Within the dynamic nature of contemporary JavaScript, it’s very important to remember the fact that ‘outdated’ doesn’t essentially imply ‘old-fashioned’, and ‘new’ doesn’t at all times suggest ‘higher’.
The important thing to choosing the proper generation lies in its alignment together with your challenge’s wishes. This theory resonates strongly when bearing in mind JavaScript module bundlers. Whether or not a bundler has stood the check of time or is freshly presented, every one comes with distinct benefits and barriers.
This newsletter explores two important and common equipment: Vite and Webpack. We assess those bundlers according to their options, distinctions, architectural philosophies, and the way they combine into the developer ecosystem.
What Is a JavaScript Module Bundler?
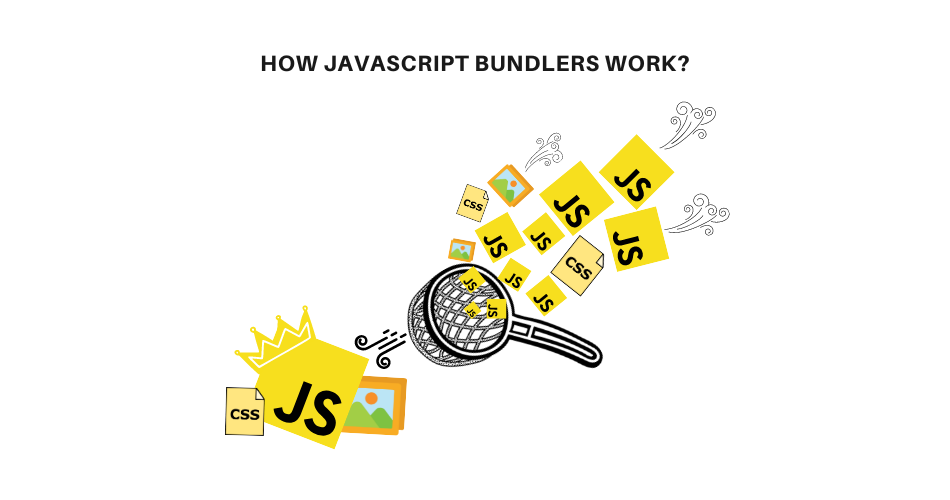
A JavaScript bundler is a device utilized in internet construction to mix more than one JavaScript recordsdata right into a unmarried dossier, referred to as a package deal. It simplifies the control of JavaScript code through decreasing the collection of requests your internet utility must make, in the long run making improvements to functionality.
For example, imagine having two separate JavaScript recordsdata: module1.js and module2.js. module1.js incorporates the next content material:
// module1.js
export const greet = (call) => {
console.log(`Hi, ${call}!`);
}
And module2.js incorporates:
// module2.js
export const farewell = (call) => {
console.log(`Good-bye, ${call}!`);
}
To package deal those modules right into a unmarried dossier, you’ll use a bundler like Rollup, Webpack, or Parcel. As an example, for those who had been to create an index.js dossier inside your challenge listing with the code beneath:
// index.js
import { greet } from './module1.js';
import { farewell } from './module2.js';
greet('Kinsta');
farewell('Server Troubles');
When using a JavaScript bundler, it combines module1.js, module2.js, and index.js right into a unmarried, optimized package deal adapted on your internet utility’s use.
Whilst trendy internet browsers fortify ES modules and applied sciences like HTTP/2, which cope with request overhead considerations, JavaScript bundlers stay indispensable for an array of code improvements. They carry out very important code transformations, together with minification, transpilation, and optimization.
As well as, JavaScript module bundlers be sure that compatibility throughout more than a few browsers. They lend a hand get to the bottom of browser-specific problems and make sure a constant revel in for customers, irrespective of the internet browser they select.
This bundling procedure no longer handiest speeds up your internet utility’s loading velocity but in addition guarantees environment friendly functionality, specifically in manufacturing environments. Now that you recognize JavaScript bundlers and their function in internet construction, let’s shift our center of attention to Vite and Webpack.
Vite and Webpack: Creation and Assessment
It’s transparent that Vite and Webpack lead within the fast-growing box of contemporary internet construction, the place useful resource control and optimized bundles are necessary. However earlier than we delve into an in depth comparability, let’s take a handy guide a rough have a look at those bundlers and perceive what makes them stand out.
Vite: Swift and On-Call for Building
Vite, pronounced “veet,” is a game-changer for internet builders, prioritizing velocity and potency. What makes Vite stand out is its on-demand bundling method. As an alternative of pre-bundling all code and property, Vite leverages local ES modules in trendy browsers, serving code immediately to the browser all through construction. This ends up in nearly rapid Scorching Module Substitute (HMR) and diminished chilly get started occasions.
Vite’s construction server shines with this on-demand method, permitting builders to peer adjustments briefly with out complete recompilation. It additionally makes use of Rollup, for environment friendly manufacturing builds. Because of this, Vite provides lightning-fast construction and forged manufacturing functionality.
Webpack: Arranged and Adaptable
Webpack serves because the cornerstone of contemporary internet construction, often evolving since 2012. What’s nice about Webpack is the way it organizes site elements. It optimizes loading occasions and consumer revel in through organizing code into modules.
The adaptability of Webpack is a exceptional benefit. Builders can customise tasks for easy or complicated duties. It empowers builders to tailor workflows and construct processes with precision.
Similarities and Variations in Vite and Webpack
Now that we’ve grasped the fundamental ideas of Vite and Webpack let’s discover their similarities and variations in additional element. As we analyze those bundlers, we read about more than a few facets to realize a complete figuring out of the way they evaluate and the place every excels.
1. Structure and Philosophy
Each bundlers be offering distinctive views on development and optimizing internet packages. They percentage a commonplace flooring of their plugin method, permitting the neighborhood to create further recommended plugins that stretch their capability, making them flexible equipment for builders.
Vite’s core philosophy revolves round leanness and extensibility. It adheres to a minimalist technique, that specialize in the commonest internet app construction patterns out of the field. This method guarantees long-term challenge maintainability.
Vite’s reliance on a rollup-based plugin gadget prevents core bloating through enabling characteristic implementation thru exterior plugins. This fosters a streamlined core and encourages a thriving ecosystem of well-maintained plugins. Moreover, Vite actively collaborates with the Rollup challenge to deal with compatibility and a shared plugin ecosystem.
Webpack empowers builders with customization, letting them tailor tasks to precise wishes, from elementary duties to complicated endeavors. It provides flexibility in configuring each and every facet of the construct procedure, making it a go-to selection for the ones looking for a personalised construction revel in.
Moreover, Webpack introduces the modular method, very similar to assembling Lego blocks for internet tasks. The entirety for your codebase is a module to Webpack, and it will probably categorical its dependencies in some ways. A couple of examples are:
- ES2015
import
observation. - CommonJS
require()
observation. - AMD
outline
andrequire
observation @import
observation inside a css/sass/much less dossier.- Symbol URL in a stylesheet
url()
or HTML
dossier.
Vite’s Philosophy in Motion
Vite’s architectural philosophy of being lean and extensible is obvious in its solution to development internet packages. Think you’re growing a internet app and need to come with trendy JavaScript options similar to ES modules. With Vite, you’ll achieve this without difficulty. Right here’s a simplified instance:
// app.js
import { greet } from './utilities.js';
const employee = new Employee(new URL('./employee.js', import.meta.url));
// Simulate a calculation within the internet employee
employee.postMessage({ enter: 42 });
employee.onmessage = (e) => {
const outcome = e.information.outcome;
console.log(`End result from the internet employee: ${outcome}`);
};
const message = greet('Hi, Vite!');
console.log(message);
On this code snippet, Vite embraces the usage of ES modules, and it without difficulty bundles the code at the fly, warding off time-consuming bundling steps all through construction. This modular method permits you to organize dependencies successfully, making a maintainable codebase. This showcases Vite’s dedication to minimalism and developer-friendly studies.
Webpack’s Philosophy in Motion
Webpack’s modular philosophy is especially recommended when operating on large-scale tasks. Believe you’re development a considerable internet utility with more than a few JavaScript modules. With Webpack, you’ll seamlessly compile those modules, making improvements to clarity, maintainability, and site loading time. Right here’s a simplified instance:
// webpack.config.js
const trail = require('trail');
module.exports = {
access: './app.js',
output: {
filename: 'package deal.js',
trail: trail.get to the bottom of(__dirname, 'dist'),
},
module: {
laws: [
{
test: /.js$/,
use: 'babel-loader',
exclude: /node_modules/,
},
],
},
};
On this instance, Webpack permits you to configure the construct procedure, optimize code, and deal with property successfully. Via organizing your challenge into modules and the use of loaders like Babel, you’ll write blank, modular code that improves consumer revel in. This demonstrates Webpack’s dedication to offering customization and versatility, making sure builders can tailor their tasks to precise wishes.
Whilst each Vite and Webpack have distinct architectural philosophies, they percentage a commonplace dedication to pushing the limits of contemporary internet construction. Vite specializes in trendy coding patterns, selling ECMAScript Modules (ESM) for supply code and inspiring trendy requirements like new Employee syntax for internet staff.
Webpack, however, advanced as a solution to demanding situations introduced through Node.js and CommonJS, riding the adoption of modules in internet construction. Webpack’s automated dependency assortment, coupled with functionality enhancements, guarantees a continuing developer revel in.
2. Reputation, Group and Ecosystem
Vite and Webpack have distinct timelines, which form their reputation and neighborhood.
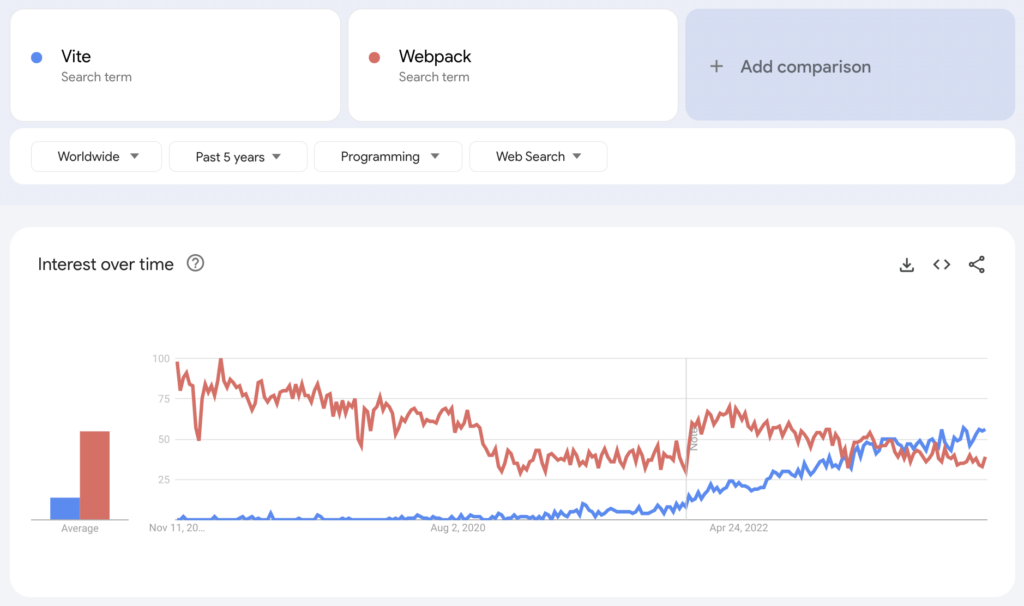
Vite is a newcomer, making its debut in 2020. In spite of its quite temporary life, Vite has unexpectedly received consideration, making it a promising participant within the box of contemporary internet construction.
By contrast, Webpack has a vital head get started, having been established in 2012. Its time within the business has allowed it to increase a mature ecosystem and a strong neighborhood.
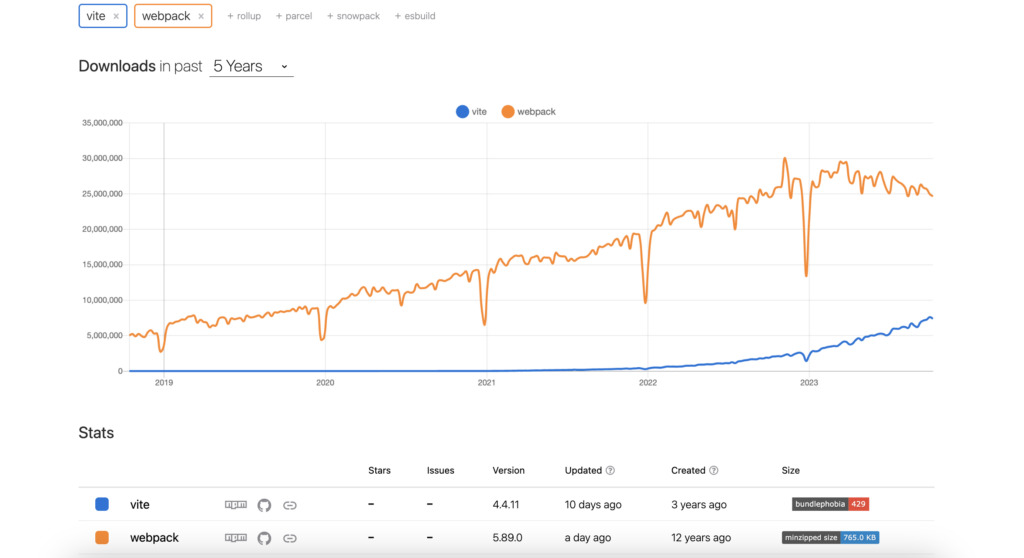
The chart above from npmtrends illustrates the obtain rely comparability between Vite and Webpack. It obviously presentations that Webpack persistently maintains a outstanding place on the subject of obtain rely, emphasizing its long-standing presence and the level of its utilization throughout the developer neighborhood.
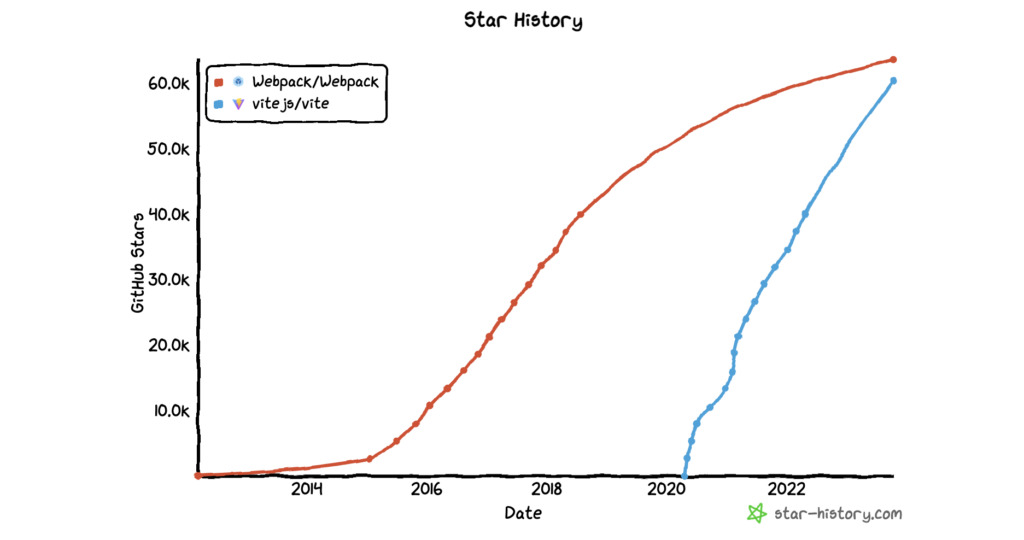
Once we have a look at GitHub stars the use of star-history, which is a measure of recognition and neighborhood fortify, we discover that Vite boasts an excellent 60,318 stars, whilst Webpack maintains a powerful presence with 63,598 stars. Those superstar counts replicate the popularity and lively engagement in each tasks. Vite’s speedy expansion and Webpack’s sustained reputation lead them to precious property throughout the internet construction panorama.
3. Configuration and Ease of Use
Each Vite and Webpack be offering a lot of configuration choices to tailor your package deal consistent with your particular wishes. Then again, there are important variations that deserve your consideration. Let’s discover the configuration and simplicity of use of each equipment.
Vite’s Streamlined Configuration
Vite units itself aside with its zero-config philosophy, designed to simplify your internet construction adventure. This implies you’ll create a elementary Vue 3 part library with minimum fuss. Right here’s a easy Vite configuration for the sort of challenge:
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
export default defineConfig({
plugins: [vue()],
})
Within the above instance, we handiest imported and put in Vite’s legit plugin for Vue.js. Vite’s magic lies in its skill to auto-detect the fitting settings for many tasks.
Webpack’s Configuration Complexity
Webpack, however, has a tendency to require extra detailed configuration. Even if it has moved in opposition to a zero-config method in fresh variations, it’s no longer as automated as Vite. For Vue 3, a elementary Webpack setup may seem like this:
const webpack = require('webpack');
const trail = require('trail');
const { HotModuleReplacementPlugin } = require('webpack');
const { VueLoaderPlugin } = require('vue-loader');
module.exports = {
access: './src/primary.js',
output: {
trail: trail.get to the bottom of(__dirname, './construct'),
filename: 'package deal.js',
},
module: {
laws: [
{
test: /.js$/,
exclude: /(node_modules|bower_components)/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env'],
},
},
},
{
check: /.vue$/,
use: {
loader: 'vue-loader',
},
},
{
check: /.css$/,
use: ['vue-style-loader', 'css-loader'],
},
],
},
get to the bottom of: {
alias: {
vue: 'vue/dist/vue.js',
},
},
plugins: [
new HotModuleReplacementPlugin(),
new VueLoaderPlugin(),
]
};
When in comparison to Vite, Webpack’s configuration comes to extra guide setup. The complexities come with specifying access and output paths, configuring loaders for various dossier sorts, and putting in place plugins for particular functionalities. Let’s destroy down every a part of the configuration and indicate the complexities:
- Access and Output:
access
specifies the access level of your utility, the place Webpack will get started bundling. On this case, it’s set to ./src/primary.js, assuming your utility’s primary JavaScript dossier is within the src listing, whilstoutput
defines the place the bundled recordsdata must be stored. The output trail is resolved the use oftrail.get to the bottom of
, and the ensuing bundled dossier is known as package deal.js and stored within the construct listing. - Module Regulations: The
module.laws
phase defines how several types of recordsdata are processed. On this case, there are laws for JavaScript recordsdata (babel-loader
for transpilation), Vue single-file elements (vue-loader
), and CSS recordsdata (vue-style-loader
andcss-loader
for dealing with kinds). - Alias Configuration: The
get to the bottom of.alias
phase defines aliases for module imports. On this case, it’s configuring an alias for Vue to vue/dist/vue.js. - Plugins: The plugins phase comprises
HotModuleReplacementPlugin
which permits scorching module alternative, a characteristic that permits you to see adjustments with out a complete web page reload all through construction whilstVueLoaderPlugin
is vital for Vue single-file part processing.
To spherical up this phase, Vite sticks out on the subject of ease of use, providing a simplified setup and streamlined construction revel in. Its minimum configuration necessities and use of local ES modules make it nice for learners and speedy construction.
By contrast, Webpack’s in depth configurability, whilst recommended for complicated tasks, can pose demanding situations for newbie builders. The intricate setup and upkeep can decelerate construction, particularly for smaller tasks.
4. Building Server
The improvement server performs a the most important function in a developer’s workflow, influencing potency and productiveness. Let’s evaluate Vite and Webpack, assessing their construction server functionality and usefulness to search out the awesome device on your internet construction challenge.
Server Configuration
Vite sticks out with its integrated, out-of-the-box construction server, frequently getting rid of the desire for in depth configuration.
By contrast, Webpack provides flexibility however calls for further setup. Builders can select choices like Webpack’s Watch Mode, webpack-dev-server
, and webpack-dev-middleware
for automated code compilation upon adjustments. Then again, configuration is normally vital to ascertain and fine-tune those choices.
Chilly Get started Velocity
Conventional bundler-based setups contain keen crawling and necessitate development all of the utility earlier than serving, resulting in noticeable delays, specifically in complicated tasks.
Vite revolutionizes chilly begins with a essentially other method, dramatically decreasing initialization time:
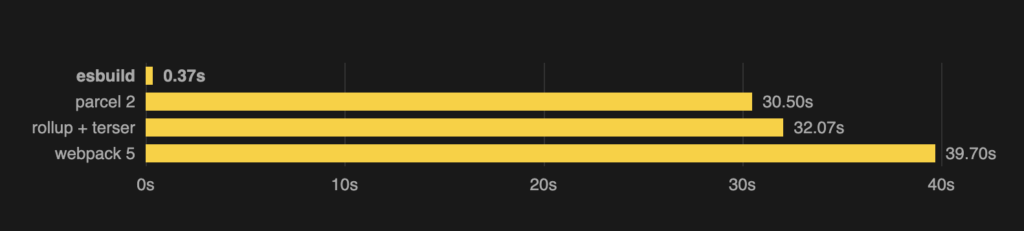
- Environment friendly Dependency Dealing with: Vite leverages esbuild, a high-performance Move-based bundler, to pre-bundle dependencies, together with simple JavaScript and massive modules. All of this considerably contributes to a faster server startup.As part of its pre-bundling procedure, Vite optimizes functionality through merging ESM dependencies with a lot of inner modules right into a unmarried module.As an example, lodash-es incorporates over 600 inner modules. When the use of conventional strategies and uploading a serve as like
debounce
, it triggers 600+ HTTP requests. Vite’s resolution is to pre-bundlelodash-es
right into a unmarried module, decreasing the HTTP requests to only one. This dramatic relief in requests considerably boosts web page load velocity within the construction serverESM founded construction server graph. (Symbol supply: Vite) - On-Call for Supply Code Loading: Vite makes use of local ES modules to serve supply code, minimizing server load and latency. Supply code transformation and serving happen upon browser requests, bettering potency and decreasing wait occasions.
Package founded construction server graph. (Symbol supply: Vite)
Webpack, however, adopts a bundle-based method, pre-bundling supply code and dependencies, extending server get started occasions all through construction. In comparison to Vite’s environment friendly initialization, Webpack’s server setup time is inherently longer.
Then again, Vite’s on-demand loading method can introduce a slight prolong when customers navigate to routes that require further information, CSS, and property. That is specifically noticeable if those sources call for additional bundling steps. Conversely, Webpack’s technique guarantees that every one web page information is to be had, resulting in quicker browser navigation to new pages throughout the construction server.
HMR (Scorching Module Substitute)
Vite employs HMR over local ESM, decreasing server load and latency through offloading some bundling paintings to the browser. This guarantees speedy updates with out full-page reloads, the most important for real-time comments all through construction.
Webpack additionally helps HMR, enabling real-time updates and keeping utility state all through construction. Then again, doable barriers in leveraging local ES modules might result in upper server load and latency.
Caching Efficiency
Caching is very important for making improvements to internet utility functionality, decreasing load and construct occasions through reusing saved property.
Caching in Vite is controlled with a dossier gadget cache, updating dependencies according to adjustments in bundle.json, lockfiles, and vite.config.js. It optimizes web page reloads through caching resolved dependency requests.
Webpack makes use of dossier gadget caching as effectively, clearing changed recordsdata in watch mode, and purging the cache earlier than every compilation in non-watch mode, requiring customized configuration for optimum caching.
To wrap the improvement server comparability, Vite and Webpack be offering distinct approaches to construction servers:
- Vite supplies an out-of-the-box construction server, minimizing configuration overhead.
- Webpack provides configuration flexibility however calls for further setup.
- Vite excels in chilly get started velocity and HMR for speedy code adjustments
- Webpack plays higher in browser navigation velocity because of pre-bundled web page information.
- Each fortify HMR however have other module-serving mechanisms.
- Vite manages native and browser caching seamlessly, whilst Webpack wishes customized configuration.
5. Construct Time and Package Dimension
Now, let’s evaluate the construct time and package deal dimension between Vite and Webpack, bearing in mind construction construct, scorching trade all through dev server, and manufacturing construct.
Our trying out surroundings comes to:
- Working checks on MacBook Air with an Apple M1 chip and 8-core GPU.
- A Vue 3 challenge of medium scale comprising 10 elements, using Vuex for state control and Vue Router for routing.
- Incorporation of stylesheets (CSS/SASS), property like photographs, and fonts, along a average collection of dependencies.
Let’s get started through evaluating bundling time:
Vite [v4.4.11] | Webpack [v5.89.0] | |
---|---|---|
Dev first construct | 376ms | 6s |
Scorching Exchange | Quick | 1.5s |
Prod construct | 2s | 11s |
Vite emerges because the transparent winner in bundling velocity, vastly decreasing construct occasions. Whilst Webpack provides configurability and strong construction equipment, it lags in the back of Vite.
Vite [v4.4.11] (KB) | Webpack [v5.89.0] (KB) | |
---|---|---|
Prod Package Dimension | 866kb | 934kb |
Those figures are according to a medium-sized Vue.js utility with a average collection of dependencies. The true package deal dimension can range relying on challenge complexity, dependencies, and optimization ways.
Vite’s small package deal dimension is because of its environment friendly pre-bundling with esbuild and local ES modules.
Webpack’s package deal dimension can also be optimized thru more than a few configuration choices and plugins, nevertheless it typically produces higher bundles because of its complete bundling procedure.
6. Construct Optimization
On the subject of optimizing the construct procedure in trendy internet construction, Vite and Webpack be offering distinct approaches, every with its personal set of options and functions. Let’s delve into construct optimization through exploring the important thing variations between Vite and Webpack.
Preload Directives Technology
Vite routinely generates directives for access chunks and their direct imports within the constructed HTML. This improves loading occasions through successfully preloading modules as wanted.
So, it should seem like this when examining the web page:
Webpack didn’t natively fortify browser hints for sources. However as of Webpack v4.6.0
, It integrated fortify for prefetching and preloading. The use of an inline directive whilst mentioning imports permits Webpack to output a useful resource trace, which supplies the browser with details about when to load the imported dossier. As an example:
import(/* webpackPreload: true */ '/module-a.js');
This may output:
CSS Code Splitting
Vite sticks out with its streamlined solution to CSS code splitting. It routinely extracts CSS utilized by modules in async chunks and generates separate recordsdata. Because of this handiest the vital CSS is loaded by way of a tag when the related async bite is loaded.
Particularly, Vite guarantees that the async bite is evaluated handiest after the CSS is loaded, fighting Flash of Unstyled Content material (FOUC). Since this selection is pre-configured, you’ll stay uploading your CSS recordsdata with none further steps:
import './primary.css';
Webpack supplies flexibility however calls for extra configuration for CSS code splitting. It permits builders to separate CSS the use of more than a few plugins and configuration choices, similar to mini-css-extract-plugin
.
// Webpack - CSS Code Splitting
const MiniCssExtractPlugin = require('mini-css-extract-plugin');
Code Splitting and Chunks Loading
Code splitting is a basic method used to divide your code into smaller, extra manageable items, loading handiest what’s wanted exactly when it’s wanted. This tradition considerably reduces preliminary load occasions and conserves sources.
Vite’s Way to Chunking
There are circumstances the place Vite makes use of Rollup to separate code into separate chunks routinely, like dynamic loading or more than one access issues, and there’s a solution to explicitly inform Rollup which modules to separate into separate chunks by way of the output.manualChunks possibility.
Excluding Vite’s pre-configured code-splitting characteristic, Vite additionally helps dynamic imports with variables:
const module = watch for import(`./dir/${kinsta}.js`)
Vite additionally permits builders to separate dealer bite the use of the legit splitVendorChunkPlugin()
:
import { splitVendorChunkPlugin } from 'vite'
export default defineConfig({
plugins: [splitVendorChunkPlugin()],
})
With all that dynamic imports and code splitting, it’s commonplace for code to be structured into modules or chunks, and a few of these modules are shared between other portions of a internet utility. Vite acknowledges commonplace chunks and optimizes the loading procedure. To raised perceive this, let’s check out the instance from Vite’s legit documentation.
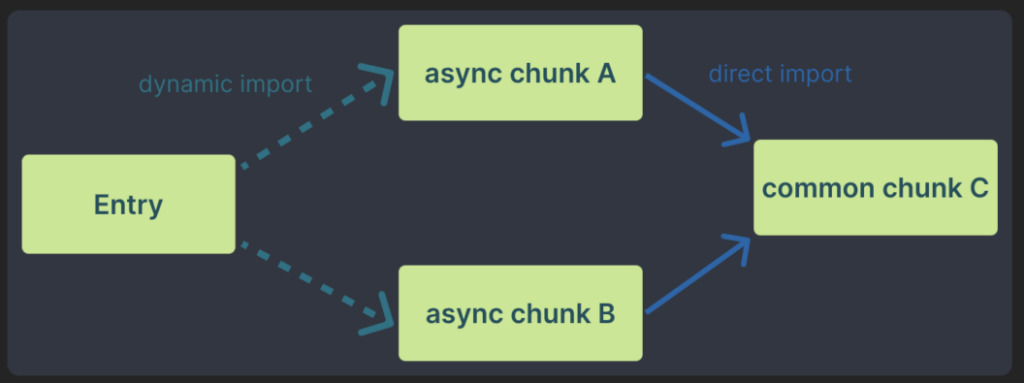
With out optimization, when a consumer opens a bit of a internet utility, let’s name it Phase A that depends upon shared code Commonplace Chew C, the browser begins through fetching Phase A. Whilst parsing Phase A, it realizes it wishes the Commonplace Chew C. This calls for an extra community roundtrip, which is able to decelerate the preliminary web page load:
Access (Phase A) ---> async bite A ---> commonplace bite C
Vite, however, employs a complicated characteristic known as Async Chew Loading Optimization. It doesn’t stay up for the browser to find its wishes; as a substitute, it proactively prepares for them. When a consumer requests Phase A, Vite sends each Phase A and the Commonplace Chew C concurrently. This parallel fetching of required chunks considerably hurries up the loading procedure:
Access (Phase A) ---> (async bite A + commonplace bite C)
Then again, it doesn’t forestall there. Commonplace Chew C may have its personal dependencies, doubtlessly inflicting additional roundtrips in a non-optimized state of affairs. Vite doesn’t omit this facet. It conscientiously analyzes those dependencies, making sure that the whole lot wanted—regardless of its intensity—is successfully loaded directly. This eradicates the need for extra community spherical journeys, making sure a extremely responsive internet utility.
Vite’s async bite loading method optimizes the loading procedure through proactively fetching and serving the entire vital code chunks in parallel. This removing of additional community spherical journeys ends up in a snappier internet revel in. It’s comparable to offering a well-prepared commute itinerary on your browser, making sure it receives all vital sources with out pointless delays.
Webpack’s Way to Break up Code
As for Webpack, There are 3 normal ways to be had for code splitting:
- Access issues: That is the best way to separate a work of code. We will be able to simply outline a brand new access level within the config dossier and Webpack with upload it as a separate bite:
const trail = require('trail'); module.exports = { mode: 'construction', access: { index: './src/index.js', one other: './src/separate-module.js', }, output: { filename: '[name].package deal.js', trail: trail.get to the bottom of(__dirname, 'dist'), }, };
Then again, this method has barriers. If modules are imported in several access chunks, they finally end up in each bundles, resulting in duplicated code. Moreover, it’s no longer very adaptable for splitting core utility common sense when wanted.
- Save you duplication: Some other method is the use of
access
dependencies orSplitChunksPlugin
to separate chunks, which is helping to scale back redundancy.Right here’s an instance of the way you’ll configure code splitting the use ofaccess
dependencies:const trail = require('trail'); module.exports = { mode: 'construction', access: { index: { import: './src/index.js', dependOn: 'shared', }, one other: { import: './src/another-module.js', dependOn: 'shared', }, shared: 'lodash', }, output: { filename: '[name].package deal.js', trail: trail.get to the bottom of(__dirname, 'dist'), }, optimization: { runtimeChunk: 'unmarried', }, };
- Dynamic imports: Finally, Webpack helps dynamic imports, a precious characteristic for on-demand code loading. It makes use of syntax conforming to the ECMAScript proposal for dynamic imports. This technique is extra versatile and granular, making it appropriate for more than a few code splitting situations.
const { default: _ } = watch for import('lodash');
We will be able to additionally use Webpack’s Magic Feedback to set a reputation for the bite, lazy-load it, specify module exports, and set a fetch precedence:
import( /* webpackChunkName: "my-chunk-name" */ /* webpackMode: "lazy" */ /* webpackExports: ["default", "named"] */ /* webpackFetchPriority: "excessive" */ 'module' );
Tree-Shaking
Tree-shaking is a the most important optimization method that each Vite and Webpack use to trim down the dimensions of your JavaScript bundles.
Vite makes use of Rollup, which no longer handiest permits the usage of ES modules but in addition statically analyzes the code you import. Because of this Vite can exclude any portions of a module that you simply don’t use, leading to smaller package deal sizes. As an example, in case you have a module with more than one purposes however handiest use one among them, Vite will come with simply that serve as within the package deal. Right here’s a easy instance:
- With out the use of ES modules, if you wish to import
ajax
from ./utils.js, you would have to import the entire dossier.const utils = require('./utils'); const question = 'Kinsta'; // Use the 'ajax' means of the 'utils' object utils.ajax(`https://api.instance.com?seek=${question}`).then(handleResponse);
- The use of ES modules, however, permits you to import handiest what you want, leading to lighter, quicker, and not more complicated libraries and packages. Since Vite makes use of particular
import
andexport
statements, it will probably carry out extremely efficient tree-shaking with out depending only on an automatic minifier to stumble on unused code.import { ajax } from './utils'; const question = 'Kinsta'; // Name the 'ajax' serve as ajax(`https://api.instance.com?seek=${question}`).then(handleResponse);
Finally, for Vite, we will use Rollup’s pre-configured choices for tree-shaking.
Webpack additionally helps tree-shaking, nevertheless it has a other mechanism. It analyzes the dependencies for your code and eliminates unused portions all through the bundling procedure. Whilst it’s efficient, it might not be as thorough as Vite’s method, specifically when dealing with broad modules or libraries.
Moreover, consistent with Webpack’s documentation. We want to mark the recordsdata as side-effect loose to make sure that it gained’t take away any code that has one other code in manufacturing that depends upon it.
The way in which that is achieved is the sideEffects
bundle.json assets:
{
"call": "kinsta-app",
"sideEffects": false
}
It’s price noting {that a} identical configuration approach to outline unwanted side effects exists in Vite’s Rollup choices as effectively.
7. Static Asset Dealing with
Static property, similar to photographs, fonts, and different recordsdata, are an integral a part of internet construction. Vite and Webpack method the dealing with of those property otherwise, every with its personal strengths and optimizations.
Vite’s Asset Dealing with
Vite’s solution to dealing with static property is streamlined and environment friendly. Whilst you import a static asset, Vite returns the resolved public URL when it’s served. For example, while you import a picture like this:
import kinstaImage from './kinsta-image.png';
All through construction, imgUrl
might be resolved to /img.png
. Within the manufacturing construct, it’ll turn into one thing like /property/img.2d8efhg.png
, optimized for caching and function.
Vite can deal with those imports with both absolute public paths or relative paths, making it versatile on your challenge’s wishes. This conduct extends to URL references in CSS, which Vite handles seamlessly.
Additionally, for those who’re the use of Vite in a Vue Unmarried Record Element (SFC), asset references within the templates are routinely transformed into imports, simplifying your construction workflow.
Vite’s asset dealing with is going even additional through detecting commonplace picture, media, and font dossier sorts, which it treats as property. Those property are integrated as a part of the construct property graph, get hashed dossier names, and can also be processed through plugins for optimization.
Webpack’s Asset Dealing with
Webpack, however, has a special solution to static asset dealing with. With Webpack, you import property such as you typically do:
import kinstaImage from './kinsta-image.png';
Webpack processes this import through including the picture for your output listing and supplying you with the overall URL to the picture. This makes it simple to paintings with property, and it additionally works inside your CSS the use of url('./my-image.png')
. Webpack’s css-loader
acknowledges this as a neighborhood dossier and replaces the trail with the overall picture URL within the output listing. The similar applies when the use of the html-loader
for
.
Webpack’s Asset Modules presented in model 5 can deal with more than a few kinds of property, no longer simply photographs. As an example, you’ll configure Webpack to deal with font recordsdata:
module.exports = {
module: {
laws: [
eot,
],
},
};
This configuration permits you to incorporate fonts for your challenge by way of an @font-face
declaration.
8. Static Websites Give a boost to
Static websites be offering a lot of benefits, similar to speedy loading occasions, stepped forward safety, and simplified web hosting. A static web page consists of HTML, CSS, and JavaScript, offering a streamlined consumer revel in and environment friendly content material supply. Each Vite and Webpack can lend a hand builders generate performant static websites however no longer with the similar potency.
Vite’s Way to Static Website online Technology
Vite provides devoted directions for static web page deployment, capitalizing on its streamlined solution to construction and deployment, specifically fitted to static websites.
Some other cool factor about Vite is that it has a preview
script, which is helping builders release their manufacturing construct in the neighborhood to peer their utility’s ultimate lead to motion. This selection permits builders to check and preview their manufacturing construct earlier than deploying it to a reside server.
Then again, it’s vital to notice that Vite’s preview
script is meant for previewing the construct in the neighborhood and isn’t intended to function a manufacturing server. This implies it’s a useful tool for builders to check their packages earlier than deployment, nevertheless it’s no longer appropriate for web hosting a reside manufacturing web page.
{
"scripts": {
"preview": "vite preview --port 3000"
}
}
It’s price highlighting VitePress, one of the crucial tough equipment within the Vite ecosystem. VitePress is a Static Website online Generator (SSG) to generate fast, content-focused internet sites. VitePress takes your Markdown-based supply textual content, applies a theme, and outputs static HTML pages that may be briefly deployed at no cost on Kinsta.
Webpack’s Way to Static Website online Technology
Whilst Webpack isn’t in particular designed for static web page era, it may be used to create static websites thru more than a few plugins and configurations. Then again, the method is typically extra complicated and not more streamlined in comparison to Vite —Webpack’s number one center of attention lies in bundling and optimizing JavaScript modules, making it an impressive device for development complicated internet packages.
9. Server-Facet Rendering Give a boost to
Server-Facet Rendering (SSR) is a internet construction method that renders internet pages at the server and sends the absolutely rendered HTML to the buyer’s browser. Let’s evaluate the 2 bundlers on the subject of SSR fortify:
- Vite: Vite helps Server Facet Rendering, providing a streamlined method for packages that require SSR. With Vite, front-end frameworks that may run the similar utility in Node.js, pre-render it into HTML, and therefore hydrate it at the shopper aspect can also be seamlessly built-in. This makes Vite a very good selection for packages that call for SSR functions, offering builders with the equipment they want to optimize their server-rendered apps.
- Webpack: Webpack will also be used for Server Facet Rendering. Then again, enforcing SSR with Webpack has a tendency to be extra intricate and calls for a deeper figuring out of configuration and setup. Builders might want to make investments overtime in putting in place SSR with Webpack in comparison to the extra streamlined method introduced through Vite.
10. JSON Give a boost to
Each Vite and Webpack fortify uploading JSON recordsdata. Excluding in Vite, JSON named imports could also be supported to lend a hand with tree-shaking.
// import an object
import json from './instance.json'
// import a root box as named exports.
import { check } from './instance.json'
11. Vue.js and JSX Give a boost to
Vue.js, a outstanding JavaScript framework, follows the SFC (Unmarried Record Element) syntax, simplifying the method of constructing consumer interfaces. By contrast, JSX is a JavaScript syntax extension, essentially utilized in React, enabling builders to outline consumer interface buildings the use of HTML-like tags and parts.
Vite provides firstclass Vue.js fortify with legit plugins that seamlessly combine Vite with Vue. It additionally handles JSX recordsdata (.jsx
and .tsx
) out of the field, due to its esbuild transpilation. Vue.js customers can make the most of the @vitejs/plugin-vue-jsx
plugin, adapted for Vue 3, offering options like HMR, world part answer, directives, and slots.
In circumstances the place JSX is used with different frameworks like React or Preact, Vite permits configuring customized jsxFactory
and jsxFragment
by way of the esbuild possibility. This stage of flexibleness is efficacious for tasks that require JSX customization.
// vite.config.js
import { defineConfig } from 'vite'
export default defineConfig({
esbuild: {
jsxFactory: 'h',
jsxFragment: 'Fragment',
},
})
However, Webpack lacks local fortify for Vue.js or some other particular libraries or frameworks. Builders want to set up related loaders and dependencies to arrange a challenge for a contemporary JavaScript framework, making it a extra guide and doubtlessly complicated procedure.
12. TypeScript Give a boost to
Vite supplies local fortify for TypeScript, enabling seamless incorporation of .ts
recordsdata into tasks. It makes use of the esbuild transpiler for swift code transformation all through construction. Vite’s center of attention is on transpilation, no longer type-checking. It expects your IDE and construct procedure to deal with sort checking.
Webpack lacks local TypeScript fortify, so builders want to manually arrange TypeScript the use of the typescript compiler and the ts-loader
. This calls for configuring tsconfig.json to specify TypeScript choices. As soon as arrange, Webpack makes use of ts-loader
to collect TypeScript code. Whilst this introduces further configuration steps, it supplies flexibility and compatibility with different Webpack options.
13. Glob Import Give a boost to
Vite helps Glob Import. This selection is used to import more than one modules from the dossier gadget by way of import.meta.glob serve as
:
const modules = import.meta.glob('./kinsta/*.js')
This may output:
const modules = {
'./kinsta/isCool.js': () => import('./kinsta/isCool.js'),
'./kinsta/isAwesome.js': () => import('./kinsta/isAwesome.js'),
'./kinsta/isFun.js': () => import('./kinsta/isFun.js'),
}
Vite additionally has fortify for Glob Import As, to import recordsdata as strings through the use of import.meta.glob
. Right here’s a code instance:
const modules = import.meta.glob('./kinsta/*.js', { as: 'uncooked', keen: true })
Which might be remodeled into this:
const modules = {
'./kinsta/rocks.js': 'export default "rocks"n',
'./kinsta/laws.js': 'export default "laws"n',
}
{ as: 'url' }
could also be supported for loading property as URLs.
Whilst Webpack calls for further plugins like webpack-import-glob-loader
and glob-import-loader
to accomplish Glob Imports. They’ll amplify this:
import modules from "./check/**/*.js";
Into this:
import * as moduleOne from "./foo/1.js";
import * as moduleTwo from "./check/bar/2.js";
import * as moduleThree from "./check/bar/3.js";
var modules = [moduleOne, moduleTwo, moduleThree];
14. Internet Staff Give a boost to
Internet Staff are very important for operating heavy duties within the background with out freezing the primary internet web page. Right here’s how Vite and Webpack deal with them:
Vite makes it simple to make use of Internet Staff. You create a separate Internet Employee dossier, import it into your primary code, and keep in touch with it. Vite provides two tactics to import a employee for your primary code:
new Employee()
and newSharedWorker()
constructors:const employee = new Employee(new URL('./employee.js', import.meta.url)); // OR const employee = new SharedWorker(new URL('./employee.js', import.meta.url));
- At once imported through appending
?employee
or?sharedworker
:import MyWorker from './employee?employee'; const employee = new MyWorker(); myWorker.postMessage('Hi from the primary thread!');
Webpack additionally helps Internet Staff, and ranging from Webpack 5, we’re no longer required to make use of a loader to make use of staff.
Const employee = new Employee(new URL('./employee.js', import.meta.url));
15. Library Building Capacity
Libraries and frameworks empower builders to create and percentage equipment that boost up the improvement of internet packages. Each Vite and Webpack be offering tough answers.
Vite takes library construction to the following stage with its specialised Library Mode, simplifying the method of constructing browser-focused libraries. Moreover, Vite supplies the versatility to externalize particular dependencies, like Vue or React, which you will desire to not come with inside your library package deal.
Webpack, however, is a flexible bundler that caters to library authors as effectively. In case you’re the use of Webpack to create a JavaScript library, you’ll configure it to fit your library bundling distinctive wishes. It permits you to outline how your library’s code must be packaged, making it an acceptable selection for development quite a lot of libraries.
16. Browser Compatibility
Vite prioritizes trendy browsers, focused on the ones with local ES Modules fortify, similar to Chrome >=87, Firefox >=78, Safari >=14, and Edge >=88. Customized objectives will also be set by way of construct.goal
, beginning at es2015. Legacy browsers are supported thru @vitejs/plugin-legacy
.
Webpack helps all ES5-compliant browsers (aside from IE8 and beneath). To deal with older browsers, polyfills are required for options like import()
and require.be sure that()
.
On the subject of browser compatibility, each are nice, however your selection must rely on your challenge’s target market and their browser functions.
Abstract
Vite provides lightning-fast construction with fast updates and in depth customization choices due to its local ES module method. Conversely, Webpack, recognized for its robustness and wide ecosystem, excels in manufacturing builds however calls for a steeper finding out curve.
When opting for between Vite and Webpack, imagine challenge wishes and your familiarity with configuration intricacies. Each have their benefits, so pick out according to your challenge’s particular necessities.
After all, In case you’re bearing in mind web hosting your Vite-powered tasks, you’ll discover Kinsta’s Static Website online web hosting, which provides a strong and environment friendly resolution for internet builders.
Percentage your most well-liked bundler and key issues guiding your variety within the feedback phase beneath.
The submit Vite vs. Webpack: A Head-to-Head Comparability seemed first on Kinsta®.
WP Hosting