p>Flask is a light-weight internet framework for Python that makes it simple to construct internet programs. In our earlier article, we’ve noticed easy methods to arrange a easy web page.
However, it’s only a easy textual content like “Hi Flask!”. In actual programs, you’ll wish to render extra than simply easy textual content. You’ll wish to render HTML templates.
In Flask, we will be able to accomplish that with Jinja.
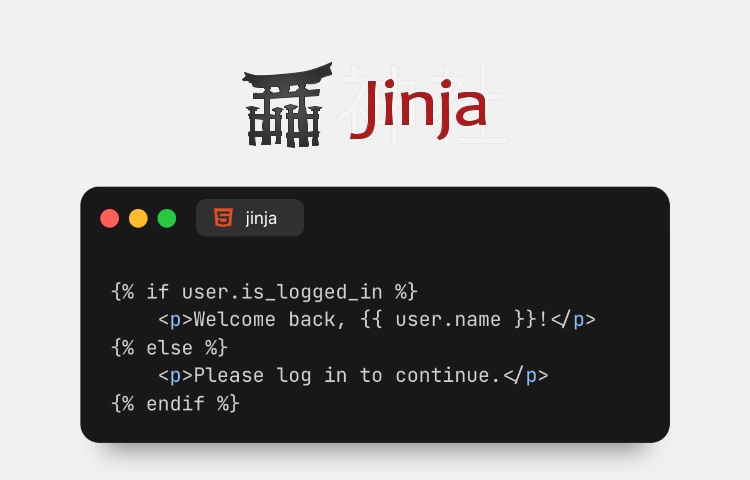
Jinja
One in all its tough options is the facility to render HTML templates the use of the Jinja templating engine.
Jinja templates are written in common HTML that you’re already acquainted with. However, on best of that, you’ll be able to use particular syntax to deal with dynamic content material, reminiscent of for passing knowledge from requests or different code assets to the template. It additionally means that you can use regulate buildings like loops and conditionals.
Let’s see the way it works in its fundamental shape:
Welcome to Flask {% if person.is_logged_in %}Welcome again, {{ person.title }}!
{% else %}Please log in to proceed.
{% endif %}
It seems like a standard HTML report, however as we will be able to see, we use some particular syntax, such because the {{ }}
to insert a variable, and we use {% %}
for including loops and conditionals.
Rendering the Templates
To render the template, we want to put it within the templates
listing.
. |-- app.py |-- templates |-- about.html |-- index.html
Then, we use the render_template
serve as in our Python app report to render HTML templates. If we lengthen the similar code from our earlier article, our code would now seem like the next:
from flask import Flask, render_template app = Flask(__name__) @app.course('/') def house(): go back render_template('index.html') @app.course('/about') def about(): go back render_template('about.html')
Passing Variables
In our template above, we’ve got {{ title }}
and {{ person.is_logged_in }}
variables. However, we haven’t handed any variables to the template but.
To go variables to the template, we will be able to accomplish that by way of passing them as arguments to the render_template
serve as.
As an example:
@app.course('/') def house(): go back render_template('index.html', person={'is_logged_in': True, 'title': 'Joann'})
Now, after we consult with the house web page, we can see the “Welcome again, Joann!” message as a substitute of “Please log in to proceed.” for the reason that is_logged_in
is ready to True
.
Template Inheritance
Jinja means that you can use template inheritance, which is beneficial when a couple of pages percentage the similar structure or parts. As an alternative of repeating not unusual components, we will be able to create a base template and reuse it in different templates.
On this instance, we’ve got a homepage and an about web page that percentage the similar structure, just like the head
, name
, and perhaps some kinds or scripts in a while. With template inheritance, we will be able to make issues more practical and extra arranged.
First, we create the bottom report. Let’s title it base.html
. Then, upload the average components:
{% block name %}Welcome to Flask{% endblock %} {% block content material %}{% endblock %}
On this template, there are two blocks: one for the name and one for the content material. The {% block %}
tag lets in kid templates to override particular sections of the bottom template.
Now, we will be able to rewrite the index.html
report to increase the base.html
report. We will take away the entire fundamental HTML construction, just like the head
and name
tags from the index.html
report, and substitute it with the extends
tag.
{% extends 'base.html' %} {% block name %}Welcome to Flask{% endblock %} {% block content material %} {% if person.is_logged_in %}Welcome again, {{ person.title }}!
{% else %}Please log in to proceed.
{% endif %} {% endblock %}
At the about web page, shall we additionally rewrite it as follows:
{% extends 'base.html' %} {% block name %}About Us{% endblock %} {% block content material %}That is the about web page.
{% endblock %}
After we consult with the homepage, we’ll see the “Welcome again, Joann!” message if the person is logged in. At the about web page, we’ll see “That is the about web page.”. The adaptation this is that each pages are cleaner and more straightforward to deal with, with out repeating code.
Wrapping Up
That’s how we will be able to render HTML templates in Flask the use of Jinja. Jinja is a formidable templating engine that includes useful options and extensions, making it more straightforward to construct dynamic internet programs in Python.
There’s nonetheless much more to discover, however I’m hoping this text provides you with a forged place to begin to rise up and operating with Flask.
The publish The way to Render Templates in Flask seemed first on Hongkiat.
WordPress Website Development Source: https://www.hongkiat.com/blog/flask-render-html-templates-jinja/