A uniform useful resource locator — or, extra often, URL — is the cope with of content material on the web. URLs incessantly function the cope with of a internet web page adopted through an extended string of reputedly random characters. Those will also be ugly and unmemorable. Thankfully, there are gear known as URL shorteners that may decrease them.
Shortening a URL has a number of advantages, together with making the cope with more uncomplicated to percentage and not more more likely to be typed inaccurately through customers. Even one lacking persona in a URL could make it totally pointless, directing customers to the unsuitable web page or to a useful resource that doesn’t even exist.
Take the instance of https://instance.com/blog-url-shorteners/48bfefiahl9adik
shortened to https://instance.com/url-shorteners
. It’s no longer laborious to peer which a consumer can be extra at risk of percentage, or which could be much more likely to result in errors whilst typing.
A URL shortener’s advantages transcend tidying up lengthy URLs. They are able to additionally lend a hand with the next:
- Making improvements to score in serps: Content material creators, companies, and start-ups all have content material on their internet sites, blogs, or social media. Search engines like google and yahoo choose hyperlinks with particular key phrases so they are able to be ranked accordingly and generate excellent effects. A brief URL generated from a identified platform can lend a hand rank your URL upper.
- Monitoring visitors to your hyperlinks: Paid URL shortener services and products like Bitly will let you observe the customers clicking your hyperlinks so you’ll be able to analyze your incoming visitors and customise your content material accordingly.
Two Approaches to a URL Shortener: A Python Library and an API
Following the directions on this instructional, you’ll construct a URL shortener internet app with Python the use of two other strategies:
- The pyshorteners library
- The Bitly API
The pyshorteners module is utilized by builders to generate brief URLs, whilst the Bitly API module generates brief URLs and offers extra tough purposes like clicks in line with URL, places of clicked URLs, customization of URLs, and extra.
To finish the educational, you’ll desire a elementary wisdom of Python, and Python should be put in to your machine.
Atmosphere Up the Undertaking Surroundings
Ahead of growing your URL shortener internet app, you want to arrange the surroundings for the challenge, together with the set up of Flask, a light-weight framework that makes growing Python internet apps more uncomplicated.
Start with those steps:
- Create a challenge folder, most likely with a reputation like url-shortener.
- Create an empty document named major.py inside of that folder.
- Create a digital setting for this challenge in order that any set up of Python libraries stays impartial of your machine. Use the command
python -m venv myenv
to your terminal to create that setting. (On this case, the surroundings recordsdata might be positioned within the listing myenv.) - Turn on the digital setting the use of the corresponding command to your working machine (and the place
is the title of the listing you created within the earlier step). - Home windows:
Scriptsactivate.bat - Linux/macOS:
supply
/bin/turn on
- Home windows:
- Set up Flask the use of the command
pip set up flask
. - Create a folder named templates within the challenge folder. (Flask will retrieve the HTML templates from this listing.)
Your paintings within the terminal to this point will glance one thing like this:
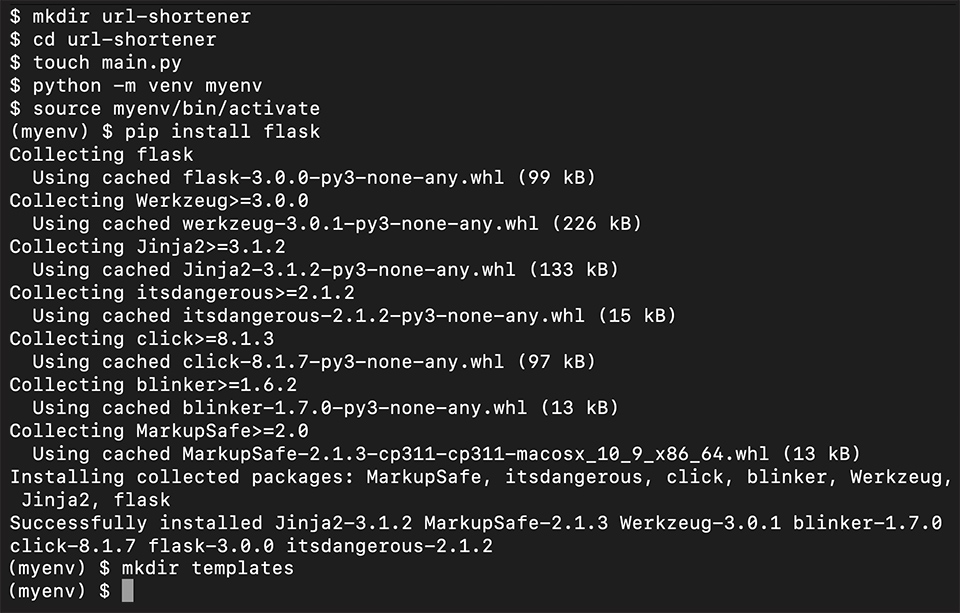
The usage of the pyshorteners Library to Construct a URL Shortener Internet App
Together with your challenge setting arrange, you’ll now create your first URL shortener the use of the pyshorteners library.
Set up the pyshorteners library with the next command:
pip set up pyshorteners
Making a Fundamental Consumer Interface for the Internet Utility
Subsequent, you’ll create a elementary shape in HTML with labels and enter fields, the place you input an extended URL and generate a shorter one.
Create a shape.html document within the templates folder, then input the next code in that document and reserve it:
URL Shortener
URL Shortener
The above code creates a kind with two labels, two enter fields, and one button.
The primary enter box known as url
, is for writing the lengthy URL, and the opposite box is for producing the quick URL.
The url
enter box has the next attributes:
title
: To spot the part (e.g., URL)placeholder
: To turn a URL instancetrend
: To specify the trend of a URL which ishttps://.*
required
: To offer a URL enter earlier than filingworth
: To view the outdated URL
The second one enter box has a worth
characteristic set to new_url
. The new_url
is a brief URL generated through the pyshorteners library from the major.py document (proven within the subsequent phase).
The access shape is depicted within the following screenshot:
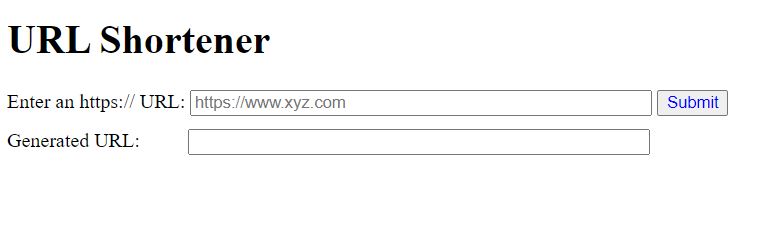
URL Shortening Code The usage of pyshorteners
Now that you just’ve created the shape, you’ll be able to upload some capability to it the use of Python and pyshorteners.
You’ll upload code to procedure the lengthy URL into a brief one and run the internet software. Navigate to the major.py document you created previous, input the next code, and reserve it:
from flask import Flask, render_template, request
import pyshorteners
app = Flask(__name__)
@app.direction("/", strategies=['POST', 'GET'])
def house():
if request.means=="POST":
url_received = request.shape["url"]
short_url = pyshorteners.Shortener().tinyurl.brief(url_received)
go back render_template("shape.html", new_url=short_url, old_url=url_received)
else:
go back render_template('shape.html')
if __name__ == "__main__":
app.run()
The code above imports the pyshorteners library and the next modules from the Flask framework, all of which you’ll want for shortening URLs:
Flask
: The Flask framework itself, which used to be up to now offered.render_template
: A template rendering package deal used to generate the output of HTML recordsdata from the foldertemplates
.request
: An object from the Flask framework that comprises all of the knowledge {that a} consumer sends from the frontend to the backend as part of an HTTP request.
Subsequent, it creates a serve as known as house()
that takes a URL submitted within the shape and outputs a brief URL. The app.direction()
decorator is used to bind the serve as to the particular URL direction for working the app, and the POST/GET strategies take care of the requests.
Within the house()
serve as, there’s an if-else
conditional observation.
For the if
observation, if request.means=="POST"
, a variable known as url_received
is about to request.shape["url"]
, which is the URL submitted within the shape. Right here, url
is the title of the enter box outlined within the HTML shape created previous.
Then, a variable known as short_url
is about to pyshorteners.Shortener().tinyurl.brief(url_received)
.
Right here, two strategies are used from the pyshorteners library: .Shortener()
and .brief()
. The .Shortener()
serve as creates a pyshorteners magnificence example and the .brief()
serve as takes within the URL as an issue and shortens it.
The brief()
serve as, tinyurl.brief()
, is without doubt one of the pyshorteners library’s many APIs. osdb.brief()
is some other API that can be used for a similar goal.
The render_template()
serve as is used to render the HTML document template shape.html and ship URLs again to the shape via arguments. The new_url
argument is about to and old_url
is about to url_received
. The if
observation’s scope ends right here.
For the else
observation, if the request means is rather than POST, simplest the shape.html HTML template might be rendered.
Demonstration of the URL Shortener Internet App Constructed with the pyshorteners Library
To display the pyshorteners URL shortener software, navigate to the default direction for the applying, http://127.0.0.1:5000/, after working the applying.
Paste a hyperlink of your selection into the primary box of the internet shape:
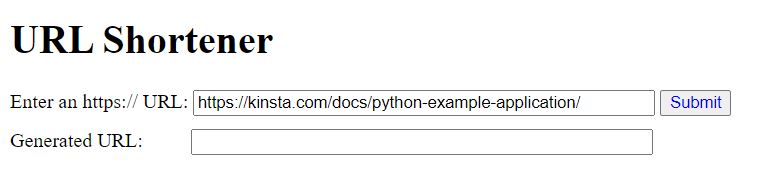
Click on the Publish button to output a brief URL with tinyurl
because the area within the Generated URL box:
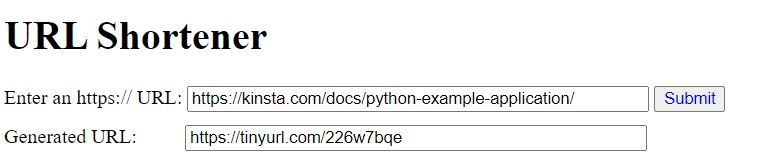
The usage of the Bitly API Module to Construct a URL Shortener Internet App
On this phase, you’ll increase a URL-shortening software the use of the Bitly API. As discussed, the Bitly API module is some other means for shortening URLs and likewise supplies detailed analytics on clicks, location, and tool kind used (reminiscent of desktop or cellular).
Set up the Bitly API the use of the next command:
pip set up bitly-api-py3
You want an get entry to token to make use of the Bitly API, which you’ll be able to get through signing up with Bitly.
After finishing the signup procedure, log in to Bitly to view your dashboard:
Click on Settings at the left sidebar, then click on the API phase discovered beneath Developer settings.
Generate an get entry to token through coming into your password within the box above the Generate token button, as proven within the symbol underneath, and stay the token to make use of within the code to your app:
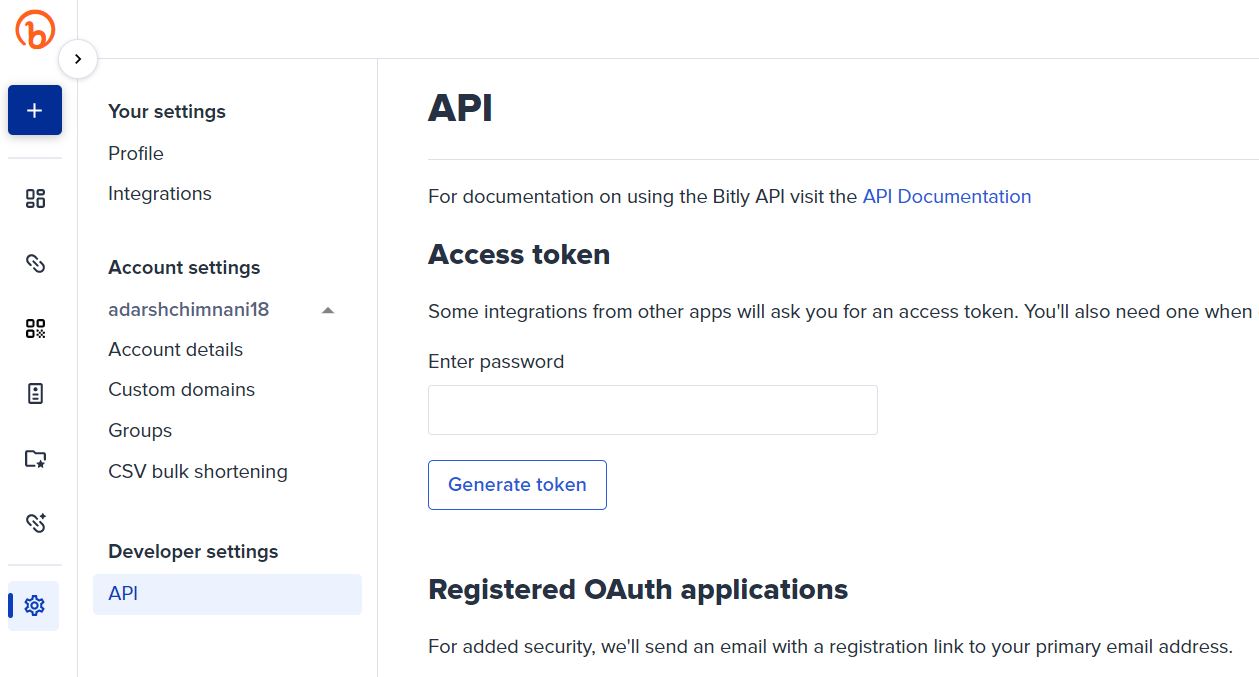
URL Shortening Code The usage of the Bitly API
Now that you’ve got the token from Bitly, you’ll be able to code the internet app to shorten the URL the use of the Bitly API.
You’ll use the similar shape you created for the pyshorteners phase however with some adjustments to the major.py document:
from flask import Flask, render_template, request
import bitly_api
app = Flask(__name__)
bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxxxx"
@app.direction("/", strategies=['POST', 'GET'])
def house():
if request.means=="POST":
url_received = request.shape["url"]
bitly = bitly_api.Connection(access_token=bitly_access_token)
short_url = bitly.shorten(url_received)
go back render_template("shape.html", new_url=short_url.get('url'), old_url=url_received)
else:
go back render_template('shape.html')
if __name__ == "__main__":
app.run()
As you’ll be able to see from the code above, bitly_api
is imported the use of import bitly_api
. The get entry to token is then stored in a variable known as bity_access_token
, as in bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxx"
.
The house()
serve as shortens the URL and comprises an if-else
conditional observation.
For the if
observation, if the process or request is POST
, then the URL submitted within the shape is about to the url_received
variable.
The bitly_api.Connection(access_token=bitly_access_token)
serve as connects to the Bitly API and passes it the get entry to token you stored previous as an issue.
To shorten the URL, the bitly.shorten()
serve as is utilized by passing the url_received
variable as an issue and saving it in a variable known as short_url
.
After all, the created shape is rendered, and URLs are despatched again to be proven within the shape the use of the render_template()
serve as. The if
observation completes right here.
For the else
observation, the shape is rendered the use of the render_template()
serve as.
Demonstration of the URL Shortener Internet App Constructed with the Bitly API
To display the Bitly API URL shortener software, navigate to the default direction for the applying, http://127.0.0.1:5000/, after working the applying.
Paste a hyperlink of your selection into the primary box of the internet shape:
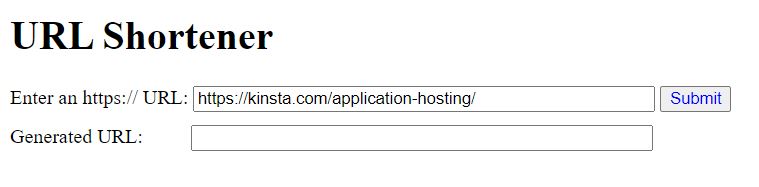
Click on Publish to generate a brief URL with bit.ly
because the area in the second one box of the internet software:
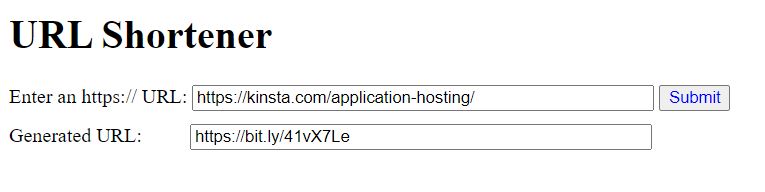
The usage of the Bitly API to shorten URLs to your Python software is a straightforward as that.
Abstract
URL shorteners provide you with brief URLs which are simple to percentage, glance cleaner, and soak up much less house. On this article, you realized about URL shorteners and their advantages, in addition to learn how to create a URL shortener internet software with Python the use of pyshorteners and the Bitly API. The pyshorteners library supplies brief URLs, whilst the Bitly API supplies detailed analytics in addition to brief URLs.
Because you’ve already made an ideal app, why no longer take it to the following degree and host it on Kinsta’s Wed Utility Web hosting provider? That can assist you get a Python app like this up and working on our platform, discover our Flask fast birth instructional.
The put up Construct a Easy URL Shortener With Python gave the impression first on Kinsta®.
WP Hosting