Jira is a well-liked venture control device that is helping you stay observe of duties inside of a venture. Then again, when running on a big venture, your Jira dashboard can turn out to be cluttered because the choice of duties and group individuals will increase.
To handle this fear, you’ll be able to use the Jira REST API to generate a simplified To-Do Record utility that presentations your assigned duties and their cut-off dates. The API permits you to programmatically have interaction with Jira to create, retrieve, replace, and delete problems and entry consumer knowledge and venture main points.
This educational walks you via growing a to-do record utility with Node.js because the server to fetch problems out of your Jira account and a React utility to show them. You additionally learn to host each the frontend and server to Kinsta.
Necessities
To observe alongside, you want:
- Node.js and Node Bundle Supervisor (npm) put in for your building gadget.
- A Jira account for having access to duties.
- A forged figuring out of Node.js and React.
How To Construct the Backend With Node.js and Specific
Specific is a well-liked Node.js framework that gives a streamlined setting for development server-side packages. Specific simplifies the dealing with of routes and facilitates interactions with exterior sources, comparable to APIs, databases, and frontend packages.
Observe the stairs underneath to arrange the server:
- Create a brand new listing and navigate to it. Then, initialize Node.js through working the command underneath:
npm init -y
This command creates a bundle.json document with the default settings on the root of your app’s folder.
- Subsequent, set up all of the important dependencies in your venture through working the next command:
npm set up specific dotenv axios
The command above installs the next:
- As soon as the set up is a success, create a .env document within the root of your venture and upload the
PORT
quantity:PORT=3000
That is the port quantity the server listens to. You’ll exchange it to a port of your selection.
- Create an index.js document to your venture’s root folder and upload the next code to import Specific, create an example of an Specific utility, and get started your server:
const specific = require('specific'); require('dotenv').config() const app = specific(); const PORT = procedure.env.PORT; // Outline routes right here app.pay attention(PORT, () => { console.log(`Server is working on port ${PORT}`); });
- In any case, to your bundle.json document, upload a script to start out your server:
"scripts": { "get started": "node index" },
Now, you’ll be able to run the beginning script to your terminal:
npm run get started
This command begins your server. You will have to see the next textual content logged within the terminal:
Server is working on port 3000
With the server up and working, you’ll be able to now configure your Jira app.
How To Configure a Jira App
To make use of the Jira REST API, you want to authenticate a consumer account along with your Jira web site. The to-do app API you construct makes use of fundamental authentication with an Atlassian account e-mail cope with and API token.
Right here’s tips on how to set it up:
- Create a Jira account or log in if in case you have one.
- Navigate to the safety phase of your Atlassian profile and click on Create API token.
- Within the conversation that looks, input a Label in your token (e.g., “jira-todo-list”) and click on Create.
- Reproduction the token for your clipboard.
- In any case, retailer the API Token to your .env document:
JIRA_API_TOKEN="your-api-token"
Now, you’ll be able to entry the Jira API the use of fundamental authentication.
Set Up Routes To Fetch Problems From Jira
Now that you’ve got configured a Jira utility. Let’s arrange routes to fetch problems from Jira to your Node.js server.
To begin a request to the Jira API, you will have to use the Jira API token you stored within the .env document. Retrieve the API token the use of procedure.env
and assign it to a variable named JIRA_API_TOKEN
to your index.js document:
const JIRA_API_TOKEN = procedure.env.JIRA_API_TOKEN
Now, you want to outline the endpoint URL in your API request. This URL accommodates your Jira area and a Jira Question Language (JQL) observation. The Jira area refers back to the URL in your Jira group and seems like org.atlassian.internet
, the place org
is your company’s identify. JQL, alternatively, is a question language for interacting with problems in Jira.
Get started through including the Jira area to the .env document:
JIRA_DOMAIN="your-jira-domain"
You additionally wish to retailer your Jira e-mail to the .env document as it might be used for authorization when creating a request to Jira:
JIRA_EMAIL="your-jira-email"
Subsequent, upload each setting variables and assemble the endpoint URL the use of the area and the next JQL observation. This question filters problems with “In development” or “To do” statuses for the logged-in consumer after which orders them through popularity:
const jiraDomain = procedure.env.JIRA_DOMAIN;
const e-mail= procedure.env.JIRA_EMAIL;
const jql = "popularityp.c20inp.c20(%22Inp.c20progressp.c22p.c2Cp.c20p.c22Top.c20dop.c22)%20ORp.c20assigneep.c20p.c3Dp.c20currentUser()%20orderp.c20byp.c20status";
const apiUrl = `https://${jiraDomain}/leisure/api/3/seek?jql=${jql}`;
Earlier than making a path, additionally import Axios into your index.js document:
const axios = require("axios")
You’ll now create a path make a GET request to the Jira API and go back the problems. In index.js upload the next code:
app.get('/problems/all', async (req, res) => {
})
Now, use the axios.get
solution to make a GET request to the Jira REST API. You create the Authorization
header through base64-encoding your Jira e-mail and API token:
const reaction = anticipate axios.get(apiUrl, {
headers: {
Authorization: `Elementary ${Buffer.from(
`${e-mail}:${JIRA_API_TOKEN}`
).toString("base64")}`,
Settle for: "utility/json",
},
});
Anticipate the reaction from the Jira API and put it aside in a variable. The reaction accommodates a belongings referred to as problems
, which holds an array of factor items:
const knowledge = anticipate reaction.json();
const { problems } = knowledge;
Subsequent, iterate over the problems
array, extract best the related details about the to-do goods, and go back it in JSON reaction:
let cleanedIssues = [];
problems.forEach((factor) => {
const issueData = {
identification: factor.identification,
projectName: factor.fields.venture.identify,
popularity: factor.fields.popularity.identify,
closing date: factor.fields.duedate,
};
cleanedIssues.push(issueData);
});
res.popularity(200).json({ problems: cleanedIssues });
If you are making a request to http://localhost:3000/problems/all
, you will have to obtain a JSON object containing the problems:
curl localhost:3000/problems/all
You’ll even take this additional through the use of a supplier like SendGrid and a cron process to ship day-to-day emails containing your assigned duties.
Host Your Node.js Software on Kinsta
Earlier than website hosting your utility on Kinsta, allow Pass-Foundation Useful resource Sharing (CORS) to forestall an access-control-allow-origin
error because you host the backend and the frontend on other domain names. To do that:
- Set up the cors npm bundle through working this command to your terminal:
npm set up cors
- Then, import the bundle in index.js.
const cors = require('cors')
- Subsequent, configure CORS as middleware to allow it for each incoming request. Upload the next code on the height of your index.js document:
app.use(cors());
You’ll now ship HTTP requests for your server from a special area with out encountering CORS mistakes.
Subsequent, push your code to a most popular Git supplier (Bitbucket, GitHub, or GitLab) and observe the stairs underneath to host it:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta along with your Git supplier.
- Click on Packages at the left sidebar, then click on Upload utility.
- Make a selection the repository and the department you need to deploy from.
- Assign a singular identify for your app and make a choice a knowledge middle location.
- Upload the surroundings variables. There’s no wish to upload the PORT as an atmosphere variable, as Kinsta handles it mechanically. Take a look at the packing containers subsequent to To be had all through runtime and To be had all through construct procedure:
Kinsta app setting variables. - Evaluation different knowledge (you’ll be able to depart the default values) and click on Create utility.
Your server is now effectively deployed to Kinsta. At the left-hand menu, click on Domain names and duplicate the main area. That is your server’s URL endpoint.
Create a React Software to Show the Problems
Subsequent, you utilize React to construct your app’s frontend and CSS to taste it. Observe the stairs underneath to create a React venture with Vite:
- Scaffold a brand new React venture named
jira-todo
:npx create-vite jira-todo --template react
- Navigate to the venture listing and set up the important dependencies:
npm set up
- Get started the improvement server:
npm run dev
Fetch Problems From the Server
- Transparent the contents in App.jsx and upload the next code:
serve as App() {
go back (
What is on my record these days?
{/* Show problems */}
);
}
export default App;
- Earlier than you get started fetching the problems, retailer the server URL from Kinsta in a .env document on the root of your app’s folder:
VITE_SERVER_BASE_URL="your-hosted-url"
- Get the URL in App.jsx through including the next line on the height of the document:
const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL
- To your part, create an async serve as named
fetchData
and make a GET request to the/problems/all
endpoint at the Specific server. When you obtain a reaction, parse it as JSON and retailer the knowledge in a state worth namedknowledge
:
import { useCallback, useEffect, useState } from "react";
serve as App() {
const SERVER_BASE_URL=import.meta.env.VITE_SERVER_BASE_URL
const [data, setData] = useState([]);
const fetchData = useCallback(async () => {
take a look at {
const reaction = anticipate fetch(`${SERVER_BASE_URL}/problems/all`);
if (!reaction.adequate) {
throw new Error('Community reaction was once no longer adequate');
}
const knowledge = anticipate reaction.json();
setData(knowledge.problems);
} catch (error) {
console.error('Error fetching knowledge:', error);
}
},[SERVER_BASE_URL]);
useEffect(() => {
// Fetch knowledge when the part mounts
fetchData();
},[fetchData]);
go back (
What is on my record these days?
);
}
export default App;
Notice that you simply use the useEffect
hook to execute the fetchData
serve as when the part mounts.
Render the Problems From Jira within the Browser
- Now, you’ll be able to alter the go back observation of your part to iterate over the problems and record them within the browser:
go back (
What is on my record these days?
{knowledge && knowledge.map(factor => {
go back
{factor.abstract}
{factor.closing date}
className="popularity">{factor.popularity}
})}
);
- To taste this utility, upload the next CSS code to App.css:
h1 {
text-align: middle;
font-size: 1.6rem;
margin-top: 1rem;
}
phase {
show: flex;
flex-direction: column;
justify-content: middle;
align-items: middle;
margin-top: 2rem;
}
.problems {
show: flex;
min-width: 350px;
justify-content: space-between;
padding: 1rem;
background-color: #eee;
margin-bottom: 1rem;
}
small {
colour: grey;
}
.status-btn {
padding: 4px;
border: 1px forged #000;
border-radius: 5px;
}
- Then, import App.css in index.js to use the types:
import './App.css'
Now, while you get started your utility, you will have to see an inventory of duties assigned to you with their popularity and closing date to your browser:
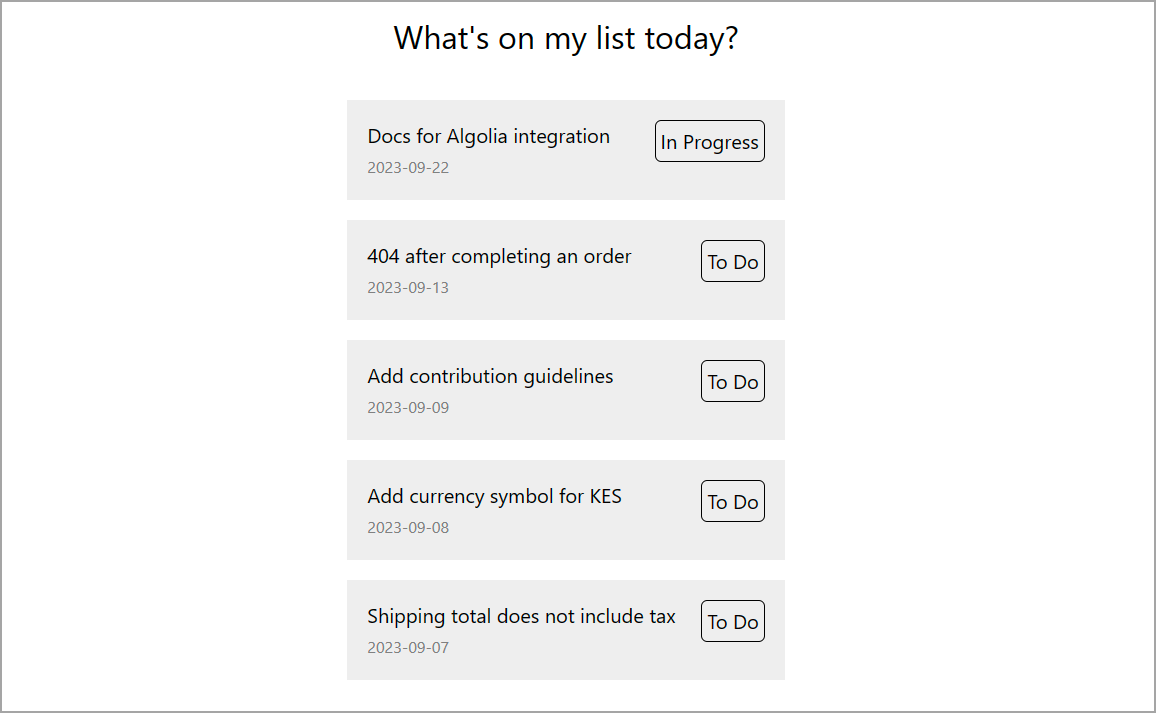
Deploy Your React Software on Kinsta
To stay issues easy, use Kinsta’s Static Web page Web hosting to deploy the applying. Kinsta’s Static Web page Web hosting is helping construct your websites right into a static web site and deploys the static recordsdata making sure swift content material supply and minimum downtime.
Create a repository on GitHub to push your supply code. As soon as your repo is in a position, observe those steps to deploy your static web site to Kinsta:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta along with your Git supplier.
- Click on Static Websites at the left sidebar, then click on Upload web site.
- Make a selection the repository and the department you need to deploy from.
- Assign a singular identify for your web site.
- MyKinsta detects the construct settings for this React venture mechanically. You notice the next settings prefilled:
- Construct command:
npm run construct
- Node model:
18.16.0
- Put up listing:
dist
- Construct command:
- Upload the URL of your server as an atmosphere variable the use of
VITE_SERVER_BASE_URL
. - In any case, click on Create web site.
And that’s it! You presently have a deployed web site inside of a couple of seconds. A hyperlink is equipped to entry the deployed model of your web site. Should you navigate for your web site’s area, you spot an inventory of Jira problems. You’ll later upload your customized area and SSL certificates if you want.
As a substitute for Static Web page Web hosting, you’ll be able to go for deploying your static web site with Kinsta’s Software Web hosting, which gives larger website hosting flexibility, a much wider vary of advantages, and entry to extra powerful options. As an example, scalability, custom designed deployment the use of a Dockerfile, and complete analytics encompassing real-time and ancient knowledge.
Abstract
On this information, you realized tips on how to create an Specific app to retrieve assigned Jira problems the use of the Jira REST API. Moreover, you hooked up a React frontend utility for your Specific utility to show those problems within the browser.
This app is a rudimentary demonstration of what you’ll be able to reach with Jira REST API. You’ll fortify your app with capability that allows you to mark finished duties, carry out complex filtering, and a lot more.
Additionally, with Kinsta, you’ll be able to host each your server and the web site in a single dashboard the use of our more than a few products and services. Take a look at Kinsta’s powerful, versatile internet website hosting for all of your packages.
The submit Create a Kinsta-Hosted To-Do Record The use of the Jira API and React gave the impression first on Kinsta®.
WP Hosting