The Vue framework for JavaScript has change into common for development consumer interfaces and single-page programs (SPAs). To make sure your huge apps serve as optimally, you want a company grab of state control — the method of managing and centralizing an software’s reactive information (state) throughout a couple of parts.
In Vue, state control has lengthy depended on Vuex, a library with a centralized retailer for all an software’s parts. Then again, fresh developments within the Vue ecosystem have given upward thrust to Vuex’s successor, Pinia.
Pinia provides a extra light-weight, modular, and intuitive control way. It integrates seamlessly with Vue’s reactivity device and Composition API, making it painless for builders to regulate and get admission to shared state in a scalable and maintainable means.
Surroundings the scene: Pinia vs Vuex
As a go-to library for state control in Vue programs, Vuex equipped a centralized retailer for all an software’s parts. Then again, with the development of Vue, Pinia represents a extra trendy resolution. Let’s discover the way it differs from Vuex.
- API variations — Pinia’s Composition API provides a extra basic and intuitive API than Vuex, making it easier to regulate the applying state. Additionally, its construction very much resembles Vue’s Choices API, acquainted to maximum Vue builders.
- Typing make stronger — Traditionally, many Vue builders have struggled with Vuex’s restricted sort make stronger. By contrast, Pinia is a completely typed state control library that gets rid of those issues. Its sort protection is helping save you doable runtime mistakes, contributes to code clarity, and facilitates smoother scaling features.
- Reactivity device — Each libraries leverage Vue’s reactivity device, however Pinia’s way aligns extra intently with Vue 3’s Composition API. Whilst the reactivity API is robust, managing complicated states in huge programs will also be difficult. Thankfully, Pinia’s easy and versatile structure eases the effort of state control on your Vue 3 programs. Pinia’s retailer development permits you to outline a shop for managing a particular portion of the applying state, simplifying its group and sharing it throughout parts.
- Light-weight nature — At simply 1 KB, Pinia integrates seamlessly into your dev atmosphere, and its light-weight nature would possibly beef up your software efficiency and cargo instances.
Tips on how to arrange a Vue mission with Pinia
To combine Pinia in a Vue mission, initialize your mission with Vue CLI or Vite. After mission initialization, you’ll set up Pinia by the use of npm or yarn as a dependency.
- Create a brand new Vue mission the use of Vue CLI or Vite. Then, apply the activates to arrange your mission.
// The use of Vue CLI vue create my-vue-ap // The use of Vite npm create vite@newest my-vue-app -- --template vue
- Trade your listing to the newly created mission folder:
cd my-vue-app
- Set up Pinia as a dependency on your mission.
// The use of npm npm set up pinia // The use of yarn yarn upload pinia
- To your major access document (in most cases major.js or major.ts), import Pinia and inform Vue to make use of it:
import { createApp } from 'vue'; import { createPinia } from 'pinia'; import App from './App.vue'; const app = createApp(App); app.use(createPinia()); app.mount('#app');
With Pinia put in and arrange on your Vue mission, you’re in a position to outline and use shops for state control.
Tips on how to create a shop in Pinia
The shop is the spine of state control on your Pinia-powered Vue software. It is helping you organize application-wide information in a cohesive and coordinated means. The shop is the place you outline, retailer, and organize the information to percentage throughout your software’s quite a lot of parts.
This centralization is significant, because it constructions and organizes your app’s state adjustments, making information float extra predictable and easy to debug.
Additionally, a shop in Pinia does greater than cling the state: Pinia’s incorporated functionalities permit you to replace the state by the use of movements and compute derived states by the use of getters. Those integrated features give a contribution to a extra environment friendly and maintainable codebase.
The next instance illustrates making a elementary Pinia retailer in a mission’s src/retailer.js document.
import { defineStore } from 'pinia';
export const useStore = defineStore('major', {
state: () => ({
depend: 0,
}),
movements: {
increment() {
this.depend++;
},
},
getters: {
doubleCount: (state) => state.depend * 2,
},
});
Tips on how to get admission to retailer state in parts
In comparison to Vuex, Pinia’s solution to state get admission to and control is extra intuitive, particularly if you happen to’re aware of Vue 3’s Composition API. This API is a collection of a number of that allow the inclusion of reactive and composable good judgment on your parts.
Imagine the next code.
{{ retailer.depend }}
Within the above snippet, the tag comprises your element’s outlined HTML markup. To show the price of the
depend
assets from the Pinia retailer, you utilize Vue’s information binding syntax, expressed as {{ depend }}
.
The useStore
serve as supplies get admission to to the Pinia retailer, so that you import it from retailer.js the use of import { useStore } from './retailer';
.
A characteristic of Vue 3’s Composition API, the setup
serve as defines your element’s reactive state and good judgment. Throughout the serve as, then you name useStore()
to get admission to the Pinia retailer.
Subsequent, const depend = retailer.depend
accesses the shop’s depend
assets, making it to be had within the element.
In the end, setup
returns the depend
, permitting the template to render it. Vue’s reactivity device signifies that your element’s template will replace the price of depend
every time it adjustments within the retailer.
Beneath is a screenshot of the output.
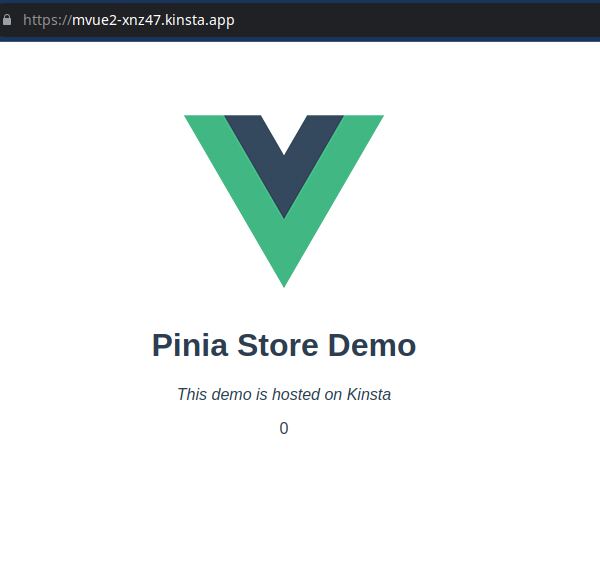
This situation illustrates Pinia’s benefits:
- Simplicity — Pinia permits direct get admission to to the shop’s state with out mapping purposes. By contrast, Vuex wishes
mapState
(or equivalent helpers) to reach the similar get admission to. - Direct retailer get admission to — Pinia permits you to get admission to state homes (like
retailer.depend
) at once, making your code extra readable and understandable. In the meantime, Vuex regularly calls for getters to get admission to even basic homes, including complexity that diminishes clarity. - Composition API compatibility — Because the setup way demonstrates, Pinia’s integration with the Composition API aligns particularly smartly with trendy Vue construction, offering a extra uniform coding enjoy.
Tips on how to alter state with Pinia
In Pinia, you alter a shop’s state the use of movements, that are extra versatile than Vuex mutations. Imagine the next serve as name, which increments the state’s depend
assets:
retailer.increment(); // Increments the depend
However, a Vuex an identical comes to defining a mutation along with a minimum of one motion:
mutations: {
increment(state) {
state.depend++;
},
},
movements: {
increment({ dedicate }) {
dedicate('increment');
},
}
The Pinia motion and its an identical Vuex code exemplify a a very powerful distinction between the libraries’ code complexity. Let’s discover those variations additional:
- Direct as opposed to oblique state mutation — Because the
increment
motion demonstrates, Pinia movements at once mutate the shop’s state. In Vuex, you’ll best alternate the state the use of mutations, which you should dedicate with movements. This procedure separation guarantees your state adjustments are trackable, however it’s extra complicated and inflexible than related Pinia movements. - Asynchronous as opposed to synchronous operations — While Vuex mutations are at all times synchronous and will’t include asynchronous processes, Pinia movements can include synchronous and asynchronous code. In consequence, you’ll carry out API calls or different asynchronous operations at once inside movements, making for a leaner and extra concise codebase.
- Simplified syntax — Vuex regularly calls for defining mutations and calling movements to dedicate them. Pinia does away with this want. The power to mutate the state inside movements reduces boilerplate code and assists in keeping your present code easier. In Vuex, elementary state updates require defining an motion and a mutation, although the motion is simply committing the mutation.
The transition from Vuex to Pinia
Transitioning to Pinia may give a large number of advantages in simplicity, flexibility, and maintainability, nevertheless it calls for cautious making plans and attention to verify a a hit implementation.
Earlier than making the transfer, make sure that you:
- Get yourself up to speed with the diversities between Pinia’s and Vuex’s structure, state control patterns, and APIs. Figuring out those variations is a very powerful to successfully refactor your code and take complete benefit of Pinia’s options.
- Analyze and refactor your Vuex state, movements, mutations, and getters to suit into Pinia’s construction. Have in mind, in Pinia, you outline state as a serve as. You’ll be able to at once mutate states with movements and will put into effect getters extra easily.
- Plan transition your Vuex retailer modules. Pinia doesn’t use modules in the similar means as Vuex, however you’ll nonetheless construction your shops to serve equivalent functions.
- Leverage Pinia’s progressed TypeScript make stronger. In case your mission makes use of TypeScript, imagine Pinia’s enhanced sort inference and typing features for higher sort protection and developer enjoy.
- Revise your checking out methods to deal with adjustments in state control. This procedure may contain updating the way you mock shops or movements on your unit and integration exams.
- Imagine how the transition impacts your mission’s construction and group. Account for elements like naming conventions and the way you import and use shops throughout parts.
- Make certain compatibility with different libraries. Take a look at for any required updates or dependency adjustments that the changeover may have an effect on.
- Evaluation any efficiency adjustments. Pinia is typically extra light-weight than Vuex, however proceed tracking your software’s efficiency throughout and after the transition to verify there are not any problems.
Changing a shop from Vuex to Pinia comes to a number of steps to deal with variations of their constructions and APIs. Imagine the Pinia retailer from previous.
The similar retailer in a Vuex retailer.js document seems as follows.
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Retailer({
state: {
depend: 0,
},
mutations: {
increment(state) {
state.depend++;
},
},
movements: {
increment(context) {
context.dedicate('increment');
},
},
getters: {
doubleCount(state) {
go back state.depend * 2;
},
},
});
As with the former Pinia retailer, this Vuex instance comprises a state
object with a unmarried depend
assets initialized to 0
.
The mutations
object comprises how you can at once mutate the state, whilst the movements
object’s strategies dedicate the increment
mutation.
Then, the getters
object holds the doubleCount
way, which multiplies the depend
state through 2
and returns the outcome.
As this code demonstrates, enforcing a shop in Pinia comes to a number of noticeable variations to Vuex:
- Initialization — Pinia does no longer require
Vue.use()
. - Construction — In Pinia, the state is a serve as returning an object, making an allowance for higher TypeScript make stronger and reactivity.
- Movements — Movements in Pinia are strategies that at once mutate the state while not having mutations, simplifying the code.
- Getters — Whilst very similar to Vuex, Getters in Pinia are outlined within the retailer definition and will at once get admission to the state.
Tips on how to use the shop in parts
With Vuex, chances are you’ll use the shop like this:
{{ doubleCount }}
For Pinia, the utilization turns into:
{{ retailer.doubleCount }}
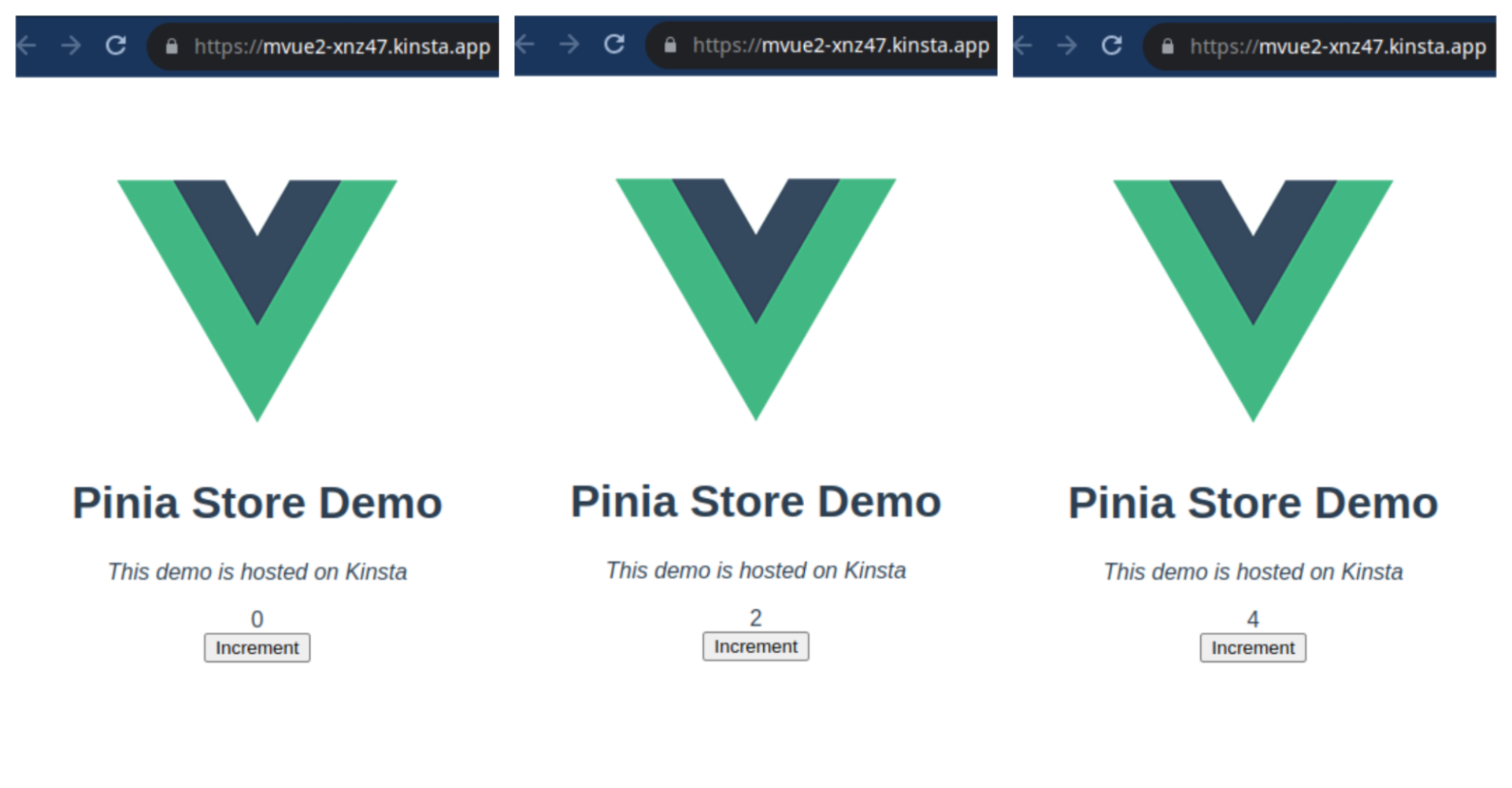
This situation covers a elementary conversion. For extra complicated Vuex shops, particularly the ones the use of modules, the conversion would contain a extra detailed restructuring to align with Pinia’s structure.
Tips on how to deploy your Vue software
Earlier than deploying, join a unfastened trial of Kinsta’s Utility Webhosting carrier. You’ll deploy the applying with the assistance of a Dockerfile.
Create a Dockerfile at your mission’s root and paste within the following contents:
FROM node:newest
WORKDIR /app
COPY bundle*.json ./
RUN npm set up
COPY ./ .
CMD ["npm", "run", "start"]
This code instructs Kinsta’s Docker engine to put in Node.js (FROM node:newest
), create the operating listing (WORKDIR /app
), set up the node modules from the bundle.json document (RUN npm set up
) and set the get started (CMD ["npm", "run", "start"]
) command that can be invoked when the Vue app begins. The COPY
instructions replica the desired information or directories to the operating listing.
After that, push your code to a most well-liked Git supplier (Bitbucket, GitHub, or GitLab). As soon as your repo is in a position, apply those steps to deploy your app to Kinsta:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta together with your Git supplier.
- Choose Utility at the left sidebar and click on the Upload Utility button.
- Within the modal that looks, make a selection the repository you wish to have to deploy. You probably have a couple of branches, you’ll choose the specified department and provides a reputation in your software.
- Choose some of the to be had information middle places.
- Make a selection your construct atmosphere and choose Use Dockerfile to arrange container symbol.
- In case your Dockerfile isn’t on your repo’s root, use Context to suggest its trail and click on Proceed.
- You’ll be able to depart the Get started command access empty. Kinsta makes use of
npm get started
to begin your software. - Choose the pod dimension and selection of cases best possible suited in your app and click on Proceed.
- Fill out your bank card data and click on Create software.
As a substitute for software internet hosting, you’ll go for deploying your Vue software as a static web site with Kinsta’s static web site internet hosting totally free.
Abstract
The transition from Vuex to Pinia marks an important evolution in state control inside the Vue ecosystem. Pinia’s simplicity, progressed TypeScript make stronger, and alignment with Vue 3’s Composition API make it a compelling selection for contemporary Vue programs.
While you’re in a position to host your Vue software with Kinsta, you’ll get admission to a quick, safe, and dependable infrastructure. Join Kinsta and use our software internet hosting carrier.
The put up Grasp state control in Vue.js gave the impression first on Kinsta®.
WP Hosting