WooCommerce is likely one of the hottest WordPress plugins for development ecommerce packages. Along with the usage of WooCommerce in a standard WordPress utility, you’ll use it as a headless resolution.
This text teaches you how one can construct a headless WordPress utility with React deployed on Kinsta.
Advent to headless WooCommerce
The time period “headless ecommerce” approach the separation of the backend (head) from the frontend (frame) of an ecommerce utility. Headless structure passes requests between the presentation layers (frontend) and the appliance layers (backend) of an ecommerce resolution via APIs.
The APIs outline interactions between intermediaries, permitting companies to switch the presentation layers with out tense the capability of utility layers and replace, edit, or upload merchandise to more than one utility layers.
In a headless WooCommerce utility, the WooCommerce API is an middleman, offering communique between the frontend and backend. Along with the advantages discussed above, this helps versatile and scalable frontend building, empowering you to concentrate on crafting attractive and dynamic person interfaces with fashionable generation stacks like React or Vue.
Additionally, the usage of headless WooCommerce future-proofs ecommerce infrastructure via serving to you adapt to evolving buyer wishes and technological developments. You’ll without difficulty replace frontend elements whilst making sure the steadiness and reliability of the backend WooCommerce platform.
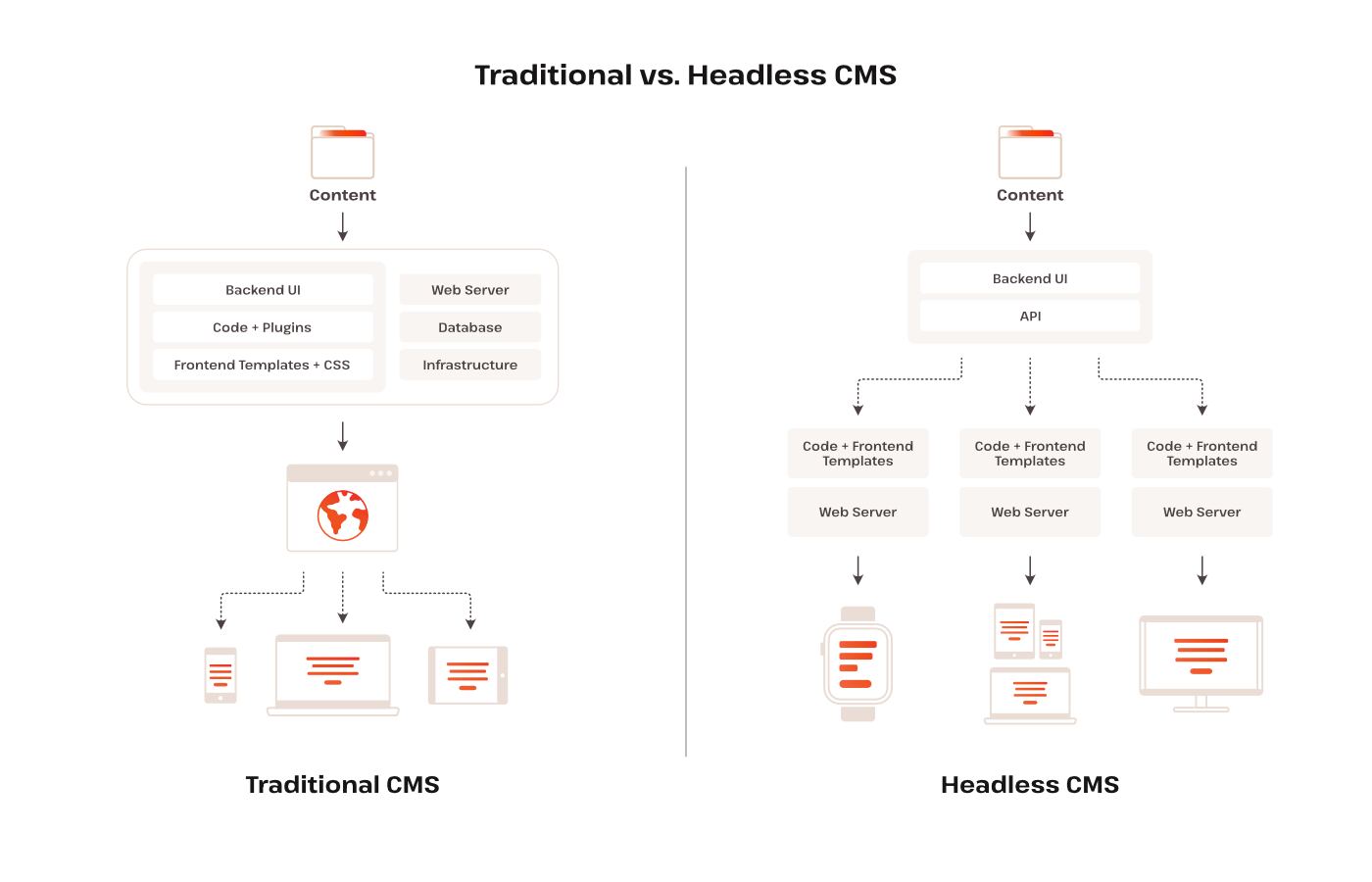
This contemporary way provides a large number of advantages in comparison to conventional WordPress ecommerce setups.
Now that you know what a headless WooCommerce utility is and its advantages, it’s time to construct one for your self. The usage of WordPress, React, and the WooCommerce plugin, you’ll create an ecommerce app and deploy it on Kinsta.
Necessities
Earlier than starting, be sure to have the next:
- An working out of React and WordPress
- A Node.js set up
- An operational WordPress web page
- An lively Kinsta account
Advantages of headless WooCommerce
When you select the headless path in your WooCommerce packages, you free up a spread of advantages—particularly the versatility of getting one backend enhance more than a few frontends in your utility.
Listed here are another advantages of headless WooCommerce:
- Enhanced customization — You’ll construct your WooCommerce utility how you wish to have the usage of any internet framework.
- Advanced web site efficiency — You’ll leverage speedy internet frameworks like React and Vue to noticeably spice up your web site’s efficiency.
- search engine optimization advantages — You might have extra regulate and versatility over search engine optimization methods to succeed in your enterprise’s objectives.
- Higher scalability — Your web site can scale extra successfully, making sure easy efficiency even all the way through classes of excessive site visitors.
- Skill to create distinctive buyer reviews — You might be loose from the restrictions of conventional templates. You’ll craft leading edge and customized reviews in your shoppers.
Putting in a headless WooCommerce web site
Right here’s a step by step information to putting in a WooCommerce web site:
- Log in in your MyKinsta dashboard
- Navigate to Upload Carrier > WordPress web site.
- Choose the Set up WordPress choice.
- At the Upload new WordPress web site web page, whole the fields required to put in WordPress.
- Take a look at the Set up WooCommerce field ahead of you click on Proceed.
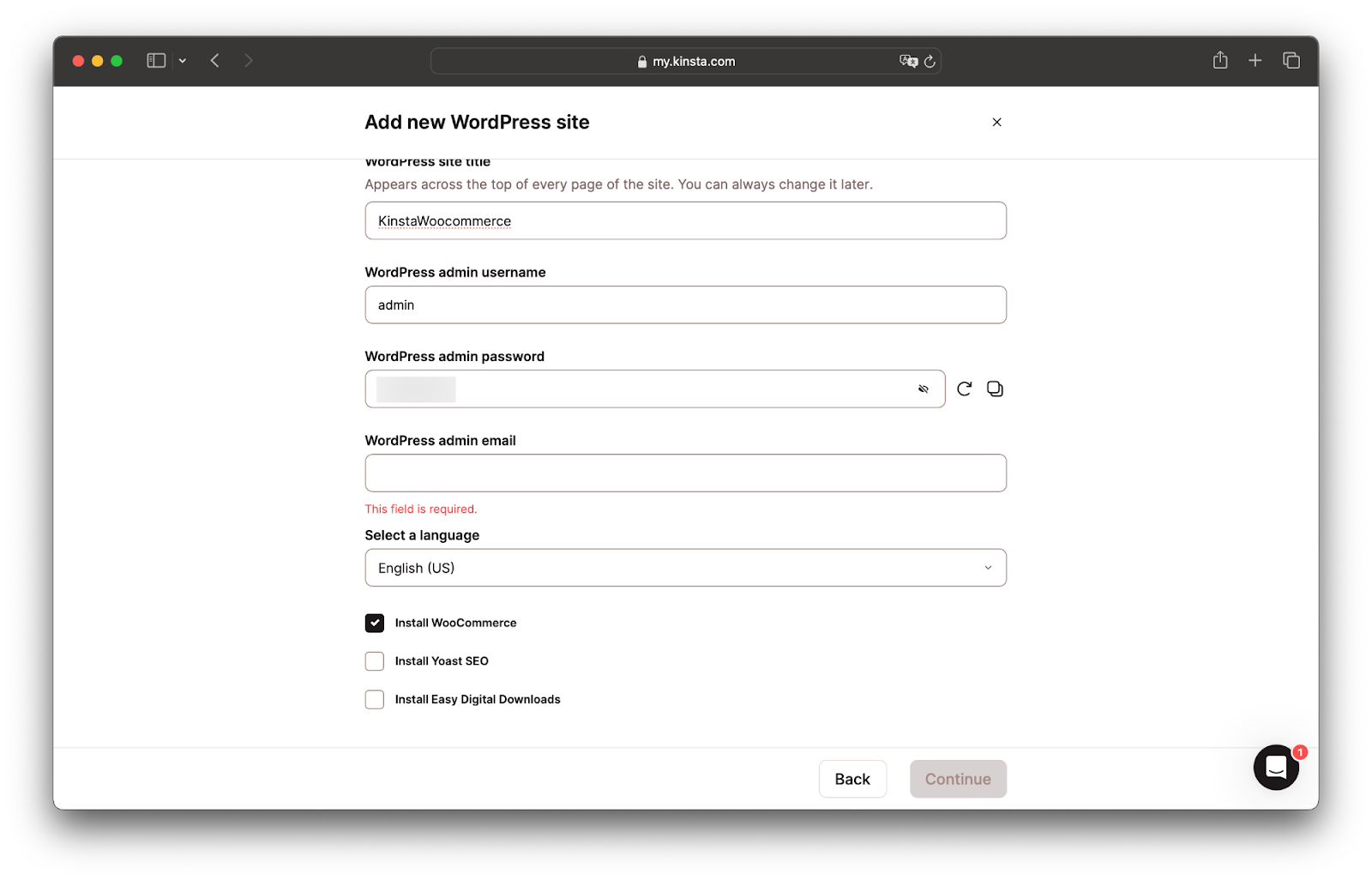
Turn on the WooCommerce plugin
- In your MyKinsta dashboard, click on Domain names at the sidebar.
- At the Domain names web page, click on Open WordPress Admin.
- Log in in your WordPress Admin dashboard, navigate to plugins, make a choice the WooCommerce plugin, and turn on it.
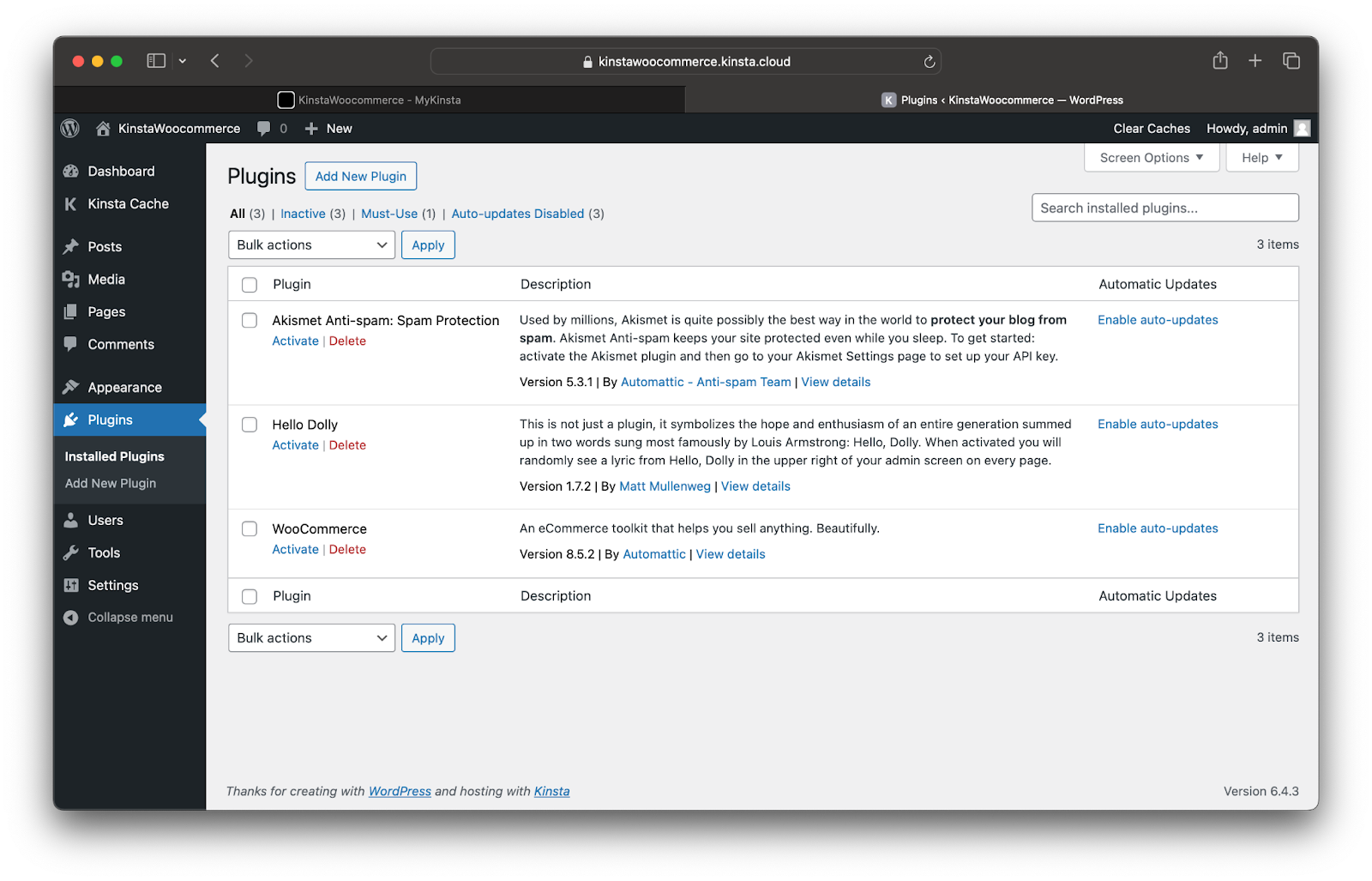
Configure WooCommerce for headless operation
To configure WooCommerce for headless operations:
- Log in in your WordPress Admin dashboard. At the sidebar, click on WooCommerce > Settings.
- At the Settings web page, click on the Complicated tab. Then, click on Leisure API.
- Now click on Upload key.
Including the important thing for the REST API. - Click on Create an API key. Give your new REST API an outline, set the Permissions box to Learn/Write, and click on Generate API key.
Producing the WooCommerce API key. - Reproduction the patron key and secret and retailer them safely to be used within the React utility.
- In spite of everything, alter the Permalink construction to verify JSON information is returned when asking for the API. At the WordPress Admin dashboard, click on Settings > Permalinks, and make a choice Publish identify.
Earlier than continuing, upload some merchandise in your WooCommerce retailer. Navigate to the WooCommerce segment for your WordPress dashboard, click on on Merchandise, and observe the activates so as to add merchandise. You’ll additionally import those pattern merchandise in your retailer.
With WordPress and WooCommerce arrange, you’re in a position to concentrate on the frontend of your headless ecommerce utility.
Arrange a React undertaking
Right here’s how one can arrange a React undertaking:
- To your most well-liked listing, use the next instructions to create a React undertaking:
# Have in mind to interchange
together with your most well-liked identify # With npx npx create-react-app && cd # With yarn yarn create react-app && cd - As soon as your undertaking is created, create a .env record within the root of your undertaking and upload the next:
REACT_APP_CONSUMER_KEY=your_Woocommerce_consumer_key REACT_APP_CONSUMER_SECRET=your_Woocommerce_consumer_secret
Substitute this together with your WooCommerce shopper key and secret generated previous.
- Subsequent, use the command beneath to put in a package deal for managing routes:
## With npm npm i react-router-dom ## With yarn yarn upload react-router-dom
- In spite of everything, release the React undertaking the usage of the next command:
## With npm npm get started ## With yarn yarn get started
Broaden the frontend in your headless WooCommerce web site
A a success ecommerce retailer showcases merchandise and facilitates purchases. Start via exhibiting the goods to be had within the WooCommerce retailer at the frontend. This comes to making requests to the WooCommerce API.
The endpoint to checklist all merchandise is wp-json/wc/v3/merchandise
. This endpoint should be appended to the host URL. Upload this variable in your .env record in your base URL, which is the host URL appended with the merchandise
endpoint. Replace http://instance.com
together with your WooCommerce web site area.
REACT_APP_BASE_URL=http://instance.com/wp-json/wc/v3/merchandise
When making API requests, you should come with your shopper key and secret within the URL. When mixed, the URL seems like this:
https://kinstawoocommerce.kinsta.cloud/wp-json/wc/v3/merchandise?consumer_key=your_consumer_key&consumer_secret=your_consumer_secret
Let’s make API requests within the React utility the usage of the Fetch API to fetch the WooCommerce merchandise.
- To your React utility, open the App.js record within the src listing and exchange its contents with the code beneath:
import {Hyperlink} from 'react-router-dom'; import {useEffect, useState} from 'react'; serve as App() { const BASE_URL = procedure.env.REACT_APP_BASE_URL; const CONSUMER_KEY = procedure.env.REACT_APP_CONSUMER_KEY; const CONSUMER_SECRET = procedure.env.REACT_APP_CONSUMER_SECRET; const [products, setProducts] = useState([]); const [loading, setLoading] = useState(true); useEffect(() => { const fetchProducts = async () => { const reaction = anticipate fetch(`${BASE_URL}?consumer_key=${CONSUMER_KEY}&consumer_secret=${CONSUMER_SECRET}`); const information = anticipate reaction.json(); setProducts(information); setLoading(false); }; fetchProducts(); }, [BASE_URL, CONSUMER_KEY, CONSUMER_SECRET]); go back loading ? (
Only a second. Fetching merchandise...
{' '}-
{merchandise ? (
merchandise.map((product) => (
-
{product.identify}
Sale worth: {product.sale_price}
{product.stock_status === 'instock' ? 'In inventory' : 'Out of inventory'}
))
) : (
- No merchandise discovered )}
This code fetches an inventory of goods from the WooCommerce API endpoint the usage of the Fetch API when the part mounts (
useEffect
hook). The endpoint URL is built the usage of atmosphere variables set previousAs soon as the information is fetched, it updates the part state (
merchandise
) the usage ofsetProducts(reaction)
and unitsloading
tofalse
the usage ofsetLoading(false)
.Whilst the information is being fetched, it presentations a loading message. As soon as the information is fetched and
loading
is about tofalse
, it maps during themerchandise
array and renders an inventory of product pieces the usage of JavaScript XML (JSX). Every product merchandise is wrapped in aHyperlink
part fromreact-router-dom
, which generates a hyperlink to the person product’s main points web page in response to its ID.The product’s identify, worth, description (rendered the usage of
dangerouslySetInnerHTML
to inject HTML content material), inventory standing, and a button are displayed for each and every product. -
- Within the src listing, open the index.js record and exchange the code with the snippet beneath:
import React from "react"; import ReactDOM from "react-dom"; import { BrowserRouter, Course, Routes } from "react-router-dom"; import "./index.css"; import App from "./App"; ReactDOM.render(
Kinsta Retailer
} /> It renders the
App
part when the/
path is named within the browser. - Run your app to view the adjustments.
## With npm npm get started ## With yarn yarn get started
The React utility now presentations an inventory of goods from the WooCommerce retailer.
WooCommerce retailer product checklist.
Create dynamic product pages
Now, to strengthen the person revel in, create dynamic product pages the usage of the WooCommerce API. This API supplies an endpoint to fetch information a few unmarried product: wp-json/wc/v3/merchandise/
. To make use of this endpoint to fetch and show information a few unmarried product within the retailer, observe those steps:
- Create an element ProductDetail.js within the src listing that fetches and presentations information a few unmarried product. This part fetches and presentations detailed details about a unmarried product.It operates in a identical way to the code within the App.js record, with the exception of it retrieves main points for a unmarried product.
import {useState, useEffect} from 'react'; import {useParams} from 'react-router-dom'; serve as ProductDetail() { const BASE_URL = procedure.env.REACT_APP_BASE_URL; const CONSUMER_KEY = procedure.env.REACT_APP_CONSUMER_KEY; const CONSUMER_SECRET = procedure.env.REACT_APP_CONSUMER_SECRET; const {identification} = useParams(); const [product, setProduct] = useState(null); useEffect(() => { const fetchSingleProductDetails = async () => { const reaction = anticipate fetch( `${BASE_URL}/${identification}?consumer_key=${CONSUMER_KEY}&consumer_secret=${CONSUMER_SECRET}` ); const information = anticipate reaction.json(); setProduct(information); }; fetchSingleProductDetails(); }, [BASE_URL, CONSUMER_KEY, CONSUMER_SECRET, id]); if (!product) { go back (
Fetching product main points...
{product.identify}
Unique Value: {product.regular_price}
Sale worth: {product.sale_price}
{product.stock_status === 'instock' ? 'In inventory' : 'Out of inventory'}
- Create a brand new path in index.js and assign the
ProductDetail
part to it. This code snippet creates a brand new path in index.js and assigns theProductDetail
part. This guarantees that after customers click on a product hyperlink, they’re directed to the respective product web page.// index.js … import ProductDetail from "./ProductDetail"; ReactDOM.render(
… } /> {/* the brand new path */} } />