Managing the state of any WordPress app — the way it handles and organizes knowledge — may also be difficult. As your venture grows, maintaining a tally of the waft of knowledge and making sure constant updates throughout parts turns into more and more tricky. The WordPress knowledge package deal can assist right here, because it supplies a strong resolution for state control.
This text appears into the WordPress knowledge package deal, exploring its key ideas, implementation methods, and very best practices.
Introducing the WordPress knowledge package deal
The WordPress knowledge package deal — formally @wordpress/knowledge
— is a JavaScript (ES2015 and better) state control library that gives a predictable and centralized technique to arrange utility state. The best implementation can assist in making it more uncomplicated to construct advanced person interfaces and take care of knowledge waft throughout your utility.
The WordPress knowledge package deal takes its inspiration from Redux, a well-liked state control library within the React ecosystem.
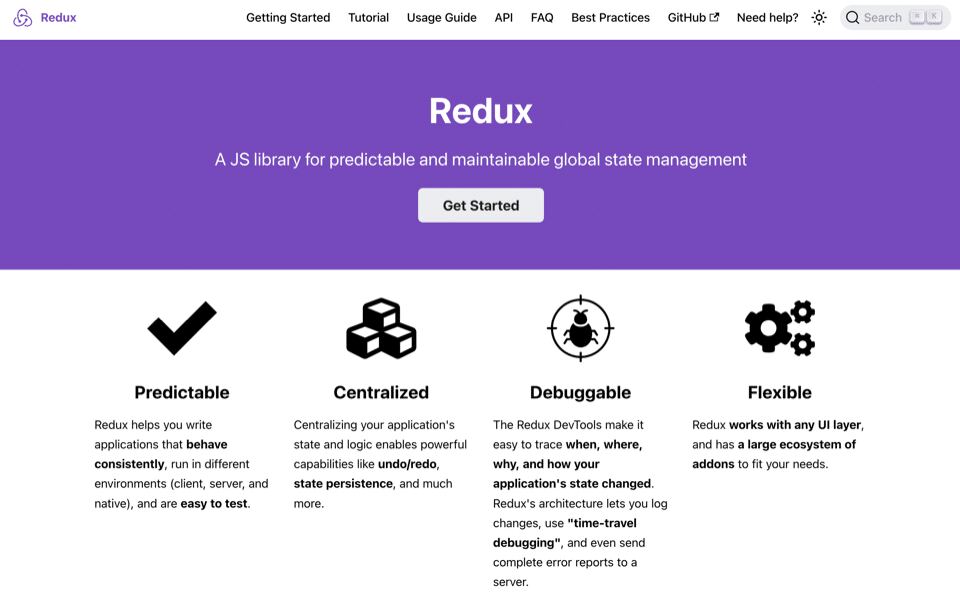
Right here, the knowledge module works throughout the WordPress atmosphere and offers integrations with WordPress-specific capability and APIs. In case you construct for the WordPress Block Editor — or it’s one thing it’s important to make stronger — the package deal shall be the most important in managing its state. By means of the use of the similar equipment and patterns to your personal plugins and subject matters, you’ll be able to create a extra constant and acquainted construction revel in.
The package deal’s courting to Redux
Whilst the WordPress knowledge package deal attracts inspiration from Redux, it’s no longer an immediate port. There are lots of diversifications to suit the WordPress ecosystem, with some key variations between the 2 answers:
- The knowledge package deal is designed to paintings seamlessly with WordPress APIs and capability, which vanilla Redux can’t do with out that adaptation.
- In comparison to Redux, the knowledge package deal supplies a extra streamlined API. This may enable you to get began.
- Not like Redux, the knowledge package deal comprises integrated make stronger for asynchronous movements. In case you paintings with the WordPress REST API, this shall be helpful.
The WordPress knowledge package deal additionally has some comparisons with the REST API. Whilst they each care for knowledge control, they serve other functions:
- The WordPress REST API supplies a technique to have interaction with WordPress knowledge over HTTP. You’ll use it for exterior apps, headless WordPress setups, and any place you wish to have to retrieve and manipulate knowledge.
- The WordPress knowledge package deal supplies a centralized retailer for knowledge and UI state. It’s a technique to take care of knowledge waft and updates inside of your app.
In lots of circumstances, you’ll use each in combination: the REST API to fetch and replace knowledge at the server and the WordPress knowledge package deal to control that knowledge inside of your utility.
Key ideas and terminology for the WordPress knowledge package deal
The WordPress knowledge package deal gives an intuitive approach of managing state. This refers back to the knowledge inside of a shop. It represents the present situation of your utility and will come with each UI state (corresponding to whether or not there’s an open modal) and information state (corresponding to an inventory of posts).
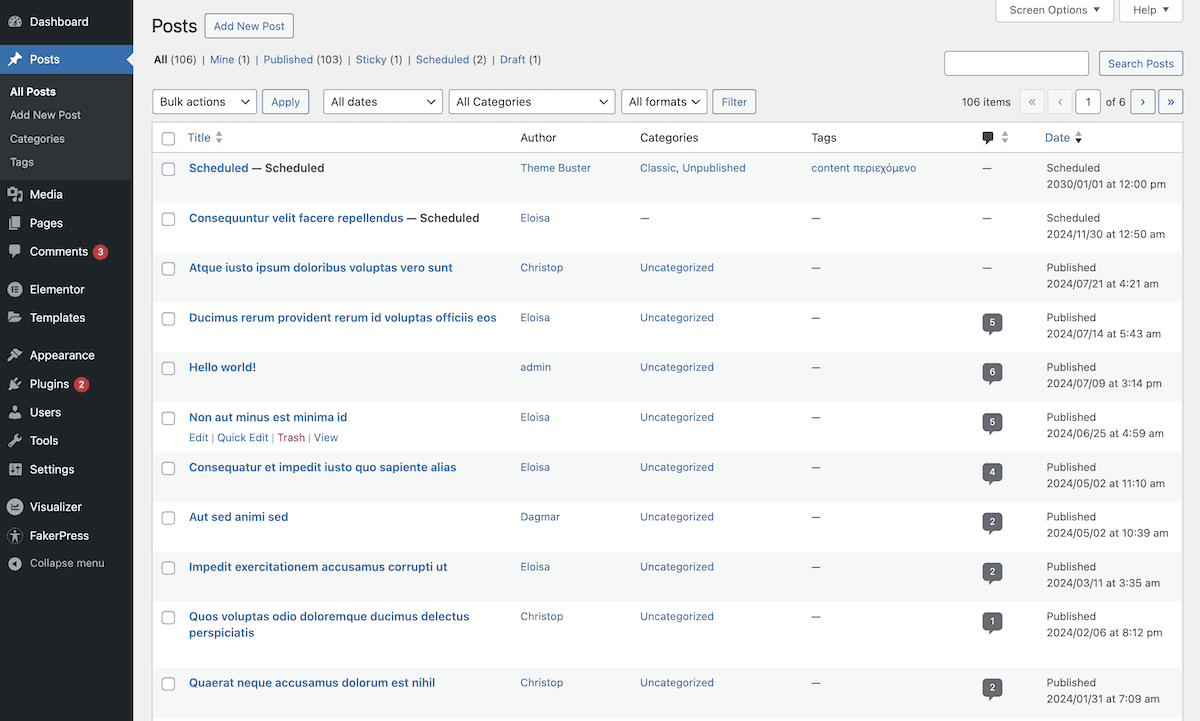
On this context, a shop is the central hub of the WordPress knowledge package deal. It holds the web page’s whole state and offers the find out how to get entry to and replace that state. In WordPress, you’ll be able to have a couple of shops. Every one shall be accountable for a selected house of your web page.
To regulate the ones shops, you wish to have a registry. This central object supplies find out how to sign up new shops and get entry to your current ones. A registry will grasp shops, and the ones shops will grasp your utility state.
There are a couple of tactics to paintings with state:
- Movements describe the adjustments to a state. Those are simple JavaScript gadgets and are the one technique to cause state updates. Movements will normally have a
kind
belongings, which describes the motion. It may additionally come with further knowledge. - Selectors extract particular items of state from the shop. Those purposes mean you can get entry to state knowledge with out the desire for direct interplay with the shop’s construction. Resolvers are comparable and take care of asynchronous knowledge fetching. You utilize those to make certain that you’ll be able to get entry to the specified knowledge in a shop prior to you run a selector.
- Reducers specify how the state must exchange in keeping with movements. They take the present state and an motion as arguments and go back a brand new state object. Regulate purposes let the reducers take care of advanced async operations with out unintended effects.
You wish to have to grasp those elementary ideas, as all of them paintings in combination to create a strong state control machine with shops at its center.
Retail outlets: the central hub of the WordPress knowledge package deal
Retail outlets are the boxes on your utility’s state and give you the find out how to have interaction with it. The WordPress knowledge package deal bundles in combination a couple of different applications, and each and every of those registers shops for the Block listing, Block Editor, core, put up enhancing, and extra.
Every retailer may have a singular namespace, corresponding to core
, core/editor
, and core/notices
. 3rd-party plugins will sign up shops, too, so you wish to have to make a choice distinctive namespaces to steer clear of conflicts. Regardless, shops you sign up will are living within the default registry typically.
This central object has a couple of duties:
- Registering new shops.
- Offering get entry to to current shops.
- Managing subscriptions to state adjustments.
When you don’t continuously have direct interplay with the registry, you do want to perceive its position in how the knowledge package deal orchestrates state control throughout WordPress.
Fundamental interplay with WordPress knowledge shops
In case you use ES2015+ JavaScript and you might be running with a WordPress plugin or theme, you’ll be able to come with it as a dependency:
npm set up @wordpress/knowledge --save
Inside of your code, you’ll import the vital purposes from the package deal on the most sensible of the report:
import { choose, dispatch, subscribe } from '@wordpress/knowledge';
Interacting with current WordPress shops calls for that you just use one of the most purposes you import. Gaining access to state knowledge with choose
, for example:
const posts = choose('core').getPosts();
It’s the similar for dispatching movements:
dispatch('core').savePost(postData);
Subscribing to state adjustments makes use of a reasonably other structure, however the concept that is similar:
subscribe(() => {
const newPosts = choose('core').getPosts();
// Replace your UI according to the brand new posts
});
On the other hand, you received’t at all times paintings with the default shops. Steadily, you’ll paintings with current further shops or sign up your individual.
Learn how to sign up a WordPress knowledge retailer
Defining your retailer’s configuration and registering it with the WordPress knowledge package deal begins with uploading the sign up
serve as:
…
import { createReduxStore, sign up } from '@wordpress/knowledge';
…
This takes a unmarried argument — your retailer descriptor. Subsequent, you must outline a default state for the shop to set its default values:
…
const DEFAULT_STATE = {
todos: [],
};
…
Subsequent, create an movements
object, outline a reducer
serve as to take care of state updates, and create a selectors
object with purposes to get entry to state knowledge:
const movements = {
addTodo: (textual content) => ({
kind: 'ADD_TODO',
textual content,
}),
};
const reducer = (state = DEFAULT_STATE, motion) => {
transfer (motion.kind) {
case 'ADD_TODO':
go back {
...state,
todos: [...state.todos, { text: action.text, completed: false }],
};
default:
go back state;
}
};
const selectors = {
getTodos: (state) => state.todos,
};
To create the shop configuration, outline it the use of the createReduxStore
object. This may initialize the movements, selectors, regulate, and different houses on your retailer:
const retailer = createReduxStore('my-plugin/todos', {
reducer,
movements,
selectors,
});
The minimal this object wishes is a reducer to outline the form of the state and the way it adjustments in keeping with different movements. In any case, sign up the shop, calling the shop descriptor you outline with createReduxStore
:
sign up(retailer);
You’ll be able to now have interaction together with your customized retailer as you could others:
import { choose, dispatch } from '@wordpress/knowledge';
// Upload a brand new todo
dispatch('my-plugin/todos').addTodo('Be told WordPress knowledge package deal');
// Get all todos
const todos = choose('my-plugin/todos').getTodos();
The important thing in the use of the WordPress knowledge package deal is how you employ the other houses and gadgets at your disposal.
Breaking down the 5 WordPress knowledge retailer houses
A lot of the use of the WordPress knowledge package deal occurs “backwards” — defining low-level knowledge retailer houses prior to the shop itself. The createReduxStore
object is an ideal instance, because it pulls in combination all the definitions you are making to create the descriptor you employ to sign up a shop:
import { createReduxStore } from '@wordpress/knowledge';
const retailer = createReduxStore( 'demo', {
reducer: ( state = 'OK' ) => state,
selectors: {
getValue: ( state ) => state,
},
} );
Those different houses additionally want setup and configuration.
1. Movements
Movements are the main technique to cause state adjustments to your retailer. They’re simple JavaScript gadgets that describe what must occur. As such, it may be a good suggestion to create those first, as you’ll be able to make a decision what states you need to retrieve.
const movements = {
addTodo: (textual content) => ({
kind: 'ADD_TODO',
textual content,
}),
toggleTodo: (index) => ({
kind: 'TOGGLE_TODO',
index,
}),
};
Motion creators take non-compulsory arguments and can go back an object to go alongside to the reducer you outline:
const movements = {
updateStockPrice: (image, newPrice) => {
go back {
kind: 'UPDATE_STOCK_PRICE',
image,
newPrice
};
},
In case you go in a shop descriptor, you’ll be able to dispatch motion creators and replace the state price:
dispatch('my-plugin/todos').updateStockPrice('¥', '150.37');
Imagine motion gadgets as directions for the reducer on the way to make state adjustments. At a minimal, you’ll most likely wish to outline create, replace, learn, and delete (CRUD) movements. It is also that you’ve a separate JavaScript report for motion sorts and create an object for all of the ones sorts, particularly in case you outline them as constants.
2. Reducer
It’s price speaking concerning the reducer right here, as a result of its central position along movements. Its activity is to specify how the state must exchange in keeping with the directions it will get from an motion. In case you go it the directions from the motion and the present state, it will possibly go back a brand new state object and go it alongside the chain:
const reducer = (state = DEFAULT_STATE, motion) => {
transfer (motion.kind) {
case 'ADD_TODO':
go back {
...state,
todos: [...state.todos, { text: action.text, completed: false }],
};
case 'TOGGLE_TODO':
go back {
...state,
todos: state.todos.map((todo, index) =>
index === motion.index ? { ...todo, finished: !todo.finished } : todo
),
};
default:
go back state;
}
};
Notice {that a} reducer will have to be a natural serve as, and it shouldn’t mutate the state it accepts (quite, it must go back it with updates). Reducers and movements have a symbiotic courting in lots of respects, so greedy how they paintings in combination is essential.
3. Selectors
To be able to get entry to the present state from a registered retailer, you wish to have selectors. It’s the main approach you “disclose” your retailer state, and so they assist stay your parts decoupled from the shop’s inside construction:
const selectors = {
getTodos: (state) => state.todos,
getTodoCount: (state) => state.todos.period,
};
You’ll be able to name the ones selectors with the choose
serve as:
const todoCount = choose('my-plugin/todos').getTodoCount();
On the other hand, a selector doesn’t ship that knowledge any place: it merely unearths it and offers get entry to.
Selectors can obtain as many arguments as you wish to have to get entry to the state with accuracy. The price it returns is the results of no matter the ones arguments succeed in throughout the selector you outline. As with movements, you may make a choice to have a separate report to carry your whole selectors, as there may well be a large number of them.
4. Controls
Guiding the execution waft of your web page’s capability — or executing common sense inside of it — is the place you use controls. Those outline the habits of execution flows on your movements. Imagine those the assistants within the WordPress knowledge package deal as they paintings as go-betweens to assemble the state to go to resolvers.
Controls additionally take care of unintended effects to your retailer, corresponding to API calls or interactions with browser APIs. They mean you can stay reducers blank whilst nonetheless enabling you to take care of advanced async operations:
const controls = {
FETCH_TODOS: async () => {
const reaction = wait for fetch('/api/todos');
go back reaction.json();
},
};
const movements = {
fetchTodos: () => ({ kind: 'FETCH_TODOS' }),
};
This cycle of fetching and returning knowledge is the most important to all of the procedure. However with out a name from an motion, you received’t have the ability to use that knowledge.
5. Resolvers
Selectors disclose a shop’s state, however don’t explicitly ship that knowledge any place. Resolvers meet selectors (and controls) to retrieve the knowledge. Like controls, they may be able to additionally take care of asynchronous knowledge fetching.
const resolvers = {
getTodos: async () => {
const todos = wait for controls.FETCH_TODOS();
go back movements.receiveTodos(todos);
},
};
The resolver guarantees that the knowledge you ask for is to be had within the retailer prior to operating a selector. This tight connectivity between the resolver and the selector method they want to fit names. That is so the WordPress knowledge package deal can perceive which resolver to invoke according to the knowledge you request.
What’s extra, the resolver will at all times obtain the similar arguments that you just go right into a selector serve as, and also will both go back, yield, or dispatch motion gadgets.
Error dealing with when the use of the WordPress knowledge package deal
You will have to put into effect correct error dealing with when running with the WordPress knowledge package deal. If you select to care for asynchronous operations, paintings with complete stack deployments, or make API calls, it’s much more necessary.
For instance, in case you dispatch movements that contain asynchronous operations, a try-catch block can be a just right possibility:
const StockUpdater = () => {
// Get the dispatch serve as
const { updateStock, setError, clearError } = useDispatch('my-app/shares');
const handleUpdateStock = async (stockId, newData) => {
attempt {
// Transparent any current mistakes
clearError();
// Try to replace the inventory
wait for updateStock(stockId, newData);
} catch (error) {
// Dispatch an error motion if one thing is going incorrect
setError(error.message);
}
};
go back (
);
};
For reducers, you’ll be able to take care of error movements and replace the state:
const reducer = (state = DEFAULT_STATE, motion) => {
transfer (motion.kind) {
// ... different circumstances
case 'FETCH_TODOS_ERROR':
go back {
...state,
error: motion.error,
isLoading: false,
};
default:
go back state;
}
};
When the use of selectors, you’ll be able to come with error checking to take care of possible problems then test for mistakes to your parts prior to you employ the knowledge.:
const MyComponent = () => {
// Get a couple of items of state together with error knowledge
const { knowledge, isLoading, error } = useSelect((choose) => ({
knowledge: choose('my-app/shares').getStockData(),
isLoading: choose('my-app/shares').isLoading(),
error: choose('my-app/shares').getError()
}));
// Maintain other states
if (isLoading) {
go back Loading...;
}
if (error) {
go back (
Error loading shares: {error.message}
);
}
go back (
{/* Your standard part render */}
);
};
The useSelect
and useDispatch
purposes provide you with a large number of energy to take care of mistakes throughout the WordPress knowledge package deal. With either one of those, you’ll be able to go in customized error messages as arguments.
It’s just right apply to be sure to centralize your error state all through preliminary configuration, and to stay error barriers on the part point. Using error dealing with for loading states can even assist to stay your code transparent and constant.
Learn how to combine your WordPress knowledge retailer together with your web page
There’s so much that the WordPress knowledge package deal can do that will help you arrange state. Striking all of this in combination could also be a realistic attention. Let’s take a look at a inventory ticker that presentations and updates monetary knowledge in real-time.
The primary activity is to create a shop on your knowledge:
import { createReduxStore, sign up } from '@wordpress/knowledge';
const DEFAULT_STATE = {
shares: [],
isLoading: false,
error: null,
};
const movements = {
fetchStocks: () => async ({ dispatch }) => {
dispatch({ kind: 'FETCH_STOCKS_START' });
attempt {
const reaction = wait for fetch('/api/shares');
const shares = wait for reaction.json();
dispatch({ kind: 'RECEIVE_STOCKS', shares });
} catch (error) {
dispatch({ kind: 'FETCH_STOCKS_ERROR', error: error.message });
}
},
};
const reducer = (state = DEFAULT_STATE, motion) => {
transfer (motion.kind) {
case 'FETCH_STOCKS_START':
go back { ...state, isLoading: true, error: null };
case 'RECEIVE_STOCKS':
go back { ...state, shares: motion.shares, isLoading: false };
case 'FETCH_STOCKS_ERROR':
go back { ...state, error: motion.error, isLoading: false };
default:
go back state;
}
};
const selectors = {
getStocks: (state) => state.shares,
getStocksError: (state) => state.error,
isStocksLoading: (state) => state.isLoading,
};
const retailer = createReduxStore('my-investing-app/shares', {
reducer,
movements,
selectors,
});
sign up(retailer);
This procedure units up a default state that comes with error and loading states, together with your movements, reducers, and selectors. While you outline those, you’ll be able to sign up the shop.
Show the shop’s knowledge
With a shop in position, you’ll be able to create a React part to show the ideas inside of it:
import { useSelect, useDispatch } from '@wordpress/knowledge';
import { useEffect } from '@wordpress/component';
const StockTicker = () => {
const shares = useSelect((choose) => choose('my-investing-app/shares').getStocks());
const error = useSelect((choose) => choose('my-investing-app/shares').getStocksError());
const isLoading = useSelect((choose) => choose('my-investing-app/shares').isStocksLoading());
const { fetchStocks } = useDispatch('my-investing-app/shares');
useEffect(() => {
fetchStocks();
}, []);
if (isLoading) {
go back Loading inventory knowledge...
;
}
if (error) {
go back Error: {error}
;
}
go back (
Inventory Ticker
{shares.map((inventory) => (
-
{inventory.image}: ${inventory.value}
))}
);
};
This part brings within the useSelect
and useDispatch
hooks (together with others) to take care of knowledge get entry to, dispatching movements, and part lifecycle control. It additionally units customized error and loading state messages, together with some code to in truth show the ticker. With this in position, you presently must sign up the part with WordPress.
Registering the part with WordPress
With out registration inside of WordPress, you received’t have the ability to use the parts you create. This implies registering it as a Block, even if it can be a widget in case you design for Vintage Issues. This situation makes use of a Block.
import { registerBlockType } from '@wordpress/blocks';
import { StockTicker } from './parts/StockTicker';
registerBlockType('my-investing-app/stock-ticker', {
identify: 'Inventory Ticker',
icon: 'chart-line',
class: 'widgets',
edit: StockTicker,
save: () => null, // This may render dynamically
});
This procedure will observe the standard means you’ll take to sign up Blocks inside of WordPress, and doesn’t require any particular implementation or setup.
Managing state updates and person interactions
While you sign up the Block, it’s important to take care of person interactions and real-time updates. This may want some interactive controls, together with customized HTML and JavaScript:
const StockControls = () => {
const { addToWatchlist, removeFromWatchlist } = useDispatch('my-investing-app/shares');
go back (
);
};
For real-time updates, you’ll be able to arrange an period throughout the React part:
useEffect(() => {
const { updateStockPrice } = dispatch('my-investing-app/shares');
const period = setInterval(() => {
shares.forEach(inventory => {
fetchStockPrice(inventory.image)
.then(value => updateStockPrice(inventory.image, value));
});
}, 60000);
go back () => clearInterval(period);
}, [stocks]);
This means helps to keep your part’s knowledge in sync together with your retailer whilst keeping up a transparent separation of issues. The WordPress knowledge package deal will take care of all state updates, which supplies your app consistency.
Server-side rendering
In any case, you’ll be able to arrange server-side rendering to make certain that the inventory knowledge is present upon web page load. This calls for some PHP wisdom:
serve as my_investing_app_render_stock_ticker($attributes, $content material) {
// Fetch the newest inventory knowledge out of your API
$shares = fetch_latest_stock_data();
ob_start();
?>
Inventory Ticker
- : $
'my_investing_app_render_stock_ticker'
));
This means supplies a whole integration of your knowledge retailer with WordPress, dealing with the entirety from preliminary render to real-time updates and person interactions.
Abstract
The WordPress knowledge package deal is a fancy but tough technique to arrange utility states on your initiatives. Past the important thing ideas lies a rabbit hollow of purposes, operators, arguments, and extra. Be mindful, although, that no longer each piece of knowledge must be in an international retailer — the native part state nonetheless has a spot to your code.
Do you spot your self the use of the WordPress knowledge package deal frequently, or do you may have any other manner of managing state? Proportion your critiques with us within the feedback under.
The put up Learn how to use the WordPress knowledge package deal to control utility states gave the impression first on Kinsta®.
WP Hosting