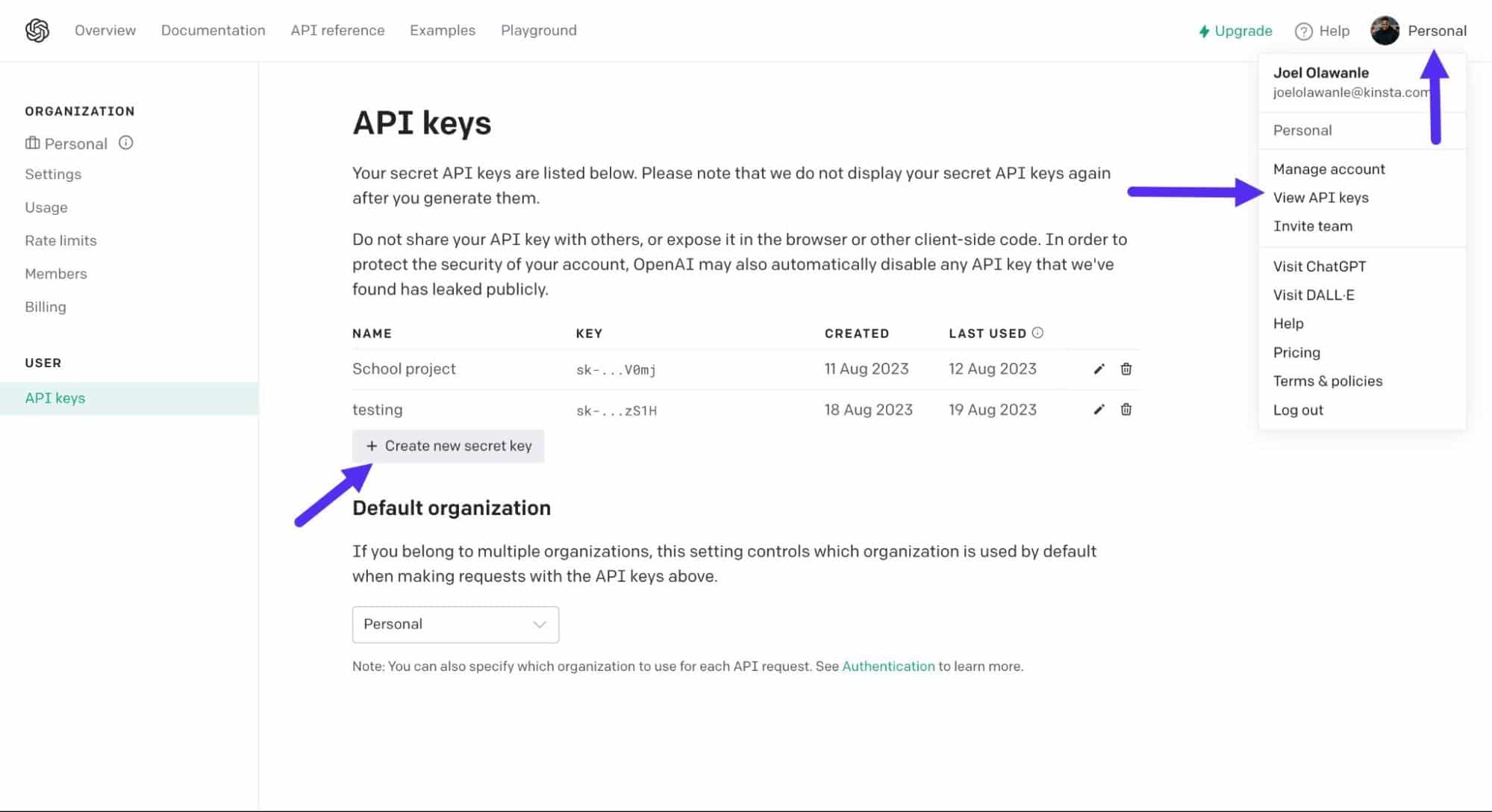
Click on the Create new secret key button, and save the important thing someplace. You are going to use it on this software to have interaction with the OpenAI’s DALL-E API.
Atmosphere Up the Building Surroundings
You’ll be able to create a React software from scratch and broaden your individual interface, or you’ll be able to snatch our Git starter template through following those steps:
- Discuss with this undertaking’s GitHub repository.
- Choose Use this template > Create a brand new repository to replicate the starter code right into a repository inside your GitHub account (take a look at the field to come with all branches).
- Pull the repository in your native laptop and turn to the starter-files department the use of the command:
git checkout starter-files
.
- Set up the essential dependencies through working the command
npm set up
.
As soon as the set up is entire, you’ll be able to release the undertaking for your native laptop with npm run get started
. This makes the undertaking to be had at http://localhost:3000/.
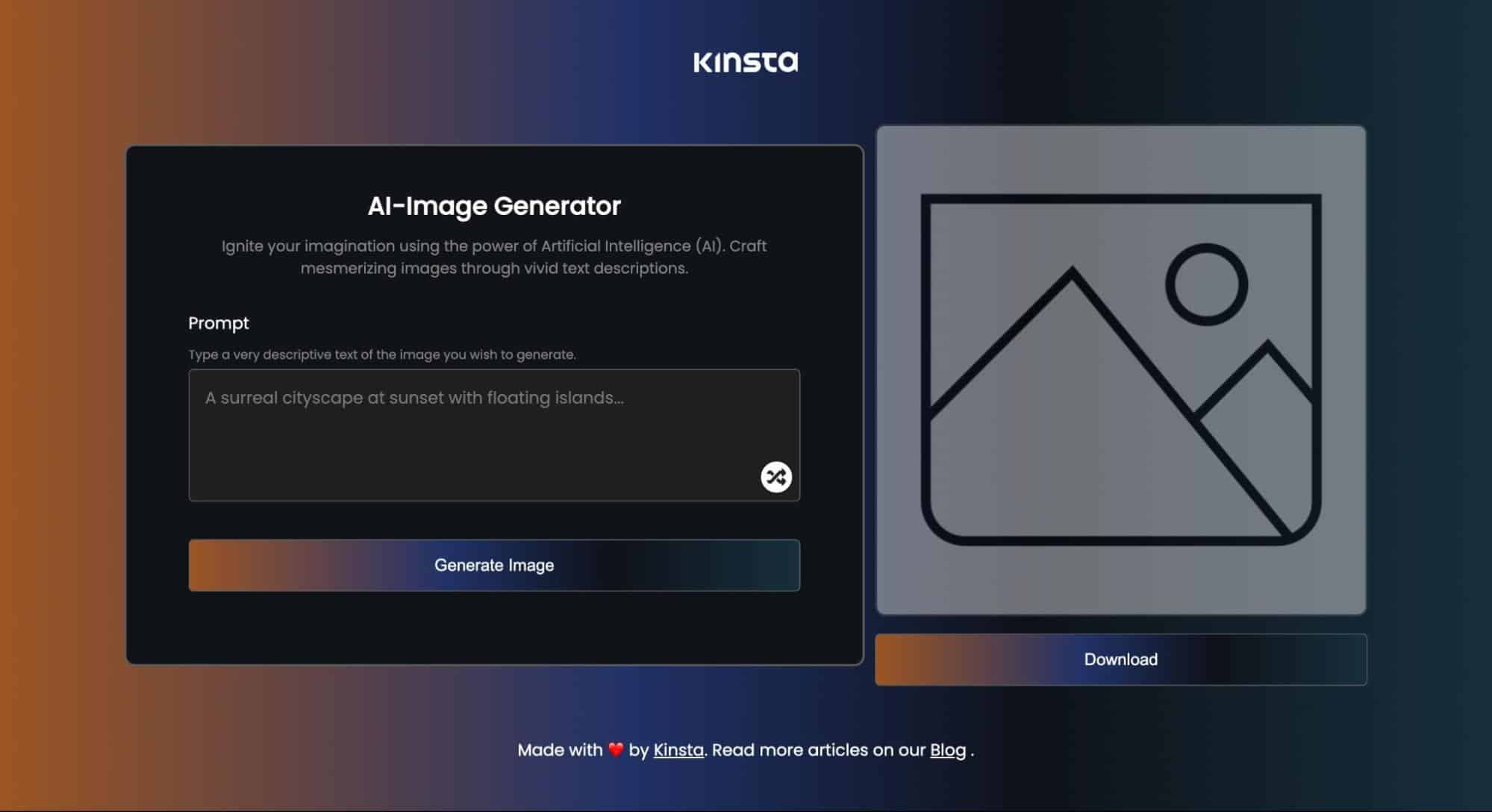
Figuring out the Mission Recordsdata
On this undertaking, we’ve added the entire essential dependencies to your React software. Right here’s an outline of what’s been put in:
- file-server: This application library simplifies the method of downloading the generated photographs. It’s related to the obtain button, making sure a clean consumer enjoy.
- uuid: This library assigns a novel identity to every symbol. This prevents any probability of pictures sharing the similar default dossier title, keeping up order and readability.
- react-icons: Built-in into the undertaking, this library without difficulty comprises icons, bettering the visible attraction of your software.
On the core of your React software lies the src folder. That is the place the very important JavaScript code for Webpack is housed. Let’s perceive the recordsdata and folders within the src folder:
- belongings: Inside this listing, you’ll in finding the pictures and loader gif which are applied all through the undertaking.
- knowledge: This folder comprises an index.js dossier that exports an array of 30 activates. Those activates can be utilized to generate various and random photographs. Be happy to edit it.
- index.css: That is the place the kinds used on this undertaking are saved.
Figuring out the Utils Folder
Inside of this folder, the index.js dossier defines two reusable purposes. The primary serve as randomizes the collection of activates describing more than a few photographs that may be generated.
import { randomPrompts } from '../knowledge';
export const getRandomPrompt = () => {
const randomIndex = Math.flooring(Math.random() * randomPrompts.duration);
const randomPrompt = randomPrompts[randomIndex];
go back randomPrompt;
}
The second one serve as handles the obtain of the generated photographs through leveraging the file-saver dependency. Each purposes are created to provide modularity and potency, and they may be able to be very easily imported into elements when required.
import FileSaver from 'file-saver';
import { v4 as uuidv4 } from 'uuid';
export async serve as downloadImage(picture) {
const _id = uuidv4();
FileSaver.saveAs(picture, `download-${_id}.jpg`);
}
Within the code above, the uuid dependency offers every generated symbol dossier a novel ID, so that they don’t have the similar dossier title.
Figuring out the Elements
Those are small blocks of code separated to make your code simple to care for and perceive. For this undertaking, 3 elements have been created: Header.jsx, Footer.jsx, and Shape.jsx. The most important part is the Shape part, the place the enter is gained and handed to the App.jsx dossier with the generateImage
serve as added as an onClick
match to the Generate Symbol button.
Within the Shape part, a state is created to retailer and replace the recommended. Moreover, a characteristic permits you to click on on a random icon to generate the random activates. That is imaginable through the handleRandomPrompt
serve as, which makes use of the getRandomPrompt
serve as you’ve already arrange. While you click on the icon, it fetches a random recommended and updates the state with it:
const handleRandomPrompt = () => {
const randomPrompt = getRandomPrompt();
setPrompt(randomPrompt)
}
Figuring out the App.jsx Record
That is the place lots of the code is living. The entire elements are introduced in combination right here. There’s additionally a delegated space to show the generated symbol. If no symbol has been generated but, a placeholder symbol (Preview symbol) is displayed.
Inside of this dossier, two states are controlled:
isGenerating
: This helps to keep observe of whether or not a picture is recently being generated. By way of default, it’s set to false.generatedImage
: This state retail outlets details about the picture that has been generated.
Moreover, the downloadImage
application serve as is imported, permitting you to cause the obtain of the generated symbol while you click on the Obtain button:
Now that the starter recordsdata and feature arrange your undertaking. Let’s get started dealing with the common sense of this software.
Producing Photographs With OpenAI’s DALL-E API
To harness the functions of OpenAI’s DALL-E API, you’ll use Node.js to ascertain a server. Inside this server, you’ll create a POST path. This path can be liable for receiving the recommended textual content despatched out of your React software after which using it to generate a picture.
To get began, set up the essential dependencies to your undertaking listing through working the next command:
npm i specific cors openai
Moreover, set up the next dependencies as dev dependencies. Those equipment will help in putting in your Node.js server:
npm i -D dotenv nodemon
The put in dependencies are defined as follows:
- specific: This library is helping create a server in Node.js.
- cors: CORS facilitates safe verbal exchange between other domain names.
- openai: This dependency grants you get admission to to OpenAI’s DALL-E API.
- dotenv: dotenv assists in managing surroundings variables.
- nodemon: nodemon is a construction instrument that screens adjustments to your recordsdata and mechanically restarts the server.
As soon as the installations are a success, create a server.js dossier on the root of your undertaking. That is the place all of your server code can be saved.
Within the server.js dossier, import the libraries you simply put in and instantiate them:
// Import the essential libraries
const specific = require('specific');
const cors = require('cors');
require('dotenv').config();
const OpenAI = require('openai');
// Create an example of the Specific software
const app = specific();
// Allow Go-Beginning Useful resource Sharing (CORS)
app.use(cors());
// Configure Specific to parse JSON knowledge and set an information restrict
app.use(specific.json({ restrict: '50mb' }));
// Create an example of the OpenAI magnificence and supply your API key
const openai = new OpenAI({
apiKey: procedure.env.OPENAI_API_KEY,
});
// Outline a serve as to start out the server
const startServer = async () => {
app.pay attention(8080, () => console.log('Server began on port 8080'));
};
// Name the startServer serve as to start out listening at the specified port
startServer();
Within the code above, you import the essential libraries. Then, determine an example of the Specific software the use of const app = specific();
. In a while, permit CORS. Subsequent, Specific is configured to procedure incoming JSON knowledge, specifying an information measurement restrict of 50mb
.
Following this, an example of the OpenAI magnificence is created using your OpenAI API key. Create a .env dossier to your undertaking’s root and upload your API key the use of the OPENAI_API_KEY
variable. In any case, you outline an asynchronous startServer
serve as and make contact with it to set the server in movement.
Now you will have configured your server.js dossier. Let’s create a POST path that you’ll be able to use to your React software to have interaction with this server:
app.publish('/api', async (req, res) => {
take a look at {
const { recommended } = req.frame;
const reaction = look ahead to openai.photographs.generate({
recommended,
n: 1,
measurement: '1024x1024',
response_format: 'b64_json',
});
const symbol = reaction.knowledge[0].b64_json;
res.standing(200).json({ picture: symbol });
} catch (error) {
console.error(error);
}
});
On this code, the path is about to /api
, and it’s designed to deal with incoming POST requests. Throughout the path’s callback serve as, you obtain the knowledge despatched out of your React app the use of req.frame
— particularly the recommended
worth.
Therefore, the OpenAI library’s photographs.generate
manner is invoked. This technique takes the supplied recommended and generates a picture in reaction. Parameters like n
resolve the collection of photographs to generate (right here, only one), measurement
specifies the size of the picture, and response_format
signifies the structure during which the reaction must be supplied (b64_json
on this case).
After producing the picture, you extract the picture knowledge from the reaction and retailer it within the symbol
variable. Then, you ship a JSON reaction again to the React app with the generated symbol knowledge, environment the HTTP standing to 200
(indicating good fortune) the use of res.standing(200).json({ picture: symbol })
.
In case of any mistakes all through this procedure, the code throughout the catch
block is performed, logging the mistake to the console for debugging.
Now the server is in a position! Let’s specify the command that will be used to run our server within the package deal.json dossier scripts
object:
"scripts": {
"dev:frontend": "react-scripts get started",
"dev:backend": "nodemon server.js",
"construct": "react-scripts construct",
},
Now while you run npm run dev:backend
, your server will get started on http://localhost:8080/, whilst when you run npm run dev:frontend
, your React software will get started on http://localhost:3000/. Make certain each are working in numerous terminals.
Make HTTP Requests From React to Node.js Server
Within the App.jsx dossier, you’re going to create a generateImage
serve as this is caused when the Generate Symbol button is clicked within the Shape.jsx part. This serve as accepts two parameters: recommended
and setPrompt
from the Shape.jsx part.
Within the generateImage
serve as, make an HTTP POST request to the Node.js server:
const generateImage = async (recommended, setPrompt) => {
if (recommended) {
take a look at {
setIsGenerating(true);
const reaction = look ahead to fetch(
'http://localhost:8080/api',
{
manner: 'POST',
headers: {
'Content material-Sort': 'software/json',
},
frame: JSON.stringify({
recommended,
}),
}
);
const knowledge = look ahead to reaction.json();
setGeneratedImage({
picture: `knowledge:symbol/jpeg;base64,${knowledge.picture}`,
altText: recommended,
});
} catch (err) {
alert(err);
} after all {
setPrompt('');
setIsGenerating(false);
}
} else {
alert('Please supply correct recommended');
}
};
Within the code above, you take a look at if the recommended
parameter has a price, then set the isGenerating
state to true
because the operation is beginning. This may increasingly make the loader seem at the display as a result of within the App.jsx dossier, we have now this code controlling the loader show:
{isGenerating && (
className="loader-comp">
)}
Subsequent, use the fetch()
strategy to make a POST request to the server the use of http://localhost:8080/api — that is why we put in CORS as we’re interacting with an API on every other URL. We use the recommended because the frame of the message. Then, extract the reaction returned from the Node.js server and set it to the generatedImage
state.
As soon as the generatedImage
state has a price, the picture can be displayed:
{generatedImage.picture ? (
) : (
)}
That is how your entire App.jsx dossier will glance:
import { Shape, Footer, Header } from './elements';
import preview from './belongings/preview.png';
import Loader from './belongings/loader-3.gif'
import { downloadImage } from './utils';
import { useState } from 'react';
const App = () => {
const [isGenerating, setIsGenerating] = useState(false);
const [generatedImage, setGeneratedImage] = useState({
picture: null,
altText: null,
});
const generateImage = async (recommended, setPrompt) => {
if (recommended) {
take a look at {
setIsGenerating(true);
const reaction = look ahead to fetch(
'http://localhost:8080/api',
{
manner: 'POST',
headers: {
'Content material-Sort': 'software/json',
},
frame: JSON.stringify({
recommended,
}),
}
);
const knowledge = look ahead to reaction.json();
setGeneratedImage({
picture: `knowledge:symbol/jpeg;base64,${knowledge.picture}`,
altText: recommended,
});
} catch (err) {
alert(err);
} after all {
setPrompt('');
setIsGenerating(false);
}
} else {
alert('Please supply correct recommended');
}
};
go back (
{generatedImage.picture ? (
) : (
)}
{isGenerating && (
)}
);
};
export default App;
Deploy Your Complete-Stack Software to Kinsta
To this point, you will have effectively constructed a React software that interacts with Node.js, which makes it a full-stack software. Let’s now deploy this software to Kinsta.
First, configure the server to serve the static recordsdata generated all through the React software’s construct procedure. That is accomplished through uploading the trail
module and the use of it to serve the static recordsdata:
const trail = require('trail');
app.use(specific.static(trail.get to the bottom of(__dirname, './construct')));
While you execute the command npm run construct && npm run dev:backend
, your complete stack React software will load at http://localhost:8080/. It’s because the React software is compiled into static recordsdata throughout the construct folder. Those recordsdata are then integrated into your Node.js server as a static listing. Because of this, while you run your Node server, the appliance can be available.
Sooner than deploying your code in your selected Git supplier (Bitbucket, GitHub, or GitLab), be mindful to change the HTTP request URL to your App.jsx dossier. Alternate http://localhost:8080/api
to /api
, because the URL can be prepended.
In any case, to your package deal.json dossier, upload a script command for the Node.js server that will be used for deployment:
"scripts": {
// …
"get started": "node server.js",
},
Subsequent, push your code in your most popular Git supplier and deploy your repository to Kinsta through following those steps:
- Log in in your Kinsta account at the MyKinsta dashboard.
- Choose Software at the left sidebar and click on the Upload Software button.
- Within the modal that looks, make a choice the repository you need to deploy. When you have a couple of branches, you’ll be able to make a choice the required department and provides a reputation in your software.
- Choose one of the crucial to be had knowledge heart places.
- Upload the
OPENAI_API_KEY
as an atmosphere variable. Kinsta will arrange a Dockerfile mechanically for you. - In any case, within the get started command box, upload
npm run construct && npm run get started
. Kinsta would set up your app’s dependencies from package deal.json, then construct and deploy your software.
Abstract
On this information, you’ve realized how you can harness the facility of OpenAI’s DALL-E API for symbol era. You may have additionally realized how you can paintings with React and Node.js to construct a fundamental full-stack software.
The probabilities are unending with AI, as new fashions are presented day by day, and you’ll be able to create superb tasks that may be deployed to Kinsta’s Software Web hosting.
What type would you like to discover, and what undertaking do you want us to jot down about subsequent? Proportion within the feedback beneath.
The publish Construct a React Software for AI-Powered Symbol Technology The use of OpenAI DALL-E API seemed first on Kinsta®.
WP Hosting