Object-oriented programming (OOP), crucial paradigm in device, is focused on “gadgets” — circumstances of categories that come with knowledge and behaviors as an alternative of “movements.”
PHP, recognized for server-side scripting, advantages very much from OOP. It’s because OPP helps modular and reusable code, making it more uncomplicated to take care of. In flip, this facilitates higher group and scalability of enormous initiatives.
Mastering OOP is vital for builders operating with WordPress topics, plugins, and customized answers. On this article, we’ll define what OOP is in PHP and the affect it has on WordPress building. We’ll additionally stroll you thru tips on how to enforce OOP ideas in PHP.
Must haves
To observe the hands-on portions of this newsletter, be sure you have:
- XAMPP (or an identical) for Apache and MySQL
- A PHP setting in model 7.4 or upper
- A code editor, like Visible Studio Code
- A native or web-hosted WordPress set up.
- Wisdom of PHP and WordPress
Benefits of OOP in PHP building
OOP considerably boosts PHP building through bettering modularity, reusability, scalability, maintainability, and teamwork. It organizes PHP code through dividing it into gadgets, each and every representing a selected a part of the applying. The usage of gadgets, you’ll be able to simply reuse code, saving time and lowering mistakes.
With this in thoughts, let’s dive into two particular benefits of OOP in PHP, highlighting the way it transforms the improvement procedure.
1. Code reusability and upkeep
OOP in PHP makes it easy to reuse code as a result of inheritance and polymorphism. Categories can use houses and techniques from different categories. This allows you to use previous code in new techniques with little trade.
OOP additionally makes it more uncomplicated to maintain your code. Encapsulation way gadgets stay their main points non-public and most effective percentage what’s wanted, the use of particular strategies known as getters and setters. This manner is helping save you adjustments in a single a part of your software from inflicting issues in others, making the code more practical to replace and take care of.
Additionally, as a result of gadgets are entire on their very own, discovering and solving insects in positive portions of the gadget is more uncomplicated. This improves total code high quality and reliability.
2. Enhanced readability and construction
OOP makes PHP code cleaner and extra arranged the use of categories and gadgets. Categories act like templates for gadgets, protecting the entirety that belongs in combination in a single position.
OOP additionally we could categories use options from different categories, that means you don’t have to put in writing the similar code many times. A majority of these help in making the code cleaner, more uncomplicated to mend, and higher arranged.
Transparent code from OOP is helping groups paintings higher in combination. It’s more uncomplicated for everybody to know what the code does, that means much less time explaining issues and extra time doing the paintings. It additionally reduces errors, serving to the venture keep on target. And when code is neat and orderly, new crew participants can briefly catch up.
Imposing OOP in PHP
In OOP for PHP, you arrange code with categories and gadgets, like blueprints and homes. You create categories for the entirety (like customers or books), together with their traits and movements. Then, you employ inheritance to construct new categories from present ones, saving time through now not repeating code. And since encapsulation assists in keeping some magnificence portions non-public, your code is more secure.
The next sections stroll you thru tips on how to use OOP ideas successfully on your PHP programming. We create a content material control gadget (CMS) for managing articles.
1. Outline a category with houses and techniques
Get started with an Article
magnificence that comes with name, content material, and standing houses — together with set and show those houses.
magnificence Article {
non-public $name;
non-public $content material;
non-public $standing;
const STATUS_PUBLISHED = 'printed';
const STATUS_DRAFT = 'draft';
public serve as __construct($name, $content material) {
$this->name = $name;
$this->content material = $content material;
$this->standing = self::STATUS_DRAFT;
}
public serve as setTitle($name) {
$this->name = $name;
go back $this;
}
public serve as setContent($content material) {
$this->content material = $content material;
go back $this;
}
public serve as setStatus($standing) {
$this->standing = $standing;
go back $this;
}
public serve as show() {
echo "{$this->name}
{$this->content material}
Standing: {$this->standing}";
}
}
2. Create gadgets and enforce way chaining
Create an editorial object and use way chaining to set its houses:
$article = new Article("OOP in PHP", "Object-Orientated Programming ideas.");
$article->setTitle("Complicated OOP in PHP")->setContent("Exploring complex ideas in OOP.")->setStatus(Article::STATUS_PUBLISHED)->show();
3. Fortify encapsulation and inheritance
Improve encapsulation through the use of getter and setter strategies and create a FeaturedArticle
magnificence that inherits from Article
:
magnificence FeaturedArticle extends Article {
non-public $highlightColor = '#FFFF00'; // Default spotlight colour
public serve as setHighlightColor($colour) {
$this->highlightColor = $colour;
go back $this;
}
public serve as show() {
echo " taste='background-color: {$this->highlightColor};'>";
father or mother::show();
echo "";
}
}
$featuredArticle = new FeaturedArticle("Featured Article", "It is a featured article.");
$featuredArticle->setStatus(FeaturedArticle::STATUS_PUBLISHED)->setHighlightColor('#FFA07A')->show();
4. Interfaces and polymorphism
Outline an interface for publishable content material and enforce it within the Article
magnificence to reveal polymorphism:
interface Publishable {
public serve as submit();
}
magnificence Article implements Publishable {
// Present magnificence code...
public serve as submit() {
$this->setStatus(self::STATUS_PUBLISHED);
echo "Article '{$this->name}' printed.";
}
}
serve as publishContent(Publishable $content material) {
$content->submit();
}
publishContent($article);
5. Use characteristics for shared conduct
PHP means that you can use characteristics so as to add purposes to categories while not having to inherit from any other magnificence. The usage of the code beneath, introduce a trait for logging actions throughout the CMS:
trait Logger {
public serve as log($message) {
// Log message to a document or database
echo "Log: $message";
}
}
magnificence Article {
use Logger;
// Present magnificence code...
public serve as submit() {
$this->setStatus(self::STATUS_PUBLISHED);
$this->log("Article '{$this->name}' printed.");
}
}
OOP in WordPress building
OOP ideas considerably make stronger WordPress building, specifically when developing topics, plugins, and widgets. With the assistance of OOP, you’ll be able to write cleaner, scalable, and extra maintainable code to your WordPress websites.
This phase opinions tips on how to follow OOP in WordPress building. We’ll supply examples you’ll be able to reproduction and paste right into a WordPress deployment for checking out.
OOP in WordPress topics: Customized publish sort registration
To reveal the use of OOP in WordPress topics, you create a category that handles the registration of a customized publish sort.
Position the next code on your theme’s purposes.php document. You’ll to find your topics within the wp-content/topics listing.
magnificence CustomPostTypeRegistrar {
non-public $postType;
non-public $args;
public serve as __construct($postType, $args = []) {
$this->postType = $postType;
$this->args = $args;
add_action('init', array($this, 'registerPostType'));
}
public serve as registerPostType() {
register_post_type($this->postType, $this->args);
}
}
// Utilization
$bookArgs = [
'public' => true,
'label' => 'Books',
'supports' => ['title', 'editor', 'thumbnail'],
'has_archive' => true,
];
new CustomPostTypeRegistrar('e-book', $bookArgs);
This code dynamically registers a customized publish sort e-book
with its main points handed thru the use of the bookArgs
array. You’ll see the newly created customized publish sort within the WordPress admin sidebar categorised Books.
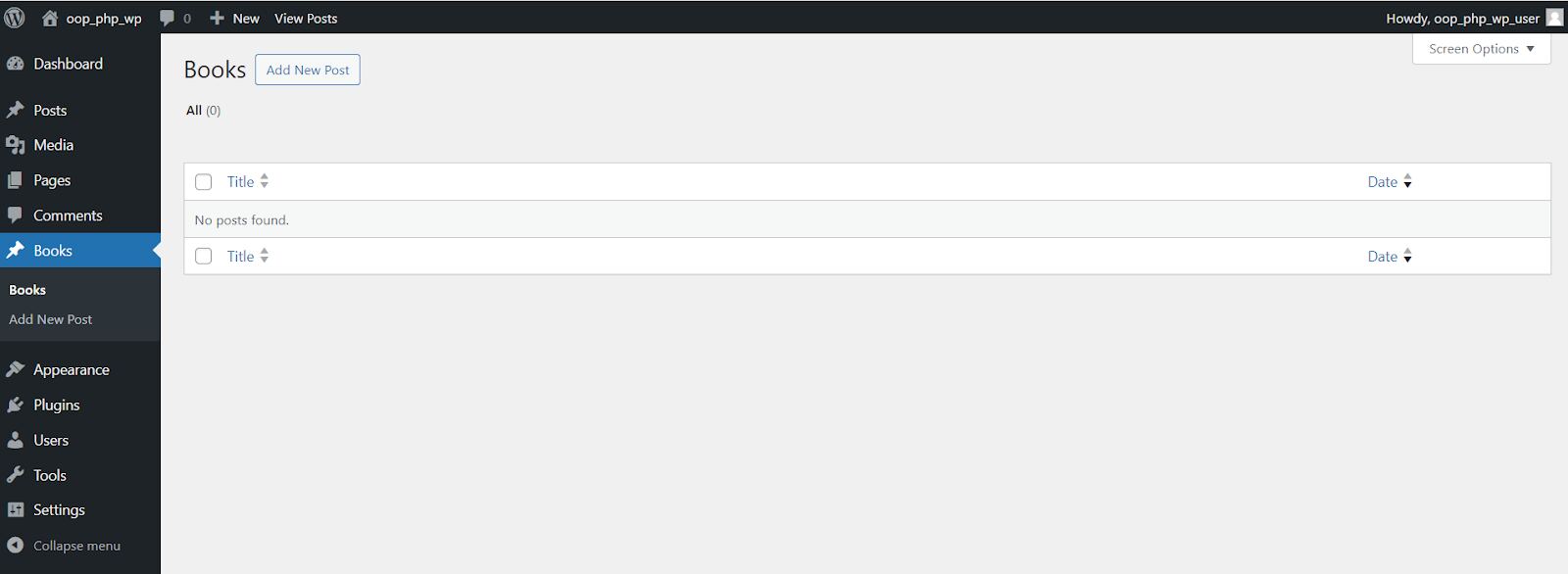
This case illustrates how OOP can encapsulate the capability for registering customized publish varieties, making it reusable for several types of posts.
OOP in WordPress plugins: Shortcode handler
For a plugin instance, you expand a category that handles a shortcode for showing a unique message. You’ll take a look at this option through including the shortcode beneath to any publish or web page.
'Hi, that is your OOP
message!'], $atts);
go back "{$attributes['message']}";
}
}
new OOPShortcodeHandler();
Save this as my-oop-shortcode-handler.php within the wp-content/plugins listing. After all, turn on the plugin.
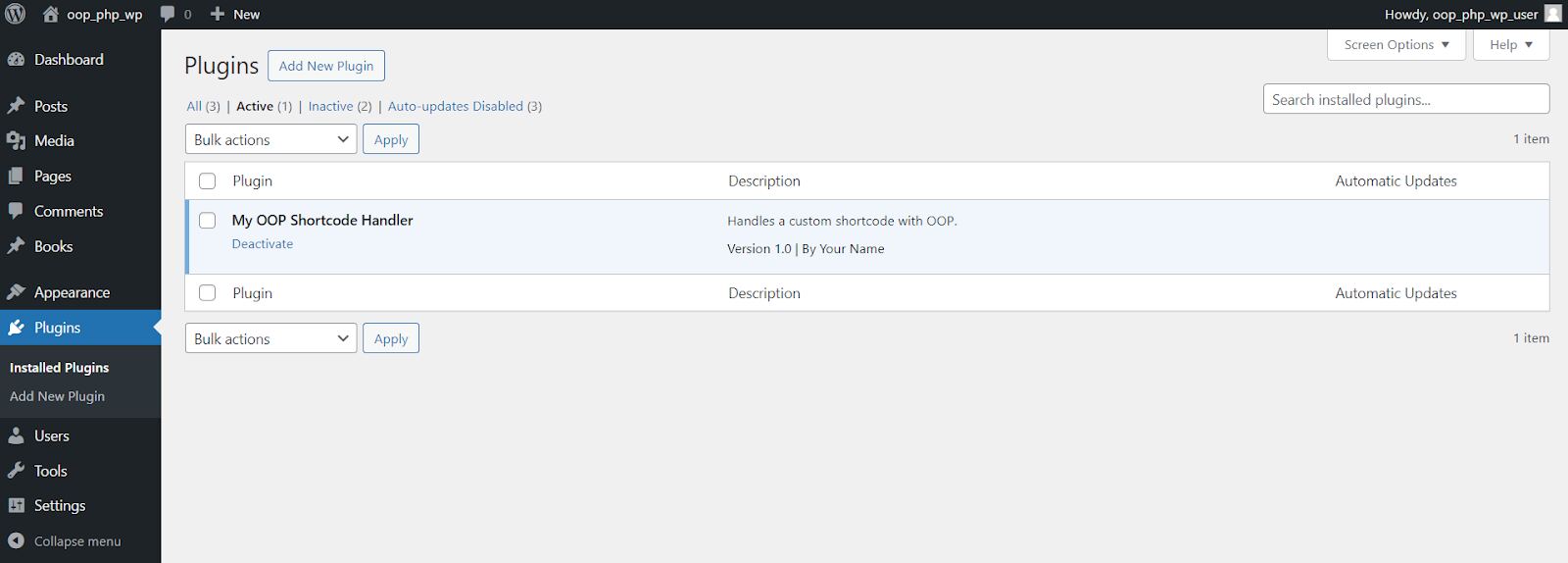
Subsequent, within the web page or publish editor sooner than publishing or updating, use the shortcodes [oop_message]
and [oop_message message="Custom Message Here"]
, as proven beneath:
After publishing or updating the web page/publish, you notice the message that the shortcodes displayed denote.
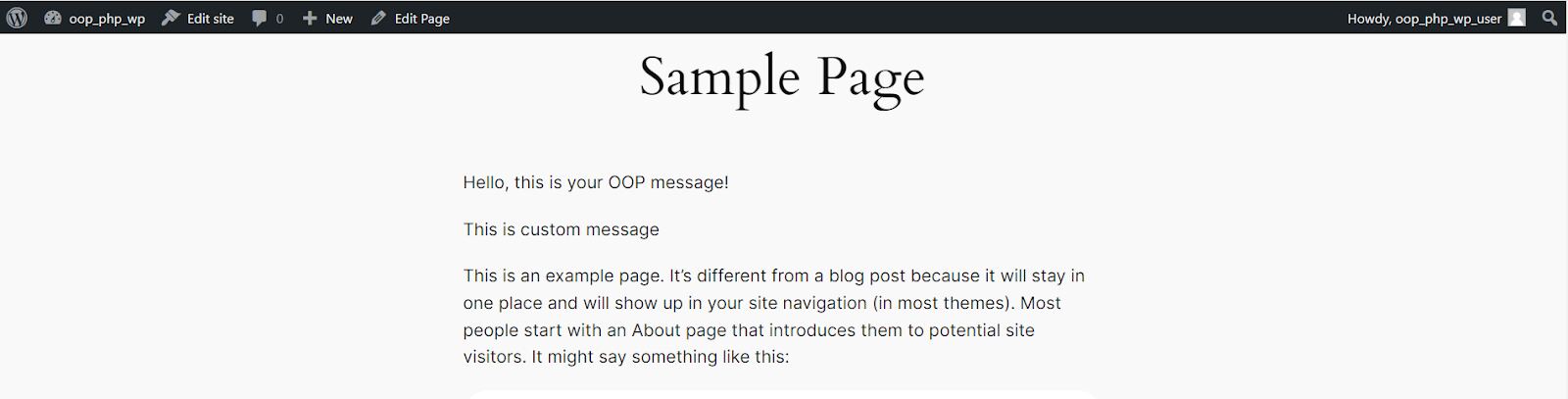
OOP in WordPress widgets: dynamic content material widget
OOP may be recommended for widgets through encapsulating their capability inside of categories. WordPress core itself makes use of OOP for widgets. Right here, you create a customized widget that permits customers to show dynamic content material with a name and textual content space.
Upload the next code on your theme’s purposes.php document or inside of a plugin. It defines a customized widget that shows the message “Hi International From My Customized Widget!”
magnificence My_Custom_Widget extends WP_Widget {
public serve as __construct() {
father or mother::__construct(
'my_custom_widget', // Base ID
'My Customized Widget', // Identify
array('description' => __('A easy customized widget.',
'text_domain'),) // Args
);
}
public serve as widget($args, $example) {
echo $args['before_widget'];
if (!empty($example['title'])) {
echo $args['before_title'] . apply_filters('widget_title',
$example['title']) . $args['after_title'];
}
// Widget content material
echo __('Hi International From My Customized Widget!', 'text_domain');
echo $args['after_widget'];
}
public serve as shape($example) {
// Shape in WordPress admin
}
public serve as replace($new_instance, $old_instance) {
// Processes widget choices to be stored
}
}
serve as register_my_custom_widget() {
register_widget('My_Custom_Widget');
}
add_action('widgets_init', 'register_my_custom_widget');
Whilst modifying the lively theme the use of the Customise hyperlink below the Look on Admin space, you’ll be able to upload a brand new customized widget anywhere wanted.
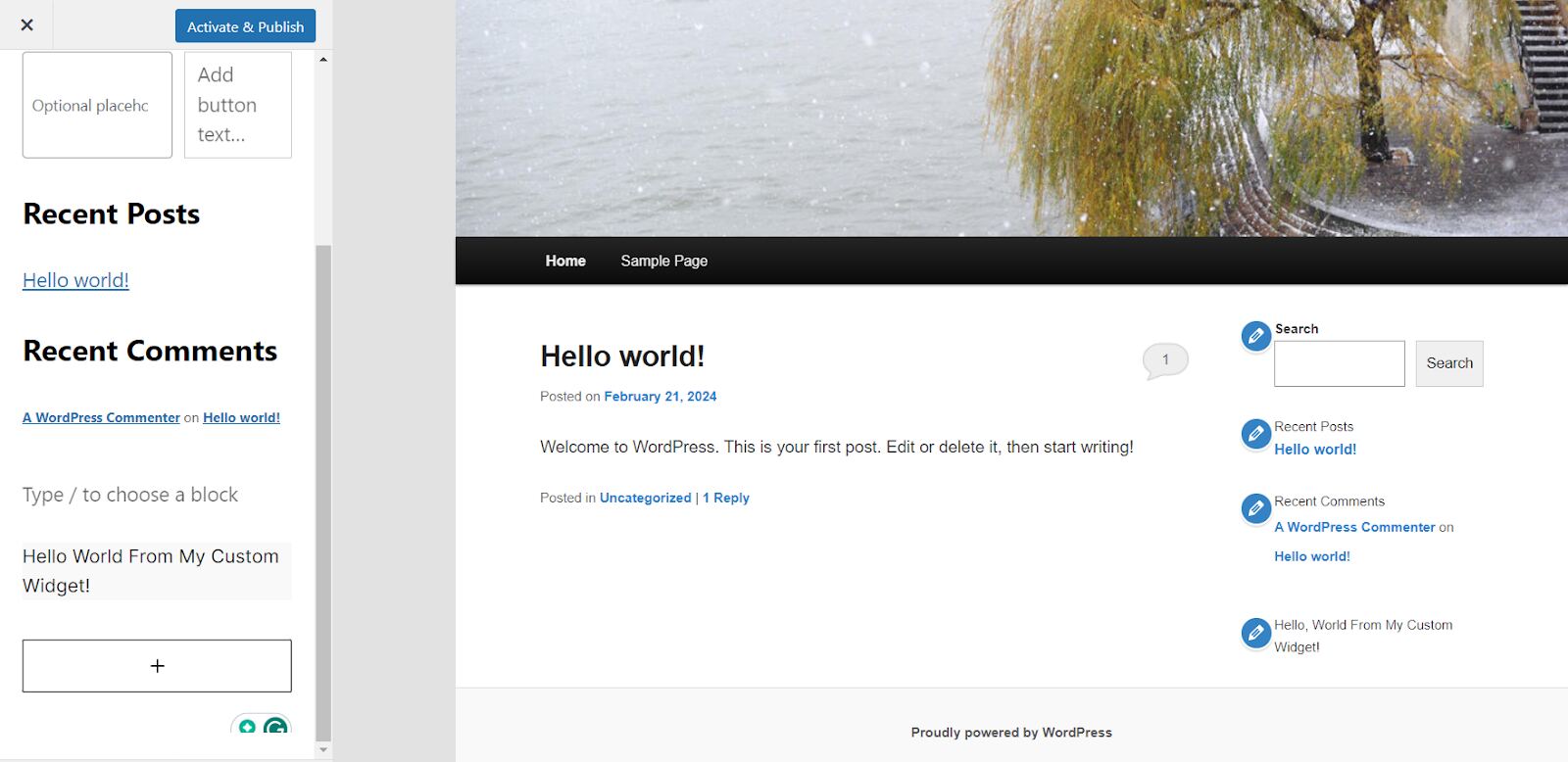
Using WordPress categories
WordPress supplies quite a lot of categories that you’ll be able to use to engage with the CMS’s core capability. Two such categories are WP_User
and WP_Post
, which constitute customers and posts, respectively.
Extending the WordPress plugin instance from above, incorporate those categories to create a shortcode that shows details about the creator of a publish and information about the publish itself.
Save this as my-oop-shortcode-handler-extended.php within the wp-content/plugins listing. After all, turn on the plugin.
$post->ID, // Default to the present publish ID
], $atts);
$postDetails = get_post($attributes['post_id']); // Getting the WP_Post
object
if (!$postDetails) {
go back "Put up now not discovered.";
}
$authorDetails = new WP_User($postDetails->post_author); // Getting the
WP_User object
$output = "
";
go back $output;
}
}
new ExtendedOOPShortcodeHandler();
On this prolonged model, you’ve created a shortcode: [post_author_details post_id="1"]
.
When added to a publish or web page, it shows information about the publish’s creator (the use of the WP_User
magnificence) and the publish itself (the use of the WP_Post
magnificence).
OOP and the WordPress REST API
The WordPress REST API is a contemporary addition to WordPress that permits you to have interaction with the web site’s knowledge in a extra programmable method. It leverages OOP broadly, using categories to outline the endpoints, responses, and the dealing with of requests. This represents a transparent transfer from its conventional procedural roots to embracing OOP.
Contrasting sharply with OOP ideas, a lot of WordPress’s core, particularly its previous elements reminiscent of the subjects and plugins API, is written in a procedural programming taste.
For instance, whilst a procedural manner would possibly without delay manipulate world variables and depend on a series of purposes for duties, OOP within the REST API encapsulates the good judgment inside of categories. Due to this fact, particular strategies inside of those categories care for duties reminiscent of fetching, developing, updating, or deleting posts.
This obviously separates issues and makes the codebase more uncomplicated to increase and debug.
Thru categories that outline endpoints and care for requests, reminiscent of fetching posts with a GET /wp-json/wp/v2/posts
request, the REST API gives a structured, scalable technique to have interaction with WordPress knowledge, returning JSON formatted responses.
Leveraging Kinsta for PHP and WordPress internet hosting
Kinsta’s internet hosting answers assist optimize PHP programs and arrange WordPress internet hosting, catering to standard PHP setups and WordPress-specific wishes. With a strong infrastructure constructed on state of the art applied sciences like Google Cloud, Kinsta facilitates extraordinary efficiency and scalability for OOP-based PHP programs.
For WordPress websites, Kinsta’s specialised WordPress Internet hosting provider gives options like computerized updates, safety tracking, and skilled make stronger. It promises hassle-free control and awesome efficiency, making it ideally suited for builders and companies alike.
Abstract
As you’ve observed right through this newsletter, OOP gives extraordinary flexibility, scalability, and maintainability in PHP and WordPress building.
As a number one controlled WordPress internet hosting supplier, Kinsta acknowledges this want and provides adapted answers to make stronger builders such as you on your OOP PHP endeavors. That’s why our Utility and WordPress Internet hosting answers are designed to help you in development performant, scalable, OOP-based WordPress initiatives.
Check out Kinsta nowadays to look how we will be able to allow you to construct top-tier WordPress websites!
Have you ever skilled the advantages of OOP on your initiatives? Or are you interested by the way it can change into your building procedure? Percentage within the feedback beneath.
The publish Object-oriented programming in PHP: reworking WordPress building gave the impression first on Kinsta®.
WP Hosting