WordPress has existed for over twenty years, powering 42.7% of all of the web pages at the Web, consistent with W3Techs. It additionally holds a 62.5% marketplace proportion for content material control techniques (CMSs) in the back of web pages.
As of late, many programming languages and frameworks are to be had to construct user-friendly, high-performance web pages which are means quicker than WordPress, it doesn’t matter what optimization you do on your WordPress dashboard. One instance is Subsequent.js, a well-liked React framework.
This information presentations easy methods to use WordPress as a headless CMS, offering information in your Subsequent.js software. It additionally explains deploying your Subsequent.js code as a static web site to Kinsta’s loose Static Website Internet hosting provider.
Working out headless WordPress
Headless WordPress refers to the usage of WordPress only for its backend features — managing and storing content material — and the usage of a separate machine, like Subsequent.js, for the frontend presentation.
This decoupling permits builders to make use of WordPress’s powerful content material control equipment whilst taking complete benefit of fashionable frontend building options, akin to server-side rendering and static web site era in Subsequent.js.
Making ready your WordPress web site
Ahead of diving into the Subsequent.js building, your WordPress web site wishes a bit of of preparation to function a headless CMS.
When you don’t have already got a WordPress web site, you’ll be able to create one simply with Kinsta. There are 3 tips on how to construct a WordPress web site the usage of Kinsta:
- Create a web site to your native device (most likely the usage of our DevKinsta equipment) prior to transferring the web site to our servers
- Create a web site remotely the usage of the MyKinsta dashboard
- Create a web site remotely the usage of the Kinsta API
After you have a WordPress web site, there are two approaches to fetching information out of your WordPress CMS into your frontend framework: WPGraphQL and REST API.
The REST API facilitates information retrieval in JSON structure the usage of JavaScript approaches just like the Fetch API or the Axios library. The REST API has been constructed into WordPress since model 4.7, which means it does no longer require any plugin to paintings. However to make use of WPGraphQL, which lets you have interaction along with your WordPress information the usage of GraphQL question, you will have to set up the WPGraphQL plugin.
Let’s use the REST API for this information. To get your WordPress information in a JSON structure, append /wp-json/wp/v2
in your WordPress web site URL:
http://yoursite.com/wp-json/wp/v2
If the JSON API isn’t enabled whilst you consult with http://yoursite.com/wp-json
by way of default, you’ll be able to allow it by way of opening Permalinks underneath Settings within the WordPress dashboard and deciding on Publish Title or another certainly one of your selection with the exception of Simple:
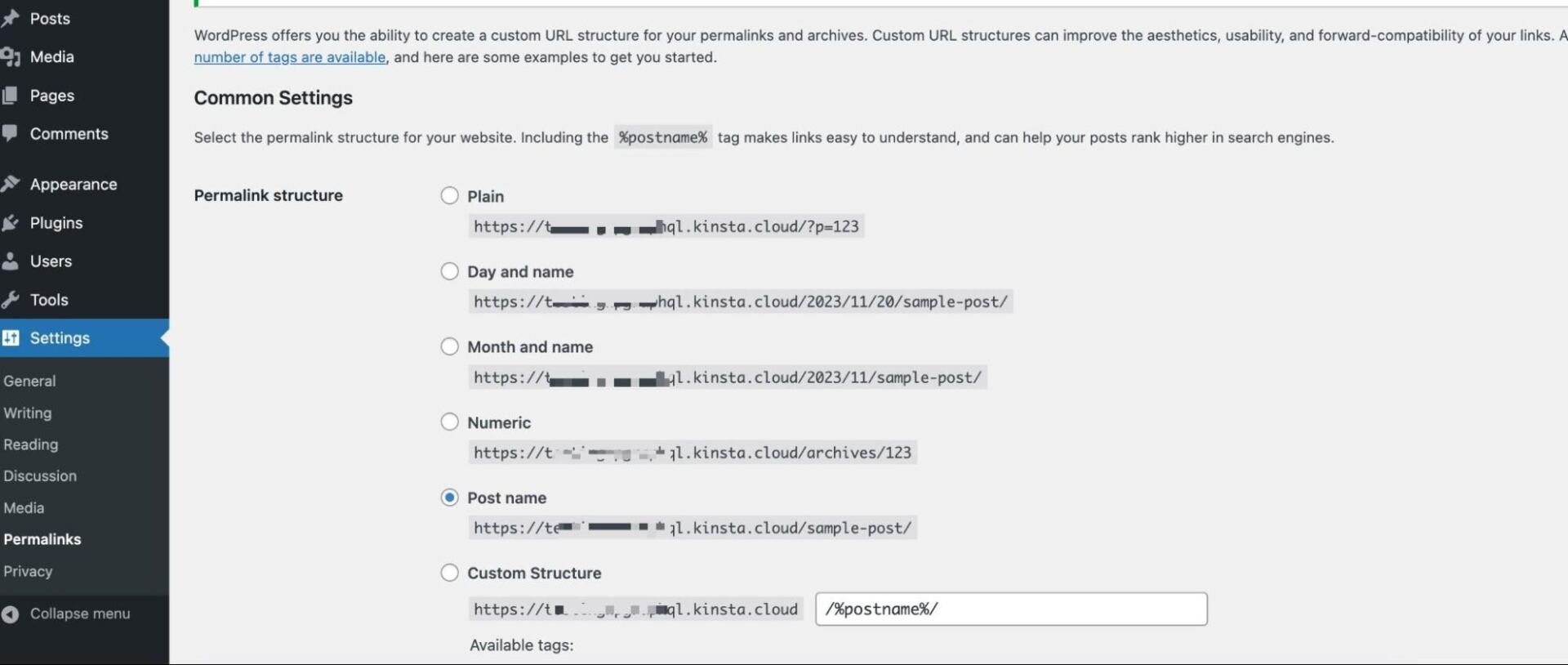
This works for native and public WordPress websites, providing endpoints for content material that incorporates posts, pages, feedback, and media. Learn our entire information to the REST API to be told extra.
Putting in place your Subsequent.js surroundings
Subsequent.js is helping builders construct internet programs conveniently, improving functionality and optimizing the improvement enjoy. One in every of its key options is file-based routing, which simplifies the advent of routes.
Subsequent.js additionally focuses closely on functionality, providing options akin to automated code splitting, which quite a bit most effective the important JavaScript for every web page, considerably decreasing the weight time.
To arrange a Subsequent.js mission, you’ll be able to run the next command and use its default responses:
npx create-next-app@newest nextjs-wp-demo
For this information, you’ll be able to snatch our Git starter template by way of following those steps:
- Seek advice from this mission’s GitHub repository.
- Make a selection Use this template > Create a brand new repository to replicate the starter code right into a repository inside your GitHub account (take a look at the field to come with all branches).
- Pull the repository in your native laptop and turn to the starter-files department the usage of the command:
git checkout starter-files
.
- Set up the important dependencies by way of working the command
npm set up
.
As soon as the set up is entire, release the mission to your native laptop with npm run dev
. This makes the mission to be had at http://localhost:3000/.
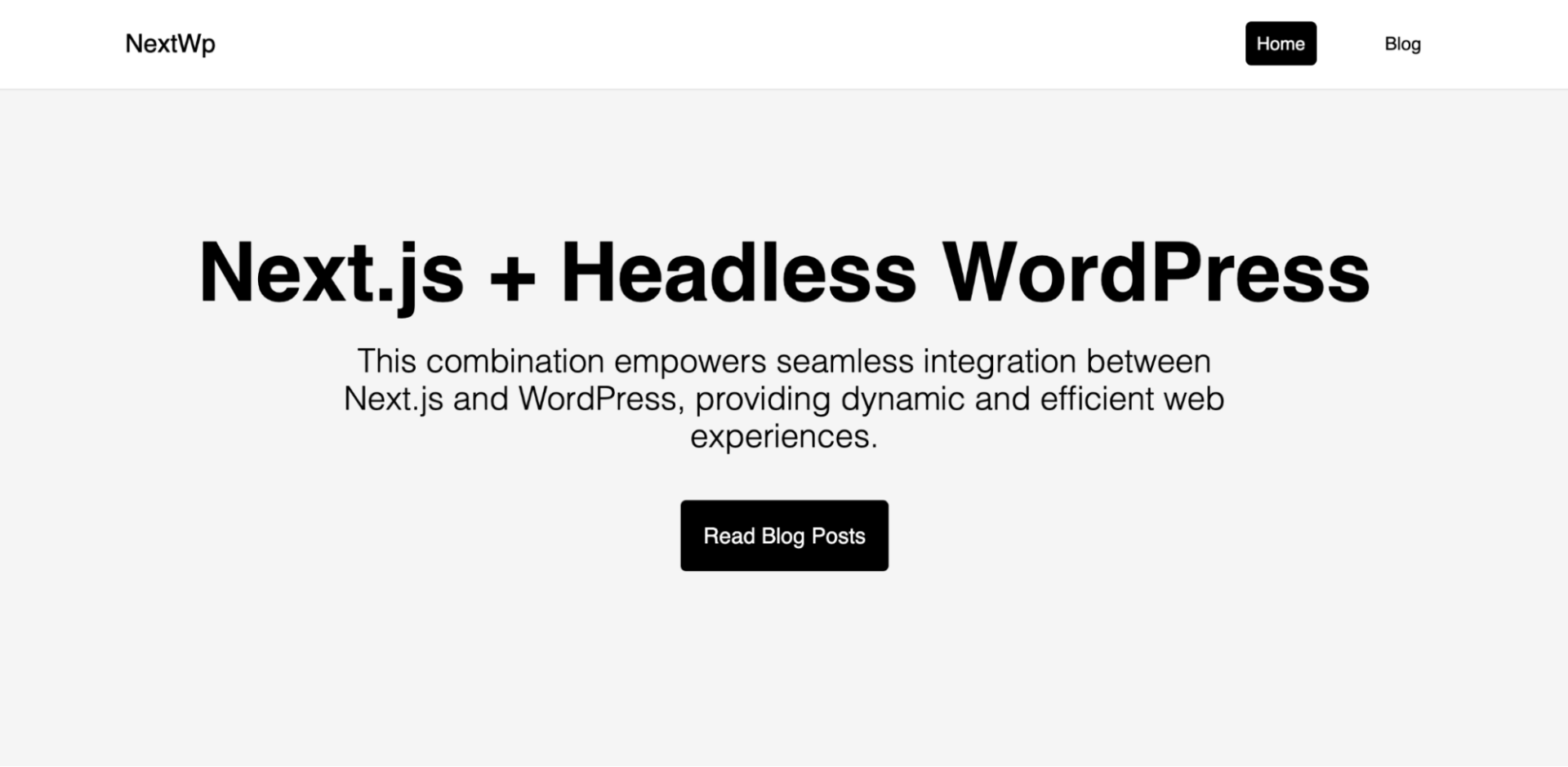
Working out the mission
The App Router used to be presented in Subsequent.js 13, changing the prevailing pages listing for routing. Routing with the App Router additionally comes to growing folders inside the app listing. Then, you nest a web page.js dossier inside the proper folder to outline your course.
On this mission, app is the core listing you might be interacting with, and you’re going to to find the next dossier construction.
/
|-- /app
|-- /weblog
|-- /[postId]
|-- web page.js
|-- web page.js
|-- globals.css
|-- structure.js
|-- navbar.js
|-- web page.js
3 pages are created: the house web page to show fundamental data, the weblog web page to show all posts out of your WordPress CMS, and the dynamic web page ([postId]/web page.js) for rendering particular person posts.
You’re going to additionally realize the navbar.js element, which is imported into the structure.js dossier to create a structure for the mission.
Fetching information from WordPress to Subsequent.js
With the WordPress REST API, you’ll be able to fetch posts, pages, and customized publish sorts by way of sending HTTP requests to precise endpoints.
Let’s make a fetch request within the weblog/web page.js dossier to fetch all posts on your WordPress CMS after which in spite of everything make a request to fetch every publish dynamically within the weblog/[postId]/web page.js in response to the identity
parameter handed.
Ahead of we make those requests, it’s just right observe so as to add your JSON API deal with to an atmosphere variable. This means guarantees your API base URL is definitely configurable and no longer hardcoded throughout more than one information.
Create a .env dossier within the root of your Subsequent.js mission and upload the next:
NEXT_PUBLIC_WORDPRESS_API_URL=https://yoursite.kinsta.cloud/wp-json/wp/v2
Make sure you substitute the URL along with your web site’s JSON API. Additionally, upload .env
in your .gitignore dossier so it does no longer push the dossier in your Git supplier.
Fetch all posts from WordPress to Subsequent.js
To fetch all posts out of your WordPress web site, create an asynchronous serve as named getPosts
on your weblog/web page.js dossier. This serve as makes use of the Fetch API to make a GET request to the /posts
endpoint of your WordPress REST API.
async serve as getPosts() {
const reaction = watch for fetch(
`${procedure.env.NEXT_PUBLIC_WORDPRESS_API_URL}/posts`
);
const posts = watch for reaction.json();
go back posts;
}
Upon receiving the reaction, it converts the reaction to JSON, development an array of publish gadgets. Those posts
can also be rendered on your Subsequent.js software, offering a dynamic checklist of weblog posts fetched immediately from WordPress.
const BlogPage = async () => {
const posts = watch for getPosts();
go back (
All Weblog Posts
All weblog posts are fetched from WordPress by the use of the WP REST API.
{posts.map((publish) => {
go back (
{publish.name.rendered}
);
})}
);
};
Throughout the Subsequent.js web page element, name getPosts
asynchronously to fetch the posts. Then, map
over the array of posts
, rendering every publish’s name
and excerpt
inside a element.
This no longer most effective presentations the posts but in addition wraps every in a hyperlink that navigates to an in depth view of the publish. That is accomplished by way of the usage of Subsequent.js’s file-based routing, the place the publish ID is used to generate the URL trail dynamically.
Fetch dynamic posts from WordPress to Subsequent.js
Within the code above, every publish is wrapped in a hyperlink this is anticipated to assist customers navigate to an in depth view of the publish.
For particular person publish pages, you employ dynamic routing in Subsequent.js to create a web page that fetches and presentations a unmarried publish in response to its ID. A dynamic web page [postID]/web page.js is already created within the stater-files code.
Create a getSinglePost
serve as, very similar to getPosts
, to fetch a unmarried publish the usage of the publish ID handed as a parameter.
async serve as getSinglePost(postId) {
const reaction = watch for fetch(
`${procedure.env.NEXT_PUBLIC_WORDPRESS_API_URL}/posts/${postId}`
);
const publish = watch for reaction.json();
go back publish;
}
Within the dynamic web page element, you extract the publish ID from the URL parameters, name getSinglePost
with this ID, and render the publish’s content material.
const web page = async ({ params }) => {
const publish = watch for getSinglePost(params.postId);
// ... the remainder of the web page code
};
You’ll then populate the web page with the fetched information:
const web page = async ({ params }) => {
const publish = watch for getSinglePost(params.postId);
if (!publish) {
go back Loading...;
}
go back (
{publish.name.rendered}
dangerouslySetInnerHTML={{ __html: publish.content material.rendered }}>
);
};
You’ll get entry to the whole code on our GitHub repository.
Deploying your Subsequent.js software to Kinsta without cost
Kinsta’s Static Website Internet hosting provider provides the facility to host as much as 100 static websites for loose.
This provider hosts simply static information. When you use a static web site generator like Subsequent.js, you’ll be able to configure choices that construct your mission from GitHub and deploy the static information to Kinsta.
Static rendering in Subsequent.js
To allow a static export in Subsequent.js model 13 above, exchange the output
mode inside of subsequent.config.js:
const nextConfig = {
output: 'export',
};
Now, whilst you construct your mission, Subsequent.js is predicted to provide an out folder that incorporates the HTML, CSS, and JavaScript belongings in your software.
From model 13, Subsequent.js has supported beginning as a static web site, then later optionally upgrading to make use of options that require a server. Whilst you use server options, development your pages received’t generate static pages.
As an example, within the dynamic course, you might be fetching those information dynamically. You want in an effort to generate all of the posts statically. This can also be carried out the usage of the generateStaticParams
serve as.
The serve as is utilized in aggregate with dynamic course segments to statically generate routes at construct time as an alternative of on-demand at request time. Whilst you construct, generateStaticParams
runs prior to the corresponding layouts or pages are generated.
In [postID]/web page.js, use the generateStaticParams
serve as to get all posts course:
export async serve as generateStaticParams() {
const reaction = watch for fetch(
`${procedure.env.NEXT_PUBLIC_WORDPRESS_API_URL}/posts`
);
const posts = watch for reaction.json();
go back posts.map((publish) => ({
postId: publish.identity.toString(),
}));
}
Whilst you run the construct command, your Subsequent.js mission will now generate an out listing with the static information.
Deploy Subsequent.js to Kinsta static web site website hosting
Push your codes in your most well-liked Git supplier (Bitbucket, GitHub, or GitLab). Subsequent, apply those steps to deploy your Subsequent.js static web site to Kinsta:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta along with your Git supplier.
- Click on Static Websites at the left sidebar, then click on Upload web site.
- Make a selection the repository and the department you need to deploy from.
- Assign a singular identify in your web site.
- Upload the construct settings within the following structure:
- Construct command:
npm run construct
- Node model:
18.16.0
- Put up listing:
out
- Construct command:
- In the end, click on Create web site.
And that’s it! You presently have a deployed web site inside a couple of seconds. A hyperlink is supplied to get entry to the deployed model of your web site. You’ll later upload your customized area and SSL certificates if you want.
As an alternative choice to static web site website hosting, you’ll be able to choose to deploy your static web site with Kinsta’s Utility Internet hosting provider, which supplies larger website hosting flexibility, a much broader vary of advantages, and get entry to to extra powerful options — like scalability, custom designed deployment the usage of a Dockerfile, and complete analytics encompassing real-time and ancient information. You additionally don’t want to configure your Subsequent.js mission for static rendering.
Abstract
On this article, you’ve got realized easy methods to leverage headless WordPress in a Subsequent.js mission to fetch and show posts dynamically. This means allows the seamless integration of WordPress content material into Subsequent.js programs, providing a contemporary and dynamic internet enjoy.
The opportunity of the headless CMS API extends past simply posts; it permits for the retrieval and control of pages, feedback, media, and extra.
Moreover, website hosting your WordPress CMS along your frontend frameworks doesn’t should be a trouble. With Kinsta’s MyKinsta dashboard, you’re introduced a unified platform for managing your WordPress web pages, programs, databases, and static websites conveniently.
The publish The way to use WordPress as a headless CMS for Subsequent.js seemed first on Kinsta®.
WP Hosting